What is Selection Sort in Java?
The Selection Sort In Java Program algorithm selects the smallest element in the array and swaps it with the array’s first element.
The array’s second smallest element is then swapped with the second element, and vice versa.
In each iteration of the selection sort algorithm, the smallest element from an unsorted list is chosen and placed at the beginning of the unsorted list.
Implementation of a Selection Sort
Let’s talk about how this selection sort works.
- Make the first element the most minimal.
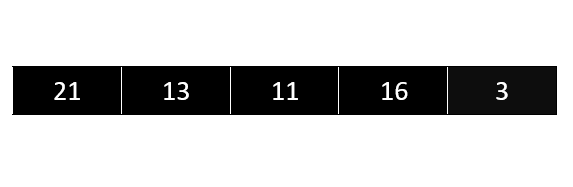
- When comparing the first and second elements, keep in mind that the first is the most important. Assign the second element as the minimum if it is smaller than the first.
Consider the third factor in comparison to the minimum.
Assign a minimum to the third element if it is smaller; otherwise, do nothing. The procedure continues until the last element.
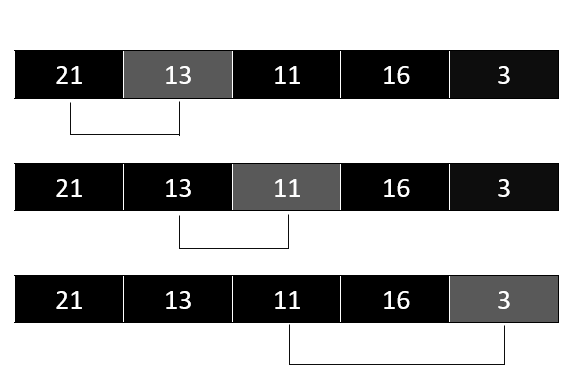
- After each iteration, the minimum is moved to the front of the unsorted list.
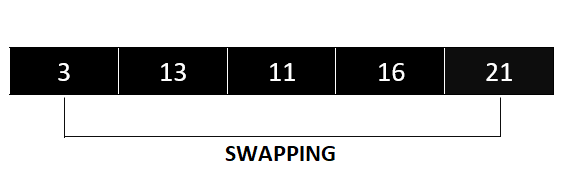
- Indexing begins with the first unsorted element for each iteration. Repeat steps 1–3 until all of the elements are in their proper places.
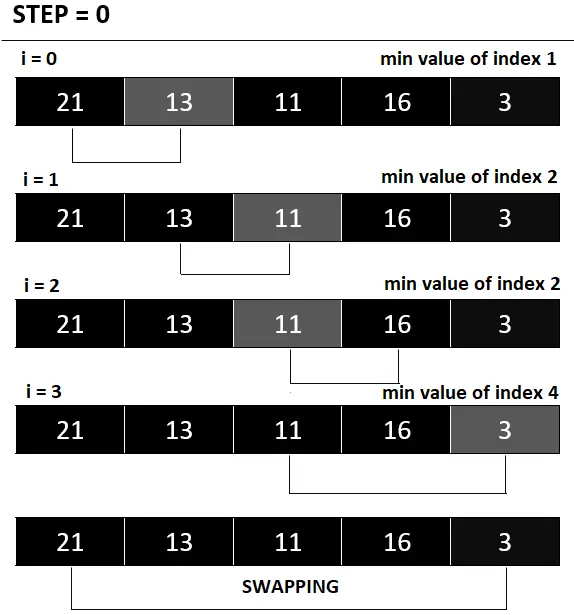
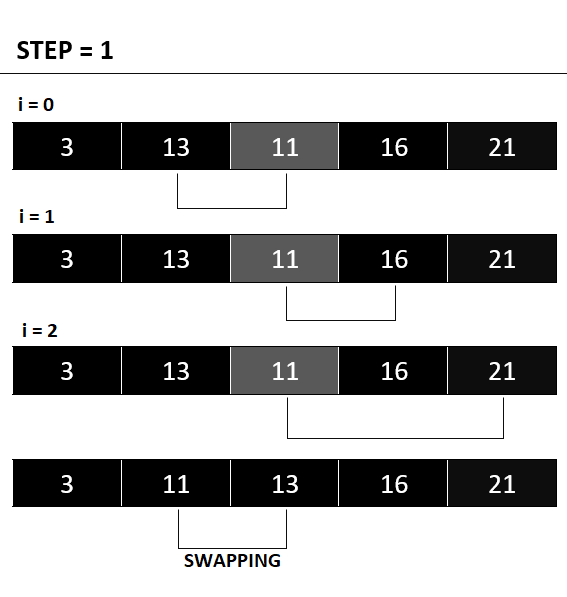
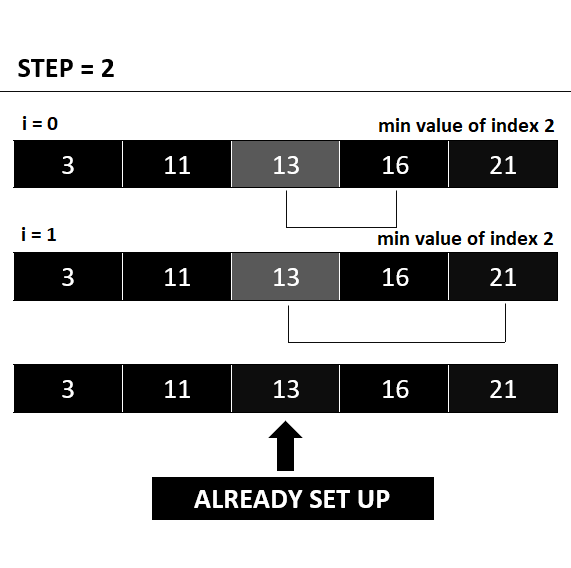
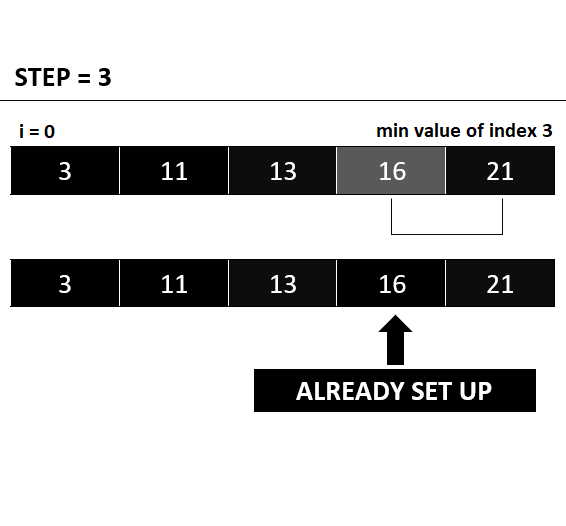
In the fourth iteration, you can see that the iteration is finished.
From the left side of all the elements, the numbers go from lowest to highest (3, 11, 13, 16, 21).
This means that the Selection Sort is complete and in perfect order.
Algorithm of Selection Sort
selection_sort(array, size)
repeat (size - 1) times
set the first unsorted element as the minimum
for each of the unsorted elements
if element < current_minimum
set element as new minimum
swap minimum with the first unsorted position
end selection_sort
You can test the above example here! ➡Java Online Compiler
Sample Java Code using Scanner
A Scanner is a class in the Java Utilities package that is used to get the input of primitive types like int, double, etc., and strings.
It is the easiest way to read input into a Java program
package selectionsort_in_java; import java.util.Scanner; public class SelectionSort_in_Java { public static void Sort(int a[]) { int n = a.length, i, j, p, temp; for (i = 0; i < n - 1; i++) { p = i; for (j = i + 1; j < n; j++) { if (a[p] > a[j]) { p = j; } } temp = a[p]; a[p] = a[i]; a[i] = temp; } } public static void printarray(int a[]) { for (int i = 0; i < a.length; i++) { System.out.print(a[i] + " "); } } public static void main(String[] args) { int n, res, i; Scanner s = new Scanner(System.in); System.out.print("Enter number of elements in the array:"); n = s.nextInt(); int a[] = new int[n]; System.out.println("Enter " + n + " elements "); for (i = 0; i < n; i++) { a[i] = s.nextInt(); } System.out.println("elements in array "); printarray(a); Sort(a); System.out.println("\nelements after sorting"); printarray(a); } }
Selection Sort Example Output Using Scanner
Enter number of elements in the array:Enter 5 elements
elements in array
21 13 11 16 3
elements after sorting
3 11 13 16 21
You can test the above example here! ➡Java Online Compiler
Sample Java Code using Array
An Array is a container object that holds a fixed number of values of the same type.
The length of an array is set when the array is made. After it is made, its length is fixed.
// Selection sort in Java by Glenn Magada Azuelo import java.util.Arrays; public class SelectionSort { void selectionSort(int array[]) { int size = array.length; for (int step = 0; step < size - 1; step++) { int min_idx = step; for (int i = step + 1; i < size; i++) { // To sort in descending order, change > to < in this line. // Select the minimum element in each loop. if (array[i] < array[min_idx]) { min_idx = i; } } // put min at the correct position int temp = array[step]; array[step] = array[min_idx]; array[min_idx] = temp; } } // driver code public static void main(String args[]) { int[] data = { 21, 13, 11, 16, 3 }; SelectionSort ss = new SelectionSort(); ss.selectionSort(data); System.out.println("Sorted Array in Ascending Order: "); System.out.println(Arrays.toString(data)); } }
Selection Sort Example Output Using Array
Sorted Array in Ascending Order:
[3, 11, 13, 16, 21]
You can test the above example here! ➡Java Online Compiler
Anyway, if you want to level up your programming knowledge, especially Java, try this new article I’ve made for you Best Java Projects With Source Code For Beginners Free Download.
Conclusion
So far, we’ve already discussed the Selection Sort algorithm for sorting in Java.
We also looked at the algorithm and a detailed example of how to use the Selection Sort technique to sort an array using a scanner.
Then we put the Java program to work on the Selection Sort. I hope this Selection Sort tutorial helps you a lot in learning Java development.
Related Sorting Algorithm in Java
Inquiries
If you have any questions or suggestions about the Quicksort, please feel free to leave a comment below.