What is Encapsulation in Java?
Encapsulation is an important part of object-oriented programming (OOP).
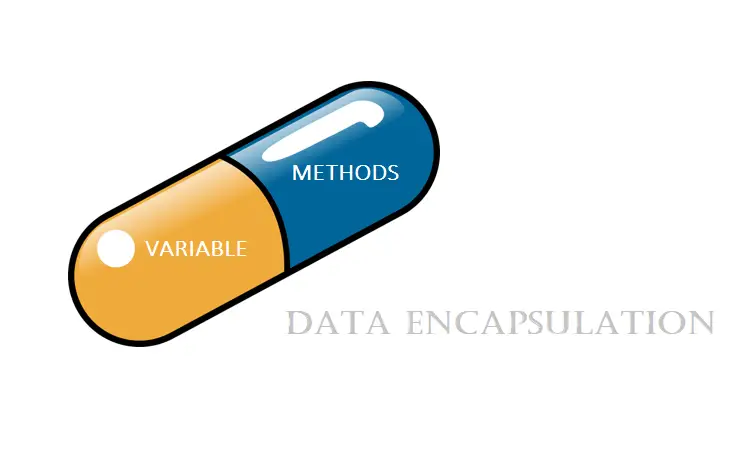
Java Encapsulation is the idea of putting data and methods that work on that data into a single unit, like a Java class.
This idea is also often used to hide from the outside what an object looks like or how it works on the inside.
This is called Data Hiding.
For instance, an object might possess a quality that is hidden from the outside.
You put it in a package with methods that let you read or write to it.
Encapsulation lets you hide certain information and control who can see what’s going on inside an object.
How do you achieve Encapsulation in Java?
- Set class to private variables
- Make public setter and getter methods available for modifying and viewing variable values
Example
The following is an example of how to achieve Encapsulation in Java:
// program created by Glenn
public class EncapTest {
private String name;
private String idNum;
private int age;
public int getAge() {
return age;
}
public String getName() {
return name;
}
public String getIdNum() {
return idNum;
}
public void setAge( int newAge) {
age = newAge;
}
public void setName(String newName) {
name = newName;
}
public void setIdNum( String newId) {
idNum = newId;
}
}
The public setYYY() and getYYY() methods of the EncapTest class can be used to get to the instance variables.
These methods are usually called “getters” and “setters.” So, if a class wants to use a variable, it should use these getters and setters.
The following code can be used to access the EncapTest class variables:
public class RunEncap {
public static void main(String args[]) {
EncapTest encap = new EncapTest();
encap.setName("Glenn Magada Azuelo");
encap.setAge(26);
encap.setIdNum("AGM021596");
System.out.print("Name : " + encap.getName() + " Age : " + encap.getAge());
}
}
Output:
This will result in the following. Name : Glenn Magada Azuelo Age : 26
Benefits of Encapsulation in Java
The following are the benefits of encapsulation in Java. Here’s what they are:
- The code that is encapsulated is more flexible and easier to change to meet new needs.
- It keeps the private fields from being accessed by other classes.
- Modifying implemented code is made possible by encapsulation without affecting other implemented code.
- It prevents unauthorized usage of data and codes. Encapsulation improves safety.
- It makes the application easier to maintain.
- The fields can be turned read-only if the setter method is not defined in the class.
- The fields can be made write-only if you don’t define the getter method in the class.
Tell me the meaning of Encapsulation
The term Encapsulation refers to the grouping together of data and the methods that manipulate that data in object-oriented computer programming OOP concepts.
Encapsulation is frequently used in several programming languages in the form of classes.
Example of Encapsulation in Java
In Java, encapsulation is the technique of combining code and data into a single entity.
For instance, a capsule containing a variety of medications. In Java, we can make a class that is completely closed off by making all of its data classes private.
Data Hiding vs. Encapsulation in Java
In encapsulation, the variables of the class are kept hidden from other classes and can only be accessed through its methods.
It is because of this, it is also called data hiding. Set a class variables to be private.
They have public setter and getter methods that let you change and look at the values of variables.
Encapsulation in Java
In Java, encapsulation is a way to treat data (variables) and the code that works on the data (methods) as a single unit.
In encapsulation, class variables are kept hidden from other classes, and they can only be accessed through its methods.
Data hiding in Java
In Java, you can do this by using the private keyword. This is called data hiding.
It is used for security so that no one can get to internal data without it.
An end user who is not authorized will not be able to access internal data.
Why Encapsulation?
- Encapsulation helps us keep fields and methods that are related together in Java. This makes our code cleaner and easier to read.
It helps us keep track of our data.
For example:
class Level {
private int max;
public void setMax(int max) {
if (max >= 0) {
this.max = max;
}
}
}
- Here, we make the max variable private and use logic within the setMax() method. The max is no longer negative.
- The getter and setter methods allow us to access our class fields in either read-only or write-only mode.
For example:
setName() // provide write-only access getName() // provide read-only access
- It makes it easier to separate parts of a system. For example, we can put code into more than one bundle.
These separate parts (bundles) can be built, tested, and fixed independently and at the same time. And changes to one part have no effect on the other parts.
- Encapsulation is another way to hide data. In the example above, if we make the length and breadth variables private, then only certain users have access to these fields.
They are also kept hidden from the upper classes. This is known as data hiding.
What are the three types of encapsulation?
The 3 types of encapsulation are listed below.
- Member variable encapsulation
- Function encapsulation
- Class encapsulation
Member variable encapsulation
In Object-Oriented Programming, all of the data members that are accessed within the Class in Java should be declared as private.
Setters and Getters functions should be used by any object that wants to change or get the value of a data member.
You may already know this as Data Member Encapsulation.
Here is an example of how setters and getters are declared in a class:
class Rectangle {
private:
//private data member
int diameter;
public:
//constructor
Rectangle();
~Rectangle();
//setter function
void setDiameter(float uValue);
//getter function
float getDiameter();
};
However, there are other ways to encapsulate data except with data members.
Functions and classes may also be encapsulated.
Member variable encapsulation
Private must always be used for API functions that are only used internally.
For example, the following class is a Rectangle:
class Rectangle {
private:
//private data member
int diameter;
//private method
void calculateCircumference();
public:
//constructor
Rectangle();
~Rectangle();
//setter function
void setDiameter(float uValue);
//getter function
float getDiameter();
};
We have a setter method that changes the Diameter of a rectangle.
This should be made public method because the user will provide this data.
But the CalculateCircumference() method shouldn’t be made public.
This method shouldn’t be available to the user. Instead, it should be marked as a Private method.
Always keep in mind that you should be hiding the implementation details in any functions that the public does not need to see.
Class encapsulation
The same rule applies to classes that are used to build your API from the inside.
These classes shouldn’t be included in any API’s public interface. They should be Private and hidden from your users.
For example, your API might have a class that sets a rectangle gradient color based on where it is placed.
If your user doesn’t need access to this Gradient class, you should wrap it up, or declare it as a Private class, as shown below:
class Rectangle {
private:
//private data member
int diameter;
//private method
void calculateCircumference();
//private class
class Gradient{
public:
Gradient();
~Gradient();
void setGradient(float uValue);
};
public:
//constructor
Rectangle();
~Rectangle();
//setter function
void setDiameter(float uValue);
//getter function
float getDiameter();
};
Summary
In summary, encapsulation in Java involves grouping data and its related methods within a class.
This helps hide the internal details of an object, promoting data security and better code organization.
By setting variables as private and providing public getter and setter methods, encapsulation allows controlled access to the data.
The benefits include flexibility, code safety, and improved maintenance.
The article also introduces three types of encapsulation: member variable, function, and class encapsulation.
I hope this lesson has helped you learn a lot.
What’s Next
The next section talks about Interfaces. At the end of the session, you’ll know what is Interfaces all about in Java.
< PREVIOUS
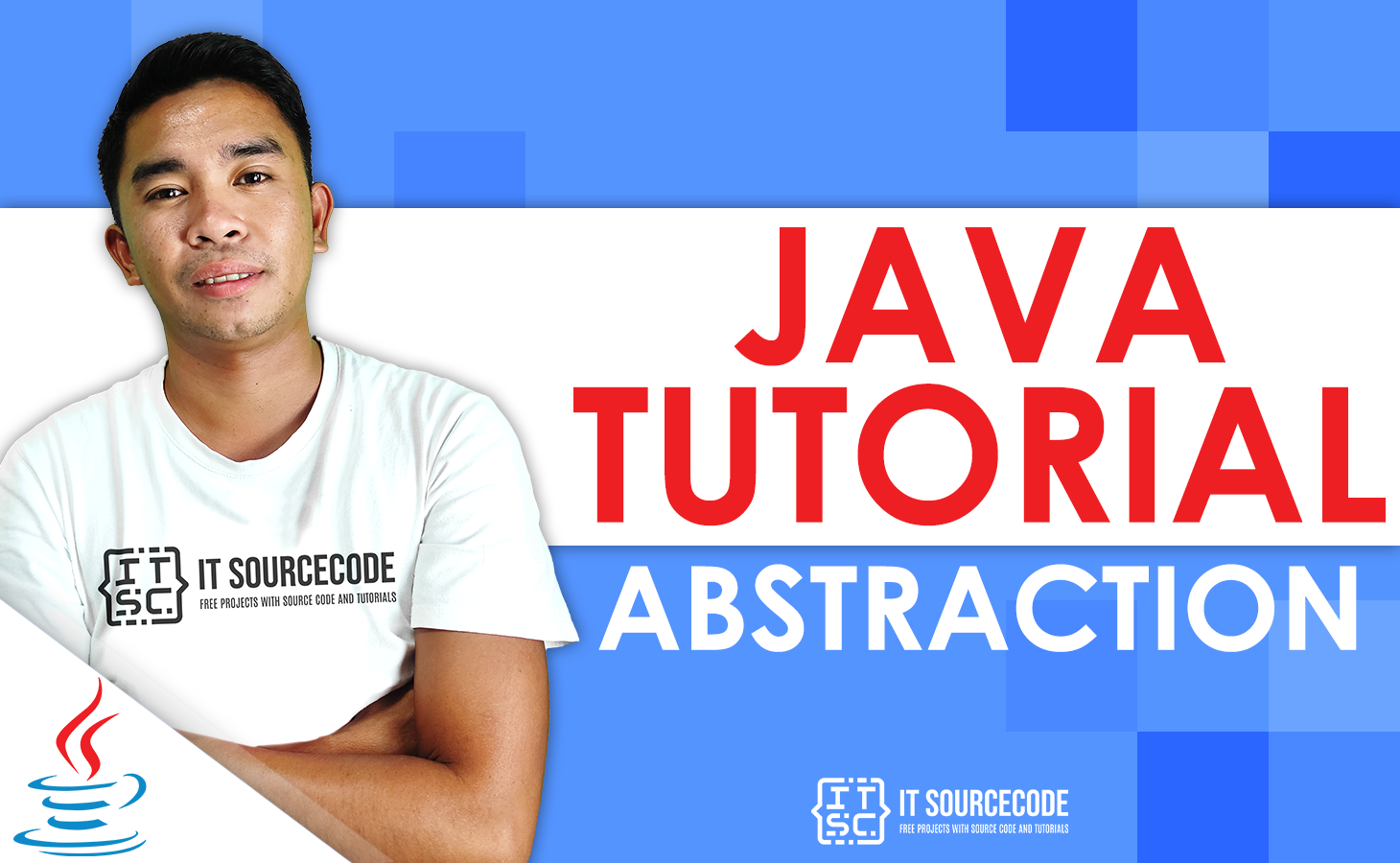
Java Abstraction
NEXT >