What is Python Programming?
- Python Programming is a high-level, interpreted, object-oriented programming language with a lot of flexibility.
- Python interpreter executes each line of code one by one, making it easy to debug.
- Python has a short, easy-to-learn syntax that puts readability first. This reduces the cost of software maintenance.
- Python works with modules and packages, which makes it easier to split up programs and reuse code.
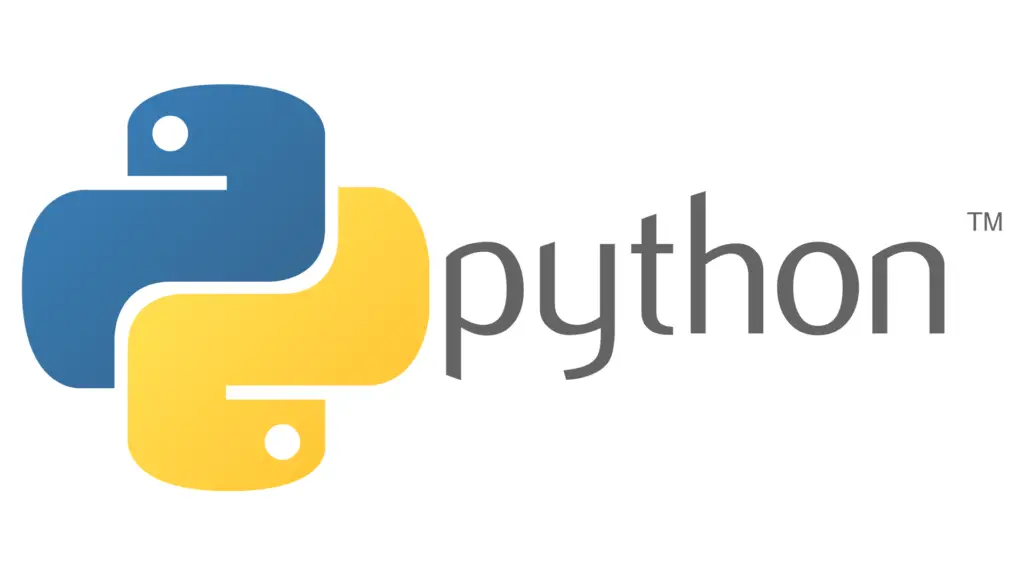
By the way, Python has web development frameworks like Django, Flask, and Web2Py.
If you want to know more about these frameworks you can check our Best Python Frameworks For Web and Software Development.
Python Programming Basics
Python programming employs new lines to complete a command, rather than using semicolons or parentheses like other programming languages.
Python relies on indentation, employing whitespace, to specify a scope; such as the scope of loops, functions, and classes. Other programming languages typically employ curly brackets for this reason.
Example 1:
# printing in Python
print("Hello, Python!")
# solving simple equations
a = 1
b = 5
c = a+b
print(c)
# loops
nation = ["India", "Philippines", "Pakistan"]
for x in nation:
print(x)
Output:
Hello, Python!
6
India
Philippines
Pakistan
Why we should learn Python Programming?
- Learning Python Programming is the best choice for beginners and experts alike since it is simple to use, strong, and versatile.
- Python is considered one of the most beginner–friendly languages.
- Python is a wonderful language for beginners because it’s understandable and does not have difficult syntax.
- Python is used in advanced areas of computer science, like Artificial Intelligence, Machine Learning, Natural Language Generation, and Neural Networks.
Characteristics of Python Programming
Here are some important Characteristics of Python Programming:
- It works with OOP as well as functional and structured programming.
- It can be used as a scripting language or compiled into byte code to build large applications.
- It has very high-level dynamic data types and supports dynamic type checking.
- It can operate on its own to gather trash.
- It is simple to connect to C, C++, COM, ActiveX, CORBA, and Java.
To get you interested in Python Programming, I’m going to give you a simple Hello World program. If you are interested, you can also try our Python Compiler.
Example 2:
print ("Hello World using Python!")
Output
Hello World using Python!
Congratulations, you have just completed your first Python program.
As can be seen, this task was not particularly difficult. This is why the Python programming language is so great.
Advantages of Python Programming
Here are the advantages of Python Programming:
- Easy-to-learn: Simple-to-learn Python has a simple structure, few keywords, and a clear syntax.
- Easy-to-read: Python-written code is easier to read and comprehend.
- Easy to maintain: The source code for Python is pretty easy to keep up with.
- A broad standard library: Most of Python’s library is easy to move around and works on UNIX, Windows, and Macintosh.
- Interactive Mode: Python has a mode called “interactive” that lets you test and fix bits of code in real time.
- Portable: Python can run on a wide range of hardware platforms, and all of them have the same interface.
- Extendable: Python interpreter can be extended with low-level modules. By doing so, programmers can customize their tools by adding to them or making changes to them.
- Databases: Python provides interfaces to all major commercial databases.
- GUI Programming: Python lets you create GUI programs that can run on Windows, Macintosh, and Unix.
- Scalable: Python is better at building and supporting large programs than shell scripting.
Python Syllabus
This lesson is for people who want to learn how to program in Python. It covers the basics of the language, with examples specifically for beginners.
Lesson 1 | Python – Introduction |
Lesson 2 | Python – Overview Know the history and the difference between Python 2 and Python 3. |
Lesson 3 | Python – Environment Setup Windows, Linux, and macOS installation. |
Lesson 4 | Python – Basic Syntax Create Your First Python Program and Learn the basics of Python. |
Lesson 5 | Python – Variable Types Learn how to assign variables and their types like numbers, strings, lists, etc. |
Lesson 6 | Python – Operators Learn the types of operators in Python, their syntax, and how to utilize them with examples. |
Lesson 7 | Python – Decision-Making Statements Learn the different types of decision-making statements with examples. |
Lesson 8 | Python – Loops Learn the different types of loops: for loops, while, and nested loops with examples. |
Lesson 9 | Python – Numbers If you want to know the mathematical, trigonometric, and random number functions. |
Lesson 10 | Python – Strings Learn the split, replace, join, reverse, uppercase, lowercase and etc. |
Lesson 11 | Python – Lists Learn how to use lists in Python and its functions and methods. |
Lesson 12 | Python – Tuples Learn what is a tuple and how to create, access, and update its values. |
Lesson 13 | Python – Sets Learn the set operations and their functions and methods. |
Lesson 14 | Python – Dictionaries Learn if the dictionary is mutable or immutable, and how to access its values. |
Lesson 15 | Python – Built-in Functions Learn how the built-in functions in Python work in an actual program. |
Lesson 16 | Python – Module Learn the differences between module, package, and library. |
Lesson 17 | Python – File Handling Learn how to handle the file in Python with examples. |
Lesson 18 | Python Exception Handling Learn what is exception and how to handle different errors in Python. |
Audience
This tutorial in Python was developed for a specific target, namely software developers who are just starting out and wish to master the Python programming language.
This Python tutorial will help you learn the basics of Python and move on to more advanced topics.
Download Python Tutorial for Beginners PDF
You can download the Python Tutorial for Beginners PDF file by clicking the download button below:
Summary
To sum up, Python Tutorial covers its characteristics, how to construct a “Hello World” program, and the target audience. You should now have a good understanding of what Python is and why it is useful.