What is Loops in Java?
The Loops in Java is a set of instructions to be run repeatedly until a certain condition is met.
Loops are also called iterating statements or looping statements.
What are the different types of Loops in Java?
There are three types of Loops In Java: the while loop, the do-while loop, and the for loop.
All three of these Java loop structures repeat a set of statements as long as a given condition is true.
This kind of situation is usually called “loop control.“
- while loop
- for loop
- do while loop
There may be times when you need to run the same block of code more than once. Statements are usually run in order.
The first statement in a function is run first, then the second, and so on.
Programming languages have different control structures that let you make execution paths that are more complicated.
A Loop Statement allows us to run a statement or group of statements more than once.
This is the basic form of a loop statement in most programming languages.
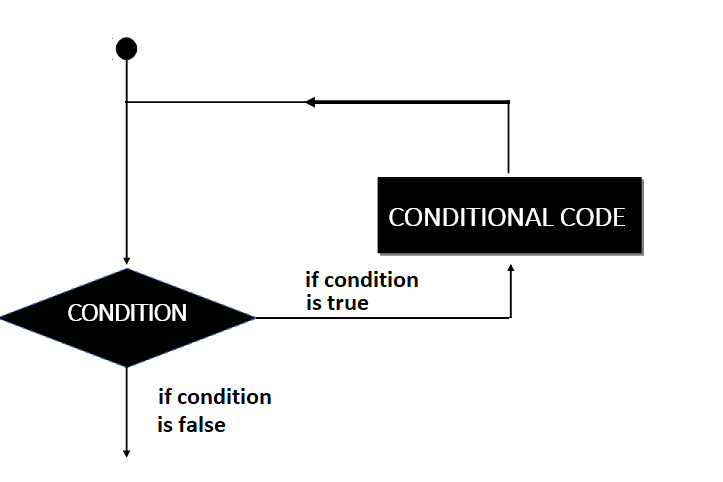
Java Programming Language has the following types of loops to handle looping needs.
Loop | Description |
while loop | Repeats a statement or group of statements as long as a given condition is true. Before running the loop body, it checks to see if the condition is true. |
for loop | Repeats a series of statements and shortens the code that controls the loop variable. |
do while loop | Like a while statement, but at the end of the loop body, it checks the condition. |
While Loop In Java
The While Loop In Java is a control flow statement that lets code run over and over again based on a Boolean condition.
The while loop is like an if statement that is repeated. When we need to run a block of statements over and over, we use the “while” loop in Java.
Syntax Of While Loop
while(test-programs)
loop-body
In a while loop, the loop body can be a single statement, a group of statements, or nothing at all.
The loop will keep going as long as the test expression or condition is true.
When the expression is no longer true, the control of the program moves to the line right after the end of the code in the loop body.
In a while loop, you have to set up a loop variable before the loop starts.
The loop variable should be changed inside the body of the while loop.
Example Program of While Loop:
//program by Glenn Magada Azuelo
public class WhileLoopDemo
{
public static void main(String args[])
{
long i = 0, fact = 1, num = 6 ;
i = num ;
while(num != 0)
{
fact = fact * num;
--num;
}
System.out.println("The factorial of " + i + " is: " +fact);
}
}
Output:
The factorial of 6 is: 720
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
You can test the above example here! ➡Java Online Compiler
In the code above, the loop body, which is the variable, is repeated as long as the value of num is not zero.
Every time the value of fact is changed by multiplying it by num, the next step is to lower the value of num.
And then the test-expression (num) is run once more. If it is false, the loop stops. If it is true, it starts over.
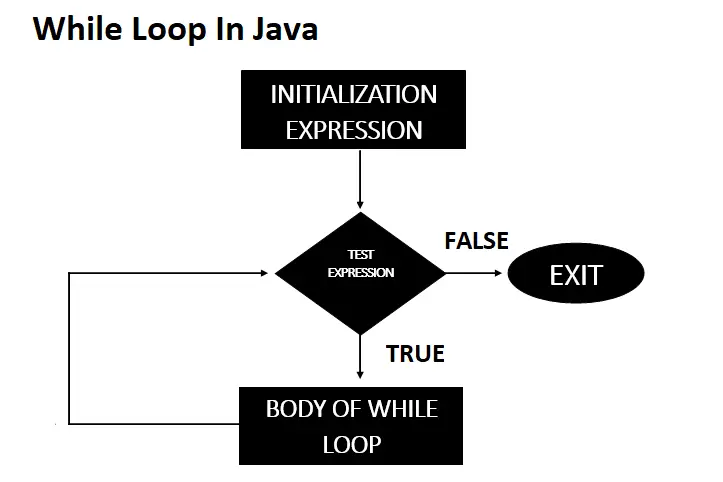
While Loop Variations
There are also different kinds of while loops. We will talk about each of these variations.
Empty While Loop
The body of an Empty While Loop does not contain any statements.
It only has a null statement, which is denoted by a semicolon after the while statement.
:
long wait = 0;
while( ++wait < 20000)
; //null statement
:
A time delay loop is what the code above does. With the time delay loop, you can stop the program for a while.
For example, if an important message flashes on the screen and the alarm goes off before you can read it.
So, you can add a time delay loop so you have enough time to read the message.
Infinite While Loop
The Infinite While Loop is a while loop where the while condition never becomes false.
When a condition never becomes false, the program goes into the loop and keeps repeating the same block of code over and over again, and the loop never ends.
The code below shows an example of an infinite while loop.
// pprogram by Glenn Magada Azuelo public class WhileLoopDemo { public static void main(String args[]) { int j = 0; while(j <= 10) { System.out.println( j * j); } j++; //writing the update expression outside the loop body makes an infinite while loop } }
Output:
0
0….
You can test the above example here! ➡Java Online Compiler
The above loop is an infinite loop because the statement j++ does not appear in the body of the loop.
The value of j stays the same, which is 0, and the loop never ends.
To make an infinite while loop, we can also write the boolean value true inside the while statement.
Since the condition will always be true, the body of the loop will be run over and over again. It can be seen below:
while(true)
System.out.println(“This program is an infinite loop”);
For Loop in Java
The For Loop is a control structure that lets you write a loop that needs to be run a certain number of times in an efficient way.
You can use a for loop when you know how many times you need to do something.
All of its loop control elements are at the top of the loop, inside the round brackets().
In other Java loop structures, the loop control elements are spread out across the program.
Basic Syntax of For Loop
for(initialization loop(s) ; test-loop ; update-loop(s))
{
body of the loop ;
}
For Loop Example:
int g = 0;
for( g = 1 ; g <= 10 ; g++ )
{
System.out.println(Value of g: “ +g);
}
Example Program of For Loop
// program by Glenn Magada Azuelo
public class ForLoopDemo
{
public static void main(String args[])
{
int g;
for(g = 10; g >= 1; g--)
{
System.out.println("The value of g is: "+g);
}
}
}
Output:
The value of g is: 10
The value of g is: 9
The value of g is: 8
The value of g is: 7
The value of g is: 6
The value of g is: 5
The value of g is: 4
The value of g is: 3
The value of g is: 2
The value of g is: 1
You can test the above example here! ➡Java Online Compiler
The image below illustrates how a for loop works.
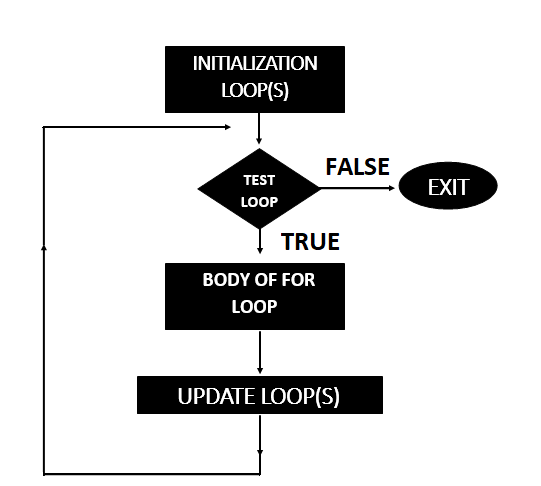
Do-While Loop in Java
The Do-While Loop is used to repeat a section of code until a certain condition is met.
A do-while loop should be used when the number of iterations is not set and the loop must be run at least once.
A Java do-while loop is called an exit control loop.
The do-while loop is different from the for and while loops in that it is an exit-controlled loop.
This means that its test-expression or test-condition is evaluated at the end of the loop after the statements in the loop-body have been run.
This means that the code in the do-while loop is always run at least once!!
Need For A Do-While Loop:
Before the loop body is run in a for or while loop, the condition is checked. If the test expression evaluates to false for the first time, the body of the loop will never be run.
But sometimes, no matter what the initial state of the test-expression is, we want the loop-body to run at least once. In this case, the best thing to do is to use the do-while loop.
Syntax Of Do-While Loop
do
{
statement(s); } while(test-expression) ;
When the loop-body only has one statement, the braces { } aren’t needed.
Example Program of Do-While Loop:
The code below explains how a do-while loop works.
//program to print all upper case letters
// program By Glenn Magada Azuelo
public class DoWhileLoopDemo
{
public static void main(String args[])
{
char ch = 'A' ;
do
{
System.out.println( ch + " " );
ch++;
} while(ch <= 'Z');
}
}
Output:
A
B
C
D
E
F
G
H
I
J
K
L
M
N
O
P
Q
R
S
T
U
V
W
X
Y
Z
You can test the above example here! ➡Java Online Compiler
The code above prints characters starting with “A” and going on until the condition ch= “Z” is no longer true.
The following picture shows how a do-while loop works:
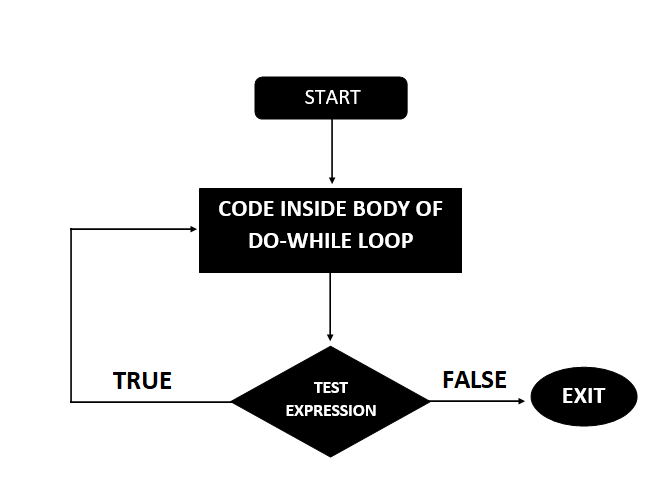
Most of the time, the do-while loop is used in menu selection systems where the user can see the menu at least once.
Then, depending on what the user does, it is either repeated or stopped.
Nested Loops In Java
A Nested Loop is a loop that contains another loop inside of it.
But when there are two loops inside each other, the inner loop must finish before the outer loop.
Example Program of Nested Loop:
public class NestedLoopDemo {
public static void main(String args[]) {
int i, j;
for(i = 1; i <= 5; ++i) { //outer loop
for(j = 1; j <= i; ++j) { //inner loop
System.out.print("* ");
}
System.out.println(); //print a newline after each row
}
}
}
You can test the above example here! ➡Java Online Compiler
Output:
*
* *
* * *
* * * *
* * * * *
Loop Control Statements
With Loop Control Statements, you can run the same block of code over and over again.
There are two kinds of loops:
For statements loop a certain number of times and keep track of each time with an index variable that goes up with each iteration. while statements keep going as long as something stays true.
Loop control statements change the normal order of execution. When execution leaves a scope, any automatic objects that were created in that scope are destroyed.
The following control statements can be used with Java.
Loop Control Statements | Description |
break statement | Terminates the loop or switch statement and moves execution to the statement that comes right after the loop or switch. |
continue statement | This makes the loop skip the rest of its body and immediately retest its condition before starting over. |
Enhanced For Loop In Java
In Java, the for-each loop is used to iterate through the items in arrays and collections (like ArrayList).
It’s also known as the Enhanced For Loop In Java.
Basic Syntax Of Enhanced For Loop In Java
for(declaration : expression) {
// Statements
}
- Declaration – The type of the new block variable is the same as the type of the array elements you are accessing. Within the for block, the variable will be available, and its value will be the same as the current array element.
- Expression – This gives you the array that you need to go through in a loop. The expression can be a variable that holds an array or a call to a method that gives back an array.
Example of Enhanced For Loop in Java:
// program by Glenn Magada Azuelo
public class Test {
public static void main(String args[]) {
int [] numbers = {26, 26, 30, 22, 20};
for(int x : numbers ) {
System.out.print( x );
System.out.print(",");
}
System.out.print("\n");
String [] names = {"Glenn", "Jude", "Adones", "Prince"};
for( String name : names ) {
System.out.print( name );
System.out.print(",");
}
}
}
Output:
26,26,30,22,20,
Glenn,Jude,Adones,Prince,
You can test the above example here! ➡Java Online Compiler
Conclusion
In this article, we talked about Loops In Java, how it works, and how this chapter could help you learn Java.
This could be one of the most important learning guides that will help you improve your skills and knowledge as a future Java Developer.
What’s Next
In the next chapter, we will learn about Decision Making Statements in Java programming.
< PREVIOUS
NEXT >