The Number class In Java is the superclass of BigDecimal, BigInteger, Byte, Double, Float, Integer, Long, and Short.
The subclasses of Number must have methods for converting the represented number to byte, double, float, int, long, and short.
What is Number Classes in Java
The Java Number Class is an abstract class that is placed in the java.lang package.
It has two concrete methods and four abstract methods.
The classes BigDecimal, BigInteger, Byte, Double, Float, Integer, Long, and Short are all subclasses of the abstract class Number.
This class only has one constructor number ().
What is the Use of Number Class
The main use of this Number class In Java is to provide methods for converting the number in question to primitive types like byte, short, int, long, double, and float.
Without further ado, I will explain it in a simple way.
Usually, when we work with Number Class, we use primitive data types like byte, int, long, double, etc.
Number Class In Java Example
int number = 6000;
float gpa = 14.65f;
double fuel = 155;
But there are times in development when we need to use objects instead of primitive data types.
Wrapper classes are part of Java so this can be done.
The number is an abstract class, and all of the wrapper classes (Integer, Long, Byte, Double, Float, and Short) are subclasses of it.
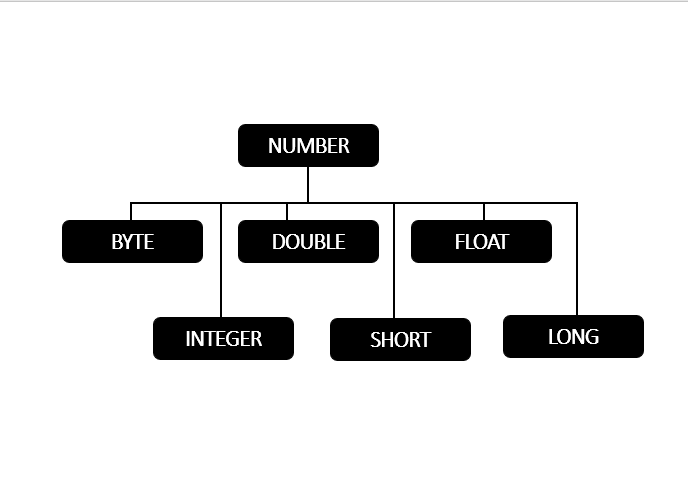
The object of the wrapper class holds or wraps its respective primitive data type.
Boxing is the process of converting primitive data types into objects, which is taken care of by the compiler.
So, when using a wrapper class, you just need to pass the value of the primitive data type to the constructor of the wrapper class.
And the Wrapper object will be turned back into a primitive data type.
This process is called “unboxing.” The Number class is a part of the java.lang package.
Here is an example of boxing and unboxing
Number Class In Java Example Program
// program by Glenn Magada Azuelo public class Test { public static void main(String args[]) { Integer x = 43; // boxes int to an Integer object x = x + 10; // unboxes the Integer to a int System.out.println(x); } }
Output:
53
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
You can test the above example here! ➡Java Online Compiler
When an integer value is given to x, which is an integer object, the compiler boxes the integer.
Later, x is taken out of its box so that the numbers can be added as a whole.
Number Methods
Here is a list of the instance methods that all of the Number class’s subclasses use.
number | Methods And Description |
1 | xxxValue() Returns the value of this Number object converted to the xxx data type. |
2 | compareTo() It compares this Number object to the argument. |
3 | equals() Checks whether this number object is equal to the argument. |
4 | valueOf() Returns an Integer object with the value of the primitive that was specified. |
5 | toString() Returns an Integer object with the value of the specified primitive. |
6 | parseInt() This method is used to find out what kind of data a String is made of. |
7 | abs() Returns the argument’s absolute value. |
8 | ceil() Returns the smallest integer that is larger than or equal to the argument. Was returned as a double. |
9 | floor() Returns the biggest integer that is less than or equal to the argument. Was returned as a double. |
10 | rint() Returns the integer whose value is closest to the one passed in. Came back as a double. |
11 | round() Returns the long or int that is closest to the argument, as shown by the return type of the method. |
12 | min() Returns which of the two arguments is smaller. |
13 | max() Returns which of the two arguments is larger. |
14 | exp() Returns e to the power of the argument, which is the base of natural logarithms. |
15 | log() Returns the natural logarithm of the given argument. |
16 | pow() Returns the value of the first argument raised to the level of the second argument. |
17 | sqrt() Returns the value’s square root. |
18 | sin() Returns the sine of the double value that was given. |
19 | cos() Returns the cosine of the double value that was given. |
20 | tan() Returns the tangent of the double value that was given. |
21 | asin() Returns the arcsine of the double value that was given. |
22 | acos() Returns the arccosine of the given double value. |
23 | atan() Returns the arctangent of the double value that was given. |
24 | atan2() Converts rectangular coordinates (x, y) into polar coordinates (r, theta) and returns theta. |
25 | toDegrees() the argument is converted to degrees. |
26 | toRadians() Changes the argument to radians. |
27 | random() It gives back a random number. |
Conclusion
In this article, we talked about Number Classes In Java, how they work and what they’re used for, and how this chapter can make it easier for you to learn Java.
This could be one of the most helpful ways to learn how to be a better Java developer.
What’s Next
In the next section, we’ll talk about the Character Class In Java. You will learn how to use object Characters and the simple data type char in Java.
< PREVIOUS
NEXT >