How can I simulate generic arrays in Java?
In Java, you can’t directly define a generic array, which means you can’t assign a parameterized type to an array reference.
But you can simulate the creation of a generic array by using object arrays and reflection features.
Generic array creation in Java
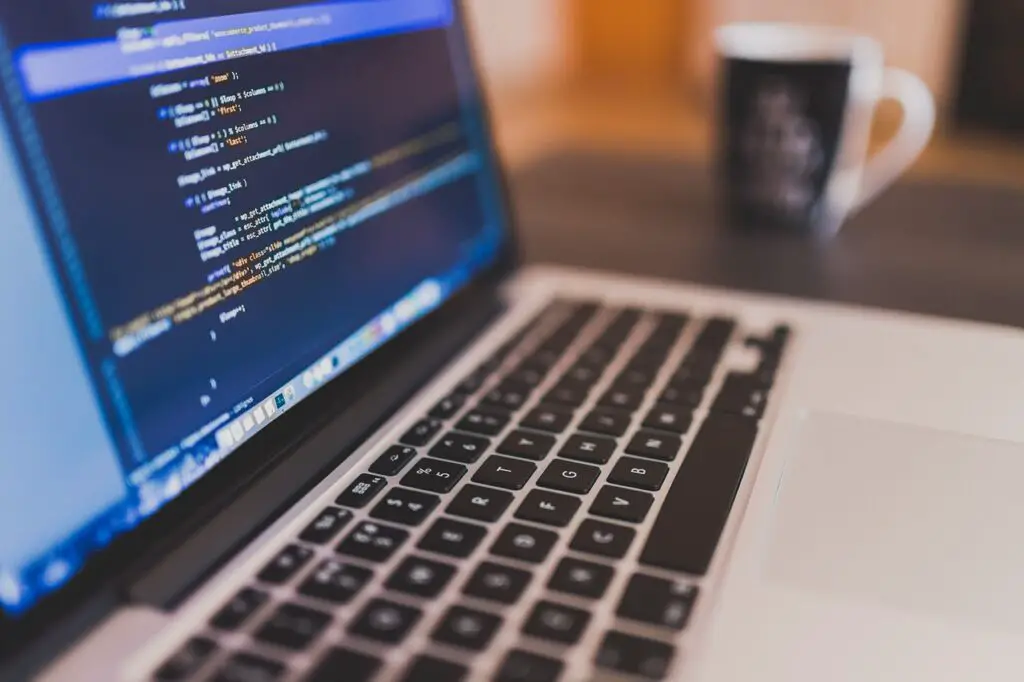
For example, let’s look at a data structure called MyStack, which is a bounded stack with a fixed size.
Since we want the stack to work with any type, a generic array would be a good way to build it.
First, we’ll make a field for our stack’s elements, which is an array of type E:
private E[] elements;
We’ll then include a constructor:
public MyStack(Class<E> clazz, int capacity) { elements = (E[]) Array.newInstance(clazz, capacity); }
Take note of how we initialize our generic array, which takes two parameters, using java.lang.reflect.Array#newInstance.
The new array’s first parameter determines the type of item it will include.
The array’s second parameter tells how much room to make for it.
We must cast the Object type returned by Array#newInstance to E[] in order to use it to build our generic array.
Use the Reflection class to create generic arrays in Java
In this method, we generate a generic array whose type will only be known at runtime using a reflection class.
The new method is similar to the old one, with just one difference: instead of passing the data type argument information to the class constructor, we use the reflection class in the constructor itself to create an object array.
The Array gets this kind of information. Method of reflection on the newInstance.
In the next program, reflection is used to make a generic array. Note that the whole structure of the program is the same as the previous method.
The only difference is that this method uses reflection features.
Example:
// program created by Glenn
importjava.util.Arrays;
class Array<E> {
private final E[] objArray;
public final int length;
// class constructor
public Array(Class<E>dataType, int length){
// create a new array with the specified data type and length at runtime using reflection
this.objArray = (E[]) java.lang.reflect.Array.newInstance(dataType, length);
this.length = length;
}
// get element at objArray[i]
Eget(int i) {
returnobjArray[i];
}
// assign e to objArray[i]
void set(int i, E e) {
objArray[i] = e;
}
@Override
public String toString() {
return Arrays.toString(objArray);
}
}
public class Main {
public static void main(String[] args){
final int length = 5;
// create array with Integer as data type
Array<Integer>int_Array = new Array(Integer.class, length);
System.out.print("Generic Array<Integer>:" + " ");
for (int i = 0; i < length; i++)
int_Array.set(i, i + 10);
System.out.println(int_Array);
// create an array with String as data type
Array<String>str_Array = new Array(String.class, length);
System.out.print("Generic Array<String>:" + " ");
for (int i = 0; i < length; i++)
str_Array.set(i, String.valueOf((char)(i + 65)));
System.out.println(str_Array);
}
}
Output:
Generic Array <Integer>: [0, 2, 4, 6, 8] Generic Array <String>: [A, B, C, D, E]
Use object arrays to create generic arrays in Java
This method uses the array of type objects as a member of the main array class.
We also use the get/set methods to read and change the elements of an array.
Then, we create an instance of the main array class, which lets us set the data type at runtime as needed.
Example:
// program created by Glenn
import java.util.Arrays;
class Array<E> {
private final Object[] obj_array; //object array
public final int length;
// class constructor
public Array(int length){
// instantiate a new Object array of specified length
obj_array = new Object [length];
this.length = length;
}
// get obj_array[i]
E get(int i) {
@SuppressWarnings("unchecked")
final E e = (E)obj_array[i];
return e;
}
// set eat obj_array[i]
void set(int i, E e) {
obj_array[i] = e;
}
@Override
public String toString() {
return Arrays.toString(obj_array);
}
}
class Main {
public static void main(String[] args){
final int length = 5;
// creating integer array
Array<Integer>int_Array = new Array(length);
System.out.print("Generic Array <Integer>:" + " ");
for (int i = 0; i < length; i++)
int_Array.set(i, i * 2);
System.out.println(int_Array);
// creating string array
Array<String>str_Array = new Array(length);
System.out.print("Generic Array <String>:" + " ");
for (int i = 0; i < length; i++)
str_Array.set(i, String.valueOf((char)(i + 97)));
System.out.println(str_Array);
}
}
Output:
Generic Array <Integer>: [0, 2, 4, 6, 8] Generic Array <String>: [a, b, c, d, e]
Java array class
The Arrays class is part of the util package in Java.
This is part of the Java Collections framework and gives you ways to create, access, and change Java arrays on the fly.
All of the methods that the Arrays class gives you are static and come from the Object class.
Generic array in Java
Generics and arrays enforce type-checking in different ways, which is a significant distinction.
Specifically, runtime-type information checking and storing occurs in arrays.
Generics, on the other hand, don’t contain type information at runtime and instead check for type issues at compile time.
Syntax:
T[] elements = new T[size];
However, if we tried to do this, a compile error would occur.
Let’s explore the following in order to get why:
public <T> T[] getArray(int size) { T[] genericArray = new T[size]; // suppose this is allowed return genericArray; }
Our procedure will be as follows at runtime since an unbound generic type T resolves to Object:
public Object[] getArray(int size) { Object[] genericArray = new Object[size]; return genericArray; }
If we call our method and save the result in a String array:
String[] myArray = getArray(6);
The code will compile fine, but a ClassCastException will be thrown when it is run.
This is because we just gave a reference to a String[] an Object[] value.
In particular, an implicit cast by the compiler won’t be able to change Object[] to the type String[] that we need.
Can you make an array of generics in Java?
Java lets you make generic classes, methods, and other things that can be declared independently of types.
However, Java doesn’t allow the array to be generic.
The reason for this is that in Java, arrays of objects contain information about their parts, and this information is used to allocate memory at runtime.
Why are there no generic arrays in Java?
Arrays of generic types aren’t allowed because they’re not safe.
The problem is caused by the interaction between Java arrays, which are not statically sound but are checked dynamically, and generics, which are statically sound but not dynamically checked and suppress warnings unchecked.
How do you create a new generic array in Java?
To make a generic array in Java, use the Reflection Class.
In this method, a reflection class is used to make a generic array whose type safety will only be known at runtime.
The only difference between this method and the last one is that this method uses the reflection class as the constructor itself.
How do you pass a generic class object as a parameter in Java?
// program created by Glenn
// generic methods
public <T> List<T> fromArrayToList(T[] a) {
return Arrays.stream(a).collect(Collectors.toList());
}
public static <T, G> List<G> fromArrayToList(T[] a, Function<T, G> mapperFunction) {
return Arrays.stream(a)
.map(mapperFunction)
.collect(Collectors.toList());
}
// bounded generics
public <T extends Number> List<T> fromArrayToList(T[] a) {
...
}
//multiple bounds
<T extends Number & Comparable>
// upper bound wildcards
public static void paintAllBuildings(List<? extends Building> buildings) {
...
}
// lower bound wildcard
<? super T>
What are generic classes in Java?
A generic class just means that the items or functions in that class can be generalized with the parameter (for example, T) to show that we can add any type as a parameter in place of T, like Integer, Character, String or Strings, Double, or any other user-defined type.
What is the main use of generics in Java?
In a nutshell, generics allow types (classes and interfaces) to be used as parameters when defining classes, interfaces, and methods.
Raw-type parameters are a way to reuse the same code with different inputs.
They work a lot like formal parameters, which are used in method declarations.
How many type parameters can be used in a generic class?
Java generics also let you use more than one type of parameter.
To do this, you just need to add another type parameter between the angle brackets and separate it with a comma.
How do you make a generic class in Java?
To update the Box class so it can use generics, you change the code “public class Box” to “public static void class Box<T>” to create a generic type declaration.
This makes the type variable, T, which can be used anywhere inside the class. As you can see, every instance of Object has been replaced with T.
How do you pass a generic function in Java?
In Java, generics are made possible by erasing types. This means that an ArrayList<T> is just an ArrayList at run time. The compiler just puts the casts in for you.
Summary
This article discusses how I can simulate generic arrays in Java.
In addition, we look at how you can pass a generic function and an instance of a generic type parameter into a generic class and generic array creation.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.
< PREVIOUS