What is Bubble Sort in Java?
The Java Program to Bubble Sort is a basic sorting algorithm that compares neighboring array elements and swaps them if the rightmost element is smaller than the leftmost.
It’s an in-place sorting method, which means it doesn’t take up any extra space and just modifies the array itself.
Bubble sort is a simple algorithm that sorts a list by letting the lowest or highest values rise to the top, just like how bubbles rise from the bottom of a glass.
The algorithm goes through a list and compares the values next to each other. If they are not in the right order, they are switched.
How does Bubble Sort work?
This is how Bubble Sort simply works:
- First Iteration (Compare and Swap)
- Compare the first and second elements starting with the first index.
- The first and second elements are swapped if the first is bigger than the second.
- The first and second elements are swapped if the first is bigger than the second.
- The method is repeated until the last element.
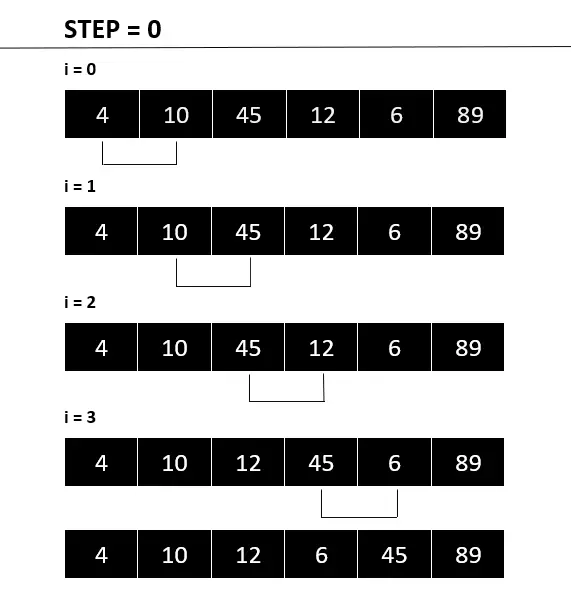
- Iteration Continues
For the remaining iterations, the same procedure is followed.
The largest element among the unsorted items is placed at the conclusion of each iteration.
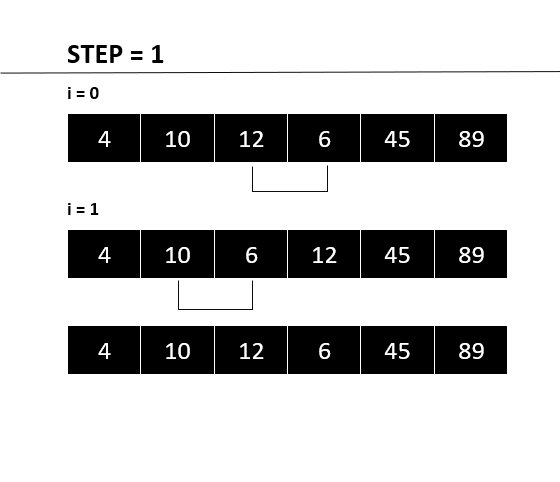
As you can see in the image, the iteration is completely finished.
From the left side of all the elements, the number of elements is from lowest to highest (4, 6, 10, 12, 45, 89), which means that the bubble is sorted completely and sorted perfectly.
Algorithm of Bubble Sort
bubbleSort(array) for i <- 1 to indexOfLastUnsortedElement – 1 if leftElement > rightElement swap leftElement and rightElement end bubbleSort
Bubble Sort Sample Code
You can run this code in your code editor if you already downloaded it to your laptop or PC, but if you don’t, you can download it.
Here’s the link. NetBeans IDE, but if you wish to run this code online, we also have an online compiler in Java.
Sample Java Code Using Scanner
A Scanner is a class in the Java Utilities package that is used to get the input of primitive types like int, double, etc., and strings.
It is the easiest way to read input into a Java program.
import java.util.Scanner; public class Bubble_Sort_Java { public static void Sort(int a[]) { int n = a.length, i, j, p, temp; for (i = 0; i < n - 1; i++) { for (j = 0; j < n - i - 1; j++) { if (a[j + 1] < a[j]) { temp = a[j + 1]; a[j + 1] = a[j]; a[j] = temp; } } } } public static void printarray(int a[]) { for (int i = 0; i < a.length; i++) { System.out.print(a[i] + " "); } } /** * @param args the command line arguments */ public static void main(String[] args) { int n, res, i; Scanner s = new Scanner(System.in); System.out.print("Enter number of elements in the array:"); n = s.nextInt(); int a[] = new int[n]; System.out.println("Enter " + n + " elements "); for (i = 0; i < n; i++) { a[i] = s.nextInt(); } System.out.println("elements in array "); printarray(a); Sort(a); System.out.println("\nelements after sorting"); printarray(a); } }
If you wish to use our online compiler to run this Bubble Sort source code, simply copy all of the code and paste it there.
You can test the above example here! ➡Java Online Compiler
Bubble Sort Example Output Using Scanner
Enter number of elements in the array:Enter 6 elements
elements in array
4 10 45 12 6 89
elements after sorting
4 6 10 12 45 89
You can test the above example here! ➡Java Online Compiler
Sample Java Code Using Array
An Array is a container object that holds a fixed number of values of the same type. The length of an array is set when the array is made. After it is made, its length is fixed.
public class Bubble_Sort_Java { static void bubbleSort(int[] arr) { int n = arr.length; int temp = 0; for(int i=0; i < n; i++){ for(int j=1; j < (n-i); j++){ if(arr[j-1] > arr[j]){ //swap elements temp = arr[j-1]; arr[j-1] = arr[j]; arr[j] = temp; } } } } public static void main(String[] args) { int arr[] ={4,10,45,12,6,89}; System.out.println("Array Before Bubble Sort"); for(int i=0; i < arr.length; i++){ System.out.print(arr[i] + " "); } System.out.println(); bubbleSort(arr);//sorting array elements using bubble sort System.out.println("Array After Bubble Sort"); for(int i=0; i < arr.length; i++){ System.out.print(arr[i] + " "); } } }
If you wish to use our online compiler to run this Bubble Sort source code, simply copy all of the code and paste it there.
You can test the above example here! ➡Java Online Compiler
Bubble Sort Example Output Using Array
Array Before Bubble Sort
4 10 45 12 6 89
Array After Bubble Sort
4 6 10 12 45 89
You can test the above example here! ➡Java Online Compiler
Anyway, if you want to level up your programming knowledge, especially Java, try this new article I’ve made for you Best Java Projects With Source Code For Beginners Free Download.
Conclusion
So far, we’ve already discussed the Bubble Sort algorithm for sorting in Java.
We also looked at the algorithm and a detailed example of how to use the Bubble Sort Technique to sort an array.
Then we put the Java program to work on the Bubble Sort. I hope this Bubble Sort tutorial helps you a lot in learning Java development.