Abstraction in OOP (Object-Oriented Programming)
Abstraction is one of the three main ideas behind object-oriented programming (along with encapsulation and inheritance).
Through the process of abstraction, a programmer hides all the information about an object except for the important parts.
This makes the program simpler and more efficient.
What is An Abstraction in Java?
In Java, Data Abstraction is the process of stripping an object down to its most basic parts so that only the most important parts are shown to the user.
Data Abstraction is the property that lets the user see only the most important data.
The user is unable to view the units that are minimally or completely unimportant.
Difference Between Abstraction and Encapsulation?
Abstraction can be used to conceal unwanted information.
On the other hand, encapsulation is a technique for shielding data from the outside world and hiding it within a single entity or unit.
What is the Use of an Abstract Class in Java?
An abstract class can be used as a template for other classes, but they are most often used for inheritance.
The best example comes from the book head first Java:
abstract class Animal(){}
Then you can extend a child class, like Dog
, Cat, or Fish.
Abstract Method in Java
Abstract methods can only be used in abstract classes and don’t have bodies.
The subclass supplies the body (inherited from).
An abstract class is not allowed to be used to make objects (to access it, it must be inherited from another class).
There can be both abstract and regular methods in an abstract class:
abstract class Animal { public abstract void animalSound(); public void wakeUp() { System.out.println("Hmmmmmm"); } }
Based on the above example, you can’t make an object of the Animal class :
Animal myObject = new Animal(); // will generate an error
Example:
// program created by Glenn
// Abstract class
abstract class Animal {
// Abstract method (does not have a body)
public abstract void animalSound();
// Regular method
public void wakeUp() {
System.out.println("Hmmmmmm");
}
}
// Subclass (inherit from Animal)
class Goat extends Animal {
public void animalSound() {
// The body of animalSound() is provided here
System.out.println("The Goat says: meeee meeee");
}
}
public class Main {
public static void main(String[] args) {
Cow myCow = new Cow();
myCow.animalSound();
myCow.wakeUp();
}
}
Output:
The Cow says: maaaa maaaa maaaa maaaa Hmmmmmmm
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
Why do we need Abstract Classes in Java?
In Java, you need Abstract Classes because they implement interfaces without even implementing interface methods.
It is then used to give all the subclasses the same implementation of a method or a default implementation.
I will explain these things more to make them clearer.
Let’s say we want to make a class that just declares the overall shape, structure, or rules of an idea without giving a full implementation of each method.
And we want this generalized form or structure of the class to be usable by all of its child classes.
The child classes will enforce these rules by filling in all the implementation details based on what is needed.
We can mark the method as abstract in the parent class because it does not need to be implemented.
That is, we will not provide you with any information on how to use these abstract methods.
If you make these methods abstract, any classes that inherit from them must implement them.
If they do not, your code will not be able to be compiled.
Lastly, if an abstract class in Java has a main () method, it can be run just like any other class.
Example:
// sample created by Glenn
abstract class GeometricShapes
{
String nameOfShape;
//abstract methods
abstract double calculateArea();
public abstract String toString();
//constructor
public GeometricShapes(String nameOfShape)
{
System.out.println("Inside the Constructor of GeometricShapes class by PIES");
this.nameOfShape = nameOfShape;
}
//non-abstract method
public String getNameOfShape()
{
return nameOfShape;
}
}
class Circle extends GeometricShapes
{
double radius;
public Circle(String nameOfShape,double radius)
{
super(nameOfShape);
System.out.println("Inside the Constructor of Circle class by PIES");
this.radius = radius;
}
//implementing the methods
@Override
double calculateArea()
{
return Math.PI * Math.pow(radius, 3);
}
@Override
public String toString()
{
return "Name of the shape is " + super.nameOfShape +
" and its area is: " + calculateArea();
}
}
class Square extends GeometricShapes
{
double length;
public Square(String nameOfShape,double length)
{
//calling Shape constructor
super(nameOfShape);
System.out.println("Inside the Constructor of Square class ");
this.length = length;
}
//implementing the methods
@Override
double calculateArea()
{
return length * length;
}
@Override
public String toString()
{
return "Name of the Shape is " + super.nameOfShape +
" and its area is: " + calculateArea();
}
}
public class AbstractionDemo
{
public static void main(String[] args)
{
GeometricShapes shapeObject1 = new Circle("Circle", 7.5);
System.out.println(shapeObject1.toString());
GeometricShapes shapeObject2 = new Square("Rectangle",9);
System.out.println(shapeObject2.toString());
}
}
Output:
Inside the Constructor of GeometricShapes class by PIES Inside the Constructor of Circle class by PIES Name of the shape is Circle and its area is: 1325.359400733194 Inside the Constructor of GeometricShapes class by PIES Inside the Constructor of Square class Name of the Shape is Rectangle and its area is: 81.0
You can test the above example here! ➡Java Online Compiler
What is Java Abstract Class?
An abstract class is a restricted class that can’t be used to make objects (to access it, it must be inherited from another class).
In Java, the abstract class can’t be used (we cannot create objects of abstract classes).
We use the keyword “abstract” to say that a class is abstract.
For example:
// create an abstract class abstract class Language { // fields and methods } ... // try to create an object Language // throws an error Language object = new Language();
Abstract classes can have both regular and abstract methods.
For Example:
// sample created by Glenn abstract class Language { // abstract method abstract void method1(); // regular method void method2() { System.out.println("This is regular method by PIES"); } }
Real-life example for Java Abstraction
1. Let us begin with a real-world example, an ATM.
We all use ATMs every day to get cash, send money to friends and family, check our minimum balance, and perform other tasks.
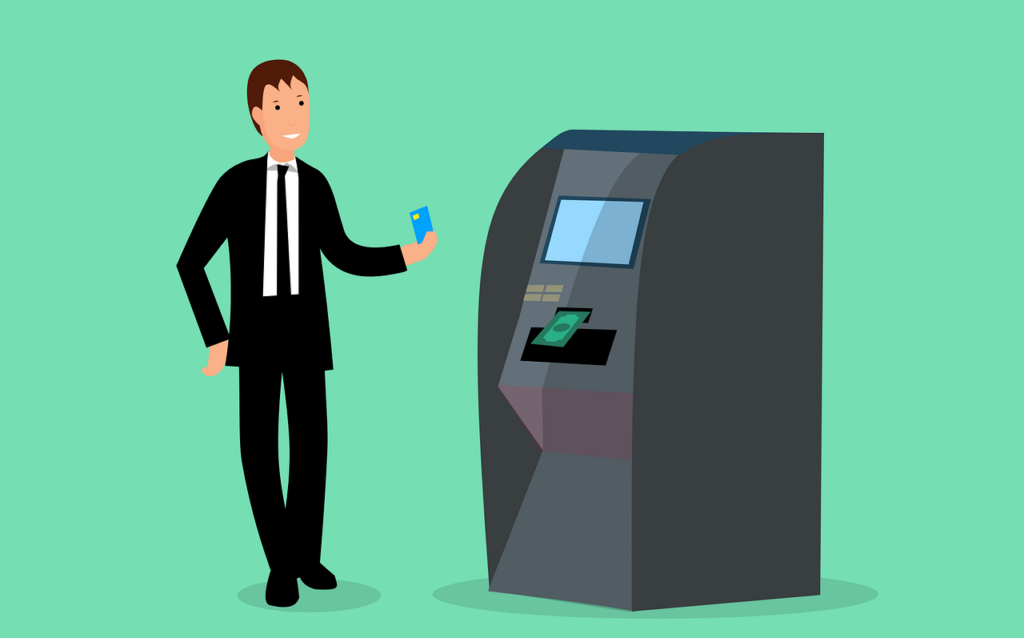
But we don’t know what happens when you put a card into an ATM.
2 . Consider yet another instance from the real world. A car owner is knowledgeable on how to drive their vehicle.
The numerous parts of a car are something he is familiar with.
For example, a person who owns a car knows that the gas pedal speeds up the car and the brake pedal slows it down.
To do these simple things, you simply need to understand how to use these parts.
You do not need to comprehend how they operate.
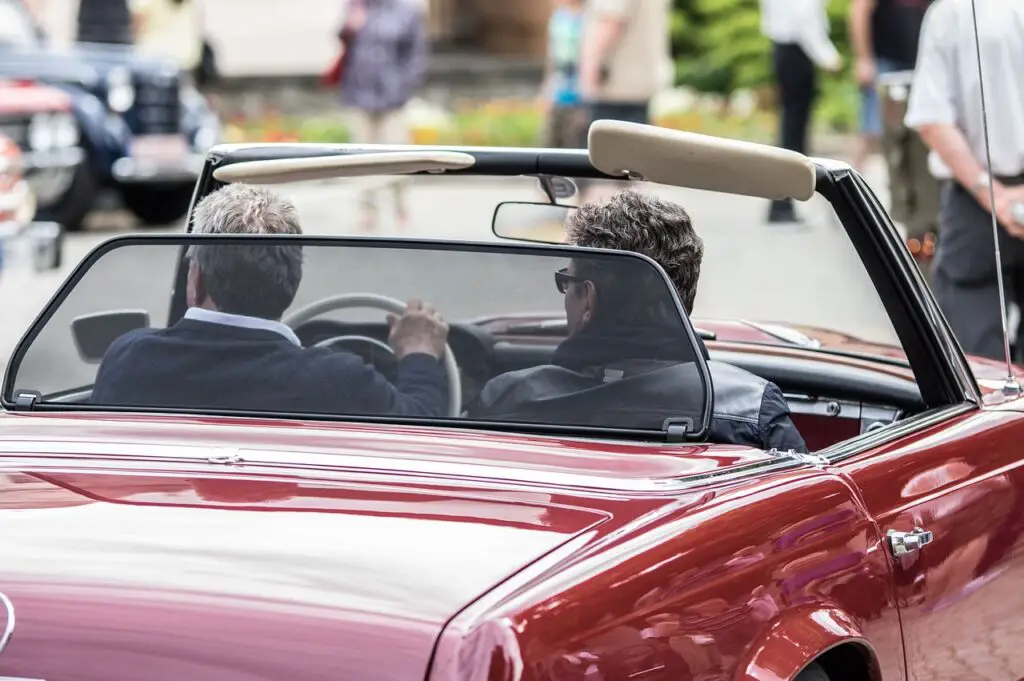
3 . You simply type the message into your phone and press the send button to send an SMS. But you have no idea how the internal message system operates.
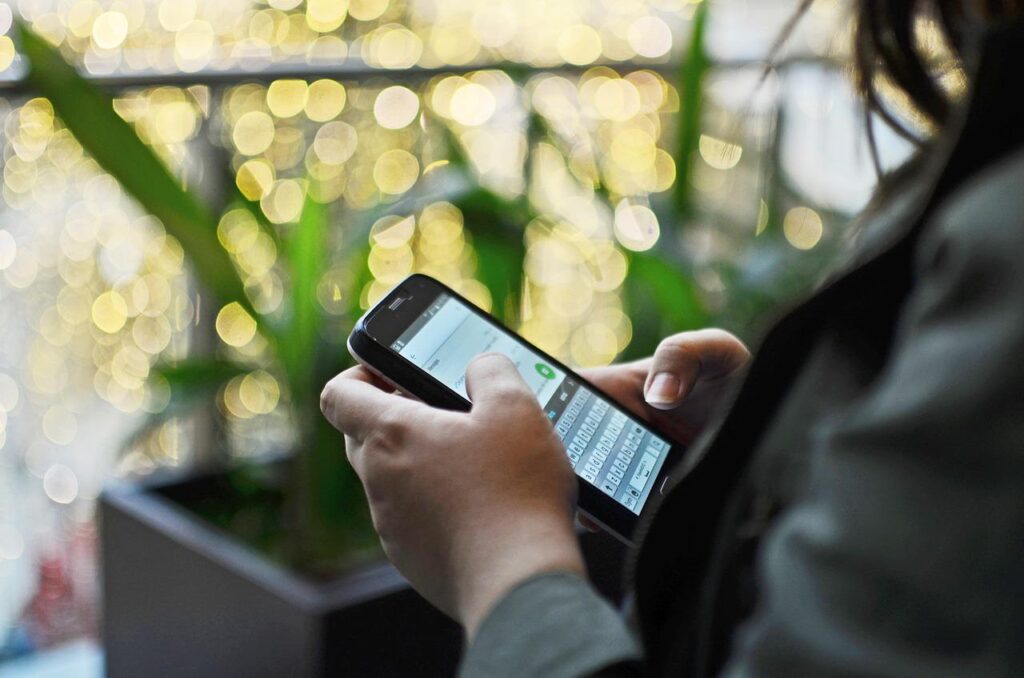
4 . A bank employee can see the customer’s name, account number, and the amount in the account.
But he can’t see private information like staff salaries, the bank’s profits or losses, the amount of interest the bank pays, loan amounts, etc.
Such information is kept from the clerk. When the bank manager needs to know this info, it will be given to the manager.
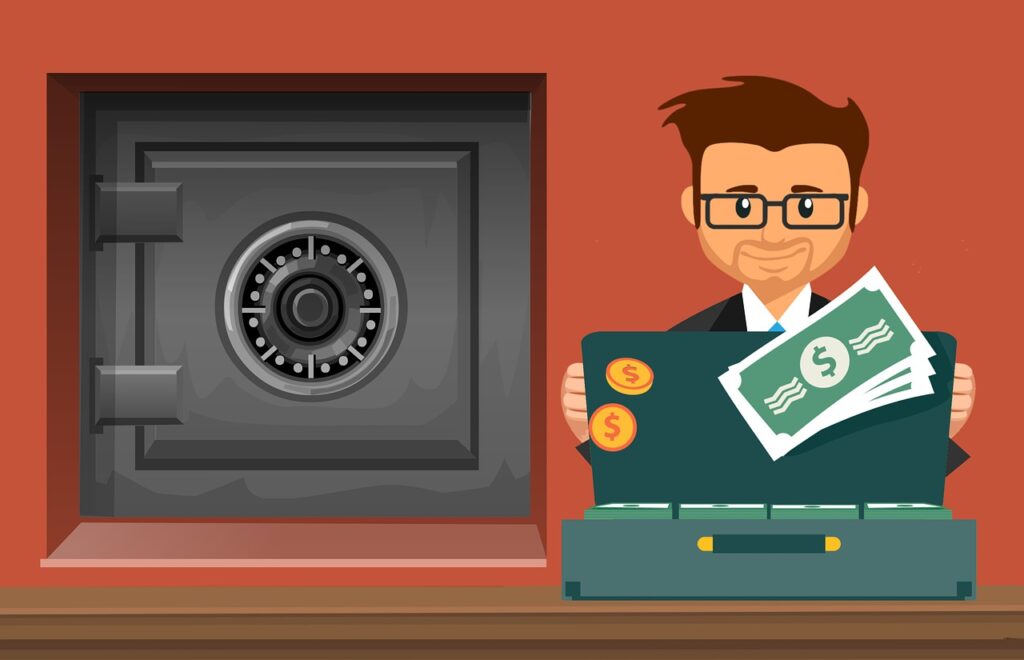
So, in the real world, there are many important things that show abstraction.
To make certain program logic work, you only need to call certain classes or methods, but you don’t know how these classes or methods work.
This idea is called abstraction in Java.
Abstract Class And Interface
An abstract class lets you make functions that subclasses can implement or change.
An interface only lets you describe functionality, not do anything with it.
While a class can only extend one abstract class, it can use multiple interfaces.
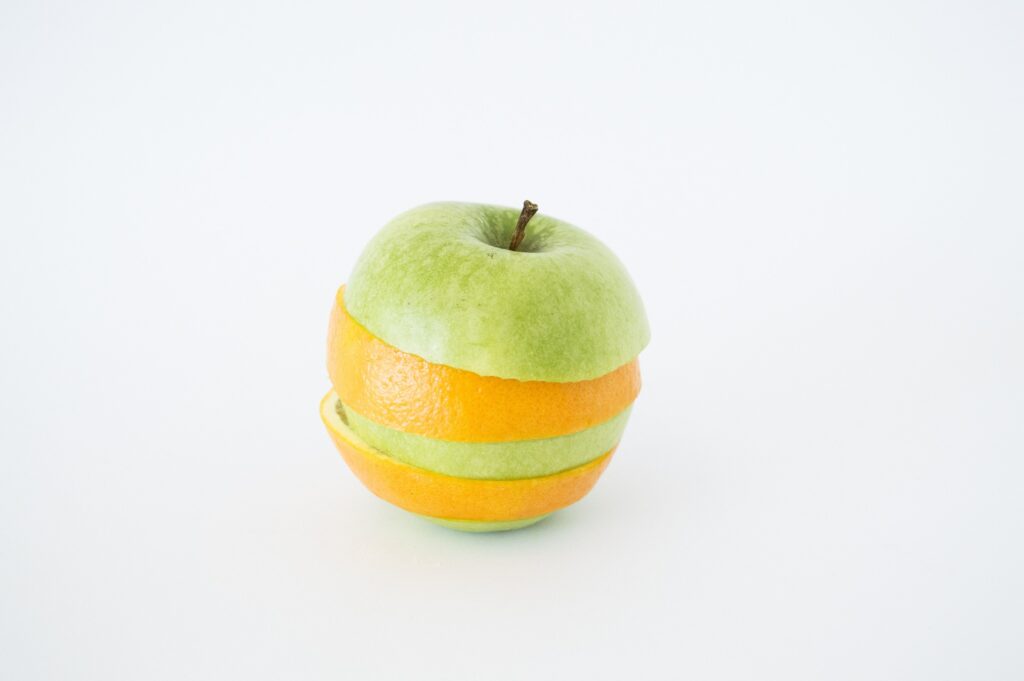
Why can’t we create an object of an Abstract Class?
An abstract class is not complete, so we cannot create an instance or object of it.
This is because abstract methods lack a body or output, an abstract class isn’t fully formed.
Let’s make one abstract class with all concrete methods and see what the error message says when an object is created.
Example:
// sample created by Glenn
abstract class Student
{
public void name() // concrete (non-abstract) method
{
System.out.println("Name is Glenn Magada Azuelo");
}
public void marks() // concrete (non-abstract) method
{
System.out.println("Marks scored are 99");
}
public static void main(String args[])
{
Student s1 = new Student(); // Error raised, see the errror in screenshot
}
}
How can I achieve Abstraction in Java?
Interfaces and abstract classes are what Java uses to achieve abstraction.
Using interfaces, we can completely separate things.
Abstract methods and Abstract Classes :
- A class that is declared with the abstract keyword is an abstract class.
- A method that is declared but not implemented is called abstract.
- It is possible for a class to contain all abstract methods or not. Possible actions you can take include some of them.
- A method defined as abstract must always be redefined in the subclass. This forces overriding or makes the subclass itself abstract.
- If a class has one or more abstract methods, the abstract keyword must also be used to declare the class.
- Abstract classes can have parameterized constructors, and they always have a default constructor.
Abstraction in Java
Abstraction is the process of hiding the implementation details from the user and only showing the functionality.
In another way, it only shows the user what they need to see and hides the internal details.
For instance, when sending an SMS, you type and send the message.
You are unaware of how internal communication transmission is managed.
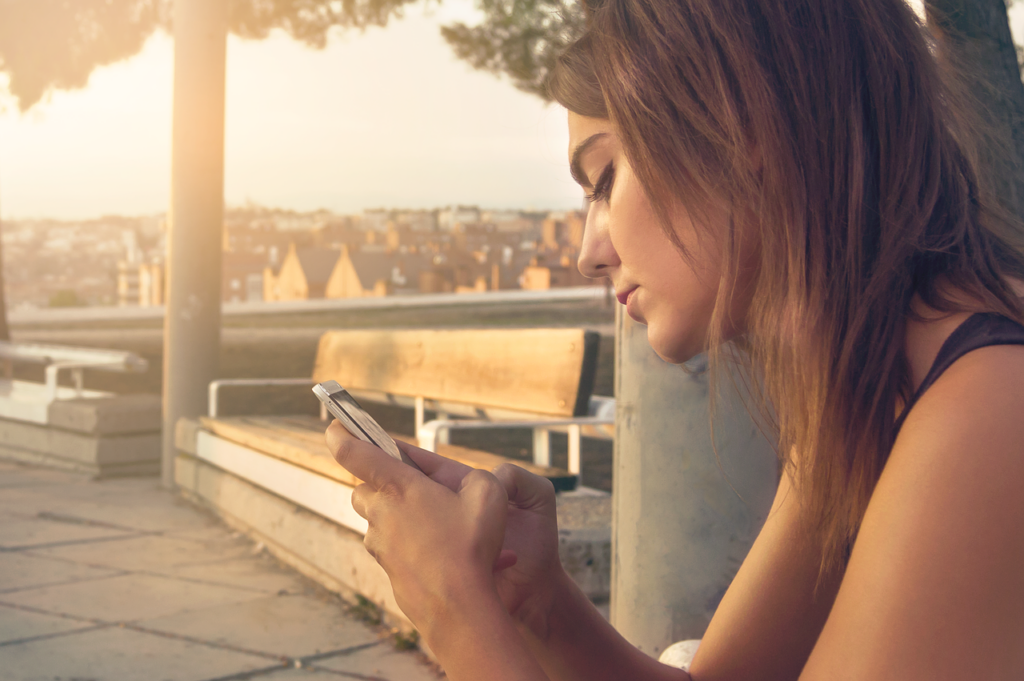
When to use Abstract Class and Interface in Java?
The following is a list of all the uses of abstract and interface classes in Java.
- An abstract class is used to give all implementations of a component the same functionality. Abstract classes let you partially implement your class, while interfaces have no implementation for any of their members.
- If you want to use the inheritance idea, an abstract class is a good choice because it gives the derived classes the same implementation as the base class.
- An interface is needed to add functionality to multiple objects. Abstract classes work well for things that are similar. Interfaces are best for giving cases that are not related to each other the same functions.
- Interfaces also work well. You can implement interfaces to mimic multiple inheritances.
Types of Abstraction in Java
In Java, there are two different kinds of abstraction:
- control abstraction
- data abstraction
Control abstraction in Java is used to add new features and combine control statements into a single unit.
This is a fundamental part of every high-level language and the main unit of structured programming.
This kind of abstraction is used whenever we want to make a function for doing a certain task.
Data abstraction in Java is the process of getting to the core of an object so that users only see the most important parts.
The attributes, methods, and interfaces of an object are what define it in terms of abstraction.
Summary
Through Data Abstraction, we can break down a real-world entity into its most important parts and only show the users what they need to see.
The idea of data abstraction is based on abstract classes in Java.
We have completed our discussion on Java. Analyzing what we have already discovered.
In our discussion and demonstration of how it functions in practice, we discussed how crucial abstraction is to Java.
We also went over a detailed explanation of abstract classes and abstract methods in Java, along with their syntax and examples.
You should now understand how important Abstraction is in OOPs and how to use it in your code.
What’s Next
The next section talks about Encapsulation. At the end of the session, you’ll know what is encapsulation all about in Java.
< PREVIOUS
NEXT >