What is serialization in Java?
Serializing an object means converting it into a byte stream so that it can be copied.
A Java object can be serialized if its class or any of its superclasses implements either the java. io. Java’s Serializable interface or one of its sub-interfaces.
What is Java IO serializable?
The Serializable class interface in Java (java. io. Serializable is a marker interface that your classes must use if they are to be serialized and deserialized.
The ObjectOutputStream is used to write (serialize) Java objects, and the ObjectInputStream is used to read (deserialize) them.
What is import Java IO serializable?
In the java.io package, you can declare a serialversionuid and find the Serializable interface.
It looks like a marker. There are no methods and fields on a Marker Interface.
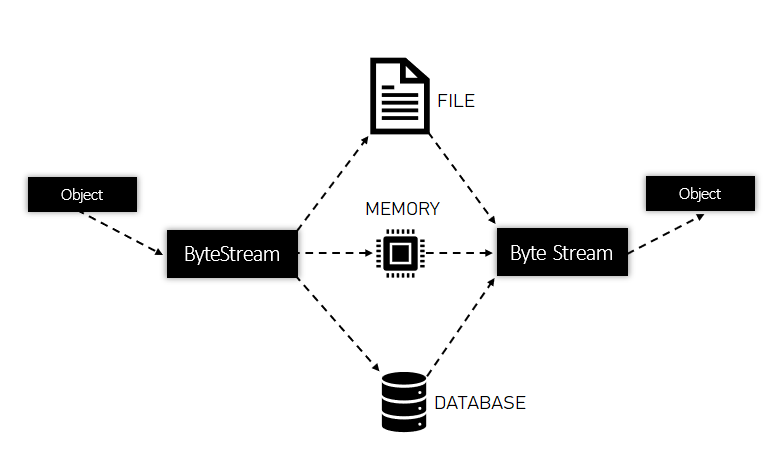
This means that classes that implementing the java.io.serializable interface don’t have to add any methods.
If a class wants its instances to be serialized or deserialized, it must implement this interface.
How to prevent a Java deserialize vulnerability?
The best way to avoid a Java deserialize vulnerability is to not use Java serialization at all.
If your app doesn’t work with serialized objects, it can’t hurt you.
Explaining Java deserialize vulnerabilities
Deserialization vulnerabilities can lead to data type corruption or application crashes, which can cause a denial of service (DoS) condition.
Many times, the same bugs can be used by remote attackers to make a system vulnerable to arbitrary code execution.
Java.io.Serializable interface
In the java.io package, you can find the Serializable interface. It looks like a marker.
There are no methods and fields on a Marker Interface.
Java Serialization with Inheritance (IS-A Relationship)
The SerializeISA class serialized the Student class object, which extends the Serializable Person class.
Subclasses inherit the properties of their parent classes, so if the parent class is Serializable, so would the subclass.
Java transient. Keywords
You can avoid serialization in Java by using the transient keyword.
Objects in a data structure won’t be serialized if they are marked as “transient.”
Serialization is the process of turning an object into a string of bytes, or byte stream.
Java Serialization with Aggregation (HAS-A Relationship)
A class that references another class must have all of those references be Serializable in order for serialization to take place.
NotSerializableException throws ioexception classnotfoundexception at runtime in this situation.
Example of Java Serialization
In this example, we will serialize the Student class object from the code above.
The ObjectOutputStream class’s writeObject() method gives you the ability to serialize the object.
The state of the object is saved in a file called f.txt.
Example:
import java.io.*;
class Persist{
public static void main(String args[]){
try{
//Creating the object
Student s1 =new Student(021596,"Glenn Magada Azuelo");
//Creating stream and writing the object
FileOutputStream fout=new FileOutputStream("f.txt");
ObjectOutputStream out=new ObjectOutputStream(fout);
out.writeObject(s1);
out.flush();
//closing the stream
out.close();
System.out.println("ITNAS PASSED");
} catch(Exception e){System.out.println(e);}
}
}
Output:
ITNAS PASSED
Externalizable in Java
Externalizable is an interface that lets you set up your own serialization rules and methods.
You need to know about Serialization before you can understand the Externalizable interface.
Java Serialization makes it easy to store an object and then re-create it later.
Java Serialization with array or collection
In Java, the ArrayList class is serializable by default, without needing to implement the Serializable interface.
Java Serialization with the static data member
Static members belong to their classes, but they are not part of the objects in those classes.
When an object is serialized, the state of static members is not saved. Static members are not part of an object’s saved state.
What is serializing and Deserializing?
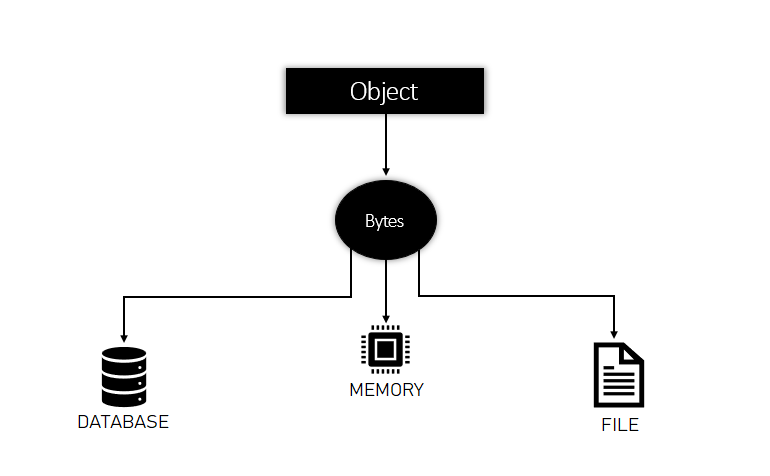
Serialization is the process of converting an object into a stream of data so that it can be stored or transmitted. Its primary function is to save how an object is configured so that it can be reconfigured as necessary.
Deserialization is the process of going backward.
Why do we need Serializable in Java?
In Java, serialization is the idea of showing the state of an object as a public int or public string bytes.
All of the information about the object is in the byte stream.
Serialization in Java is most often used in Hibernate, JMS, JPA, and EJB. It helps move code from one JVM to another and then de-serialize it there.
Why do we need a Serializable interface in Java?
Serialization is the process of converting an object into a stream of data that can be stored or transmitted.
This allows you to save objects so that they can be used later.
Deserialization is the reverse process of changing an Object stream into a real Java object that our program can use.
What happens without serialization in Java?
In a graph, you might come across an readobject method that doesn’t support the Serializable interface.
In this case, the NotSerializableException will be thrown, and it will say what class of object can’t be serialized.
Summary
In summary, you have learned about serialization in Java.
This article discusses what is serialization, what is Java IO serializable, and how to prevent a Java deserialization vulnerability.
Serialization with an array or collection and why do we need a serializable interface.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.
< PREVIOUS