This C++ Programming Tutorial For Beginners tutorial explains the ideas behind C++ in a way that is easy to understand and useful for software engineers of all levels.
Furthermore, these courses are created for beginners, so you will have no trouble understanding them even if you have no prior C++ experience.
What is C++?
- C++ is a middle-level programming language that was created at Bell Labs by Bjarne Stroustrup beginning in 1979.
- C++ works on many platforms, like Windows, Mac OS, and the different versions of UNIX.
- C++ is a general-purpose programming language that can be used to make programs for a wide range of tasks.
- It can be used to make things like operating systems, browsers, games, etc.
- C++ lets you program differently, like procedurally, object-oriented, functionally, etc.
- This makes C++ both powerful and easy to use.
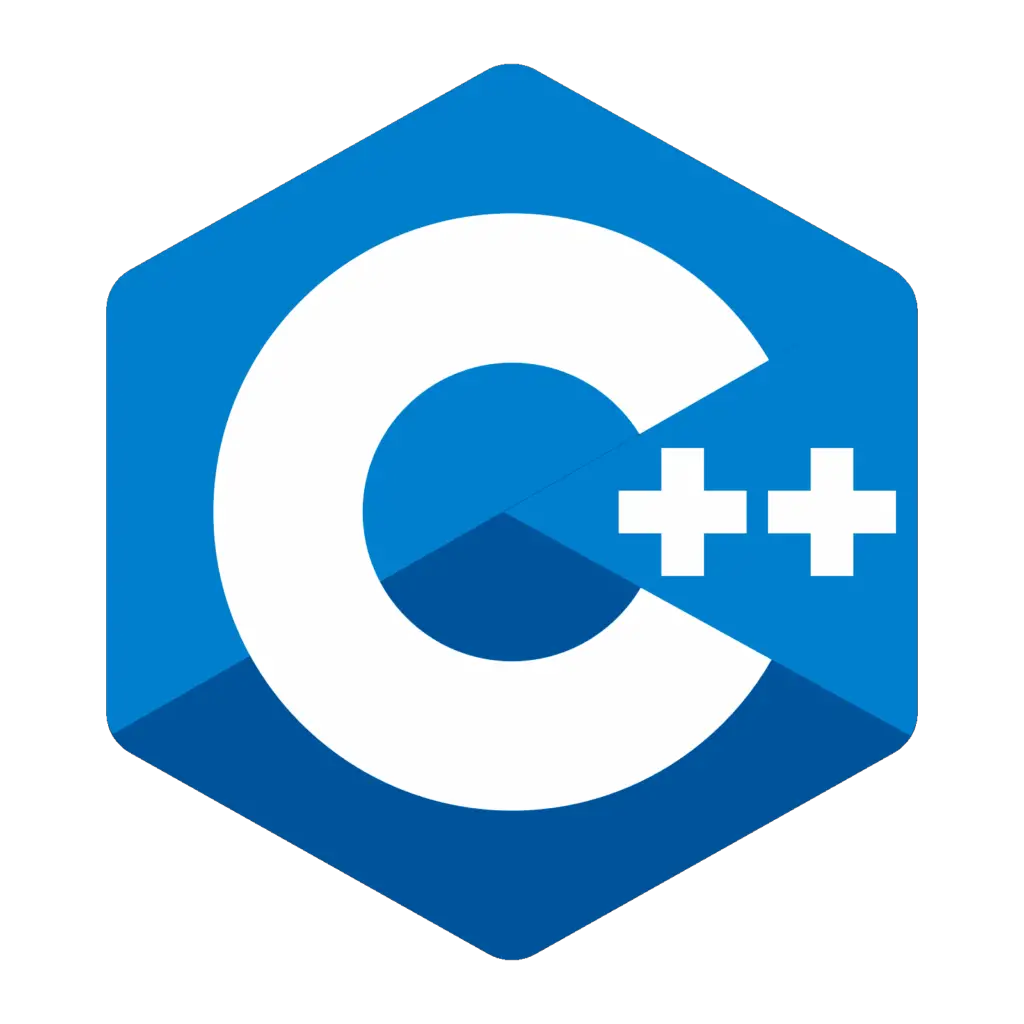
Why to Learn C++?
Learning C++ is a MUST for anyone who wants to become a great Software Engineer, whether they are a student or an employee.
I will list some of the most important reasons why you should learn C++:
- C++ is close to hardware, so you can work at a low level for better memory management, performance, and software development.
- C++ programming teaches object-oriented programming. Implementing virtual tables, virtual table pointers, or dynamic type identification will help you understand low-level polymorphism.
- C++ is one of the most popular programming languages, and software developers love it. If you’re a great C++ programmer, you’ll never be unemployed and will be well paid.
- C++ is the most popular programming language for both system and application programming. So you can choose the part of software development that you are most interested in.
- C++ helps you learn the difference between a compiler, a linker, and a loader, as well as the different data types, storage classes, variable types, their scopes, and so on.
Hello World using C++
C++ is an extension of the C programming language that implements object-oriented concepts.
I will give you a small, standard C++ "Hello World"
program to get you excited about programming in C++.
Here’s a simple Hello World program.
#include <iostream>
using namespace std;
// main() is where program execution begins.
int main() {
cout << "Hello World"; // prints Hello World
return 0;
}
There are a lot of C++ compilers you can use to compile and run the program above:
- Apple C++. Xcode
- Bloodshed Dev-C++
- Clang C++
- Cygwin (GNU C++)
- Mentor Graphics
- MINGW – “Minimalist GNU for Windows”
- GNU CC source
- IBM C++
- Intel C++
- Microsoft Visual C++
- Oracle C++
- HP C++
There are so many compilers out there that it would be impossible to list them all here.
The C++ community is simply too large and constantly evolving.
Applications of C++ Programming
C++ is a widely used programming language, as previously mentioned. It can be found almost everywhere in the software development process.
I’ll list some of them here:
- Application Software Development: Most major operating systems, like Windows, Mac OSX, and Linux, were made with C++ programming. Aside from operating systems, C++ is also used to write the main parts of many browsers like Mozilla Firefox and Chrome. C++ was also used to make MySQL, the most popular database system.
- Creating new programming languages: C++ has been used extensively to create new programming languages like C#, Java, JavaScript, Perl, UNIX’s C Shell, PHP, Python, Verilog, and others.
- Computational Programming: Scientists love C++ because it is fast and helps them do their work more efficiently.
- Games Development: C++ is very fast, which lets programmers use procedural programming for functions that use a lot of CPU time and gives them more control over hardware. Because of this, it has been used a lot to build gaming engines.
- Embedded System: C++ is used a lot to make software for MRI machines, high-end CAD/CAM systems, and other medical and engineering software.
This list goes on, and there are many other ways that software developers use C++ to make great programs.
I think you should learn C++ and help the community by creating great software.
Audience
This C++ Programming tutorial was made for people just starting with C++ to help them learn everything from the basics to more advanced ideas.
Prerequisites
Before you try out the different examples in this C++ programming tutorial, you should know the basics of using a computer program and a computer programming language.