What are Checked Exceptions in Java?
In Java, a checked exception, also called a logical exception, is something that has gone wrong in your code but might be fixable.
At the time of compilation, these exceptions are checked.
If some code inside a method throws a checked exception, the method must either handle the exception or specify it using the throws keyword.
The following listed below are 2 types of exceptions in Java:
- Fully check exceptions
- Partially check exceptions
For example, the following Java program opens the file at “C:\test\a.txt” and prints the first three lines of it.
The program doesn’t compile because the function main() calls FileReader(), and FileReader() throws a checked exception called FileNotFoundException.
It also uses the readLine() and close() methods, which both throw checked exceptions. IOException.
Example:
// program created by Glenn
// Java Program to Illustrate Checked Exceptions
// Where FileNotFoundException occurred
// Importing I/O classes
import java.io.*;
// Main class
public class PIES {
// Main driver method
public static void main(String[] args)
{
// Reading file from path in local directory
FileReader file = new FileReader("C:\\test\\a.txt");
// Creating object as one of ways of taking input
BufferedReader fileInput = new BufferedReader(file);
// Printing first 3 lines of file "C:\test\a.txt"
for (int counter = 0; counter < 3; counter++)
System.out.println(fileInput.readLine());
// Closing file connections
// using close() method
fileInput.close();
}
}
Output:
/PIES.java:16: error: unreported exception FileNotFoundException; must be caught or declared to be thrown
FileReader file = new FileReader(“C:\test\a.txt”);
^
/PIES.java:23: error: unreported exception IOException; must be caught or declared to be thrown
System.out.println(fileInput.readLine());
^
/PIES.java:27: error: unreported exception IOException; must be caught or declared to be thrown
fileInput.close();
^
3 errors
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
To fix the above program, we need to either list the exceptions using throws or use a try-catch block.
The program below uses throws. Since FileNotFoundException is a subclass of IOException, we can just put IOException in the throws list and make the above program compile without any errors.
Example:
// program crreated by Glenn
// Java Program to Illustrate Checked Exceptions
// Where FileNotFoundException does not occur
// Importing I/O classes
import java.io.*;
// Main class
public class PIES {
// Main driver method
public static void main(String[] args)
throws IOException
{
// Creating a file and reading from local repository
FileReader file = new FileReader("C:\\test\\a.txt");
// Reading content inside a file
BufferedReader fileInput = new BufferedReader(file);
// Printing first 3 lines of file "C:\test\a.txt"
for (int counter = 0; counter < 3; counter++)
System.out.println(fileInput.readLine());
// Closing all file connections
// using close() method
// Good practice to avoid any memory leakage
fileInput.close();
}
}
Output:
The First three lines of file “C:\test\a.txt”
What are Unchecked Exceptions in Java?
An Unchecked Exception In Java, also called a runtime exception, is something that has gone wrong with a Java program and can’t be fixed.
These are the exceptions that aren’t checked at compile time. All exceptions are unchecked in C++, so the compiler doesn’t force you to either handle or specify the exception.
Look at the next Java program. It compiles fine, but when it’s run, it throws an ArithmeticException.
ArithmeticException is an unchecked exception, so the compiler lets it compile.
Example:
// program created by Glenn
// Java Program to Illustrate Un-checked Exceptions
// Main class
public class PIES {
// Main driver method
public static void main(String args[])
{
// Here we are dividing by 0
// which will not be caught at compile time
// as there is no mistake but caught at runtime
// because it is mathematically incorrect
int g = 1;
int x = 20;
int z = g / x;
}
}
Output:
Exception in thread “main” java.lang.ArithmeticException: / by zero
at Main.main(Main.java:5)
Java Result: 2
What is the Difference Between Unchecked and Checked Exceptions?
The Difference Between Unchecked And Checked Exceptions Checked Exceptions are checked when the program is running, but Unchecked Exceptions are checked when the program is being compiled.
What are the Different Types of Exceptions in Java?
The following listed below are the two main types of exceptions in Java.
- Checked exception
- Unchecked exception
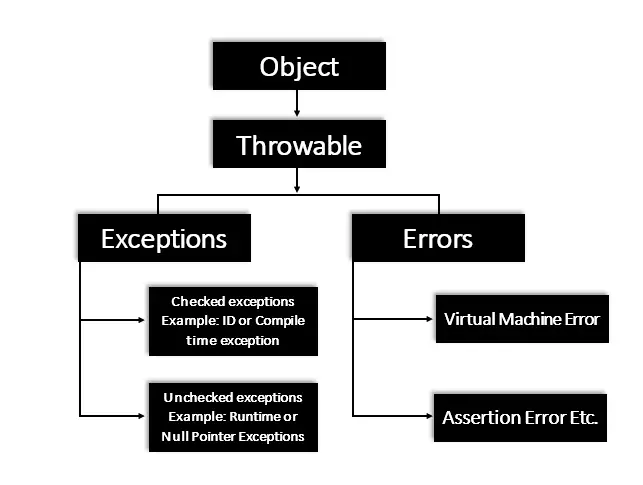
How to Handle Multiple Exceptions in Java?
The only way to Handle Multiple Exceptions In Java is must use the Java multi-catch block.
One or more catch blocks can come after a try block. Each catch block must have its own handler for an error.
So, if you need to do different things when different exceptions happen, you can use the Java multi-catch block.
Java Exceptions Try Catch
The try statement lets you set up a block of code that will be checked for errors as it runs.
With the catch statement, you can set up a block of code to run if an error happens in the try block.
Syntax of Java Exceptions Try Catch
try {
// Basic syntax
// Block of code to try
}
catch(Exception e) {
// Block of code to handle errors
}
Parent Class of All Exceptions in Java
The Parent Class Of All Exceptions In Java is the java.lang.Exception.
The class Exception and its subclasses are type of Throwable that shows situations that an application might want to catch.
The following figure shows the different kinds of Java exceptions.
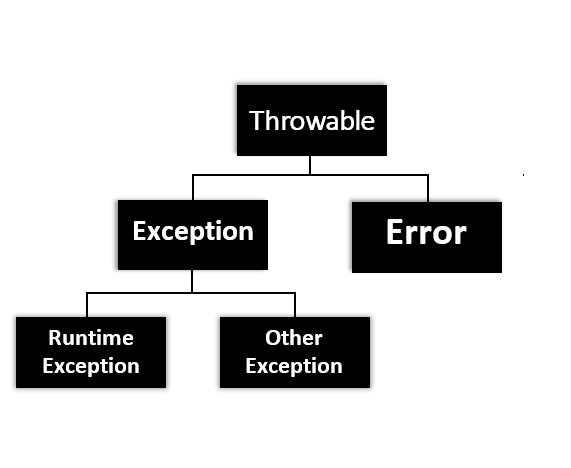
Errors vs Exceptions In Java
Errors are usually caused by the environment where the application is running.
For instance, an error will happen when there aren’t enough system resources.
Exceptions are created by the code of the application itself. Errors can’t be fixed.
How to Throw Multiple Exceptions in Java?
In Java, we might have to deal with several exceptions. You can’t throw multiple exceptions in Java.
We can list multiple exceptions, but only one will be thrown.
But there are a few other ways we can simulate throwing more than one exception.
When we need to handle more than one exception, we can use “chained exceptions.”
These kinds of exceptions mean that the one that was thrown was caused by another exception.
Example:
// program created by Glenn
public class Main
{
public static void main(String[] args)
{
try
{
NumberFormatException ex =
new NumberFormatException("NumberFormatException is thrown");
ex.initCause(new NullPointerException(
"NullPointerException is the main cause"));
throw ex;
}
catch(NumberFormatException ex)
{
System.out.println(ex);
System.out.println(ex.getCause());
}
}
}
Output:
java.lang.NumberFormatException: NumberFormatException is thrown
java.lang.NullPointerException: NullPointerException is the main cause
You can test the above example here! ➡Java Online Compiler
When do Exceptions in Java Arise in Code Sequence?
Exceptions in Java arise in code sequence in Run Time.
Because Exceptions are determined at run time in Java, they are errors that happen at run time.
What are Runtime Exceptions in Java With Example?
Runtime Exceptions In Java are things that happen inside your application and usually can’t be fixed.
For example, a null object is one that should have a value but doesn’t.
A NullPointerException exception would be thrown in this case.
The following are the 10 most common examples of runtime exceptions in Java:
- ArithmeticException
- NullPointerException
- ClassCastException
- DateTimeException
- ArrayIndexOutOfBoundsException
- NegativeArraySizeException
- ArrayStoreException
- UnsupportedOperationException
- NoSuchElementException
- ConcurrentModificationException
Summary
In summary, Checked Exceptions in Java are errors that occur at runtime but are checked at compile time.
They must be either handled using try-catch blocks or specified using the throws keyword.
On the other hand, Unchecked Exceptions, also known as runtime exceptions, are not checked at compile time and typically indicate irrecoverable errors in the program.
The key difference between them is when they are checked—Checked Exceptions during compilation, and Unchecked Exceptions during runtime.
What’s Next
The next section talks about Inner Class In Java. At the end of the session, you’ll know what Inner Class is all about in Java.
< PREVIOUS
NEXT >