This Basic Java Syntax serves as a fundamental learning guide for you to master Java and become a good Java developer in the future.
What is Basic Syntax In Java?
The Basic Java Syntax is the most important rules of a programming language.
Without these rules, it is impossible to write code that works. Each language has its own set of rules that make up its basic syntax.
Naming conventions are an important part of Basic Syntax conventions, and they vary from language to language.
When we think of a Java program, it may be described as a group of objects that interact by calling each other’s methods.
Now let’s take a quick look at what class, object, methods, and instance variables mean.
Class
Basic Java Classes can be thought of as a model or blueprint that specifies the actions or conditions that a certain type of object can take.
Object
Objects have both states and behaviors.
Example: A dog has states like color, name, and breed, as well as behaviors like wagging their tail, barking, and eating. An object is a single instance of a class.
Methods
A method is essentially a behavior. A class can have many methods. In methods, the logic is written, the data is changed, and all the actions are executed.
Instance Variables
Each object has a set of instance variables that are unique to it. The values that are given to these instance variables make up the state of an object.
Example Java Program
Let’s look at a simple code that will print Hello World.
public class helloWorld { /* This is my first java program . * This will print 'Hello World' as the output * This Tutorial is made by Glenn Magada Azuelo */ public static void main(String []args) { //System.out.println is a command that can print the output of the program System.out.println("Hello World"); // print Hello World } }
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you don’t have it, you can download it. Here’s the link. NetBeans IDE.
You can also test the above example here! ➡Java Online Compiler
Java Basic Syntax – Output
Hello World
Now that we have already seen how to run this sample code in the Java Online Compiler, we will now take a look at how this sample code runs in my favorite code editor, which is NetBeans.
You can actually run this code in different code editors like Eclipse, IntelliJ, and so on. It depends on what you like.
Please follow the steps.
Time needed: 5 minutes
- Step 1 : Open NetBeans and Create New Project.
- Step 2 : Press Java Application and Press Next.
- Step 3 : Name your project, for example, ‘helloWorld’. Any name will do. Lastly Press Finish the button.
- Step 4 : Look for the project name that you’ve been created, then press the Source Packages folder.
Once you already open, you will see the default package folder.
Right click on it and drag the New button and press Java Class. - Step 5 : Create the file name of the new Java class.
Once you’re done naming the class, just press the Finish button. - Step 6 : Copy and Paste the example code in the Java Class.
- Step 7 : Right Click and press Run File button.
NetBeans Project Output
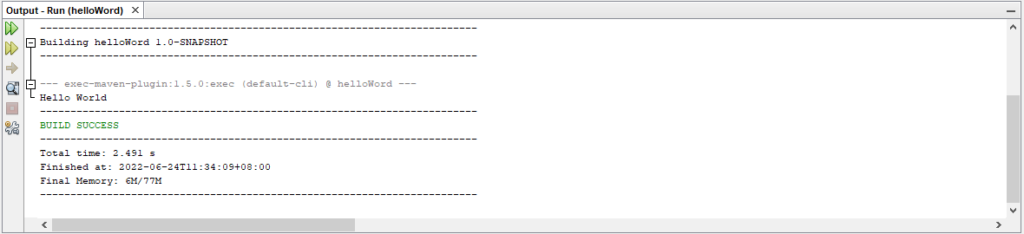
Basic Syntax of Java
The following things are very important to keep in mind about Java programs:
- Case Sensitivity – Java has a case-sensitive syntax, thus identifier In Java, the words hello and hello would indicate different meaning.
- Class Names – All class names must begin with an Upper Case letter. If the class name consists of multiple words, the first letter of each inner word should be capitalized.
Example : class MyFirstProgramInJava
- Method Names – The first letter of every method name must be Lower Case. If the method’s name is composed of numerous words, the first letter of each inner word should be Upper Case.
Example : public void myMethodName()
- Program File Name – The class name must exactly match the name of the program file.
Remembering that Java is case sensitive, you should save the file with the class name and append.java to the end of the name (if the file name and the class name do not match, your program will not compile).
Please keep in mind, however, that the file name can differ from the class name if there isn’t a public class present in the file.
Additionally, including a public class in the file is optional.
Example : Let’s assume MyFirstProgramInJava is the class name, so it means that the file name should save as MyFirstProgramInJava.java
- public static void main(String []args) – The main() method, which is an essential component of every Java program, is where Java program processing begins.
Identifiers in Java
The Identifiers In Java are the names that are used to identify things like classes, methods, variables, packages, and so on in Java.
Every Java component needs names. Identifiers are the names given to classes, variables, and methods.
There are various identifier-related Java concepts to keep in mind.
The list is as follows:
- Each and every identifier must start with a letter (from A to Z or a to z), a dollar sign ($), or an underscore (_).
- Identifiers may consist of any number of characters after the first character.
- A key word can’t be used to be an identifier.
- Identifiers are case-sensitive, which is the most important feature.
- Age, salary, value, and __1 value are a few examples of legal identifiers.
- 123abc and -salary are examples of illegal identifiers.
Modifiers in Java
The Modifiers In Java can be used to change classes, methods, etc., just like in other languages.
There are two types of categories of modifiers.
- Access Modifiers – default, protected, public, and private.
- Non-Access Modifiers – abstract, strictfp, and final.
In the part after this, we’ll explore modifiers in further detail.
Variable in Java
In Java, a variable is a place where data is stored while a Java program is running.
Every variable has a data type that tells what kind of value it can hold and how many of them it can hold.
A variable is the name of a place in memory where data is stored.
A variable is a place in memory that is given a name.
The types of Variable In Java are as follows:
- Local Variables
- Instance Variables (Non-Static Variables)
- Class Variables (Static Variables)
Arrays in Java
The Arrays In Java are objects that hold many variables of the same type.
However, an arrays is itself an object on the heap.
In the next chapters, we will look at how to declare, construct, and initialize.
Enums in Java
A Java Enum is a special type of Java code that is used to define groups of constants.
To be more specific, a Java enum type is a kind of Java class.
An enum can contain constants, methods etc. Enums were added to Java in version 5.
By using enums, you can cut down on the number of bugs in your code.
For example, if someone wanted to open a T-Shirt Store, the T-Shirt sizes could be limited to small, medium, and large.
This would make sure that no one could order any size besides small, medium, or large.
Example Program for Enums in Java
class SizeTshirt { enum SizeOfTshirt{ SMALL, MEDIUM, LARGE } SizeOfTshirt size; } public class Tshirt { public static void main(String args[]) { SizeTshirt size = new SizeTshirt(); size.size = SizeTshirt.SizeOfTshirt.MEDIUM ; System.out.println("Size: " + size.size); } }
You can run this code in your code editor if you already downloaded it to your laptop or PC, but if you don’t, you can download it.
You can test the above example here! ➡Java Online Compiler
Output:
Size: MEDIUM
Note – You can declare an enums on its own or inside a class. enums can also have methods, variables, and constructors.
Keywords in Java
Here is a list of the Keywords In Java that can’t be used. These special words can’t be used as names for constants, variables, or identifier names.
abstract | assert | boolean | break |
byte | case | catch | char |
class | const | continue | default |
do | double | else | enum |
extends | final | finally | float |
for | goto | if | implements |
import | instanceof | int | interface |
long | native | new | package |
private | protected | public | return |
short | static | strictfp | super |
switch | synchronized | this | throw |
throws | transient | try | void |
volatile | while |
Comments In Java
Java allows both single-line and multiple-line comments, just like C and C++.
The Java compiler ignores any character that is inside a comment.
Example:
public class helloWorld { /* This is my first java program . * This will print 'Hello World' as the output * This Tutorial is made by Glenn Magada Azuelo */ public static void main(String []args) { //System.out.println is a command that can print the output of the program System.out.println("Hello World"); // print Hello World } }
You can run this code in your code editor if you already downloaded it to your laptop or PC, but if you don’t, you can download it.
You can test the above example here! ➡Java Online Compiler
Output:
Hello World
Using Blank Lines In Java
A line with only white space, possibly with a comment, is called a “blank line,” and Java ignores it completely.
Inheritance In Java
Classes can be made from other classes in Java.
If you need to make a new class and there is already a class with some of the code you need, you can derive your new class from the code that is already there.
With this idea, you can use the fields and methods of an existing class without having to write the code all over again in a new class.
In this case, the class that already exists is called the “superclass,” and the class that was made from it is called the “subclass.”
Interfaces In Java
In the Java programming language, an interface is a deal between two objects about how to talk to each other.
Interfaces are a very important part of the idea of inheritance.
An interface tells a deriving class (also called a subclass) which methods it should use.
But it’s up to the subclass to decide how to use the methods.
Summary
In summary, you have learned about Java Basic Syntax. This tutorial has covered the following topics: What is Basic Syntax In Java, Identifiers In Java, Modifiers In Java, Variable In Java, Arrays In Java, Enums In Java, Keywords In Java, Comments In Java, Inheritance In Java and Interfaces In Java.
I hope this lesson has helped you learn what is Java Basic Syntax and how to use them.
What’s Next
In the next section, we will talk about Objects and Classes in Java programming. At the end of the session, you’ll know what objects and classes are in Java.
< PREVIOUS
NEXT >
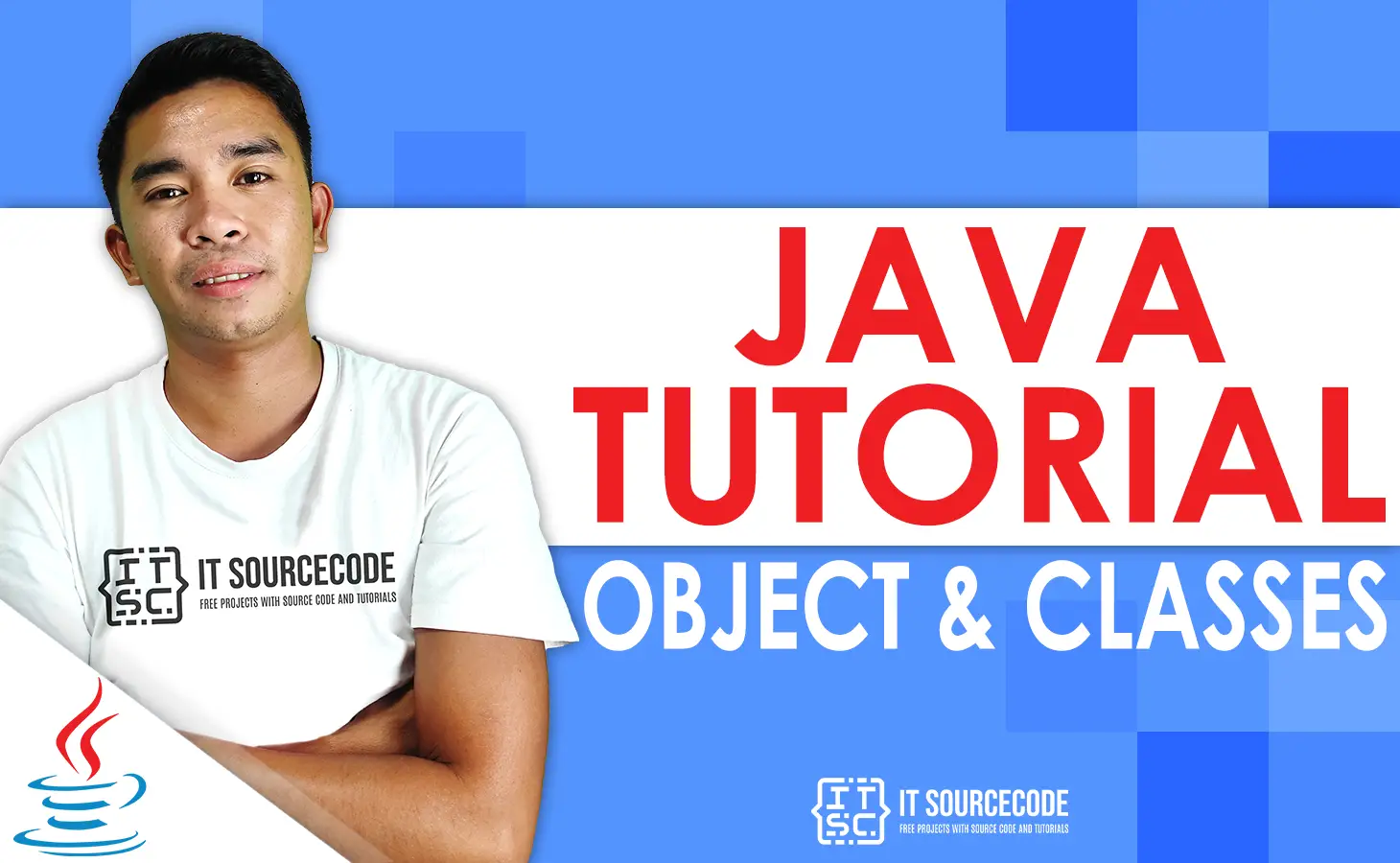
Java Object & Classes