How to Implement Interface in Java? Adding an implement clause to the class declaration allows you to declare a class that complies with an interface.
A list of the interfaces that your class implements, separated by commas, comes after the keyword implements since your class may implement more than one interface.
What is an interface in Java?
An interface is a completely “abstract class” that is used to group functions with empty bodies that do similar things.
Example:
// interface in Java
interface Cat {
public void carSound(); // interface method (does not have a body)
public void run(); // interface default method (does not have a body)
}
To gain access to the interface’s methods, another class must “implement” the interface by using the implements keyword (instead of the extends keyword).
This is analogous to inheriting the interface’s functionality.
The “implement” class is in charge of providing the body of the abstract method defined, which is often called the “implementation.”
Example:
// Interface
interface Car {
public void carSound(); // interface method (does not have a body)
public void start(); // interface method (does not have a body)
}
// BMW "implements" the Car interface
class BMW implements Car {
public void carSound() {
// The body of carSound() is provided here
System.out.println("Brooommm Brooommm broooommmmm");
}
public void start() {
// The body of sleep() is provided here
System.out.println("Start engine");
}
}
public class Main {
public static void main(String[] args) {
BMW myCar = new BMW();
myCar.start();
myCar.carSound();
}
}
Output:
Start engine Brooommm Brooommm broooommmmm
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
How to declare an Interface in Java?
The interface keyword is used to declare an interface. It is applied to offer complete abstraction.
This means that by default, all fields in an interface are public, static methods, and final and that all methods in an interface are declared with an empty body.
Example of an interface in Java
Let us look at a Java Interface example that is more useful.
// program created by Glenn
// To use the sqrt function
interface Polygon {
void getArea();
// calculate the perimeter of a Polygon
default void getPerimeter(int... sides) {
int perimeter = 0;
for (int side: sides) {
perimeter += side;
}
System.out.println("Perimeter: " + perimeter);
}
}
class Triangle implements Polygon {
private int a, b, c;
private double s, area;
// initializing sides of a triangle
Triangle(int a, int b, int c) {
this.a = a;
this.b = b;
this.c = c;
s = 0;
}
// calculate the area of a triangle
public void getArea() {
s = (double) (a + b + c)/2;
area = Math.sqrt(s*(s-a)*(s-b)*(s-c));
System.out.println("Area: " + area);
}
}
public class Main {
public static void main(String[] args) {
Triangle t1 = new Triangle(6, 5, 7);
// calls the method of the Triangle class
t1.getArea();
// calls the method of Polygon
t1.getPerimeter(6, 5, 7);
}
}
Implementation of interfaces
To declare a class that uses an interface, add a “implements” clause.
Your class can implement more than one interface, so the word implement is followed by a comma-separated list.
What is the use of the interface in Java?
In Java, an interface provides an abstract type that describes how a class should act.
This technology supports abstraction, polymorphism, and multiple inheritance, which are all core concepts of Java.
In Java, abstraction is done using interfaces.
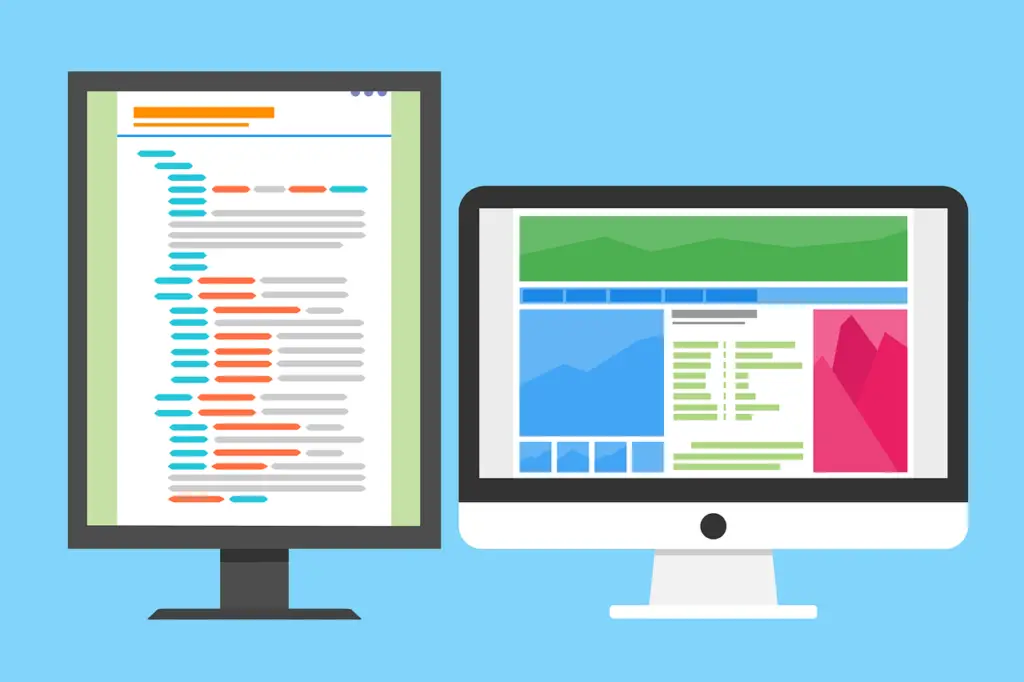
Extending multiple interfaces
There is only one parent class that a Java class can extend. There is a restriction to implement multiple interfaces.
As opposed to classes, interfaces can extend several parent interfaces and are not considered to be classes.
A comma separates the list of parent interfaces from the extends keyword, which is only used once.
Extending interfaces
A class can extend another class in the same way that an interface can extend another interface.
The extends keyword is used to add to an interface, and the methods of the parent interface are passed on to the child interface.
Example:
// Filename: Sports.java
public interface Sports {
public void setHomeTeam(String name);
public void setVisitingTeam(String name);
}
// Filename: Basketball.java
public interface Basketball extends Sports {
public void homeShotScored(int points);
public void visitingShotScored(int points);
public void endOfQuarter(int quarter);
}
// Filename: SepakTakraw.java
public interface SepakTakraw extends Sports {
public void homeKickScored();
public void visitingKickScored();
public void endOfPeriod(int period);
public void overtimePeriod(int ot);
}
The Sepak Takraw interface has four methods, but it gets two from Sports.
This means that a class that implements Sepak Takraw must implement all six methods.
In the same way, a class that implements Basketball needs to define the three Basketball methods and the two Sports methods.
Advantages of the interface in Java
Here are the most important reasons why you should use an interface in Java.
- Easier to test
- Faster development
- Increased flexibility
- Loosely couple code
- Dependency Injection
Easier to test
One of the best things about using an interface in Java programming is that unit testing is easier.
DAO and parser classes are good examples. When testing, you can substitute interface dependencies with a mock, stub, or dummy.
Faster development
This is the fourth practical benefit of the Java interface I’ve seen. In a real project, your work depends on your partner’s or team’s.
Increased flexibility
The third benefit of the Java interface is that it makes code more flexible.
Loosely couple code
The fourth benefit of Java interface is that it makes loosely coupled programming easier to adapt and update. In loosely coupled code, you can modify bits without affecting the complete structure.
Dependency Injection
Lastly, the injection of dependencies is based on an interface.
In DI, instead of a developer putting in dependencies, the framework does it.
This is done by declaring dependencies as interfaces.
Summary
In summary, you have learned about interfaces in Java.
This article discusses what is an interface in Java, how to declare an interface in Java, an example of an interface in Java, use of an interface in Java, Interface Implementation, Extending Multiple Interfaces, Extending Interfaces, and Interface Advantages in Java
I hope this lesson has helped you learn a lot.
Check out my previous and latest articles for more life-changing tutorials which could help you a lot.
What’s Next
The next section talks about Packages. At the end of the session, you’ll know what is Packages all about in Java.
< PREVIOUS
NEXT >
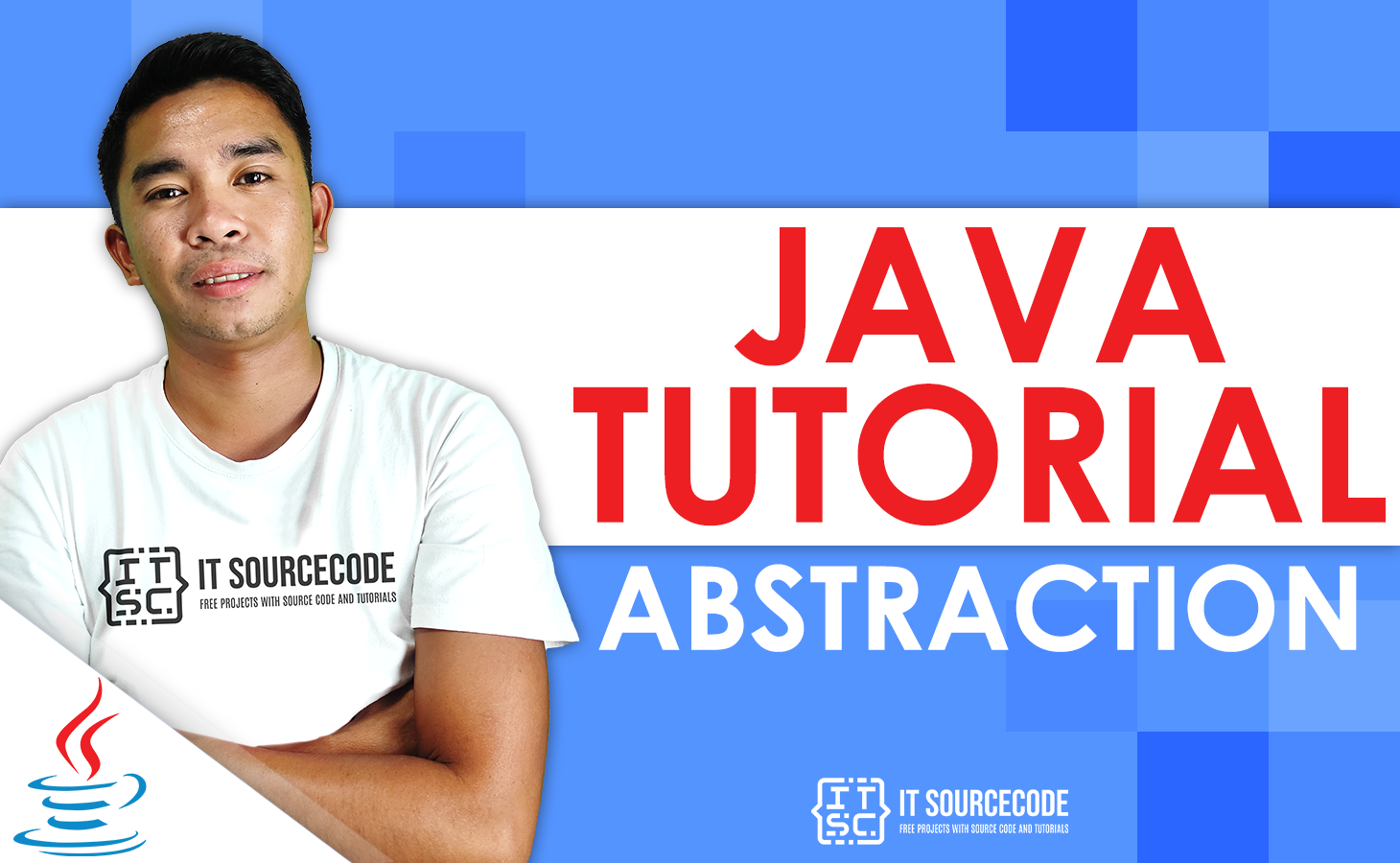
Java Packages