What is Class and Object in Java?
In Java, everything is linked to classes and objects, as well as their attributes and methods.
In real life, a car is an example of an object.
The car has things like weight and color, and it can be driven and stopped.
A Class is like an object constructor or a “blueprint” for making objects.
Object-Oriented Programming Basics
Java is a language that is based on objects.
Java is an Object-Oriented language, which means that it supports the following basic ideas which are Classes And Objects In Java.
- Polymorphism
- Inheritance
- Encapsulation
- Abstraction
- Classes
- Objects
- Instance
- Method
- Message Passing
Difference Between Class And Object In Java
The Difference Between Class And Object In Java is that a Class is a template that is used to make objects and define the data types and methods of those objects.
Classes are like groups, while Objects are the things that belong to each group.
All objects of a class should have the basic properties of that class.
Without further ado, I will further explain the Classes And Objects In Java.
What is a Class in Java?
A Class in Java is a group of objects that have similar properties.
It is a plan or template from which objects are made. It is a logical thing. It can’t be a physical thing.
Lists of Class in Java:
- Fields
- Methods
- Constructors
- Blocks
- Nested Class And Interface
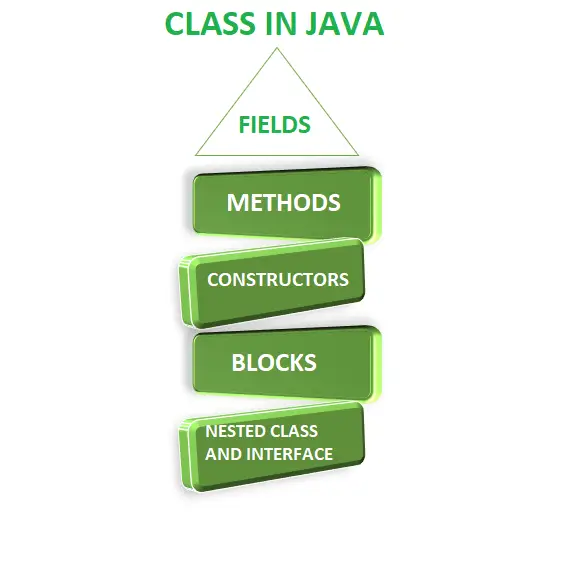
Syntax On How To Declare a Class In Java
class <class_name> {
field;
method;
}
What is an Object in Java?
The Object In Java is a member of a Java class, which is also called an instance.
Each thing has a name, a way it acts, and a state. Fields (variables) store the state of an object, while methods (functions) show how the object acts.
At run time, objects are made from templates, which are also called classes.
Fields (variables) store an object’s state, whereas methods (functions) show the object’s action.
Runtime objects are built from templates, also referred to as classes.
An object is an entity with state and behavior, such as a bag, books, laptop, and airplane. It could be logical or physical (tangible and intangible).
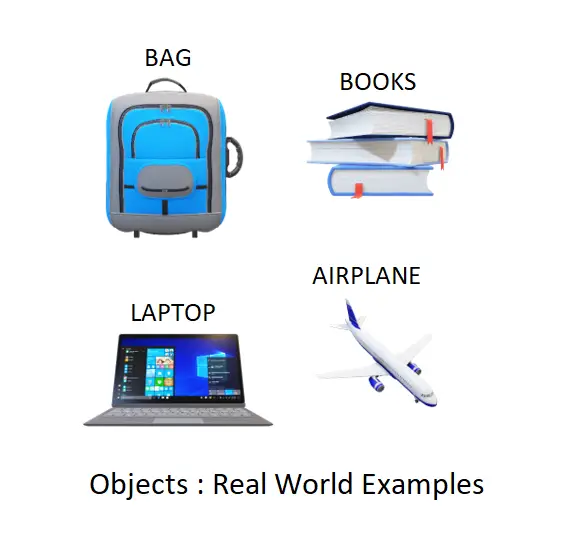
The following are lists of categories of Objects In Java.
- State – represents an object’s data, which is called its value.
- Behavior – represents how an object acts (what it can do), like deposit, withdraw, etc.
- Identity – A unique ID is often used to give an object an identity. The external user can’t see what the value of the ID is. However, the JVM uses it to give each object a unique identifier.
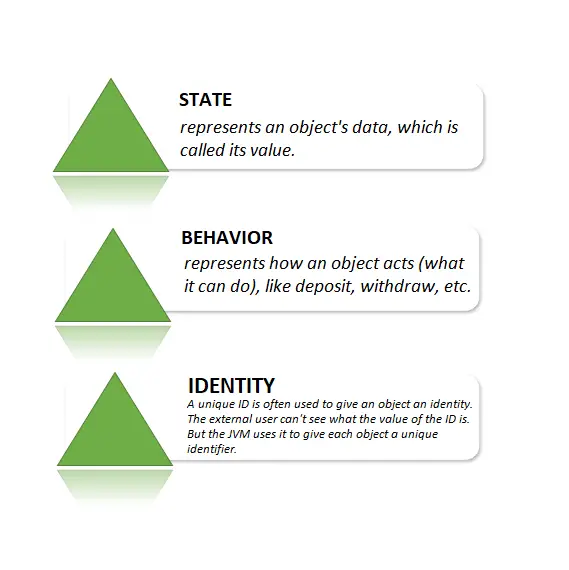
A pen is an example of an Object. Its name is Reynolds, and white is his name and state. Since it is used to write, writing is his behavior.
Object Definitions
- A real-world thing is an object.
- A runtime entity is an object.
- The object is an entity with a state and behavior.
- The object is a specific instance of a class.
Instance Variable In Java
The Instance Variable In Java is a variable that is created inside the class but not in a method.
At compile time, an instance variable doesn’t get any memory. When an object or instance is created, it gets memory at runtime.
It is because of this, it is called an instance variable.
Method In Java
The Method In Java is a blocks of code that only run when they are called.
You can send parameters, which are pieces of data, into a method.
Methods are ways to do things, and they are also called functions.
Advantage of Method In Java
The following are lists of methods in Java.
- Code Reusability
- Code Optimization
New Keyword In Java
The New Keyword In Java is a Java operator that creates an object.
Initialization: The new operator is followed by a call to a constructor, which sets up the new object.
Object And Class In Java With Example – Main Within The Class
In this example, we’ve made a class called Student with two data members: id and name.
By using the new keyword, we’re making an object of the Student class and printing the object’s value.
Here, we’re creating a main() method inside the class.
//Java Program to illustrate how to define a class and fields //Defining a Student class. class Student{ //defining fields int id;//field or data member or instance variable String name; //creating main method inside the Student class public static void main(String args[]){ //Creating an object or instance Student s1=new Student();//creating an object of Student //Printing values of the object System.out.println(s1.id);//accessing member through reference variable System.out.println(s1.name); } }
Output:
0
null
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you don’t have it, you can download it. Here’s the link. NetBeans IDE, but if you wish to run this code online.
You can test the above example here! ➡Java Online Compiler
Object And Class In Java With Example – Main Outside The Class
In real-time development, we make classes and use them in other classes.
It’s a better plan than the one we were using before. Let’s look at a simple case where the main() method is in a different class.
We can have more than one class in a single Java file or in separate Java files.
If you define more than one class in the same Java source file, you should save the file with the name of the class that has the main() method.
//Java Program to demonstrate having the main method in //another class //Creating Student class. class Student{ int id; String name; } //Creating another class TestStudent1 which contains the main method public class TestStudent1{ public static void main(String args[]){ Student s1=new Student(); System.out.println(s1.id); System.out.println(s1.name); } }
You can test the above example here! ➡Java Online Compiler
Output
0
null
3 Ways To Initialize Object
The following are three ways to initialize an object in Java.
- By Reference Variable
- By Method
- By Constructor
1. Object And Class In Java With Example – Initialization through reference
Initializing an object means storing data in the object. Let’s look at a simple case where we’ll use a reference variable to set up an object.
class Student{ int id; String name; } public class TestStudent2{ public static void main(String args[]){ Student s1=new Student(); s1.id=211; s1.name="Glenn"; System.out.println(s1.id+" "+s1.name);//printing members with a white space } }
You can test the above example here! ➡Java Online Compiler
Output:
211 Glenn
We can also create multiple objects and store information in them using reference variables.
class Student{ int id; String name; } public class TestStudent3{ public static void main(String args[]){ //Creating objects Student s1=new Student(); Student s2=new Student(); //Initializing objects s1.id=211; s1.name="Glenn"; s2.id=212; s2.name="Jude"; //Printing data System.out.println(s1.id+" "+s1.name); System.out.println(s2.id+" "+s2.name); } }
You can test the above example here! ➡Java Online Compiler
Output:
211 Glenn
212 Jude
2. Object And Class In Java With Example – Initialization through method
In this example, we’re making two objects of the Student class and setting their values by calling the insertRecord method.
By calling the displayInformation() method, we show the state (data) of the objects in this case.
class Student{ int rollno; String name; void insertRecord(int r, String n){ rollno=r; name=n; } void displayInformation(){System.out.println(rollno+" "+name);} } public class TestStudent4{ public static void main(String args[]){ Student s1=new Student(); Student s2=new Student(); s1.insertRecord(122,"Glenn"); s2.insertRecord(123,"Jude"); s1.displayInformation(); s2.displayInformation(); } }
You can test the above example here! ➡Java Online Compiler
Output:
122 Glenn
123 Jude
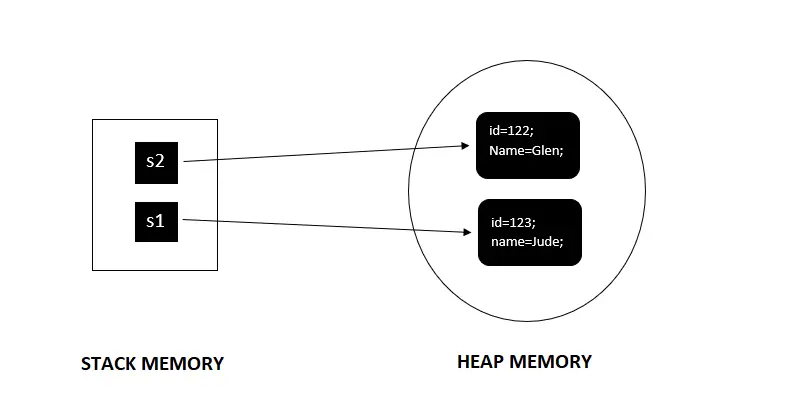
As you can see in the figure above, the memory for an object is stored in the heap memory area.
The object in the heap memory area is linked to the reference variable.
In this case, s1 and s2 are both reference variables that point to objects in memory.
3. Object And Class In Java With Example – Initialization through constructors
We will further discuss constructors in the next chapter of this course.
Object And Class In Java With Example – Employees
Let’s look at an example of how we keep track of employees.
class Employee{ int id; String name; float salary; void insert(int i, String n, float s) { id=i; name=n; salary=s; } void display(){System.out.println(id+" "+name+" "+salary);} } public class TestEmployee { public static void main(String[] args) { Employee e1=new Employee(); Employee e2=new Employee(); Employee e3=new Employee(); e1.insert(101,"Glenn",10000); e2.insert(102,"Adones",25000); e3.insert(103,"Jude",55000); e1.display(); e2.display(); e3.display(); } }
You can test the above example here! ➡Java Online Compiler
Output:
101 Glenn 10000.0
102 Adones 25000.0
103 Jude 55000.0
Object And Class In Java With Example – Rectangle
Another example is given that keeps track of the records of the Rectangle class.
class Rectangle{ int length; int width; void insert(int l, int w){ length=l; width=w; } void calculateArea(){System.out.println(length*width);} } public class TestRectangle1{ public static void main(String args[]){ Rectangle r1=new Rectangle(); Rectangle r2=new Rectangle(); r1.insert(21,5); r2.insert(32,15); r1.calculateArea(); r2.calculateArea(); } }
You can test the above example here! ➡Java Online Compiler
Output:
105
480
What are the Different Ways to Create an Object In Java
There are many ways to make an Object In Java.
- By new keyword
- By newInstance() method
- By factory method etc.
- By clone() method
- By deserialization
We will learn how to make objects in these ways in the next chapter.
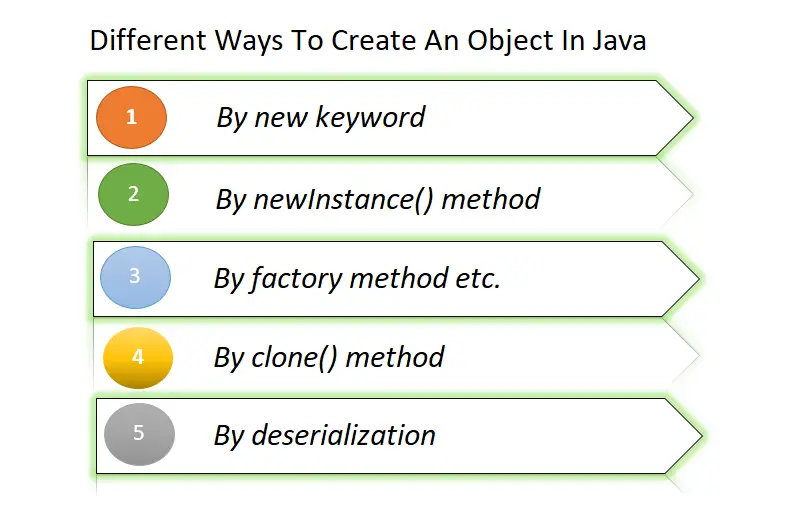
Anonymous Object
The Anonymous Object is a value that doesn’t have a name.
Because they don’t have names, there is no way to talk about them after they are made.
So, they have “expression scope,” which means that they are created, evaluated, and thrown away all in the same expression.
An anonymous object is a good choice if you only need to use an object once.
For example:
new Calculation();//anonymous object
Using a reference to call a method:
Calculation c=new Calculation();
c.fact(6);
Calling a method through an anonymous object.
new Calculation().fact(6);
Let’s look at a full example of what a Java anonymous object looks like.
public class Calculation{ void fact(int n){ int fact=1; for(int i=1;i<=n;i++){ fact=fact*i; } System.out.println("factorial is "+fact); } public static void main(String args[]){ new Calculation().fact(6);//calling method with anonymous object } }
You can test the above example here! ➡Java Online Compiler
Output:
factorial is 720
Creating Multiple Objects By One Type Only
We can make more than one object of the same type, just like we do with primitives.
Setting up primitive variables from scratch:
int a=20, b=50;
Setting up the reference variables:
Rectangle rec1=new Rectangle(), rec2=new Rectangle(); //creating two objects
Here’s an example:
//Java Program to illustrate the use of Rectangle class which //has length and width data members class Rectangle{ int length; int width; void insert(int l,int w){ length=l; width=w; } void calculateArea(){System.out.println(length*width);} } public class TestRectangle2{ public static void main(String args[]){ Rectangle rec1=new Rectangle(),rec2=new Rectangle();//creating two objects rec1.insert(21,54); rec2.insert(13,14); rec1.calculateArea(); rec2.calculateArea(); } }
You can test the above example here! ➡Java Online Compiler
Output:
1134
182
Real-Life Example Program: Account
//Java Program to demonstrate the working of a banking-system //where we deposit and withdraw amount from our account. //Creating an Account class which has deposit() and withdraw() methods class Account{ int acc_no; String name; float amount; //Method to initialize object void insert(int a,String n,float amt){ acc_no=a; name=n; amount=amt; } //deposit method void deposit(float amt){ amount=amount+amt; System.out.println(amt+" deposited"); } //withdraw method void withdraw(float amt){ if(amount<amt){ System.out.println("Insufficient Balance"); }else{ amount=amount-amt; System.out.println(amt+" withdrawn"); } } //method to check the balance of the account void checkBalance(){System.out.println("Balance is: "+amount);} //method to display the values of an object void display(){System.out.println(acc_no+" "+name+" "+amount);} } //Creating a test class to deposit and withdraw amount public class TestAccount{ public static void main(String[] args){ Account a1=new Account(); a1.insert(132345,"Glenn Azuelo",21000); a1.display(); a1.checkBalance(); a1.deposit(1240000); a1.checkBalance(); a1.withdraw(25000); a1.checkBalance(); }}
You can test the above example here! ➡Java Online Compiler
Output:
132345 Glenn Azuelo 21000.0
Balance is: 21000.0
1240000.0 deposited
Balance is: 1261000.0
25000.0 withdrawn
Balance is: 1236000.0
Conclusion
In this article, we talked about the Class And Object In Java, how it works, and how this chapter could help you with your Java studies.
This could be one of the most important learning guides that can help you improve your skills and knowledge as a Java Developer.
What’s Next
The next section talks about Constructors in Java programming. At the end of the session, you’ll know what Constructors are all about in Java.
< PREVIOUS
NEXT >