This is a continuation of the previous topic, entitled String.
With the help of some examples, we will learn how to declare, initialize, and access array elements.
The Java Arrays Are used to store more than one value in a single variable, instead of declaring a separate variable for each value.
To declare an array, define the type of the variable with square brackets: Cars;
Now, we have set up a variable that holds an array of strings.
How to Declare an Array in Java?
We Declare An Array In Java the same way we declare other variables, by giving it a type and a name:
The following example below is a basic syntax on How To Declare an Array In Java.
int[] myArray;
We can use the following shorthand syntax to initialize or instantiate an array as we declare it.
This means that we can assign values to the array at the same time we create it.
int[] myArray = {14, 15, 16};
Or, you could make a stream of values and add them back to the array:
The following example below is another example of adding back to the array.
int[] intArray = IntStream.range(1, 12).toArray();
int[] intArray = IntStream.rangeClosed(1, 12).toArray();
int[] intArray = IntStream.of(1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11).toArray();
Declaration Of Arrays in Java
The same rules apply when declaring an array object in Java as when declaring a variable.
We tell the computer what type of data each array element is and what the name of the variable is.
We also add square brackets [] to show that it is an array.
The following are two ways to declare an array in Java.
int[] intArray;
int intArray[];
What is Arrays in Java
The Arrays In Java is a container object that holds a set number of values of the same type.
When an array is created, its size is decided. Its length is set once it has been made.
You’ve already seen an example of an array in the “Hello World!” application’s main method.
Java Arrays Method
The following listed below are the Top 10 Methods In Java Arrays.
- Print An Array In Java
- Create An ArrayList from an array
- Check if an array contains a certain value
- Concatenate two arrays
- Declare an array inline
- Joins the elements of the provided array into a single String
- Convert an ArrayList to an array
- Convert an array to a set
- Reverse an array
- Remove element of an array
Types of Array in Java
- Single Dimensional Array
- Multi-Dimensional Array
Single Dimensional Array in Java
In Java programming, a One-Dimensional Array is a special type of variable that can hold multiple values of a single data type, like int, float, double, char, structure, pointer, etc., at the same place in computer memory.
Multi-dimensional Array
A Multi-Dimensional Array In Java is one that has more than one level.
For example, a 2D array, which stands for “two-dimensional array,” is a matrix of rows and columns (think of a table).
Adding a third dimension to an array makes it an array of arrays of arrays.
Without further ado, I will further explain Arrays In Java in order for you to easily understand the topic.
The Java Array is a type of data structure in Java that stores a fixed-size collection of elements of the same type in order.
An array is a place to store a group of data, but it is often easier to think of it as a group of variables of the same type.
Instead of declaring individual variables like number0, number1,…, and number99, you declare one array variable like numbers and use numbers[0], numbers[1],…, and numbers[99] to represent individual variables.
This tutorial shows you how to declare array variables, make arrays, and use indexed variables to work with arrays.
Declaring Array Variables
To use an array in a program, you have to declare a variable that points to the array and say what kind of array the variable can point to.
Here is how to set up an array variable.
Syntax For Declaring Array
// sample created by Glenn
dataType[] arraysRefVar; // preferred way.
or
dataType arraysRefVar[]; // works but not preferred way.
Note – The dataType[] arraysRefVar style is better. The dataType arraysRefVar[] style comes from the C/C++ programming language, and it was added to Java to help C/C++ programmers.
Example in Declaring Array Variables
Examples of this in Declaration Of Arrays In Java are shown below.
// sample created by Glenn
double[] myLists; // preferred way.
or
double myLists[]; // works but not preferred way.
Creating Arrays In Java
The following syntax allows you to use the new operator to create an array.
The Syntax for Creating Arrays in Java
// syntax created by Glenn
arraysRefVar = new dataType[arraySize];
The above statement does two things.
- It creates an array by using new dataType[arraySize].
- It provides the arraysRefVar variable with the reference to the array that was just made.
Declaring an array variable, making an array, and assigning the array’s reference to the variable can all be done in one statement, as shown below.
dataType[] arraysRefVar = new dataType[arraySize];
You can also make arrays by doing the following:
dataType[] arraysRefVar = {nameOne, nameTwo, …, namek};
The index is used to get to the array elements. Array indices start at 0, so they go up to arraysRefVar.length-1.
Example:
The next statement creates a 10-element array of the type double and gives its reference to the array variable myList.
// sample created by Glenn
double[] myLists = new double[10];
The array of myLists is shown in the picture below. Here, myLists has ten double values, and the indices range from 0 to 9.
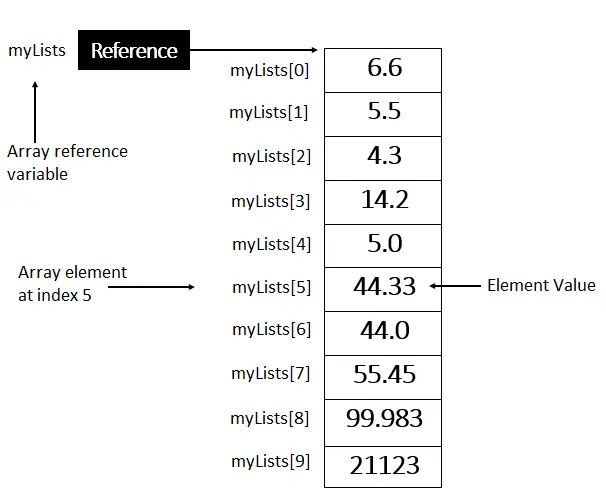
Processing Arrays In Java
We usually use either the for loop or the foreach loop to process array elements because all of the elements in an array are the same type and the size of the array is known.
Processing Arrays Example Program In Java
Here is a complete example of how to create, initialize, and process arrays.
// program by Glenn Magada Azuelo public class TestArray { public static void main(String[] args) { double[] myList = {3.10, 3.9, 5.4, 3.7}; // Print all the array elements for (int i = 0; i < myList.length; i++) { System.out.println(myList[i] + " "); } // Summing all elements double total = 0; for (int i = 0; i < myList.length; i++) { total += myList[i]; } System.out.println("Total is " + total); // Finding the largest element double max = myList[0]; for (int i = 1; i < myList.length; i++) { if (myList[i] > max) max = myList[i]; } System.out.println("Max is " + max); } }
Output:
3.1
3.9
5.4
3.7
Total is 16.1
Max is 5.4
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
You can test the above example here! ➡Java Online Compiler
For Each Loop in Java
JDK 1.5 added a new for loop called the “foreach loop” or “enhanced for loop.” With this loop, you can move through an array in order without using an index variable.
For Each Loop Example Program in Java
The following code shows all the elements in the array myLists.
// program by Glenn Magada Azuelo public class TestArray { public static void main(String[] args) { double[] myList = {3.10, 3.9, 5.4, 3.7}; // Print all the array elements for (double element: myList) { System.out.println(element); } } }
Output:
3.1
3.9
5.4
3.7
You can test the above example here! ➡Java Online Compiler
Passing Arrays To Methods In Java
You can pass arrays to methods just as you can pass primitive type values.
For example, the following method shows the elements in an int array:
Passing Arrays To Methods Example In Java
// created by Glenn Magada Azuelo public static void printArray(int[] array) { for (int i = 0; i < array.length; i++) { System.out.print(array[i] + " "); } }
An array can be used to call it. For example, the following statement uses the printArray method to display 4, 2, 3, 7, 5, and 3.
Example:
printArray(new int[]{4, 2, 3, 7, 5, 3});
Returning an Array to a Method in Java
An array can also be returned by a method. The following method, for example, gives back an array that is the opposite of another array.
Returning an Array to a Method Example In Java
// sample created by Glenn Magada Azuelo public static int[] reverse(int[] list) { int[] result = new int[list.length]; for (int i = 0, j = result.length - 1; i < list.length; i++, j--) { result[j] = list[i]; } return result; }
Arrays Class In Java
The java.util.Arrays class has many static methods that can be used to sort and search arrays, compare arrays, and fill array elements.
All primitive types have more than one way to use these methods.
# | Method & Description |
---|---|
1 | public static int binarySearch(Object[] a, Object key) Searches the specified array of Object ( Byte, Int , double, etc.) for the specified value using the binary search algorithm. The array must be sorted prior to making this call. This returns index of the search key, if it is contained in the list; otherwise, it returns ( – (insertion point + 1)). |
2 | public static boolean equals(long[] a, long[] a2) Returns true if the two specified arrays of longs are equal to one another. Two arrays are considered equal if both arrays contain the same number of elements, and all corresponding pairs of elements in the two arrays are equal. This returns true if the two arrays are equal. Same method could be used by all other primitive data types (Byte, short, Int, etc.) |
3 | public static void fill(int[] a, int val) Assigns the specified int value to each element of the specified array of ints. The same method could be used by all other primitive data types (Byte, short, Int, etc.) |
4 | public static void sort(Object[] a) Sorts the specified array of objects into an ascending order, according to the natural ordering of its elements. The same method could be used by all other primitive data types ( Byte, short, Int, etc.) |
Summary
In summary, you have learned about how to declare an array in Java. This tutorial has covered the following topics: What Are Arrays In Java, Java Arrays Method, Types Of Array In Java, Creating Arrays In Java, For Each Loop In Java, Passing Arrays To Methods In Java, and Arrays Class In Java.
What’s Next
We’ll talk about the Date and Time in the next section. You will learn how to use Date & Time and how this helps you a lot when building Java projects.
< PREVIOUS
NEXT >