How do I create packages?
To make a package, you give it a name and put a package statement with that name at the top of each source file.
That contains the classes, interfaces, enumerations, and annotation types you want to include in the package.
What does a package keyword do in Java?
A package is a keyword in Java. It gives the Java class a “name space.”
It needs to be at the top of the Java file, on the first line of the Java statement.
Most of the time, the company URL is used starting in the back word to make sure that each vendor has a unique package name.
Syntax:
package package;
Example:
package com.pies.myapplication.mymodule;
Is ‘import’ a keyword in Java?
Import is a keyword in Java. It tells the code that comes after it how to use a Java class.
How to Import self created package in Java?
To import a Java package into a class, we use the Java import keyword, which is used to access the package and its classes in a Java program.
You can import built-in and user-defined packages into your Java source file so that a class in one package can directly refer to a class in another package by its name.
Why do I need to import in Java programming language?
With the help of a single statement, an import statement in Java can take a class or all classes that are visible to a program under a package.
It’s helpful because the programmer doesn’t have to write out the entire class definition.
Therefore, it makes the program easier to read.
Which of the following is not a keyword in Java?
true, false, and null are not keywords. Instead, they are literal and reserved words that can’t be used as identifiers.
How many keywords are in Java?
In the programming language Java, a keyword is any of 67 words that are set aside and already have a meaning.
This means that programmers can’t use keywords as names for variables, methods, classes, or any other kind of identifier.
How is the package created in Java?
For each data type source file system that contains the kinds (classes, interfaces, enumerations, and annotation types) that you want to include in the package, you must add a package statement with that name at the top.
How do I create and access a package?
The first statement in the Java source file should contain a package command along with a name for the package.
Classes, interfaces, enumerations, and annotation types can all be found in the Java source file if you choose to include them in the package.
The sentence that follows, for instance, creates a package called MyPackage.
What is a package statement in Java?
The first line of the source file should be the package statement.
Each source file can only have one package statement, which applies to all types in the file.
If you don’t use a package statement, the class, interface, enumeration, and annotation types will go into the default package.
What is the Java util package?
The Java util package has the collections framework, legacy collection classes, event model, date and time facilities, internationalization, and other useful classes.
What is Java’s default package?
By default, the Java compiler imports the java.lang package internally. It gives you the basic classes you need to make a simple Java program.
What is the use of the super keyword?
The super keyword is used to talk about objects’ superclasses or parents. It is used to call superclass methods and to get to the superclass’s constructor.
The most common use of the super keyword is to avoid confusion between superclasses and subclasses whose methods have the same name.
Access Protection in Java Packages
- Access to the public members is everywhere.
- Only members of the same class are able to access private members.
- Every child class can access the protected members (same package or other packages).
- The default members can be accessed from within the same package, but not from outside.
How to Import Packages?
We can bring in a certain class using an importing statement.
To import a certain class, use the following syntax.
Syntax:
import packageName.ClassName;
Let’s look at an example of an import statement that uses a built-in package and the Scanner class.
package myPackage;
import java.util.Scanner;
public class ImportingExample {
public static void main(String[] args) {
Scanner read = new Scanner(System.in);
int x = read.nextInt();
System.out.println("You have entered a number " + x);
}
}
What is Package in Java?
In Java, a package is used to group classes that are similar.
Think of it like a folder in a list of files. We use packages to avoid name conflicts and make code that is easier to maintain.
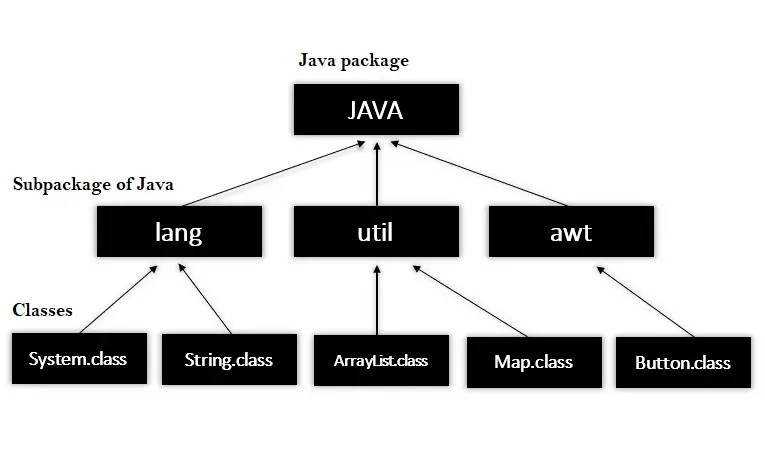
Built-in packages
In Java, packages are used to keep classes, interfaces, subclasses, etc. from having the same name and to control who can use them.
A package is a group of classes, sub-classes, interfaces, enumerations, etc. that are all similar.
When using packages, it’s easier to find related classes, and packages give a project with a lot of classes and files a good structure or outline.
Subpackage in Java
A subpackage is a package inside another package.
The packages that are lower in the naming hierarchy are called “sub packages” of the packages that are higher in the hierarchy.
For example, the package that we are putting into another package is called a “sub package.”
How to run Java package program?
Let’s look at a code example that makes a package called Cars.
To avoid confusion with the names of classes and interfaces, it is best to use lowercase letters when naming packages.
Example
/* File name : Car.java */
package Cars;
interface Car {
public void break();
public void travel();
}
Now, let’s use the above interface in the Cars package.
package Cars; /* File name : BMW.java */ public class BMW implements Animal { public void break() { System.out.println("Stop the car"); } public void travel() { System.out.println("BMW travels"); } public int noOfWheels() { return 0; } public static void main(String args[]) { BMW m = new BMW(); m.break(); m.travel(); } }
Output:
Stop the car BMW travels
How to access a package from another package?
The package can be accessed in 3 different ways from outside the package.
- import fully qualified name
- imports the packagename. *
- import package.classname
Example of the package by import fully qualified name
//save by mj.java
package pack;
public class mj{
public void msg(){
System.out.println("Welcome Glenn Magada Azuelo");
}
}
//save by gl.java
package mypack;
class gl{
public static void main(String args[]){
pack.mj name = new pack.mj();//using fully qualified name
name.msg();
}
}
Output:
Welcome Glenn Magada Azuelo
Example of package that imports the packagename. *
//save by mj.java
package pack;
public class mj {
public void msg()
{
System.out.println("Welcome Glenn Magada Azuelo");
}
}
//save by gl.java
package mypack;
import pack.*;
class gl
{
public static void main(String args[])
{
mj obj = new mj();
obj.msg();
}
}
Output:
Welcome Glenn Magada Azuelo
Example of package by import package.classname
//save by A.java
package pack;
public class mj{
public void msg(){
System.out.println("Welcome Glenn Magada Azuelo");
}
}
//save by gl.java
package mypack;
import pack.mj;
class gl{
public static void main(String args[]){
mj obj = new mj();
obj.msg();
}
}
Output:
Welcome Glenn Magada Azuelo
Packages defined by users
User-defined packages are packages that a developer creates or designs to organize classes and packages.
They are very similar to what comes with Java. It can be imported into other classes and used like built-in packages.
Advantages of Java Package
Using Java Packages has many advantages, some of which are listed below.
- Make it easy to find classes and interfaces or search for them.
- Avoid naming problems. For example, there can be two classes with the name Student, one in the university.csdept.Student package and the other in the college.itdept.Student package.
- Implement data encapsulation (or data-hiding).
- Provide controlled access. Access control is built into the access specifiers protected and default. When a member is marked as protected, classes in the same package and subclasses of that class can access it. Classes in the same package can only access a member that doesn’t have an access specifier but has a default specifier.
- Use the classes that are in other programs’ packages.
- Compare classes from different packages in a unique way.
Types of packages in Java
They are classified into 2 types:
- User-defined packages
- Java API packages or built-in packages
A simple example of a Java package
//save as mySimple.java
package mypack;
public class mySimple{
public static void main(String args[]){
System.out.println("Welcome to Pies package");
}
}
Conclusion
In conclusion, packages in Java are like folders that help organize and manage similar classes.
They prevent naming conflicts and make code easier to maintain.
It gives the Java class a “name space”, and the import keyword allows you to use classes from other packages.
Access protection ensures controlled access to package members.
Using packages has advantages like easy class finding, avoiding naming issues, and implementing data encapsulation. There are two types of packages: user-defined and built-in.
Overall, packages are essential for well-organized and readable Java code.
< PREVIOUS