What is Polymorphism in Java?
A Polymorphism In Java, it means “many forms,” and it happens when we have a lot of classes that inherit from each other.
As we said in the last chapter, inheritance takes the properties and methods of one class and uses them in another.
Polymorphism makes use of these ways to do different things. This means that we can do the same thing in different ways.
Polymorphism is inherently a good thing. It means that something has more than one form. It can refer to both objects and methods.
Polymorphism lets you code to an interface, which reduces coupling, makes code more reusable, and makes it easier to read.
For example, think of a superclass named Animal that has a method called animalSound(). Pigs, Cats, Goats, and Birds could all be subclasses of Animals.
Each subclass also has its own way of making an animal sound (the pig oinks, the cat meows, etc.):
Example:
// program created by Glenn
class Animal {
public void animalSound() {
System.out.println("The animal makes a sound");
}
}
class Goat extends Animal {
public void animalSound() {
System.out.println("The Goat says: mee mee");
}
}
class Cat extends Animal {
public void animalSound() {
System.out.println("The Cat says: meow meow");
}
}
We can now make Goat and Cat objects and call their animalSound() methods:
Example:
// program created by Glenn
class Animal {
public void animalSound() {
System.out.println("The animal makes a sound");
}
}
class Goat extends Animal {
public void animalSound() {
System.out.println("The Goat says: mee mee");
}
}
class Cat extends Animal {
public void animalSound() {
System.out.println("The Cat says: meow meow");
}
}
public class Main {
public static void main(String[] args) {
Animal myAnimal = new Animal(); // Create a Animal object
Animal myGoat = new Goat(); // Create a Goat object
Animal myCat = new Cat(); // Create a Pig object
myAnimal.animalSound();
myGoat.animalSound();
myCat.animalSound();
}
}
Output:
The animal makes a sound The Goat says: mee mee The Cat says: meow meow
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
What is Polymorphism in Java with examples?
A Polymorphism In Java is one of OOP’s features that lets us do the same action differently.
For example, let’s say we have a class Animal that has a method named sound().
Since this is a generic class, we can’t give it a specific implementation like Roar, Meow, Oink, etc.
We had to give a general message.
Real-life Examples of Polymorphism
Different people can have different kinds of relationships with the same person.
A woman can be a mother, a daughter, a sister, and a friend all at the same time.
This means that in different situations, she acts in different ways.
There are different organs in the body. Every organ in the body has a different job to do.
The heart pumps blood, the lungs breathe, the brain thinks, and the kidneys get rid of waste.
So, we have a standard method function that does different things for each organ.
Types Of Polymorphism In Java
There are two kinds of Polymorphism In Java listed below.
- polymorphism at compile time
- polymorphism at run time
This Java Polymorphism is also called a static polymorphism and a dynamic polymorphism.
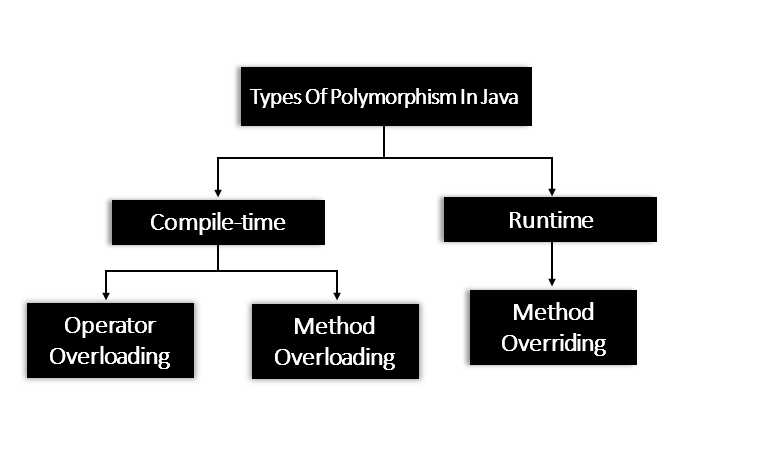
Run-time Polymorphism In Java
The Runtime Polymorphism In Java is when an object’s functionality is tied to it at run time.
It is also known as late binding or dynamic binding. Method overriding means that the same method is declared in both the parent class and the child class.
It means that if a child class provides a different way to do something that one of its parents already did, this is called “method overriding.”
Compile-time Polymorphism in Java
A Compile-Time Polymorphism In Java is a polymorphism that is resolved during the compilation process.
Overloading of methods is done through the reference variable of a class.
Polymorphism at compile time is achieved through method overloading and operator overloading.
Where is Polymorphism used in Java?
A Java Polymorphism is the ability of an object to change into different things.
In Object-Oriented Programming, polymorphism is most often used when a parent class reference is used to refer to an object of a child class.
Polymorphic describes Java objects that can pass more than one IS-A test.
Conclusion
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.