What is the Java.io package?
The java.io package In Java has almost every class you could ever need to do I/O (input and output) in Java.
All of these streams have a source and a place where they end up.
The stream in the java.io package can hold many types of data, including primitives, objects, localized data, and characters.
The Java.io Package in Java it gives a set of input streams and a set of output streams that you can use to read and write data to files and other input and output sources.
Why is Java.io used?
The Java I/O (Input and Output) is used to process the input and create the output.
Java uses the idea of a stream to make I/O operations fast.
The java.io package contains all the classes needed for input and output operations.
Java I/O API lets us handle files in Java.
import Java.io.* meaning
Import basically means adding a Library or Package full of ready-made classes and methods.
The standard for Java Input/Output is Java.IO.
So, basically, you’re adding more I/O functionality. With the * at the end, you’re importing all in the Java.IO Package.
What are Java.io classes?
The following is the important list of java.io packages:
- BufferedInputStream
- BufferedOutputStream
- BufferedReader
- BufferedWriter
- ByteArrayInputStream
- ByteArrayOutputStream
- CharArrayReader
- CharArrayWriter
- Console
- DataInputStream
- DataOutputStream
- File
- FileDescriptor
- FileInputStream
- FileOutputStream
- FilePermission
- FileReader and FileWriter
- FilterInputStream
- FilterOutputStream
- FilterReader
- FilterWriter
- InputStream
- InputStreamReader
- LineNumberInputStream
- LineNumberReader
- ObjectInputStream
- ObjectInputStream.GetField
- ObjectOutputStream
- ObjectOutputStream.PutField
- ObjectStreamClass
- ObjectStreamField
- OutputStream
- OutputStreamWriter
- PipedInputStream
- PipedOutputStream
- PipedReader
- PipedWriter
- PrintStream
- PrintWriter
- PushbackInputStream
- PushbackReader
- RandomAccessFile
- Reader
- SequenceInputStream
- SerializablePermission
- StreamTokenizer
- StringBufferInputStream
- StringReader
- StringWriter
- Writer
- ZipInputStream class in Java
- ZipEntry class in Java
- JarEntry class in Java
- ZipOutputStream class in Java
- Zip.InflaterInputStream class in Java
- Zip.DeflaterInputStream class in Java
- Zip.DeflaterOutputStream class in Java
What is the import java.io file?
The import java.io.* entails adding all the classes and interfaces that the io package has already specified.
It is advised that you import just the specific class or interface you require rather than the full package if you want to use that package.
Is java.io an API
The Java io is an API that is designed for reading and writing data (input and output).
Do we need to import the java.io package?
No, the java. lang package is already included in Java, so you don’t need to import it.
Stream in Java
What is Stream Class in Java?
A Stream Class In Java is a list of objects that support different methods that can be chained together to get the desired result.
The features of a Java stream are: A stream is not a data structure; instead, it gets data from Collections, Arrays, or I/O channels.
The following listed below are the two kinds of Stream in Java.
- InPutStream – reads data from a source.
- OutPutStream – writes data to a destination.
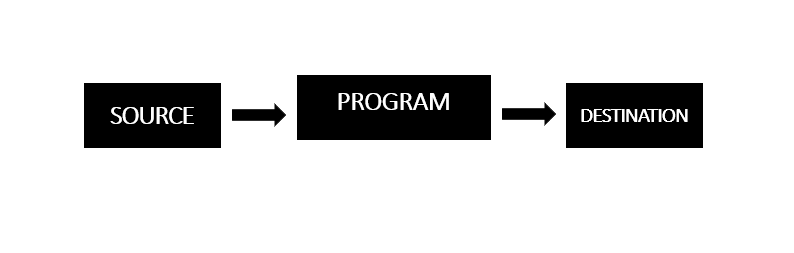
Java has strong but flexible I/O support for files and networks, but this tutorial only covers very basic functions related to streams and I/O. We’ll look at the most common examples one by one.
Byte Stream in Java
What is Byte Stream in Java?
A Byte stream In Java is used to send and receive bytes with 8 bits.
They are used to read bytes from the input stream and send bytes to the output stream.
They are mostly used to read and write binary data.
Even though there are many classes that deal with byte streams, FileInputStream and FileOutputStream are the ones that are used the most.
The following is an example of how to use these two classes to copy an input file into an output file.
Example:
// program created by Glenn
import java.io.*;
public class CopyFile {
public static void main(String args[]) throws IOException {
FileInputStream in = null;
FileOutputStream out = null;
try {
in = new FileInputStream("input.txt");
out = new FileOutputStream("output.txt");
int c;
while ((c = in.read()) != -1) {
out.write(c);
}
}finally {
if (in != null) {
in.close();
}
if (out != null) {
out.close();
}
}
}
}
Now, let’s make a file called input.txt that contains the following:
This is example test for copy file.
The next step is to compile and run the above program. This will create an output.txt file with the same content as the input.txt file.
So let’s put the above code in the CopyFile.java file and do the following:
// sample created by Glenn
$javac CopyFile.java
$java CopyFile
Character Stream in Java
What is Character Stream in Java?
Java Byte streams are used for the input and output of 8-bit bytes, while Character Stream In Java is used for the input and output of 16-bit unicode.
Even though there are many classes that deal with character streams, the most common ones are FileReader and FileWriter.
Even though FileReader and FileWriter both use FileInputStream and FileOutputStream internally, the main difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
We can change the above example to use these two classes to copy a file with unicode characters from an input file to an output file.
Example:
// program created by Glenn
import java.io.*;
public class CopyFile {
public static void main(String args[]) throws IOException {
FileReader in = null;
FileWriter out = null;
try {
in = new FileReader("input.txt");
out = new FileWriter("output.txt");
int c;
while ((c = in.read()) != -1) {
out.write(c);
}
}finally {
if (in != null) {
in.close();
}
if (out != null) {
out.close();
}
}
}
}
Now, let’s make a file called input.txt that contains the following:
This is example test for copy file.
As the next step, compile and run the above program. This will create an output.txt file with the same content as input.txt.
So let’s put the above code in the CopyFile.java file and then do the following:
// program created by Glenn
$javac CopyFile.java
$java CopyFile
Standard Stream in Java
What is Standard Stream in Java?
The Standard Stream In Javas is an idea from the C library that has been incorporated into the Java language.
Simply put, a stream is a flow of characters. The standard input stream is a stream that reads characters from the keyboard.
Standard I/O is supported by all programming languages.
This means that a user’s program can take input from the keyboard and then show something on the computer screen.
If you know how to write code in C or C++, you should know about three standard devices: STDIN, STDOUT, and STDERR.
The following listed below are the 3 standard streams in Java:
- Standard Input – This is where the information that the user’s program needs is found. System.in stands for the standard input stream, which is usually a keyboard.
- Standard Output – This is where the data created by the user’s program goes. The standard output stream, which is represented by System.out, is usually the computer screen.
- Standard Error – This is used to show the error data made by the user’s program. It is usually shown on the computer screen and is called System. err.
The following is a simple program that makes an InputStreamReader that reads the standard input stream until the user types “q.”
Example:
// program created by Glenn
import java.io.*;
public class ReadConsole {
public static void main(String args[]) throws IOException {
InputStreamReader cin = null;
try {
cin = new InputStreamReader(System.in);
System.out.println("Enter characters, 'q' to quit.");
char c;
do {
c = (char) cin.read();
System.out.print(c);
} while(c != 'q');
}finally {
if (cin != null) {
cin.close();
}
}
}
}
Let’s keep the above code in the ReadConsole.java file and try to compile and run it as shown in the following program.
This program will keep reading and printing the same character until we press ‘q’
// sample created by Glenn
$javac ReadConsole.java
$java ReadConsole
Enter characters, ‘q’ to quit.
1
1
e
e
q
q
Reading and Writing Files in Java
As we’ve already talked about, a stream is a series of data.
The InputStream is used to read data from a source, and the OutputStream is used to write data to a destination.
The following is a hierarchy of classes to deal with input and output streams:
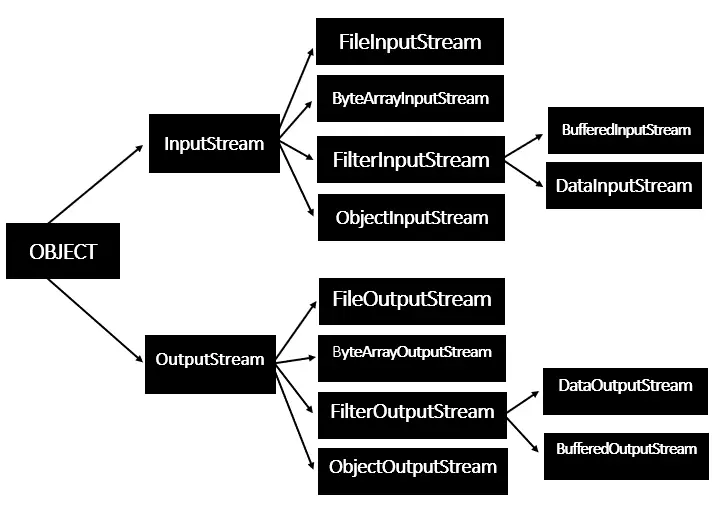
The two most important streams In Java are FileInputStream and FileOutputStream, which will be discussed below.
- FileInputStream
- FileOutputStream
FileInputStream in Java
What is FileInputStream in Java?
A FileInputStream In Java is used to read the data from the files.
Objects can be made using the keyword new, and there are different types of constructors that can be used.
The following constructor takes a file name as a string and creates an input stream object to read the file.
InputStream f = new FileInputStream(“C:/java/Glenn Magada Azuelo”);
The following constructor takes a file object and makes an input stream object to read the file.
First, we use the File() method to make a file object.
File fi = new File(“C:/java/Glenn Magada Azuelo”);
InputStream fi = new FileInputStream(fi);
The following listed below are helper methods that can be used to read the Input Streams In Java or to do other operations on the streams.
# | Method & Description |
---|---|
1 | public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | public int read(int r)throws IOException{} This method reads the specified byte of data from the InputStream. Returns an int. Returns the next byte of data and -1 will be returned if it’s the end of the file. |
4 | public int read(byte[] r) throws IOException{} This method reads r.length bytes from the input stream into an array. Returns the total number of bytes read. If it is the end of the file, -1 will be returned. |
5 | public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
FileOutputStream in Java
What is FileInputStream in Java?
A FileOutputStream In Java is used to create a file and write data to it.
If there wasn’t already a file, the stream would make one before opening it for output.
The following constructor takes a file name as a string to make an input stream object that can be used to write the file.
OutputStream f = new FileOutputStream(“C:/java/Glenn Magada Azuelo”)
The following example below is a constructor that takes a file object and creates an output stream object to write the file.
First, we use the File() method to make a file object.
File f = new File(“C:/java/Glenn Magada Azuelo”);
OutputStream f = new FileOutputStream(f);
The following listed below are helper methods that can be used to read the Output Streams In Java or to do other operations on the streams.
# | Method & Description |
---|---|
1 | public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | public void write(int w)throws IOException{} This methods writes the specified byte to the output stream. |
4 | public void write(byte[] w) Writes w.length bytes from the mentioned byte array to the OutputStream. |
The following is the example below to demonstrate InputStream and OutputStream.
Example:
// program created by Glenn
import java.io.*;
public class fileStreamTest {
public static void main(String args[]) {
try {
byte bWrite [] = {11,21,3,40,5};
OutputStream os = new FileOutputStream("test.txt");
for(int x = 0; x < bWrite.length ; x++) {
os.write( bWrite[x] ); // writes the bytes
}
os.close();
InputStream is = new FileInputStream("test.txt");
int size = is.available();
for(int i = 0; i < size; i++) {
System.out.print((char)is.read() + " ");
}
is.close();
} catch (IOException e) {
System.out.print("Exception");
}
}
}
The code above would make the file test.txt and write the numbers given in binary format.
The output on the stdout screen would be the same.
Directories in Java
A Directory In Java is a file that can contain a list of other files and directories.
You can use the File object to create directories and make a list of the files that are in a directory.
For full information, look at a list of all the methods you can call on a File object and what they have to do with directories.
Creating Directories
The following listed below are two useful File utility methods, which can be used to create directories.
- mkdir() – This method makes a directory and returns true if it works and false if it doesn’t. Failure means that the path given in the File object already exists, or that the directory can’t be made because the entire path doesn’t exist yet.
- mkdirs() – method creates a directory and all of its parent directories.
The following example below creates “/tmp/user/java/bin” directory
Example:
// program created by Glenn
import java.io.File;
public class CreateDir {
public static void main(String args[]) {
String dirname = "/tmp/user/java/bin";
File d = new File(dirname);
// Create directory now.
d.mkdirs();
}
}
Compile and run the above code to create “/tmp/user/java/bin“.
Note – Java takes care of path separators automatically on both UNIX and Windows, following conventions.
If you use a forward slash (/) on a Windows version of Java, the path will still be resolved correctly.
Listing Directories in Java
You can list all the files and directories in a directory using the File object’s list() method as follows:
Example:
// program created by Glenn
import java.io.File;
public class ReadDir {
public static void main(String[] args) {
File file = null;
String[] paths;
try {
// create new file object
file = new File("/tmp");
// array of files and directory
paths = file.list();
// for each name in the path array
for(String path:paths) {
// prints filename and directory name
System.out.println(path);
}
} catch (Exception e) {
// if any error occurs
e.printStackTrace();
}
}
}
Output:
hsperfdata_root
jdoodle
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
Conclusion
In conclusion, the java.io package in Java is a comprehensive library that provides classes for input and output operations.
It includes various types of streams capable of handling different data, such as primitives, objects, localized data, and characters.
The Java I/O API allows developers to read and write data to files and other input/output sources efficiently.
What’s Next
The next section talks about Exceptions In Java. At the end of the session, you’ll know what Exceptions are all about in Java.
< PREVIOUS
NEXT >