Most programming languages support references, but C and C++ also support pointers.
C++ is interesting because it supports both pointers and references.
A pointer in C++ is a variable that stores the memory address and size on the stack.
While a reference is a different name for an existing variable.
Once a reference has been set to a variable, it can’t be changed to refer to another variable.
So, a reference is similar to a const pointer.
Pointers Variables
Pointers are variables that hold the memory address of another variable and require space on the stack.
To access the memory location it points to, the pointer needs to be dereferenced with the * operator.
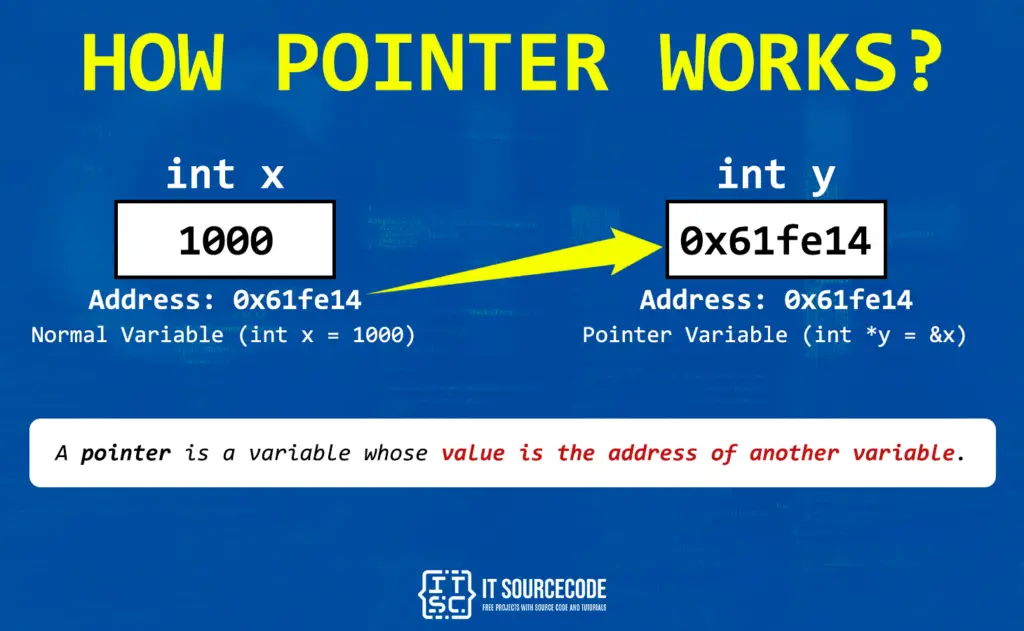
Syntax:
datatype *variable_name;
Example:
#include<iostream>
using namespace std;
int main()
{
int x = 1000;
int *y= &x;
cout << x << endl;
cout << &x << endl;
cout << y << endl;
}
The example above shows how a pointer can be used to store the address of another variable.
In this case, the pointer y stores the address of the x variable.
By using the pointer y and the reference &x, we can print out the memory address of the variable x.
References Variables
A reference variable is an alias or a different name for an existing variable.
A reference is accomplished by saving the address of an object, similar to a pointer.
Reference can be created by simply using an ampersand &
operator.
It is like a constant pointer, except the compiler automatically applies the *
operator when needed.
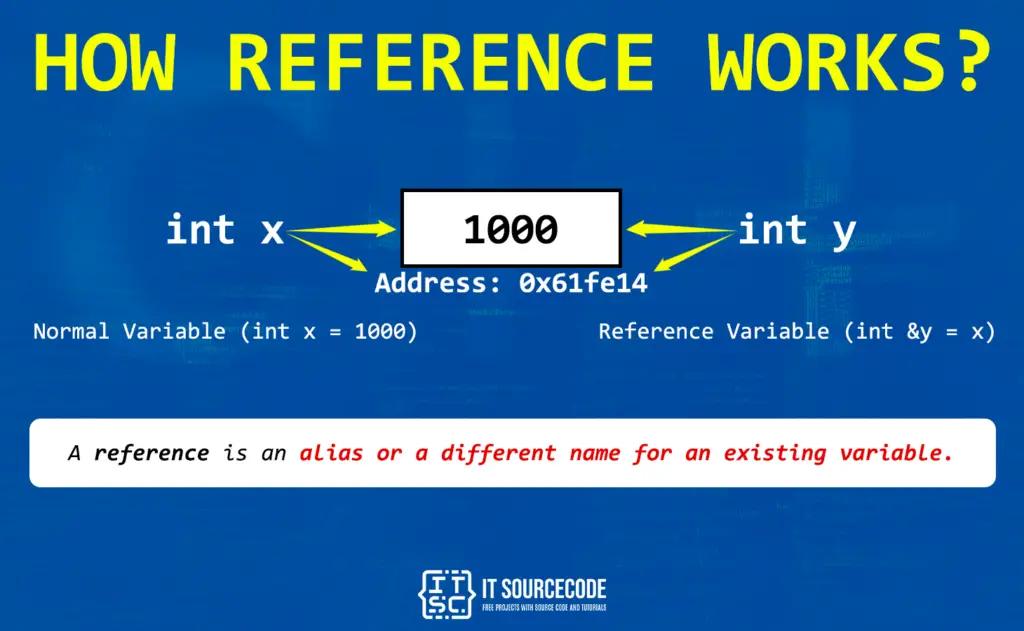
Syntax:
Type &newname = existing variable_name;
Example:
#include<iostream>
using namespace std;
int main()
{
int x = 1000;
int &y = x;
cout << y;
}
The example above shows that the variable y
is the reference of the int x
.
And there is no need to use the *
to dereference a reference variable.
What is the Difference Between Pointers and References in C++
- References are used to create an alias for an existing variable, while pointers store the address of a variable.
- A null value can’t be given to a reference, but it can be given to a pointer.
- A reference variable can be referenced using a pass-by value, whereas a pointer can only be referenced via a pass-by reference.
- It is not necessary in the case of the pointer that references must be initialized on a declaration.
- A reference, on the other hand, utilizes the same memory location as the original variable but requires additional stack space.
int x = 10;
// A pointer to variable x (or stores address of x)
int *ptr = &x;
// A reference (or alias) for x.
int &ref = x;
Difference Between C++ Pointers and References
Initialization
Here are some ways to set up a pointer:
int x = 10;
int *p = &x;
// You can also try the code below
int *p;
p = &x;
// We can declare and initialize pointer at same step or in multiple line.
While in references,
int x=10;
int &p = x; // it is correct
// The code below is not the correct way to declare reference.
int &p;
p=x;
// it is incorrect as we should declare and initialize references at single step or line.
NOTE: These differences may be different between compilers.
Reassignment
A pointer can be reassigned to point to different data. This is useful for implementing data structures like linked lists and trees. For example:
int x = 10;
int y = 7;
int *p;
p = &x;
p = &y;
On the other hand, a reference can’t be reassigned; it must be assigned at initialization.
int x = 10;
int y = 7;
int &p = x;
int &p = y; // At this line it will show error as "multiple declaration is not allowed".
// However if you comment the invalid line, the code below is valid statement.
int &q = p;
Memory Address
A pointer has its memory address, whereas a reference shares the same memory address with the original variable.
NOTE: If we want a real address to use as a reference, we should write the following:
int x = 10;
int &p = x;
cout << &p;
NULL Value
Pointers can be set to NULL
, but references cannot.
This is because references come with certain constraints (no NULL, no reassignment) that prevent them from causing exceptions.
Indirection
You can have pointers to pointers, which offer more than one level of indirection.
References, on the other hand, only offer one level of indirection.
int x = 10;
int *p;
int **q; // it is valid.
p = &x;
q = &p;
Whereas in references,
int &p = x;
int &&q = p; // it is not correct to refer a reference, it will prompt an error.
Arithmetic Operations
Arithmetic operations can be performed on pointers but not on references.
(However, pointer arithmetic can be performed on the address of an object pointed to via a reference, as in &obj + 5).
Summary
You should use references when you can and pointers when you have to, according to ISOCPP FAQs.
References are usually preferred over pointers whenever you don’t need “reseating”.
This usually means that references are most useful in a class’s public interface.
References typically appear on the skin of an object and pointers on the inside.
I hope you’ve learned the differences between C++ Pointers and References and how to use them properly.