In this course, we will learn about C++ class and object structures and how to use them using examples.
This programming paradigm is known as object-oriented programming.
But before we can create objects and use them in C++, we first need to learn about classes.
Defining Class and Object
A class is a user-defined type that describes how an object of a certain type will look.
There are two parts to a class description: a declaration and a definition.
On the other hand, objects are instances of Classes.
When a class is defined, no memory is allocated; however, memory is allocated when an object is instantiated.
In C++, everything is connected to classes and objects, as well as their properties and methods.
In real life, a car is an example of an object. The car has things like weight and color, and it can be driven and stopped.
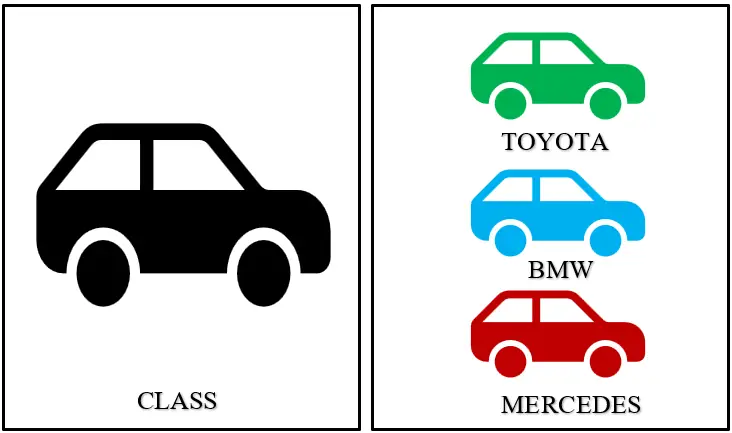
What is Class in C++?
In C++, a class is a foundation for Object-Oriented programming.
It is a user-defined data type with its own data members and member functions that can be accessed and used by making an instance of that class.
- Data members are the data variables, and member functions are the functions used to change these variables. Together, these make up the Class properties and behavior of the objects.
Create a class in C++
In C++, a class
is defined with the keyword class followed by the class name.
The class’s body is defined within the curly brackets and ends with a semicolon.
Syntax to Define Class
Here is the syntax to define a class
:
class MyClass {
};
Example:
class MyClass {
public:
int myNum;
string myString;
double height;
};
Here, we defined a class named MyClass
.
- The
class
keyword is used to create theMyClass
class. - The
public
keyword is an access specifier. - Inside the class, there is an
integer
variablemyNum
, astring
variablemyString
, anddouble
variableheight
. When variables are declared within a class, they are calledattributes
. - Finally, terminate the class declaration with a semicolon
;
.
What is Objects in C++?
Objects are instances of Classes and are used to access all data members and member functions of a class.
Create Objects in C++
In C++, an object is created from a class. We have already created the class name MyClass
, so now we can use this in creating objects.
To create an object of MyClass
, specify the class name, followed by the object name.
Syntax:
className objectVariableName;
Example:
We can create objects of myRoom
class as follows:
void sampleFunction() {
myRoom room1, room2;
}
int main(){
myRoom room3, room4;
}
Here, two objects of the myRoom
class, room1
, and room2
are generated in sampleFunction()
. Similarly, the room3
and room4
objects are generated in the main()
.
We can make objects of a class in any function of the program, as we can see.
We can also make objects of a certain class inside that class or in other classes.
Accessing Data Members and Member Functions in C++
The object’s data members and member functions can be accessed using the dot (‘.
‘) operator.
For instance, if the object’s name is obj
and you wish to call the member function named printName()
, you must write obj.printName()
.
Accessing Data Members
The same method is used to access the public data members; however, the private data members cannot be accessed directly by the object. Access modifiers provide this access restriction in C++.
There are 3 access modifiers : public
, private
, and protected
.
C++ Program to Demonstrate Accessing of Data Members
We have a free C Online Compiler for you to use if you’d rather run the code online.
#include <bits/stdc++.h>
using namespace std;
class Myname
{
public:
string myname;
void printname()
{
cout << " My name is: " << myname;
}
};
int main() {
Myname obj1;
obj1.myname = "May";
obj1.printname();
return 0;
}
In this program, we used the Myname
class. In main()
, declare an object of class Myname
obj1
and access data members.
We then called the functions printname()
to print obj1
.
The keyword
public
used in the program means that the public members are accessible from outside the class.
We can also create private members using the private
keyword.
The private members of a class can only be accessed from within the class.
Output:
Output:
My name is: May
Member Functions in classes
There are two definitions of a member function:
- Inside class definition – treated as inline functions automatically if the function definition doesn’t contain looping statements or complex multiple line operations.
- Outside class definition – To define a member function outside the class definition, we have to use the scope resolution
::
operator along with class name and function name.
C++ Program to Demonstrate Member Function Class
Here’s the example program:
#include <bits/stdc++.h>
using namespace std;
class Name
{
public:
string myname;
int id;
void printname();
void printid()
{
cout << "Name id is: " << id;
}
};
void Name::printname()
{
cout << "My name is: " << myname;
}
int main() {
Name obj1;
obj1.myname = "xyz";
obj1.id=15;
obj1.printname();
cout << endl;
obj1.printid();
return 0;
}
Output:
Output:
My name is: xyz
Name id is: 15
In this program, we used the Name
class. In main()
, declare an object of class Name
obj1
and access data members.
Next, called the functions printname()
and printid()
to execute member function class.
Note that the printname()
is not defined inside the class definition which is why we have to use the scope resolution ::
operator along with class
name and function
name.
While the printid()
is defined inside class definition this means the function definition doesn’t contain looping statements or complex multiple-line operations.
Constructors
Constructors are unique class members invoked by the compiler whenever an object of a particular class is instantiated.
It has the same name as the class and can be defined either within or outside of the class declaration.
3 Types of Constructors
There are three types of constructors:
- Default constructors
- Parameterized constructors
- Copy constructors
C++ Program to Demonstrate Constructors
#include <bits/stdc++.h>
using namespace std;
class Names
{
public:
int id;
Names()
{
cout << "Default Constructor called" << endl;
id=-1;
}
Names(int x)
{
cout << "Parameterized Constructor called" << endl;
id=x;
}
};
int main() {
Names obj1;
cout << "Name id is: " <<obj1.id << endl;
Names obj2(21);
cout << "Name id is: " <<obj2.id << endl;
return 0;
}
Output:
Output:
Default Constructor called
Name id is: -1
Parameterized Constructor called
Name id is: 21
Destructors
Destructor is another special member function that is called by the compiler when the object’s life is over.
C++ Program to Explain Destructors
#include <bits/stdc++.h>
using namespace std;
class Names
{
public:
int id;
~Names()
{
cout << "Destructor called for id: " << id <<endl;
}
};
int main()
{
Names obj1;
obj1.id=7;
int i = 0;
while ( i < 5 )
{
Names obj2;
obj2.id=i;
i++;
}
return 0;
}
Output:
Output:
Destructor called for id: 0
Destructor called for id: 1
Destructor called for id: 2
Destructor called for id: 3
Destructor called for id: 4
Destructor called for id: 7
Conclusion
In summary, the main focus of this session was to learn What is a Class and Object in C++ with Examples and get deeper to access data members and functions as well as example programs and outputs.
That’s it! What is a Class and Object in C++ with Examples is now complete!
This is very important to our understanding of the C++ Programming language Tutorial.