What is Polymorphism in C++ with Example?
The generic definition of the word polymorphism is the occurrence of something in several different forms.
In C++, polymorphism signifies that the same entity (function or object) behaves differently depending on the situation.
Polymorphism is the Greek term for having multiple forms. It arises when multiple classes are related by inheritance.
For example, suppose we have the Noise()
function. When a vehicle invokes this function, the engine noise will be produced.
A barking sound will be produced whenever a dog makes a call to the same function.
Although we have a single function, it responds differently depending on the situation.
We have accomplished polymorphism as the function has multiple variants.
Another example is that the "+"
operator in C++ can do two different things depending on the situation.
When the "+"
operator is used with numbers, it adds them together.
int x = 50;
int y = 100;
int sum = x + y; // sum =150
When the same "+"
operator is used in a string, it performs concatenation.
string word1 = "C++ ";
string word2 = "Polymorphism";
string name = word1 + word2; // name = "C++ Polymorphism"
What are Two Types of Polymorphism in C++?
In C++, there are two main types of polymorphism:
- Compile-time Polymorphism
- Runtime Polymorphism
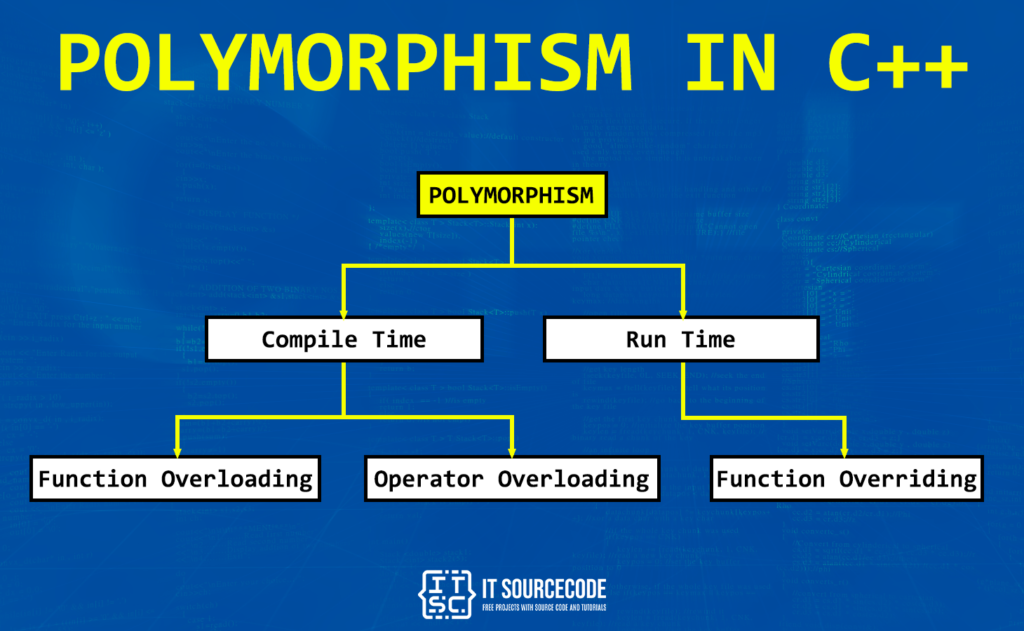
Compile-time Polymorphism
This type of polymorphism is made possible by function overloading or operator overloading.
Function Overloading
C++ allows for two functions with the same name as long as their arguments are different.
The function that is called depends on the number and type of arguments.
For Example:
#include <iostream>
using namespace std;
int mul(int x, int y) {
return x * y;
}
double mul(double x, double y) {
return x * y;
}
int mul(int x, int y, int z) {
return x * y * z;
}
int main() {
cout << "The multiplication of 2 integers: " << mul(6, 4) << endl;
cout << "The multiplication of 2 double numbers: " << mul(10.6, 22.9) << endl;
cout << "The multiplication of 3 integers: " << mul(51, 2, 55) << endl;
return 0;
}
Output:
The multiplication of 2 integers: 24
The multiplication of 2 double numbers: 242.74
The multiplication of 3 integers: 5610
Here, we’ve made three different mul()
functions, each with a different number and type of parameters.
And a certain mul()
is called based on the arguments passed to a function call.
It’s a compile-time polymorphism because the compiler already knows which function to run before the program is compiled.
Operator Overloading
We can overload operators in C++ if we are working with user-defined types like objects and structures.
We cannot overload operators on fundamental types such as int
, double
, etc.
Operator overloading is essentially a form of function overloading in which many operator functions use the same symbol but have distinct operands.
Different operator functions are executed based on the operands.
Example:
#include <iostream>
using namespace std;
class Count {
private:
int cvalue;
public:
Count() : cvalue(29) {}
void operator ++() {
cvalue = cvalue + 1;
}
void display() {
cout << "The count: " << cvalue << endl;
}
};
int main() {
Count i;
++i;
i.display();
return 0;
}
Output:
The count: 30
Here, the ++
operator, which operates on Count
class objects have been overloaded (object i
in this case).
The value variable of the object i
has been directly incremented by 1 using an overloaded operator.
This is an example of compile-time polymorphism.
Runtime Polymorphism
The function overriding makes it feasible for this kind of polymorphism to exist.
Function Overriding
When the function is invoked using an object of the derived class, the function of the derived class is executed rather than the function of the base class.
So, distinct functions are executed based on the object that invokes the function. C++ refers to this as function overriding.
Example:
#include <iostream>
using namespace std;
class Base {
public:
virtual void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived x;
x.print();
return 0;
}
Output:
Derived Function
Here, we have used the print()
method in the Base
class and the same method in the Derived
class.
When print()
is called on the Derived object x
, it executes the print()
function of the Derived
class instead of the print()
function of the Base
class.
It is a runtime polymorphism since the function call is not resolved by the compiler but rather at runtime.
Compile-Time Polymorphism Vs. Run-Time Polymorphism
The following are the major differences between the two:
Compile-Time Polymorphism | Run-Time Polymorphism |
---|---|
It’s also known as early binding or static polymorphism. | It’s also known as late binding, dynamic binding, or dynamic polymorphism. |
The method is called during the compilation process. | During run time, the method is called. |
Implemented using operator overloading and function overloading | Method overriding and virtual functions were used to make it happen. |
The code runs faster because the methods are found when the code is compiled. | Method discoverer is done during runtime, which slows down the execution. |
Since everything is known at compile time, there is less room for solving problems. | Since methods are found during runtime, there is a lot of flexibility for solving complex problems. |
What is Polymorphism in OOPs?
Polymorphism, which means having more than one form, is another important OOP concept.
Polymorphism lets you use a single interface, like a data type or a class, with different forms underneath, like different data types.
For example, we can have methods with the same name in more than one class or class that is a child of it.
Polymorphism is the ability to take on many different forms. It is the key concept in object-oriented programming.
This enables us to write easily extensible applications.
Why is Polymorphism Useful C++?
Polymorphism is when a hierarchy of classes is related by inheritance, and a member function is executed differently depending on the type of object invoking the function.
Polymorphism means that an object or method can be used in many different ways.
Polymorphism lets you code to an interface that makes your code easier to read, reduces coupling, and makes it easier to use more than once.
Why is Polymorphism Used?
One of the most important parts of Object-Oriented Programming is polymorphism.
With polymorphism, we can do the same thing in different ways.
In other words, polymorphism lets you define just one interface and have different ways of implementing it.
Where is Polymorphism Used?
Polymorphism is when something can change into different things.
In OOP, polymorphism is most often used when a parent class reference is used to point to an object of a child class.
What are the Benefits of Polymorphism?
Polymorphism allows us to reuse code by creating one function or operator that can be used for multiple purposes.
This saves time and makes for a more streamlined program.
Polymorphism also helps the programmer reuse the codes, which means that classes can be used again and again after they have been written, tested, and put into place.
A lot of time is saved. More than one type of data can be stored in a single variable. The codes are easy to fix.
What are the Advantages and Disadvantages of Polymorphism?
We can achieve flexibility in our code by using methods with the same names for different operations, depending on our requirements.
The main benefit of using this approach, called polymorphism, is that we can provide an implementation for an abstract base class or interface.
One downside of polymorphism is that it can be tough for developers to implement in code.
Additionally, runtime polymorphism can cause performance issues, as the machine has to decide which method or variable to invoke at runtime, which can slow things down.
Summary
In this lesson, we discussed what polymorphism is, the two different types of polymorphism, and their respective types.
We also discussed the advantages and disadvantages of polymorphism.
I hope you learned how to use numbers in C++ properly.
If you missed any of our previous lessons, check out our list of C++ Programming Tutorials for Beginners.
Inquiries
If you have any questions or suggestions regarding C++ Polymorphism, please do not hesitate to contact me.
Simply leave a comment on this page or send me a message using the contact page of our website.