C++ allows us to set the memory for a variable or an array while the program runs and it’s called C++ dynamic memory allocation.
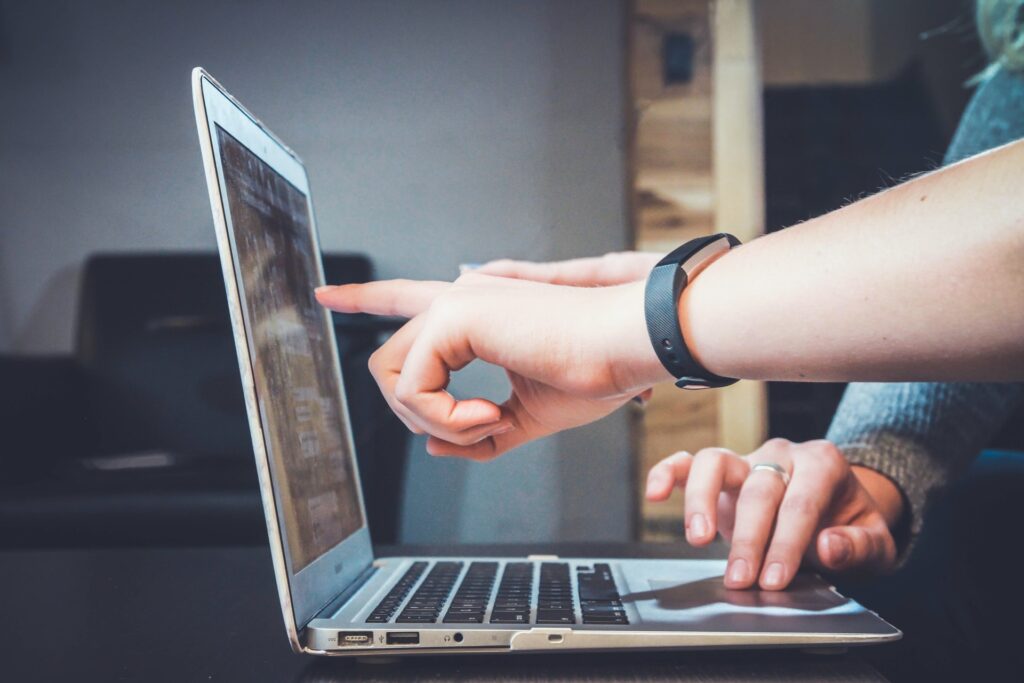
What is C++ Dynamic Memory?
C++ allows us to allocate variable or array memory at runtime.
This refers to dynamic memory allocation. In other programming languages, such as Java
and Python
, the compiler maintains variable memory automatically.
A dynamically allocated array can be used to give out memory of different sizes, which is not possible with compiler-allocated memory except for arrays with variable lengths.
The most important use is that it gives programmers flexibility.
For example:
Dynamic memory allocation
#include <iostream>
using namespace std;
int main() {
// declare an int pointer
int* pointInt;
// declare a float pointer
float* pointFloat;
// dynamically allocate memory
pointInt = new int;
pointFloat = new float;
// assigning value to the memory
*pointInt = 54;
*pointFloat = 54.54f;
cout << *pointInt << endl;
cout << *pointFloat << endl;
// deallocate the memory
delete pointInt;
delete pointFloat;
return 0;
}
Output:
54 54.54
New and delete operator for arrays
// sample created by Glenn
// C++ Program to store GPA of n number of students and display it
// where n is the number of students entered by the user
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter total number of students: ";
cin >> num;
float* ptr;
// memory allocation of num number of floats
ptr = new float[num];
cout << "Enter GPA of students." << endl;
for (int i = 0; i < num; ++i) {
cout << "Student" << i + 1 << ": ";
cin >> *(ptr + i);
}
cout << "\nDisplaying GPA of students." << endl;
for (int i = 0; i < num; ++i) {
cout << "Student" << i + 1 << ": " << *(ptr + i) << endl;
}
// ptr memory is released
delete[] ptr;
return 0;
}
Output:
Enter total number of students: 5 Enter GPA of students. Student1: 5.6 Student2: 54 Student3: 7.6 Student4: 6.6 Student5: 7.9 Displaying GPA of students. Student1: 5.6 Student2: 54 Student3: 7.6 Student4: 6.6 Student5: 7.9
In this program, we prompted the user to enter the number of pupils.
The float array point memory was allocated dynamically using new.
New and delete Operator for objects
#include <iostream>
using namespace std;
class Student {
private:
int age;
public:
// constructor initializes age to 26
Student() : age(26) {}
void getAge() {
cout << "Age = " << age << endl;
}
};
int main() {
// dynamically declare Student object
Student* ptr = new Student();
// call getAge() function
ptr->getAge();
// ptr memory is released
delete ptr;
return 0;
}
Output:
Age = 26
In this program, we’ve made a class called Student
with a private variable called age
.
In the default Student () constructor, we set age to 26 as an int p new int and print its value with the getAge function().
How do you dynamically allocate memory in C++?
In C++, some standard library functions are used to allocate a block of memory from the heap
.
Meanwhile, malloc()
and free()
are the two most important functions of dynamic memory.
The only thing that the malloc()
function needs are the size in bytes of the memory area that is being asked for.
It returns the address of the memory that was just allocated and a pointer to it.
Why do we use dynamic memory allocation in C++?
The most important benefit of dynamic memory allocation is that it gives programmers a data type, including the freedom to assign and release memory as needed to blocks of memory.
Which operator is used to allocate memory dynamically C++?
In C++, the new operator is used to allocate memory dynamically.
New operator in C++
The new operator tells the free store that memory needs to be allocated at namespace std int.
For example:
// sample created by Glenn
// declare an int pointer
int* pointVar;
// dynamically allocate memory
// using the new keyword
pointVar = new int;
// assign value to allocated memory
*pointVar = 54;
Using the new operator, we have given an int variable its own memory dynamically.
Notice that we used the pointer pointVar to make the memory allocation happen dynamically.
This is because the new operator gives back the location’s address.
When an array is used, the new operator gives back the address of the first item in the array.
For example:
pointerVariable = new dataType;
Delete operator in C++
When the delete pointer (ptr) command is used to free up memory for a C++ class object, the object’s destructor is called before the memory is freed up (if the object has a destructor).
The syntax of this operator:
delete pointerVariable;
Consider the following code:
// declare an int pointer
int* pointVar;
// dynamically allocate memory
// for an int variable
pointVar = new int;
// assign value to the variable memory
*pointVar = 54;
// print the value stored in memory
cout << *pointVar; // Output: 54
// deallocate the memory
delete pointVar;
Here, we used the pointer pointVar to allocate memory dynamically for an int variable.
Note: If the program uses a lot of unwanted memory, the system might crash because the operating system won't have enough memory. In this case, the delete operator can help keep the system from crashing at run time.
Summary
This article discusses the C++ Dynamic Memory.
It also tackles the different memory allocation programs, allocates memory, use of dynamic memory allocation, operator use to allocate memory, new operator, and delete operator.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.