When we start learning a programming language, the C++ data types is the most important thing we need to know to start coding in that language.
The data type is the kind of data that a variable can hold, such as integer
, float
, string
, or anything else.
Every language has more than one type of data, so we would need to learn more about data types to use them correctly and efficiently.
What is a data type in C++?
In C++, there are three types of data: primitive data types
, abstract data types
, and derived data types
.
Integer, floating-point, character, boolean, double floating-point, valueless or void, and wide character are all examples of primitive data types.
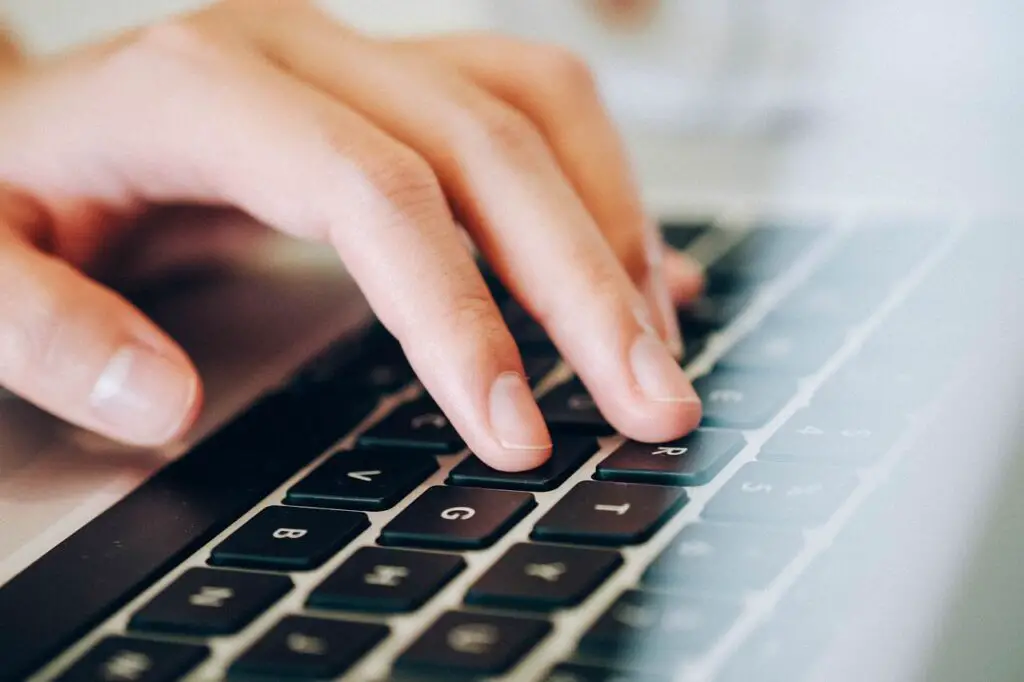
What is a data type in C++ explain in detail?
A data type tells you what kind of data a variable can hold, such as an integer, a float, a string, etc.
How many data types are there in C++?
In C++, there are three main types of data: primitive data types
, abstract data types
, and derived data types
.
Primitive data types include integer, floating-point, character, boolean, double floating-point, valueless or void, and wide character.
What are the 3 data types in C++?
There are three different kinds of data types in C++:
- Primitive
- Derived
- User Defined
What are the 5 data types?
In most modern computer languages, there are five main types of data: integral
, floating point
, character
, character string
, and composite
.
Within each of these categories, there are a number of specific subtypes.
What is the largest data type in C++?
The long long data type
is the largest built-in integral data type in standard C99 and C++0x.
Just like all the other integral data types, long does not have a specific size in bytes. Instead, it is defined to be at least a 64-bit number.
What is the data type in OOP?
In programming, a data type is a classification that says what kind of value a variable has and what kinds of mathematical, relational, or logical operations can be done on it without making an error.
In C++, data types are variables for declarations.
This decides what data variables have and how much data they have. For instance,
int age = 26;
Here, age is an integer-type variable. This means that the variable can only hold numbers that are either 2 or 4 bytes long.
Data types in C++ with examples
In the table below, you can see the basic data types, what they mean, and how big they are (in bytes):
Data Type | Meaning | Size (in Bytes) |
---|---|---|
int | Integer | 2 or 4 |
float | Floating-point | 4 |
double | Double Floating-point | 8 |
char | Character | 1 |
wchar_t | Wide Character | 2 |
bool | Boolean | 1 |
void | Empty | 0 |
Now, let’s talk about these basic data types in more depth.
C++ Int data type
- To identify the user-defined data types as integers, the int keyword is utilized.
- The variable type is int. It is typically 4 bytes in size, the int. It can therefore store values between -2147483648 and 2147483647.
For Example:
int salary = 7500;
C++ Float and Double data type
float and double
are used to store numbers with decimal points and floating point numbers (decimals and exponentials).
- The size of float takes up
4 bytes
, and double takes up8 bytes
. So, the size double is twice as accurate as float and makes less use of the amount of memory.
For Example:
float area = 26.56; double volume = 145.79794;
As mentioned above, these two data types are also used for exponentials.
For Example:
double kilometers = 55E26 // 45E12 is equal to 55*10^26
C++ Char data type
- For characters, the keyword
char
is used.
- It is 1 byte in size.
- In C++, characters are put inside single quotes, which look like this ‘ ‘.
For Example:
char sample = 'g';
Note: In C++, a char variable stores an integer value instead of the actual character.
C++ wchar_t data types
- The
wchar_t data type
is similar to the char data type, but it has two bytes instead of one. - It is used to stand in for characters that take up more memory than a single char.
For Example:
wchar_t test = G'ם' // storing Hebrew character;
Take note of the L that comes before the quotation marks.
Note: Additionally, C++11 introduces the fixed-size character types char16_t and char32_t.
C++ bool data type
True or false
is the only two valid values for the bool data type.- Loops and conditional statements both employ booleans.
For Example:
bool condition = true;
C++ void data type
- The
void keyword
indicates that there is no data. It means “nothing
” or “of no value.” - We’ll use void when we learn about functions and pointers.
Note: We can’t use void as a type for a variable.
C++ type modifiers
long
– In C++, long is a data type for a constant or variable that can hold 64 bits of information. It is a signed integer data type that stores constants or variables with larger values than the standard 32-bit integer data type.
short
– Short type must be at least 16 bits wide. A long type must have at least 32 bits wide. A long long type must have at least 64 bits wide.
signed
– All types of numbers in C++ can have or do not have a signed char. You can tell an int to only represent positive integers, for example. Unless something else is said, all integer data types are signed, and unsigned char, meaning their values can be either positive or negative.
unsigned
– The unsigned keyword is a data type specifier that tells a variable that it can only hold numbers that are not negative (positive numbers and zero). It can only be used on types of unsigned char, short, int, and long.
With the above modifiers, we can change the following data types:
- int
- double
- char
C++ Modified Data Types List
Data Type | Size (in Bytes) | Meaning |
---|---|---|
signed int | 4 | used for integers (equivalent to int ) |
unsigned int | 4 | can only store positive integers |
short | 2 | used for small integers (range -32768 to 32767) |
unsigned short | 2 | used for small positive integers (range 0 to 65,535) |
long | at least 4 | used for large integers (equivalent to long int ) |
unsigned long | 4 | used for large positive integers or 0 (equivalent to unsigned long int ) |
long long | 8 | used for very large integers (equivalent to long long int ). |
unsigned long long | 8 | used for very large positive integers or 0 (equivalent to unsigned long long int ) |
long double | 12 | used for large floating-point numbers |
signed char | 1 | used for characters (guaranteed range -127 to 127) |
unsigned char | 1 | used for characters (range 0 to 255) |
Here are some examples:
long b = 5677932; long int c = 5344346; long double d = 363454.56376; short d = 6433213; // Error! out of range unsigned int a = -6; // Error! can only store positive numbers or 0
Derived data types
Derived data types
are data types that come from fundamental data types. There are four kinds of derived data types: Function, Array, Pointer, and Reference.
Summary
Data types are an essential part of every programming language. Data type specifies what kind and how much data a variable can store.
In C++, data types are grouped into fundamental, derived, and user-defined types.
I hope this lesson has helped you learn a lot.
Check out my previous and latest articles for more life-changing tutorials which could help you a lot.