The C++ programming language provides programmers with a variety of features and capabilities, such as interface and abstract classes.
In addition, it allows object-oriented programming, which is essential for creating programs.
What is an Interface in C++?
C++ interfaces can be implemented using abstract classes.
It is possible to specify the behavior of a class in C++ without committing to a specific implementation.
This characteristic is provided by C++ objects and classes (header files).
For Example:
class Box { public: virtual double getVolume() = 0; private: double length; double width; double height; };
Data abstraction, which separates implementation details from associated data, should not be confused with abstract classes.
Abstract classes can implement C++ interfaces and at least one of a class’s functions is “pure virtual” for the class to be abstract.
The zero parameter value will be set upon adding “= 0” to the declaration of a pure virtual function.
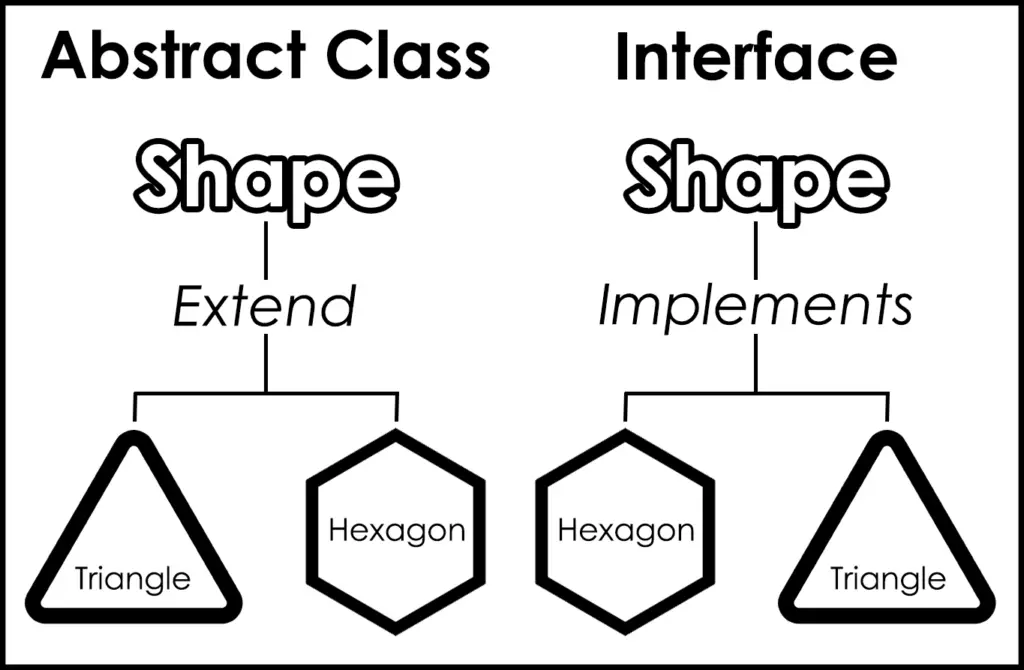
Note:
Abstract classes and data abstraction are not the same. In contrast, data abstraction is all about separating vital information from related data.
Nevertheless, the C++ interfaces and abstract classes transmit the same concept.
Abstract Class in C++
Abstract classes (commonly referred to as ABCs) create a suitable foundation class from which other classes can inherit.
They cannot express classes and merely serve as an interface. A compilation error occurs when an abstract class object is visible.
To create a subclass of ABC, it must implement each of the virtual functions, which indicates that it supports the interface provided by ABC.
A compilation error is made if a derived class doesn’t override a pure virtual function before trying to make objects of that class.
Concrete classes are able to express objects.
Abstract Class Example
Consider the following example in which the parent class provides the base class with an interface to implement the getArea()
function:
#include <iostream> using namespace std; class Shape { public: virtual int getArea() = 0; void setWidth(int w) { width = w; } void setHeight(int h) { height = h; } protected: int width; int height; }; class Rectangle: public Shape { public: int getArea() { return (width * height); } }; class Triangle: public Shape { public: int getArea() { return (width * height)/2; } }; int main(void) { Rectangle Rect; Triangle Tri; Rect.setWidth(5); Rect.setHeight(7); cout << "Total Rectangle area: " << Rect.getArea() << endl; Tri.setWidth(5); Tri.setHeight(7); cout << "Total Triangle area: " << Tri.getArea() << endl; return 0; }
Output:
Total Rectangle area: 35 Total Triangle area: 17
You can see how an abstract class provided an interface in terms of the getArea()
function, and how two additional classes implemented the same function but with different algorithms to determine the area of a certain form.
Designing Strategy
An object-oriented system could employ an abstract base class to provide a standardized and uniform interface for all external applications.
Then, by inheritance from this abstract base class, builds similarly get classes.
The abstract base class provides the capabilities (i.e., the public functions) provided by external applications as pure virtual functions.
The implementations of these pure virtual functions are provided in the application-specific derived classes.
This architecture also enables the simple addition of additional applications to a system even after defining it.
What is the difference between Interface and Abstract Class in C++?
The concept of a contract between clients and implementation is embodied by an “interface“.
On the other hand, an “abstract class” contains code that is available for sharing several interface implementations.
Even though the interface is usable through an abstract class method, it is sometimes useful to state the contract on its own.
Characteristics of an Interface in C++:
- allows for multiple implementations
- does not contain data members and constructors.
- An inheritance contains only an incomplete member (signature of a member).
- cannot have access modifiers by default; thus, everything is assumed as public.
- Members of an interface cannot be static.
Characteristics of an Abstract Class in C++:
- does not allow for inheritance
- containing constructors and data members.
- contains both incomplete (abstract) and complete members.
- can contain access modifiers for the subs, functions, and properties.
- does not include incomplete members of the abstract class to be static.
What is a Class Interface?
In C++, an interface is a way to describe the behavior of a class without taking the implementation of that class.
In layman’s terms, the C++ interface is a pure virtual function (interface or abstract class is the same).
This implies that an interface can inherit functions from any base interface as long as they are public member functions.
All interfaces are abstract, so we cannot create an instance of an object.
C++ Abstract Class Constructor
In C++, the abstract class constructor is used to initialize an object, not to build it.
If a user hasn’t given an abstract class a constructor, the program’s virtual machine will use the default constructor.
C++ Interface Destructor
In the case of the destructor, it is possible to declare a pure virtual destructor.
A destructor that deletes the allocated memory for the class is required.
Additionally, the pure virtual destructor is a destructor with a return value of 0, but it must be defined by the same class because destructors are often overridden.
Does C++ have an Interface?
No, interfaces are not a built-in concept in C++.
It can be implemented using abstract classes containing only pure virtual methods.
Since it allows multiple inheritances, you can use this class to build a new class that has the object interface by inheriting from it.
Why Interface is used in C++?
Interfaces in C++ are a means of describing the behavior of a class without committing to a particular implementation of that class.
It is an additional feature provided by C++ classes and objects.
In short, an interface is an additional feature in C++ programming that describes the behavior of a class.
What is Interface in OOP?
An “interface” in Object-Oriented Programming is a list of all the functions that an object must have.
Interfaces permit the specification of which methods a class must implement.
Interfaces simplify the use of multiple distinct classes in the same manner.
When multiple classes share the same interface, this is known as “polymorphism“.
Conclusion
In conclusion, the C++ interface and abstract class are relatively similar and different at the same time.
This may sound confusing, but they work similarly in C++ programs for different purposes.
Nevertheless, the interface in C++ is highlighted in this tutorial with examples.
The main function of the interface is to show the behavior of an object but not build the object.