In C++, Data structures are ways to organize, store, and change information.
Data structures are an important part of both computer science and software engineering.
They can be coded in any programming language.
In a coding interview, you’ll be expected to know how to use data structures to work with data efficiently.
What is a Data Structure in C++?
A data structure
is a way to put data in the computer’s memory in a way that makes it easier to use.
Data structures are a crucial part of computer science.
They are used in many areas, like statistical analysis
, operating systems
, and artificial intelligence
.
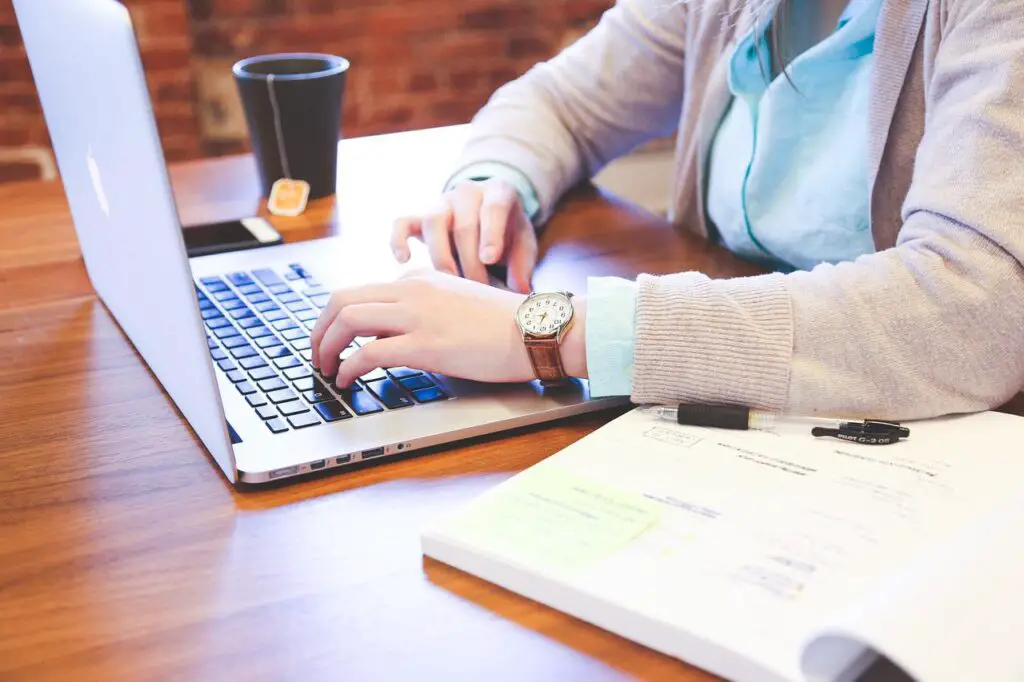
A data structure in C++
is a way to organize data in a computer’s memory so it can be used better.
Data structures are very important to computer science.
They are used in many fields, including operating systems, artificial intelligence, and statistical analysis.
Even though it is not a programming language, a data structure can be used in any language to organize the data in memory.
9 C++ Data Structures You Need To Know
Arrays
An array
is a type of linear data structure that stores elements of the same type in memory locations next to each other.
The number of elements in an array
determines how big it is.
A one-dimensional array with a size of six is shown in the picture.
In general, this is how you set up a one-dimensional array:
ArrayName [ArrayIndex] = value;
In this code, we set up an array named Example with the numbers 300 and 301 at index 0 and index 1.
#include <iostream>
using namespace std;
int main() {
int Example[2];
Example[0] = 300;
Example[1] = 301;
}
Graphs
Graphs
are non-linear data structure members.
They have a finite number of vertices (also called nodes) connected by edges.
The number of vertices in a graph is what gives it its order.
How big a graph is depends on how many edges it has.
The image above is a representation of vertices and edges. The graph also shows a cyclic graph.
A graph is cyclic if at least one of its nodes has a path that leads back to itself.
Graphs are often used to model real-life relationships in various settings, from social networks to neural networks.
There are usually 3 kinds of graphs:
- Undirected graphs
- Direct graphs
- Weighted graphs
Undirected graphs
An undirected graph
is made up of a set of nodes and a set of connections between the nodes.
Each node is called a vertex
, while each link is called an edge, and each edge connects two vertices to the namespace std struct.
The order of the two connected vertices doesn’t matter. An undirected graph
is made up of a finite number of vertices and a finite number of edges.
Direct graphs
A directed graph
, also known as a digraph
, comprises a set of vertices and a group of directed edges that connect a pair of ordered vertices.
A directed edge goes from the first vertex in a pair to the second vertex in the same pair.
Weighted graphs
Weighted graphs
are data structures for graphs in which each edge has a weight or value that depends on the type of graph being shown.
Unweighted graphs are graph data structures that don’t have any weight or value associated with them.
Linked Lists
When dealing with dynamic data elements, a linked list is the most popular data structure.
A data element
in a linked list is called a “node
.” Each node has two fields: one field has data, and the other is an address that points to the next node.
Hash Tables
Hash Table
is a type of data structure that stores data in a way that links it to other data.
This diagram shows how a hash table stores pairs of keys
and data
.
Stack and Queues
Stacks and queues
are separate but similar linear data structure types. They both hold objects and are based on arrays.
They come in different sizes and pointers to structures.
- Last-In-First-Out (LIFO) or First-In-Last-Out (FILO) is the order in which stack operations are done.
- First-in, first-out (FIFO) order is how queues work.
Strings
A string
is usually thought of as a data type. It is often implemented as an array of bytes (or words) that stores a sequence of elements, usually characters, using some kind of character encoding.
char greeting[] = "hello"
Tree
A tree
is a non-linear, hierarchical data structure made up of nodes.
Each node stores a value and a list of references to other nodes, which are called “children
.”
Binary trees
A binary tree
is a non-linear data structure in the shape of a tree. Each parent can have up to two children.
Binary search trees
A Binary Search
tree is a data structure called a node-based binary tree
.
It has the following features:
- The node’s left subtree only has nodes whose keys are less than the node’s key.
- The node’s right subtree only has nodes whose keys are bigger than the node’s key.
- Each subtree on the left and right must also be a binary search tree.
The image below shows what a binary search tree looks like.
Tries
Tries
are a very special and useful way to organize data. They are based on the first part of a string
.
They are used to show the “Retrieval
” of data, which is how they got their name.
A Tries is a special data structure for storing strings that can be seen as a graph. It is made up of points and lines and valid variables.
Every node in a try has:
- A value (can be null)
- A list of links to child nodes (can also be null)
Summary
In conclusion, you have gained knowledge of data structures.
The nine data structures covered in this article include arrays, graphs, various forms of graphs, linked lists, hash tables, stacks and queues, strings, and various tree types.
I hope this lesson helped you learn a lot.
Check out my previous and latest articles for more life-changing tutorials that could help you a lot.