C++ files and streams
In C++, files and streams are used to create files
, add information to files
, and read information from files
.
It is used to pull information out of files. It can create files and write information to them.
We use a standard C++ library called fstream classes
to read and write files.
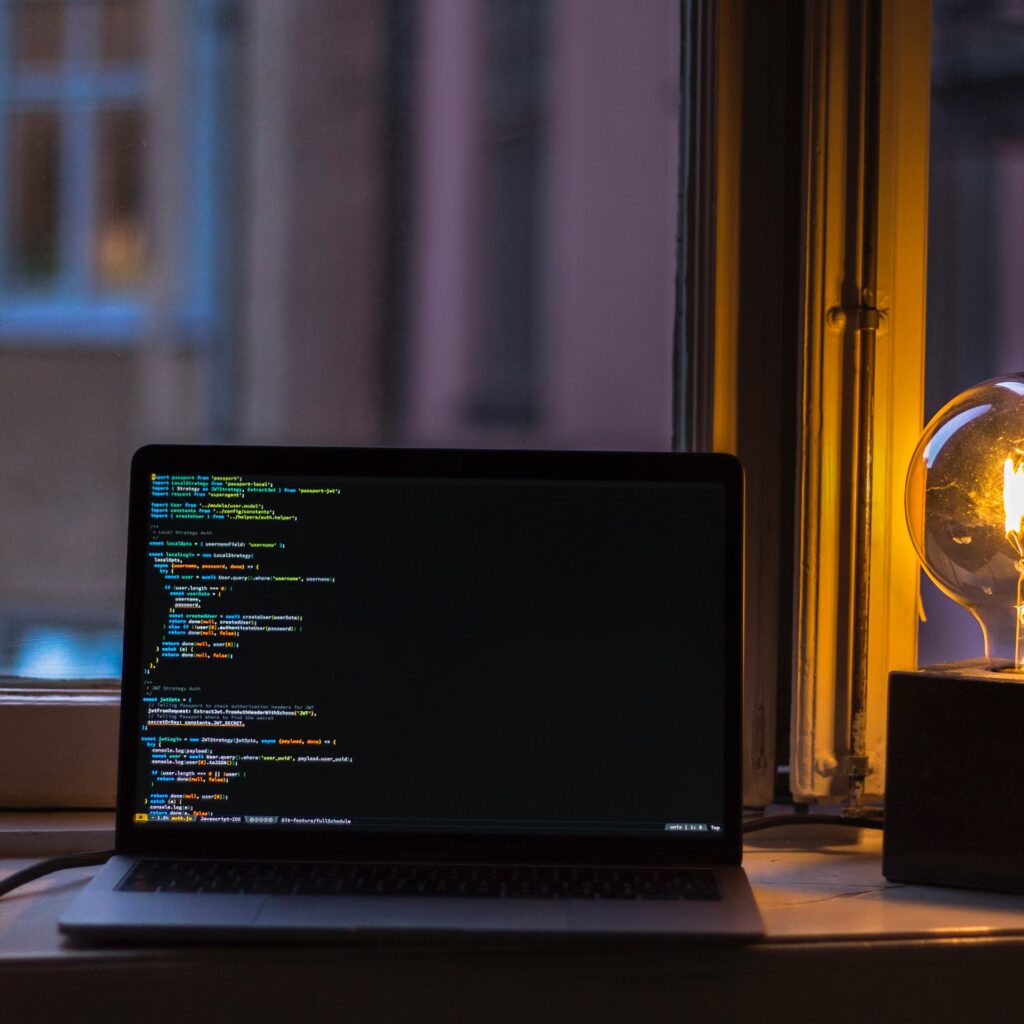
Let’s look at the data types that the fstream library can handle:
Data Type | Description |
---|---|
fstream | It is used to create files, write information to files, and read information from files. |
ifstream | It is used to read information from files. |
ofstream | It is used to create files and write information to the files. |
fstream in C++
A file stream is what the word “fstream
” stands for. A “stream
” is a series of characters that move from the disk to the C++ program or from the C++ program to the disk.
Inputting means moving characters from a file on the disk to the program.
Outputting is sending characters from a program to a file on the disk.
ifstream in C++
Ifstream is short for input-file-stream
. When characters from a file are read into a program, this is called “input
.”
ofstream in C++
This data type is a general representation of the file stream.
It has the capabilities of both fstream
and ifstream
, so it can create files, write information to files, and read information from files.
What are C++ files?
Files are used to store information in a permanent way on a storage device.
File handling lets you store the results of a program in a file and do different things with it.
What are C++ streams?
A stream in C++ is a way for data to flow into or out of a program, like when data is written to cout
or read from cin
.
The Stream Class Hierarchy:
istream
– is a general purpose input stream. cin is an example of an istream.
ostream
– is a general purpose output stream. cout and cerr are both examples ofostreams
.
ifstream
– is an input file stream. It is a special kind of an istream that reads in data from a data file.
ofstream
– is an output file stream. It is a special kind of ostream that writes data out to a data file.
What are the file stream?
A file stream is a list of bytes that is used to store file data
.
Most of the time, a file only has one file stream, which is the default data stream.
But on file systems that can handle more than one data stream, each file can have more than one data stream.
The default data stream, which has no name, is one of these.
Opening a File
Before we can read or write to a file, we have to open it.
To open a file for writing, we can use either the ofstream
or fstream
object.
And the only thing we can do with an ifstream
object is read from a file.
The standard way to use the open()
function, which is part of the fstream
, ifstream
, and ofstream
objects, is shown below.
void open(const char *filename, ios::openmode mode);
Here, the first argument tells the open()
member function the name and location of the file to be opened, and the second argument tells the function how to open the file.
Sr.No | Mode Flag & Description |
---|---|
1 | ios::app Append mode. All output to that file is to be appended to the end. |
2 | ios::ate Open a file for output and move the read/write control to the end of the file. |
3 | ios::in Open a file for reading. |
4 | ios::out Open a file for writing. |
5 | ios::trunc If the file already exists, its contents will be truncated before opening the file. |
We can use OR
to combine two or more of these values.
For example, if we want to open a file in write mode and truncate it if it already exists, the syntax is as follows:
ofstream outfile;
outfile.open("file.dat", ios::out | ios::trunc );
In the same way, we can open a file to read and write by doing the following:
fstream afile;
afile.open("file.dat", ios::out | ios::in );
Closing a File
When a C++ application finishes running, it immediately closes all open files, flushes all streams, and releases all memory that has been allocated.
But before finishing a program, a programmer should always make sure that all open files are closed.
The standard way to write the close()
function, which is a part of the fstream
, ifstream
, and ofstream
objects, is shown below.
void close();
Writing to a File
Using the stream insertion operator (<<) in C++, we can write information to a file just like we write information to the screen.
The only difference is that when we write, we use an ofstream
or ifstream
object instead of the cout
object.
Reading from a File
With the stream extraction operator (>>), we can read information from a file into a program, just like how we can type information from the keyboard. We use an ifstream
or ofstream
object instead of a cin
object, which is the only difference.
File Position Pointers
Both ifstream and ofstream have functions for moving the file-position pointer.
The seekg (“seek get
“) function for ifstream and the seekp (“seek put
“) function for ofstream are examples of member functions.
Most of the time, a long number is given as the argument to seekg
and seekp
.
A second argument can be used to show where the search should go.
We can set the seek direction to ios::beg, which is the default, to move to the beginning of a stream, ios::cur to move to the current position in a stream, or ios::end to move to the end of a stream.
The file-position pointer is an integer that indicates your current file location in terms of how many bytes you are from the file’s start.
The “get” file-position pointer can be positioned in the following ways:
// position to the nth byte of fileObject (assumes ios::beg)
fileObject.seekg( n );
// position n bytes forward in fileObject
fileObject.seekg( n, ios::cur );
// position n bytes back from end of fileObject
fileObject.seekg( n, ios::end );
// position at end of fileObject
fileObject.seekg( 0, ios::end );
C++ Read and Write Example
The C++ application that enables reading and writing to files is available here.
The program reads a file called afile.dat that the user has entered information into and displays the data on the screen.
#include <fstream>
#include <iostream>
using namespace std;
int main () {
char data[100];
// open a file in write mode.
ofstream outfile;
outfile.open("afile.dat");
cout << "Writing to the file" << endl;
cout << "Enter your name: ";
cin.getline(data, 100);
// write inputted data into the file.
outfile << data << endl;
cout << "Enter your age: ";
cin >> data;
cin.ignore();
// again write inputted data into the file.
outfile << data << endl;
// close the opened file.
outfile.close();
// open a file in read mode.
ifstream infile;
infile.open("afile.dat");
cout << "Reading from the file" << endl;
infile >> data;
// write the data at the screen.
cout << data << endl;
// again read the data from the file and display it.
infile >> data;
cout << data << endl;
// close the opened file.
infile.close();
return 0;
}
When the code above is compiled and run, the following are some examples of input and output operations:
$./a.out
Writing to the file
Enter your name: Glenn
Enter your age: 26
Reading from the file
Glenn
26
The aforementioned examples make use of cin
object properties like getline()
for reading from the outside and ignore()
for ignoring extra characters left over from the read command.
Summary
This article discusses files and streams in C++. It also tackles file opening, closing a file, writing to a file, reading from a file, and file position pointers.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.
terimakasih infonya menarik kerren
Thanks to your compliment