This discussion will give you a well-detailed explanation of PHP web concepts.
It covers everything you need to know about PHP applications in web development and how the language provides dynamic content pages in different browser types.
Determine Browser and Platform
PHP programming language generates environment variables that are utilized to configure the PHP environment.
The language also has a function called getenv() that lets you see the values of all environment variables.
PHP sets the HTTP_USER_AGENT environment variable, which identifies the user’s browser and operating system.
With the HTTP_USER_AGENT environment variable, you can make dynamic content that is specific to a browser.
Example:
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<?php
function getBrowser() {
$user_agent = $_SERVER['HTTP_USER_AGENT'];
$base_name = 'Unknown';
$platform = 'Unknown';
$version = "";
//First get the platform?
if (preg_match('/linux/i', $user_agent)) {
$platform = 'linux';
}elseif (preg_match('/macintosh|mac os x/i', $user_agent)) {
$platform = 'mac';
}elseif (preg_match('/windows|win32/i', $user_agent)) {
$platform = 'windows';
}
// Next get the name of the useragent yes seperately and for good reason
if(preg_match('/MSIE/i',$user_agent) && !preg_match('/Opera/i',$user_agent)) {
$base_name = 'Internet Explorer';
$user_browser = "MSIE";
} elseif(preg_match('/Firefox/i',$user_agent)) {
$base_name = 'Mozilla Firefox';
$user_browser = "Firefox";
} elseif(preg_match('/Chrome/i',$user_agent)) {
$base_name = 'Google Chrome';
$user_browser = "Chrome";
}elseif(preg_match('/Safari/i',$user_agent)) {
$base_name = 'Apple Safari';
$user_browser = "Safari";
}elseif(preg_match('/Opera/i',$user_agent)) {
$base_name = 'Opera';
$user_browser = "Opera";
}elseif(preg_match('/Netscape/i',$user_agent)) {
$base_name = 'Netscape';
$user_browser = "Netscape";
}
// finally get the correct version number
$known = array('Version', $user_browser, 'other');
$pattern = '#(?<browser>' . join('|', $known) . ')[/ ]+(?<version>[0-9.|a-zA-Z.]*)#';
if (!preg_match_all($pattern, $user_agent, $matches)) {
// we have no matching number just continue
}
// see how many we have
$i = count($matches['browser']);
if ($i != 1) {
//we will have two since we are not using 'other' argument yet
//see if version is before or after the name
if (strripos($user_agent,"Version") < strripos($user_agent,$user_browser)){
$version= $matches['version'][0];
}else {
$version= $matches['version'][1];
}
}else {
$version= $matches['version'][0];
}
// check if we have a number
if ($version == null || $version == "") {$version = "?";}
return array(
'userAgent' => $user_agent,
'name' => $base_name,
'version' => $version,
'platform' => $platform,
'pattern' => $pattern
);
}
// now try it
$ua = getBrowser();
$yourbrowser = "Your browser: " . $ua['name'] . " " . $ua['version'] .
" on " .$ua['platform'] . " reports: <br >" . $ua['userAgent'];
print_r($yourbrowser);
?>
</body>
</html>
Output:
Your browser: Google Chrome 105.0.0.0 on windows reports: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/105.0.0.0 Safari/537.36
Remember:
The method preg_match()
looks for a pattern in a string and returns true
if the pattern is found or false
otherwise.
However, if the optional input parameter pattern array is available, it will, if relevant, contain several subpattern components.
Also, if this flag is present as PREG_OFFSET_CAPTURE, the string offset for each match returns.
Please take note that the output of the example program varies on the type of platform you use and the browsers that are present on your device.
Display Randomly Images
Programmers may also use PHP programming to display images on the web.
The rand() function from the PHP’s built-in functions can generate random numbers within a specific range.
Example:
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<?php
$num = rand( 1, 4 );
switch( $num ) {
case 1: $image_file = "/PHPtuts/images/ITSCblack.png";
break;
case 2: $image_file = "/PHPtuts/images/ITSCblue.png";
break;
case 3: $image_file = "/PHPtuts/images/LOGOblack.png";
break;
case 4: $image_file = "/PHPtuts/images/LOGOblue.png";
break;
}
echo "<img src=$image_file />";
?>
</body>
</html>
Output:
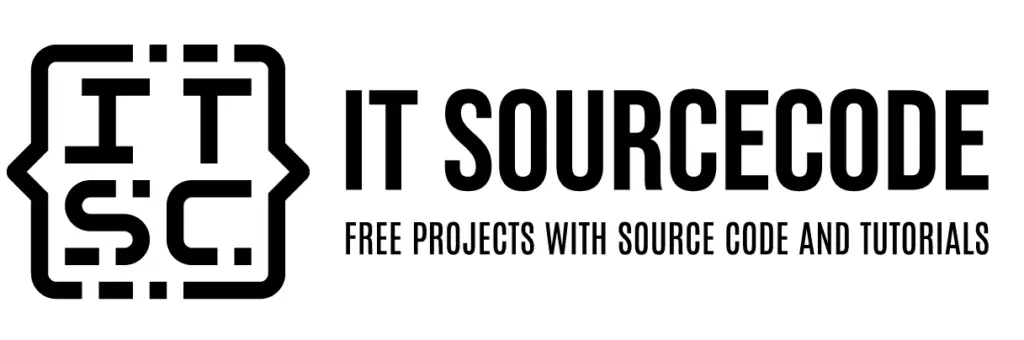
The output of the example program changes as you reload the web page. In the program, four different images display randomly as you reload the URL.
In the example, the path "/PHPtuts/images/..."
represents the folder where we store the images.
This must be included and observed properly to avoid errors. Additionally, the file that the web page runs must also be with .PHP
or .php
file extension.
For example, our program was saved as images.php
, then the web page shows the image below:
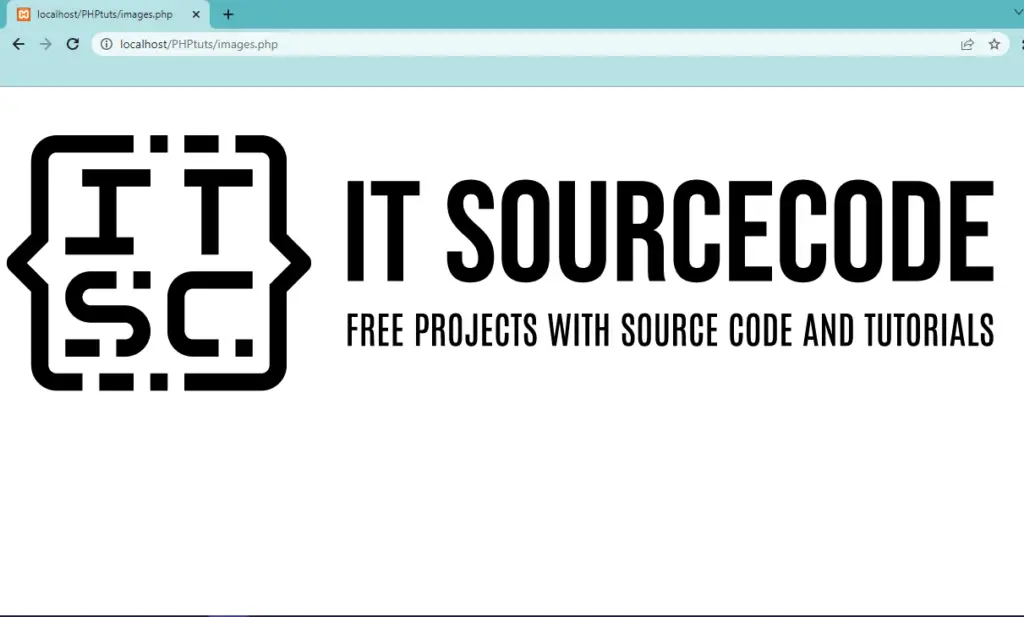
You also need to make sure that you have your server activated on your computer. In this example, the server that enabled the program to run was XAMPP.
Now, to run the images.php file, the localhost (XAMPP) server needs to be indicated in the URL. As a result, the full path of the program to run on a web page is localhost/PHPtus/images.php.
Using HTML Forms
Another web concept in PHP is the use of HTML forms and you will notice this when you deal with both HTML and PHP.
This is because any of HTML’s form elements will also appear in the PHP scripts automatically.
The question is, how does PHP work with HTML forms? Let us see the example programs below.
Example:
HTML Form as form.html
<html>
<body>
<form action="welcome.php" method="post">
Name: <input type="text" name="name"><br>
<br/>
Gender: <input type="text" name="gender"><br>
<br/>
<input type="submit">
</form>
</body>
</html>
This example program will generate a form that will look like the sample form below.
Output:
With this empty form, you may enter any input that corresponds to the field that the form asks.
For example:
After inputting information in the form’s field, you may click the submit button to see how the PHP reacts with the HTML form.
But, you must first make sure that you have the PHP script available in your files.
This script should have the sample code such as below:
Program Example: PHP Script as welcome.php
<html>
<body>
<?php
echo 'Welcome '.$_POST["name"]."<br>";
echo 'You are a: '.$_POST["gender"];
?>
</body>
</html>
The PHP script (welcome.php) will serve as the receiver of the inputs from the HTML form (form.html). This script uses the $_POST[]
the function of PHP to display the inputs “name
” and “email
” from the HTML form along the string in the echo function.
As a result of this PHP script, you will see the output as follows:
As seen in the example programs and outputs from the process, the interaction between HTML form and PHP script is clear as crystal.
Through the process, you can conclude that PHP helps HTML deliver an efficient way of receiving inputs and displaying outputs.
Take Note: The example shown only displays the basic web concept of PHP regarding HTML forms.
Additionally, you can merge the HTML form with the PHP script in one file.
For example:
<html>
<body>
<form action = "<?php $_PHP_SELF ?>" method = "POST">
Name: <input type = "text" name = "name"/>
Age: <input type = "text" name = "age"/>
<input type = "submit" />
</form>
<?php
if( empty($_POST["name"]) || empty($_POST["age"]) ) {
echo "";
}else{
echo "Welcome ". $_POST['name']. "<br />";
echo "You are ". $_POST['age']. " years old.";
exit();
}
?>
</body>
</html>
Output:
Upon running the example program, an empty form will display and it is available to receive inputs as shown below.
After filling in the fields of the form, you may then click the submit button.
Welcome Johnny You are 28 years old.
As soon as you click the submit button, this output will display. This proves that either separated files or merged, the PHP script and the HTML form can work according to the functions assigned to them.
Either of the examples can be available and applicable to your program as long as you are comfortable using them. It is always up to the developer which of the methods looks more organized.
Browser Redirection
A redirection enables the user’s browser to be redirected to a different URL. One of the methods that provide the browser with raw HTTP headers is the PHP header() function.
The script for redirection should be placed at the very top of the page to prevent any other content from loading.
Moreover, the Location: header specifies the target as the parameter of the header()
function. After invoking this procedure, the exit()
function can be used to stop parsing the remaining code.
Example:
<?php
if(empty($_POST["location"])) {
echo " ";
}else{
$location = $_POST["location"];
header( "Location:".$location );
exit();
}
?>
<html>
<body>
<p>Choose a site to visit :</p>
<form action = "<?php $_SERVER['PHP_SELF'] ?>" method ="POST">
<select name = "location">.
<option value = "http://www.ITSourceCode.com">
itsourcecode.com
</option>
<option value = "http://www.PythonforFree.com">
pythonforfree.com
</option>
</select>
<input type = "submit" />
</form>
</body>
</html>
Output:
This output will show as soon as you run the example program. You may choose any of the options and then click the submit button to test if the program works properly.
Related Articles
- Constants in PHP (Definition With Examples)
- GET and POST Method In PHP (GET vs POST)
- PHP Chmod Dir Function With Examples
- PHP Anonymous Functions with Example Programs
- PHP Trim Function With Examples
Conclusion
In conclusion, this discussion about the PHP web concepts lets you discover the functions and possibilities when you are into PHP programming.
It highlights the different concepts and applications of PHP in developing the web along with its demonstrations in the examples.
Nevertheless, there are a lot of concepts and opportunities to discover when using PHP programming for web development.
Stay tuned to this site to learn more about boosting skills in the PHP programming language.