What is decision-making in PHP?
Decision-making in PHP is the method that enables programmers to create options or decisions through conditional statements.
Moreover, for decision-making statements to work, the programmer must define one or more conditions that the program will test or evaluate.
If the condition is true, the conditional statements will be run. However, if the condition is false, other statements will sometimes be run instead.
Take a good look at the flow of a simple decision-making statement below.
What are the different types of decision-making statements in PHP?
There are different types of decision-making statements that we can explore in this article.
PHP programming enables the application of decision-making by the following conditional statements:
- if Statement
- if … else Statement
- elseif Statement
- switch Statement
PHP enables actions to be performed based on logical and comparative conditions. In addition, the result of these circumstances (either TRUE or FALSE) will be a user-requested action.
Consequently, a conditional statement functions similarly to a two-way path: if you desire a particular outcome, the program executes as instructed; otherwise, the opposite occurs.
To define the different types of decision-making in PHP, let us start with…
1. if Statement
The PHP if statement is the simplest form of conditional statement when applied to a program. A piece of code is only executed if the condition evaluates to true.
In addition, the if statement allows programmers to determine an action depending on a particular condition. The syntax for the PHP if statement is presented below for your reference.
Syntax:
if (condition){
// execute this block if true
}
Let us try the if statement with an example program and see how this condition works.
Example:
<?php
$num = 25;
if ($num > 10) {
echo "$num is greater than 10.";
}
?>
Output:
25 is greater than 10.
This is how the statement works, the value of the variable $num is 25 and it is compared to the assigned value in the condition which is 10.
So if the condition $num > 10 is true, the output will show that 25 is greater than 10.
However, if the condition result is false, the program will not show any output.
Here’s a flowchart that will help you understand how the if statement flows
Flowchart:
2. if…Else Statement:
The if statement is understood as a condition with one assigned function and a supporting action once the result is true.
But if the result is false, the program will not show anything unless you add the else statement.
The if … else decision-making in PHP allows programmers to have two executable blocks of code to support either true or false results.
Syntax:
if (condition) {
// execute this block if the result is true
}
else{
// execute this block if the result in the first block is false
}
Let us have an example program where we can implement the if … else statement.
Example:
<?php
$num = 25;
if ($num > 100) {
echo "$num is greater than 100.";
}
else{
echo "$num is less than 100.";
}
?>
Output:
25 is less than 100.
To explain the program, the first block of code (if condition) will display "25 is greater than 100."
if the condition is true.
But if it is false, the program will execute the second block of code (else condition) and display "25 is less than 100."
.
Flowchart:
3. elseIf Statement
Another condition in PHP is the elseif statement, which enables programmers to include multiple conditions within the if… else statements.
As a clarification, you can use the else
statement (after an if
) to describe a block of code that will only be executed if the first condition is false.
But if the first test condition is false, use elseif
to define another test condition.
Syntax:
if (condition) {
// execute this block if the result is true
}
elseif {
// execute this block if the result in the first block is false
}
else {
// execute this block if the result in the first and second blocks are false
}
Example:
<?php
$num = 100;
if ($num > 100) {
echo "$num is greater than 100.";
}
elseif ($num < 100) {
echo "$num is less than 100.";
}
else{
echo "$num is equal to 100.";
}
?>
Output:
100 is equal to 100.
Flowchart:
4. switch Statement
The switch statement executes several cases that match the condition and executes the case block as appropriate.
It evaluates an expression before comparing it with each case’s values. As a result, whenever a case is matched, an identical case is run.
However, to use the switch statement in PHP decision-making, you have to be familiar with two distinct terms:
- break – After the code for the first true case has been run, it tells the program to leave the switch-case statement block.
- default – It is applied when there is no case in which the constant-expression value equals the expression’s value. If there is no default statement and there is no case match, none of the statements in the switch body are run.
Syntax:
switch(condition) {
case statement1:
execute this block if the condition mathched with statement1;
break;
case statement2:
execute this block if the condition mathched with statement2;
break;
case statement3:
execute this block if the condition mathched with statement3;
break;
......
default:
default code to be executed if none of the cases matched the condition;
Example:
<?php
$day = "Sunday";
switch($day) {
case "Monday":
echo "Today is Monday";
break;
case "Teusday":
echo "Today is Teusday";
break;
case "Wednesday":
echo "Today is Wednesday";
break;
case "Thursday":
echo "Today is Thursday";
break;
case "Friday":
echo "Today is Friday";
break;
case "Saturday":
echo "Today is Saturday";
break;
case "Sunday":
echo "Today is Sunday";
break;
default:
echo "Doesn't exist";
}
?>
Output:
Today is Sunday
Flowchart:
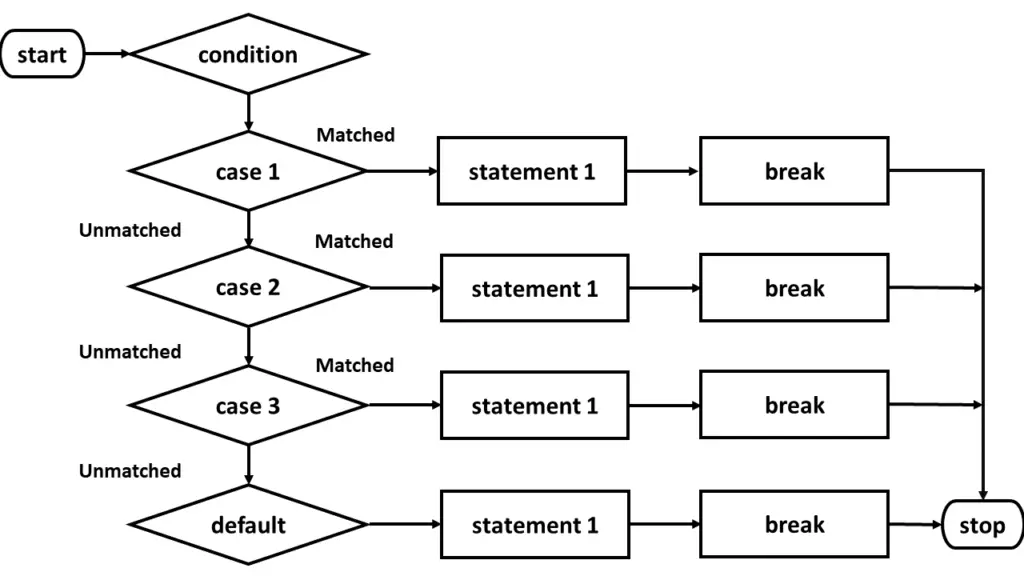
Related Articles
- Types of Loops in PHP (With Flowcharts and Program Examples)
- PHP Shorthand If ElseIf Else
- PHP Elvis Operator (Detailed Explanation With Examples)
- Not in Array PHP (With Advanced Program Examples)
- PHP Tutorial For Beginners – Easy Learning In PHP
Conclusion
In conclusion, the Decision-Making in PHP topic has covered all of the conditional statements used in PHP programming.
The conditional statements will help you apply the programming logic and expand your skills in decision-making and creating reasonable options.
Hoping that you have learned the main reason for this discussion. If you have any concerns you can tap us through the comments below.