What is an Operator in PHP?
An operator in PHP is a symbol that is used to perform an operation on the operands.
In a simple way, these operators can also be used to perform an operation on values or variables.
For example:
$sum = 20 + 30; (20 and 30) are operands and (+) is the operator.
The Operator in PHP is something that takes (one or more) expressions or any values in programming and submits another value in order to become an expression by itself.
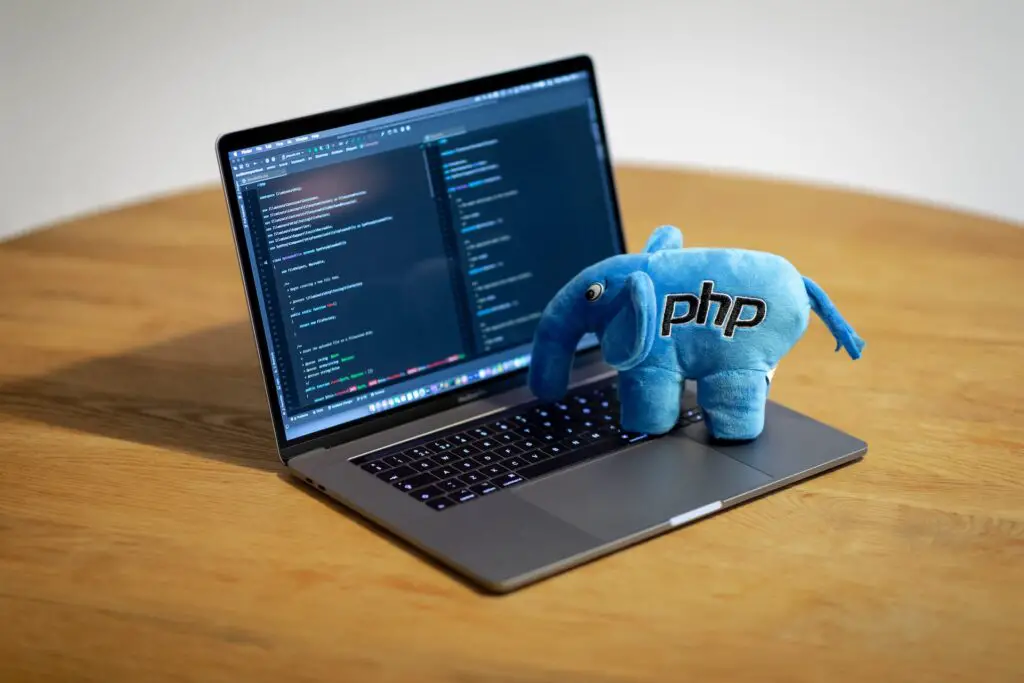
What are the different types of operators in PHP?
There are 8 different types of operators in PHP
- String operators
- Conditional assignment operators
- Array operators
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
String operators in PHP
A concatenation operator and concatenating assignment operator are the two string operators which are only designed for strings.
The concatenation operator (‘. ‘) returns a concatenation from the right and left arguments, and the concatenating assignment operator (‘ . = ‘) appends an argument from the right side to the left side of the argument.
Operator | Name | Syntax | Operation |
---|---|---|---|
. | Concatenation | $g.$l | Concatenated $g and $l |
.= | Concatenation and assignment | $g.=$l | First concatenates then assigns, same as $g = $g.$l |
Conditional assignment / Ternary operators in PHP
The conditional assignment operator or conditional operator is an operand of assigned values that is based on the outcome of a specific program condition.
Once the program condition becomes true the value is assigned, but once the program condition is false the value is not assigned.
Syntax:
$var = (condition)? value1 : value2;
Operator | Name | Operation |
---|---|---|
?: | Ternary | If the condition is true? then $g : or else $l. This means that if the condition is true then the left result of the colon is accepted otherwise the result is on right. |
Array operators in PHP
The array operators are commonly used to compare (arrays).
Operator | Name | Syntax | Operation |
---|---|---|---|
+ | Union | $g + $l | Union of both i.e., $x and $y |
== | Equality | $g == $l | Returns true if both have the same key-value pair |
!= | Inequality | $g != $l | Returns True if both are unequal |
=== | Identity | $g === $l | Returns True if both have the same key-value pair in the same order and of the same type |
!== | Non-Identity | $g !== $l | Returns True if both are not identical to each other |
<> | Inequality | $g <> $l | Returns True if both are unequal |
Arithmetic operators in PHP
In PHP, arithmetic operators return numeric values to perform mathematical operations such as (multiplication, addition, subtraction, and addition).
Operator | Name | Syntax | Operation |
---|---|---|---|
+ | Addition | $g + $l | Sum the operands |
– | Subtraction | $g – $l | Differences the operands |
* | Multiplication | $g * $l | Product of the operands |
/ | Division | $g / $l | The quotient of the operands |
** | Exponentiation | $g ** $l | $x raised to the power $y |
% | Modulus | $g % $l | The remainder of the operands |
Assignment operators in PHP
The assignment operators are used in numeric values to write a value to a declared variable.
In addition, the most basic assignment operator in PHP is an equal sign ( = ) which means that the left operands are equal to the right value of the assigned result.
Operator | Name | Syntax | Operation |
---|---|---|---|
= | Assign | $g = $l | Operand on the left obtains the value of the operand on the right |
+= | Add then Assign | $g += $l | Simple Addition same as $g = $g + $l |
-= | Subtract then Assign | $g -= $l | Simple subtraction same as $g = $g – $l |
*= | Multiply then Assign | $g *= $l | Simple product same as $g = $g * $l |
/= | Divide then Assign (quotient) | $g /= $l | Simple division same as $g = $g / $g |
%= | Divide then Assign (remainder) | $g %= $l | Simple division same as $g = $g % $g |
Comparison operators in PHP
The comparison operators are used to compare two values a number or string and return true if the (comparison) is correct and otherwise is false.
== | Equal to | Return true if both operands are equal; otherwise, it returns false . |
!=, <> | Not equal to | Return true if both operands are equal; otherwise, it returns false . |
=== | Identical to | Return true if both operands have the same data type and equal; otherwise, it returns false . |
!== | Not identical to | Return true if both operands are not equal or do not have the same data type; otherwise, it returns false . |
> | Greater than | Return true if the operand on the left is greater than the operand on the right; otherwise, it returns false . |
>= | Greater than or equal to | Return true if the operand on the left is greater than or equal to the operand on the right; otherwise, it returns false . |
< | Less than | Return true if the operand on the left is less than the operand on the right; otherwise, it returns false . |
<= | Less than or equal to | Return true if the operand on the left is less than or equal to the operand on the right; otherwise, it returns false . |
Increment/Decrement operators in PHP
An increment operator is used to increment a value assigned to a variable and the decrement operator is used to decrement a value assigned to a variable.
Operator | Name | Syntax | Operation |
---|---|---|---|
++ | Pre-Increment | ++$x | First increments $x by one, then return $x |
— | Pre-Decrement | –$x | First decrements $x by one, then return $x |
++ | Post-Increment | $x++ | First returns $x, then increment it by one |
— | Post-Decrement | $x– | First returns $x, then decrement it by one |
Logical operators in PHP
The logical operators are used to combine conditional statements and expressions.
Operator | Name | Syntax | Operation |
---|---|---|---|
and | Logical AND | $g and $l | True if both the operands are true else false |
or | Logical OR | $g or $l | True if either of the operands is true else false |
xor | Logical XOR | $g xor $l | True if either of the operands is true and false if both are true |
&& | Logical AND | $g && $l | True if both the operands are true else false |
|| | Logical OR | $g || $l | True if either of the operands is true else false |
! | Logical NOT | !$g | True if $x is false |
Related Articles
- PHP Shorthand If ElseIf Else
- PHP Spread Operator (with Advanced Program Examples)
- PHP Elvis Operator (Detailed Explanation With Examples)
- PHP Coding Standards with Best Example
- PHP Tutorial For Beginners – Easy Learning In PHP
Summary
This article discusses the different Types of Operators in PHP such as string operators, conditional assignment operators, array operators, arithmetic operators, assignment operators, comparison operators, increment/decrement operators, and logical operators.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.