This article explains the difference between lists and dictionaries and when to use each one in Python programming.
Python has various in-built data structures that make programming easy and efficient, like any other programming language. All these data structures are sequential and store data in different formats. Some of the most simple yet powerful data structures are lists and dictionaries.
What are Lists in Python?
Python has a data structure called a list, which is an ordered sequence of mutable elements. Each element in a list is called an item. Lists are made up of values that are separated by commas and enclosed in square brackets ([]
).
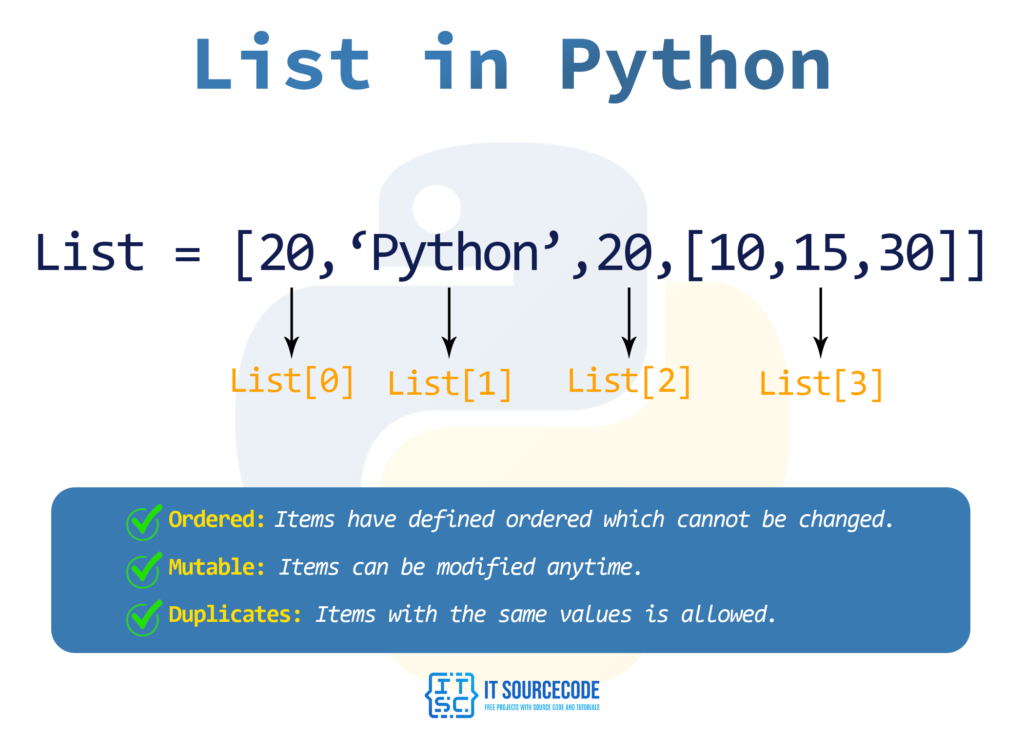
When you need to deal with related values, a list is a great tool to use. Since lists can be changed, you can add, update, or delete items at any time while programming.
For Example:
x_list = ["IT", "Source", "Code"]
print(x_list)
Output
['IT', 'Source', 'Code']
What is Dictionary in Python?
Dictionary is a type of data structure that comes with Python. It is used to store a collection of data in the form of key-value pairs.
A dictionary used to be unordered collection, but in Python 3.6, the order is preserved to some extent.
Dictionary entries are written inside the curly brackets {}
, with commas between each entry. The key and value of the data, on the other hand, are separated by a semicolon (:
).
Dictionary elements are in a certain order, can be changed, and can’t be duplicated. Remember that every data value should have a unique key name, and that case matters.
To access the elements in a dictionary, you can use the name of the key to find the associated data value.
For Example:
x_dict = {
"programming": "Python",
"device": "laptop",
"drink": "coffee"
}
print(x_dict)
Output
{'programming': 'Python', 'device': 'laptop', 'drink': 'coffee'}
Difference Between List and Dictionary in Python
Definition – A list is a collection of various elements. A dictionary is a collection of key-value pairs in a hashed structure.
Syntax – A list is a collection of items enclosed in square brackets ([]
), with each item separated by a comma (,
). A dictionary is a collection of key-value pairs in curly brackets ({}
) separated by a semi-colon (:
).
Index Type – A list index starts at
. The keys in a dictionary can be any data type.0
Mode of Access – Lists can access elements by index value, while dictionaries can access elements by key.
Order of Elements – List elements always maintain their original order, but dictionaries do not.
Mutability – Lists are mutable, which means they can be changed. Dictionary entries are also mutable, but you cannot have duplicate keys.
Creation – To create a list object, you can call the list()
function. To create a Dictionary object, you can call the dict()
function.
Sort()
– Sort()
method in list sorts the elements in ascending or descending order. Dictionary sorts the keys in the dictionary by default
Count()
– List returns the number of elements that appeared in the list. In dictionary, Count()
method does not exist.
Reverse()
– Reverse()
method in list reverses the list elements. In a dictionary, items cannot be reversed as they are key-value pairs.
When to Use a Dictionary vs List in Python?
Lists are data structures that store ordered collections of data in a specific order. Dictionaries store data using key-value pairs that are organized using a hash table. Dictionaries are more efficient than lists for looking up elements, as they are faster and take less time to traverse.
Lists preserve the order of data elements, while dictionaries do not. Therefore, if the order of data elements is important, it is advisable to use a list.
A list data structure is recommended for data values that might need to be changed in the future. It can be difficult to modify dictionary keys later on because they need to be unique.
Dictionaries in Python take up much less space to store data elements than lists do.
When considering all the above parameters, it is clear that dictionaries are more efficient to use than lists.
Applications of List
Dictionaries are often used in Python to store data, make indexes, make map objects, and create data frames.
Applications of Dictionary
Dictionaries are used for a variety of purposes in Python, including storing large amounts of data for quick and easy access, building indexes for content, creating map objects, and creating data frames with lists.
Conclusion
Lists and dictionaries are the most fundamental data structures in Python programming. Dictionaries and lists are both essential for storing linear data while programming, but they have different syntax, storage methods, and implementations.
Inquiries
If you have questions or suggestions about the difference between lists and dictionaries in Python, please let me know. You may either leave a comment on this page or contact me via our website.