Like other programming languages, PHP has also built-in and user-defined functions that you may explore.
Now, this PHP function with advanced examples will guide you through the language’s functions and discover more of your programming skills.
PHP Built-in Functions
PHP programming is a very efficient and effective scripting language with a large number of built-in functions. These built-in functions come with the installation package setup.
Additionally, there are other categories into which the language’s built-in functions are in.
This is because it has more than 1000 built-in functions that need to show where they belong in programming.
PHP User Defined Functions
The PHP user-defined functions, on the other hand, are the functions from the programmer’s concept.
These functions were not based on the language but were created to support the function that is not available in the language’s package.
Programmers apply user-defined functions to create unique functions in their programs. For this instance, the characteristic of a user-defined function includes:
- It is a collection of statements that repeats throughout a program.
- This function does not execute automatically when a webpage loads.
- Its call to a function will execute the function.
Create a User-Defined Function in PHP
In this section, you will learn how to create a user-defined function in PHP. But note that the example below varies depending on the kind of function that you want to imply.
Syntax:
SampleFunction FunctionName() {
code for the user-defined function;
}
Remember that the first character of a function name must be a letter or an underscore. However, the function names are case-insensitive.
Example:
In this example, we will try to create a user-defined function named “Mytxt()
“.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<?php
function Mytxt() {
echo "Welcome to ITSourceCode.com!";
}
Mytxt(); // call the function
?>
</body>
</html>
Output:
Program Explanation:
The sample function Mytxt()
implements its scope within the open and closed curly braces ({ function; }
).
As seen in the output, the function displays the text containing “Welcome to ITSourceCode!
“.
PHP Function Arguments
In PHP, arguments are similar to variables and they often pass through value.
This indicates that a copy of the value is available within the function, while the variable given to the function is unchangeable.
In addition, arguments are present within parentheses following the function name.
You may include as many arguments as you like, but you must separate them with commas.
Example:
<!DOCTYPE html>
<html>
<body>
<?php
function Country($cityName) {
echo "$cityName Philippines<br>";
}
Country("Pasig City,");
Country("Bacolod City,");
Country("Manila City,");
Country("Baguio City,");
Country("Davao City,");
?>
</body>
</html>
Output:
Program Explanation:
To explain the scenario, when we call the Country()
method, it also takes a corresponding $cityName
as it was assigned as the function’s parameter.
This $cityName
is the basis of the code to create a list of different city names with the same country name.
PHP is a Loosely Typed Language
PHP is different from other scripting languages in that it doesn’t have strict rules about how code should be written.
However, based on the value of the variable, PHP automatically assigns a data type to it. Since the data types are not strictly defined, it is possible to add a string to an integer without running into problems.
Type declarations, however, were included in PHP 7. Including the strict
declaration will cause it to throw a “Fatal Error
” if the data types don’t match.
Now, the example below will try to call the function without using strict
while passing both an integer and a string:
Example:
<?php
function AddNum(int $x, int $y) {
return $x + $y;
}
echo AddNum(10, "4");
?>
Output:
Program Explanation:
The example program has two variable integers which are the $x
and $y
, and it should return the sum of the two variables through the return $x + $y; function
.
The output proves that the program can still perform the addition function even if the other variable value is a string.
PHP Default Argument Value
In PHP, a value specified in the function declaration serves as the default argument.
If the caller function doesn’t supply a value for the argument, the compiler will automatically assign the default.
Moreover, the default value is overridden if the calling function has an assigned value.
Let us demonstrate the implementation of the PHP default argument value in the example below.
Example:
<?php
function setTime($time = "05:00") {
echo "The time is : $time <br>";
}
setTime();
setTime("05:15");
setTime("05:30");
?>
Output:
Program Explanation:
The example clarifies how the PHP program implements the default argument value.
As seen in the program, it assigns "05:00"
as the default value of the argument $time
.
But the other calling functions such as setTime("05:15");
and setTime("05:30");
has their argument values which override the assigned default value.
PHP Functions – Returning values
PHP’s return keyword effectively terminates a function. Sometimes, this keyword utilizes the result of an argument as the function’s return value.
If the return is applied outside of the function, it will terminate the PHP code file from running.
Example:
<?php
function trySum(int $a, int $b) {
$c = $a + $b;
return $c;
}echo "The sum of 3 and 9 is: " . trySum(3,9) . "<br>";
echo "The sum of 10 and 4 is: " . trySum(10,4) . "<br>";
echo "The sum of 12 and 7 is: " . trySum(12,7);
?>
Output:
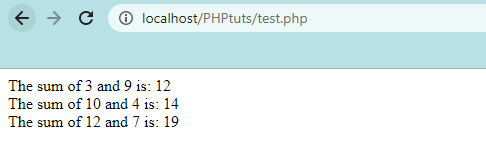
Program Explanation:
This example shows that the return keyword returns the value of the variable $c
it is the sum of the arguments $a
and $b
.
PHP Return Type Declarations
This time, let us use the PHP return for type declarations and the example below will demonstrate how this method works.
Example:
<?php
function addNumbers(float $a, float $b) : float {
return $a + $b;
}
echo addNumbers(12.8, 1.2);
?>
Output:
Program Explanation:
The return statement in PHP 7 also supports Type Declarations. When defining the function, place the type declaration just before the initial curly bracket ({), followed by a colon (:).
Passing Arguments by Reference
Another PHP function is the passing of arguments by reference and in this section, we will have an example of that.
Example:
<?php
function addInteger(&$sum) {
$sum += 5;
}
$int = 11;
addInteger($int);
echo $int;
?>
Output
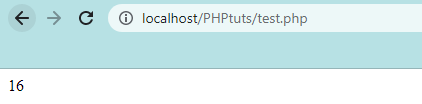
Program Explanation:
The & (ampersand) symbol inserted before the variable parameter is used to pass an argument by reference, implementing a PHP function.
Frequently Ask Questions (FAQs)
What are PHP functions?
A function is a collection of statements that can be utilized several times within a program.
This will not be automatically executed when a webpage loads. A call to a function will execute the function.
What is a PHP function example?
A function is a piece of code that accepts an additional input in the form of a parameter, processes it, and returns a value.
This functionality is a piece of code that can be used more than once, and it takes a list of parameters and returns a value.
What are the types of functions in PHP?
There are two types of functions in the PHP programming language:
- Built-in Functions
- User-defined Functions
What is the function name in PHP?
Function names must follow rules when implemented in the program. The name must start with a letter or an underscore and not with numeral figures.
Related Articles
- PHP Session Function (Start and Destroy)
- PHP Anonymous Functions with Example Programs
- PHP Chmod Dir Function With Examples
- PHP Glob() Function With Examples
- PHP Unlink Function With Example Program
Summary
In summary, this discussion has covered all the details that you need to understand functions in PHP.
It is also provided with advanced examples to demonstrate the different kinds of potential functions that you may find in the language.