The Blackjack Game In Python is a fully functional console based application project that includes all of the components that IT students and computer-related courses will need for their college projects or for leisure time purposes.
This Blackjack Python is a card game in which participants try to get as close to 21 as possible without exceeding it. This Python Blackjack is beneficial for practicing Java development and gaining new skills.
This project is quite useful, and the concept and logic are simple to grasp. The source code is open source and is free to use. Simply scroll down and click the download button.
Table of contents
- Watch These Related Solitaire in JavaScript with Source Code From Start To Finish!
- Blackjack Game In Python : Project Output
- What Is Blackjack Game In Python ?
- Rules Of Playing Blackjack Game In Python
- About the Project : Blackjack Game In Python With Source Code
- Project Details And Technology : Blackjack Game In Python
- The given code below is a python file for main.py
- Blackjack Game In Python : Steps on how to run to run the project
- Download Source Code below
- Summary
- Related Articles
- Inquiries
Watch These Related Solitaire in JavaScript with Source Code From Start To Finish!
Blackjack Game In Python : Project Output
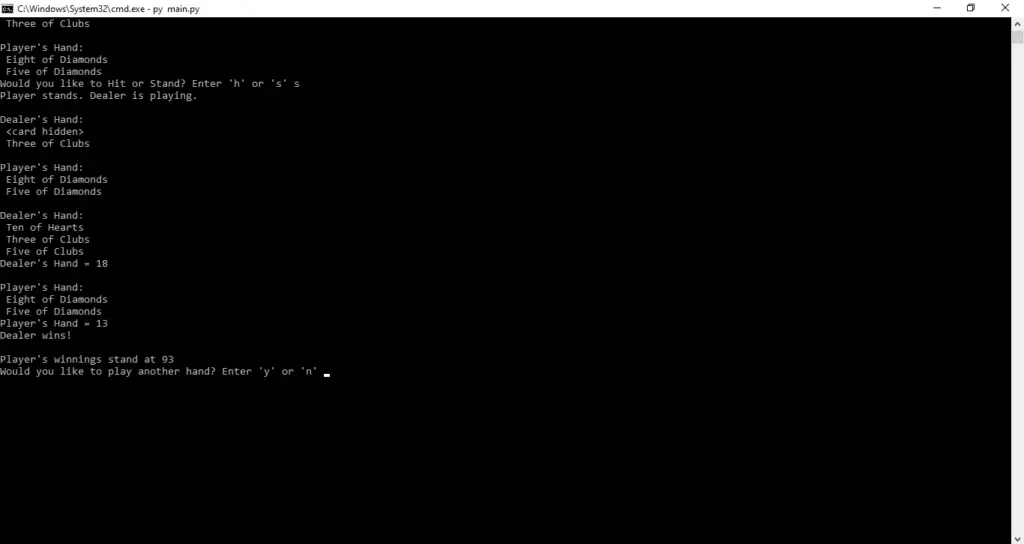
What Is Blackjack Game In Python?
The Python Blackjack Code is a casino card game. The players in this game compete against the casino’s assigned dealer, not each other.
In this post, we’ll create a Blackjack game for a player and a dealer from the ground up, which can be played on a terminal.
Rules Of Playing Blackjack Game In Python
We’ll give you a quick summary of the rules if you’ve never played blackjack before. The magical number in blackjack is 21. When a player’s total card value exceeds 21, the player is considered bust and loses instantly.
If a player receives an exact 21 against the dealer, he or she wins. Otherwise, to win, the sum of the player’s cards must be greater than the sum of the dealer’s cards.
Each face card has a constant value of 10, but depending on the player’s odds of winning, the ace can be counted as one or eleven. The quantity of the remaining cards determines their value.
About the Project : Blackjack Game In Python With Source Code
The Blackjack Program In Java is a simple desktop program built with the programming language Python. With the Python programming language, we can also make very interesting games.
One of these games is blackjack. The project system file has both a Python script and resource files. The game’s graphics are smooth, and it’s easy to figure out how to play.
This Blackjack Game Code includes a tutorial and a code development guide. This is a simple and easy-to-understand learning project.
Since Black Game is open source, you can download the zip file and change it to fit your needs. You can also change this project to fit your needs and make a great advanced-level project out of it.
Project Details And Technology : Blackjack Game In Python
Project Name : | Blackjack Game In Python |
Abstract : | This Blackjack Game In Python is a useful program that IT students can use to get better at writing Python code. This project is simple. You can use this project if you want to have some leisure time. |
Language Used : | Python |
IDE Used : | PyCharm |
Type : | Terminal-Based Application |
Developer : | Glenn Magada Azuelo |
The given code below is a python file for main.py
# WELCOME TO BLACKJACK GAME CODE, IF YOU HAVE NO IDEA WHAT IS A BLACKJACK GAME, PLEASE CHECK IT OUT IN GOOGLE # let's import Random library import random # Let's give the info of the card's suits, ranks and values suits = ('Hearts', 'Diamonds', 'Spades', 'Clubs') ranks = ('Two', 'Three', 'Four', 'Five', 'Six', 'Seven', 'Eight', 'Nine', 'Ten', 'Jack', 'Queen', 'King', 'Ace') values = {'Two':2, 'Three':3, 'Four':4, 'Five':5, 'Six':6, 'Seven':7, 'Eight':8, 'Nine':9, 'Ten':10, 'Jack':10, 'Queen':10, 'King':10, 'Ace':11} playing = True # Following below is the Card Class which will initiate a card with the given suit and rank class Card: def __init__(self,suit,rank): self.suit = suit self.rank = rank def __str__(self): return self.rank + ' of ' + self.suit # Following below is the Deck Class which will create a deck from the given cards class Deck: def __init__(self): self.deck = [] # start with an empty list for suit in suits: for rank in ranks: self.deck.append(Card(suit,rank)) # build Card objects and add them to the list def __str__(self): deck_comp = '' # start with an empty string for card in self.deck: deck_comp += '\n '+card.__str__() # add each Card object's print string return 'The deck has:' + deck_comp def shuffle(self): # shuffle function will shuffle the whole deck random.shuffle(self.deck) def deal(self): # deal function will take one card from the deck single_card = self.deck.pop() return single_card # Following is the Hand Class which will add the cards from deck class to the player's hand class Hand: def __init__(self): self.cards = [] # start with an empty list as we did in the Deck class self.value = 0 # start with zero value self.aces = 0 # add an attribute to keep track of aces # add_card function will add a card to the player's hand def add_card(self,card): self.cards.append(card) self.value += values[card.rank] # since ace can have two values as 1 or 11, adjust_for_ace will adjust the value of ace def adjust_for_ace(self): while self.value > 21 and self.aces: self.value -= 10 self.aces -= 1 ''' In addition to decks of cards and hands, we need to keep track of a Player's starting chips, bets, and ongoing winnings. This could be done using global variables, but in the spirit of object oriented programming, let's make a Chips class instead! ''' class Chips: def __init__(self): self.total = 100 # This can be set to a default value or supplied by a user input self.bet = 0 def win_bet(self): self.total += self.bet def lose_bet(self): self.total -= self.bet # FUNCTIONS HERE: # FUNCTION FOR TAKING BETS def take_bet(chips): while True: try: chips.bet = int(input('How many chips would you like to bet? ')) except ValueError: print('Sorry, a bet must be an integer!') else: if chips.bet > chips.total: print("Sorry, your bet can't exceed",chips.total) else: break # function for taking hits def hit(deck,hand): hand.add_card(deck.deal()) hand.adjust_for_ace() # function prompting the Player to Hit or Stand def hit_or_stand(deck,hand): global playing # to control an upcoming while loop while True: x = input("Would you like to Hit or Stand? Enter 'h' or 's' ") if x[0].lower() == 'h': hit(deck,hand) # hit() function defined above elif x[0].lower() == 's': print("Player stands. Dealer is playing.") playing = False else: print("Sorry, please try again.") continue break # functions to display cards def show_some(player,dealer): print("\nDealer's Hand:") print(" <card hidden>") print('',dealer.cards[1]) print("\nPlayer's Hand:", *player.cards, sep='\n ') def show_all(player,dealer): print("\nDealer's Hand:", *dealer.cards, sep='\n ') print("Dealer's Hand =",dealer.value) print("\nPlayer's Hand:", *player.cards, sep='\n ') print("Player's Hand =",player.value) # functions to handle end of game scenarios def player_busts(player,dealer,chips): print("Player busts!") chips.lose_bet() def player_wins(player,dealer,chips): print("Player wins!") chips.win_bet() def dealer_busts(player,dealer,chips): print("Dealer busts!") chips.win_bet() def dealer_wins(player,dealer,chips): print("Dealer wins!") chips.lose_bet() def push(player,dealer): print("Dealer and Player tie! It's a push.") # AND NOW ON TO THE GAME!!! while True: # Print an opening statement print('Welcome to BlackJack! Get as close to 21 as you can without going over!\n\ Dealer hits until she reaches 17. Aces count as 1 or 11.') # Create & shuffle the deck, deal two cards to each player deck = Deck() deck.shuffle() player_hand = Hand() player_hand.add_card(deck.deal()) player_hand.add_card(deck.deal()) dealer_hand = Hand() dealer_hand.add_card(deck.deal()) dealer_hand.add_card(deck.deal()) # Set up the Player's chips player_chips = Chips() # remember the default value is 100 # Prompt the Player for their bet take_bet(player_chips) # Show cards (but keep one dealer card hidden) show_some(player_hand,dealer_hand) while playing: # recall this variable from our hit_or_stand function # Prompt for Player to Hit or Stand hit_or_stand(deck,player_hand) # Show cards (but keep one dealer card hidden) show_some(player_hand,dealer_hand) # If player's hand exceeds 21, run player_busts() and break out of loop if player_hand.value > 21: player_busts(player_hand,dealer_hand,player_chips) break # If Player hasn't busted, play Dealer's hand until Dealer reaches 17 if player_hand.value <= 21: while dealer_hand.value < 17: hit(deck,dealer_hand) # Show all cards show_all(player_hand,dealer_hand) # Run different winning scenarios if dealer_hand.value > 21: dealer_busts(player_hand,dealer_hand,player_chips) elif dealer_hand.value > player_hand.value: dealer_wins(player_hand,dealer_hand,player_chips) elif dealer_hand.value < player_hand.value: player_wins(player_hand,dealer_hand,player_chips) else: push(player_hand,dealer_hand) # Inform Player of their chips total print("\nPlayer's winnings stand at",player_chips.total) # Ask to play again new_game = input("Would you like to play another hand? Enter 'y' or 'n' ") if new_game[0].lower()=='y': playing=True continue else: print("Thanks for playing!") break # END OF THE PROGRAM!
To start executing this Blackjack Game In Python, make sure you have PyCharm IDE installed in your computer.
By the way if you are new to python programming and you don’t know what would be the the Python IDE to use, I have here a list of Best Python IDE for Windows, Linux, Mac OS that will suit for you. I also have here How to Download and Install Latest Version of Python on Windows.
Blackjack Game In Python : Steps on how to run to run the project
Time needed: 5 minutes
These are the steps on how to run Blackjack Game In Python
- Step 1: Download source code.
First, download the source code given below.
- Step 2: Extract file.
Next, after you finished download the source code, extract the zip file.
- Step 3: Open Project Path and Open CMD (Command Prompt).
In order for you to run the project, you just need to open the project path and type CMD. The first thing you need to do is type py main.py in the command prompt. After that, just wait for a few seconds to load the system.
Download Source Code below
Summary
This Blackjack Game In Python is a quick and easy Python project for beginners and intermediates alike, and it broadens knowledge of Python applications.
Finally, this entire Python project with free source code is an absolute project and a valuable means for users to learn and explore more about it.
Related Articles
- Sales Management System Project in Python with Source Code
- Time Table Management System Project in Python with Source Code
- Cloth Store Management System Project in Python with Source Code
- Pharmacy Management System Project in Python with Source Code
- Fees Management System Project in Python with Source Code
Inquiries
If you have any questions or suggestions about the Blackjack Game In Python With Source Code, please feel free to leave a comment below.