TypeScript Variables
A variable in Typescript serves as a storage spot utilized to hold data or information that can be referred to and utilized by software programs.
Essentially, it serves as a storage space for data values within a program.
The declaration of a variable can be done using the var keyword. Variables can be defined using the let and const keywords.
These variables have a similar syntax for declaration and initialization but differ in scope and usage.
In TypeScript, it is generally recommended to define a variable using the let keyword as it ensures type safety.
The let keyword bears some similarities to the var keyword, and const is a variant of let that prevents a variable from being re-assigned.
The naming conventions for variables in TypeScript, adhere to the same rules as those used in JavaScript variable declarations.
Rules in naming conventions for variables in TypeScript:
- A variable name should consist of alphabets or numerical digits.
- A variable name should not begin with numerical digits.
- A variable name should not include spaces or special characters, with the exception of the underscore (_) and the dollar ($) sign.
Please note that before a variable can be used, it needs to be declared. The var keyword is used for declaring variables.
Variable Declaration in TypeScript
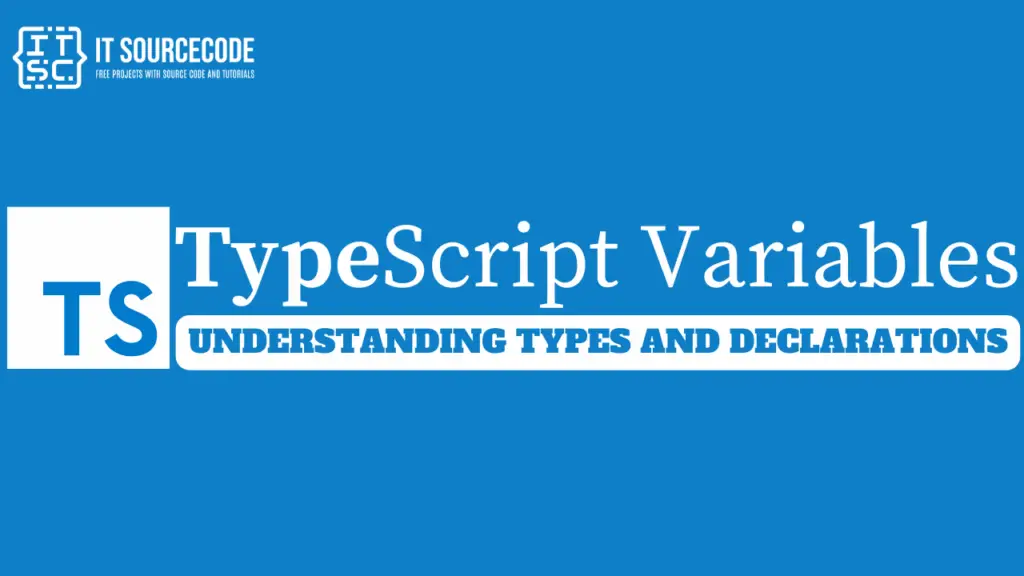
There are three (3) main keywords used for declaring variables in TypeScript:
- var
var is the traditional method that is used in JavaScript. However, it has some peculiarities and scoping rules that can lead to unexpected results.
Variables declared with var are accessible anywhere within their enclosing function, module, namespace, or global scope.
- let
This was introduced to overcome the limitations of var. let offers block-scoping, which means you can declare variables that are limited to specific blocks of code (like loops or conditional statements).
This helps to avoid unintentional variable redeclaration and improves the clarity of the code.
- const
This is similar to let, but const also has block-scoping. The difference is that it prevents re-assignment after initialization.
Once a value is assigned to a const variable, you cannot change it.
Use const for values that you don’t want to change in your program.
Different methods to declare a variable
There are four different methods that we can use to declare a variable:
- Specify both its type and value in a single statement
Syntax:
var [identifier] : [type-annotation] = value; ✅
For example:
var sampleVariable: number = 18;
In our example:
- sampleVariable is the identifier.
- number is the type annotation.
- 18 is the value assigned to sampleVariable.
This statement declares a variable named myVariable, specifies that its type should be a number and assigns it an initial value of 10.
From this point forward, sampleVariable can be used in the code to refer to the number 18.
If you try to assign a value of a different type to sampleVariable (for example, a string), the TypeScript compiler will give an error.
This is because sampleVariable has been explicitly declared to be of type number.
- Specify its type without assigning a value. In this scenario, the variable will default to undefined.
Syntax:
var [identifier] : [type-annotation];
For example:
var sampleVariable: number;
In our example:
- sampleVariable is the identifier.
- number is the type annotation.
This statement declares a variable named sampleVariable and specifies that its type should be number.
However, no value is assigned to sampleVariable at this point, so its initial value is undefined.
- Assign a value without specifying a type. The type of the variable will automatically be determined based on the value assigned to it.
Syntax:
var [identifier] = value;
For example:
var myVariable = 18;
In our example:
- sampleVariable is the identifier.
- 18 is the value assigned to sampleVariable.
This statement declares a variable named sampleVariable and assigns it an initial value of 18. The type of sampleVariable is not explicitly specified, so TypeScript will automatically infer its type based on the assigned value.
In this case, because 18 is a number, TypeScript will infer that sampleVariable is of type number.
- Declare a variable without specifying a type or assigning a value, the variable will default to the any type and will be initialized with a value of undefined.
Syntax:
var [identifier];
For example:
var sampleVariable;
In our example:
- sampleVariable is the identifier.
This statement declares a variable named sampleVariable without specifying a type or assigning a value.
As a result, TypeScript will default sampleVariable to any type and its initial value will be undefined.
Type Annotations
Type Annotations in TypeScript are used to specify the type of a variable, functions, etc.
They tell us what kind of data (like number, string, etc.) these things should hold. We write these labels after the name of the thing we’re labeling. Once we label something, it can only hold that type of data.
If we try to put a different type of data in it, TypeScript will give us an error.
For example:
let website: string = 'Itsourcecode';
let year: number = 2024;
const offer: string = 'Free Source Code and Tutorials';
TypeScript Variable Scope
The visibility of a variable, or where it can be accessed in the program, is determined by its scope.
There are three main scopes for variables in TypeScript:
- Block or Local Scope:
If a variable is declared inside a block of code (like inside a loop or an if-else statement), it can only be accessed within that block.
- Function or Class Scope:
If a variable is declared inside a function or a class, it can only be accessed within that function or class.
- Global Scope:
If a variable is declared outside all functions, classes, or blocks of code, it’s in the global scope. This means it can be accessed from any part of the program.
TypeScript introduces keywords for declaring variables (as I’ve already mentioned above) that help manage scope, which are let and const.
Both of these provide block-scoping, meaning the variables are only accessible within the nearest set of curly braces (function, if-else block, or for-loop).
- let:
This is similar to var, but it’s block-scoped rather than function-scoped.
- const:
This is similar to let, but once a const variable is assigned a value, it can’t be changed.
It’s generally recommended to use let or const instead of var to avoid scope issues.
Conclusion
In conclusion, we’ve learned that variables in TypeScript serve as storage spaces for data values within a program, facilitating data manipulation and reference.
Also, the different methods wherein you can use it to declare a variable.
Variables can be declared using the var, let, or const keywords, each with its own scope and usage nuances.
I hope this article helps you understand the variables in TypeScript and the different methods to declare them.