What is a Typescript?
TypeScript is a high-level programming language that is both free and open-source and was created by Microsoft.
It is a superset of JavaScript, which means any valid JavaScript code is also valid TypeScript code.
However, TypeScript introduces optional static typing and other features that make it a powerful tool for building large-scale applications.
For example
function greet(name: string) {
return `Welcome to, ${name}!`;
}
let message = greet("Itsourcecode");
console.log(message);
Output:
Welcome to Itsourcecode!
For further understanding, let’s take a look at another example:
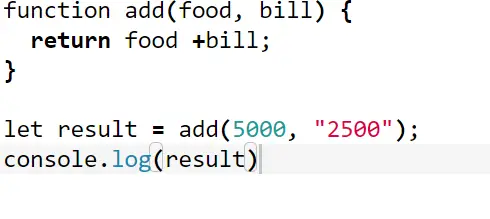
As you can see our given code defines an add function that doesn’t enforce type checking, meaning it’s dynamically typed.
There are no restrictions on the data types of the parameters “food” and “bill”.
Consequently, passing a string instead of a number to this function won’t trigger an error. Instead, it will join the string values, which could lead to unanticipated outcomes.
Output:
50002500
There’s no error but the result is “50002500” instead of 7500
It introduces the concept of optional static typing. This feature gives developers the ability to define the types of variables, function parameters, and return values.
As a result, it’s possible to detect errors related to types during the development process.
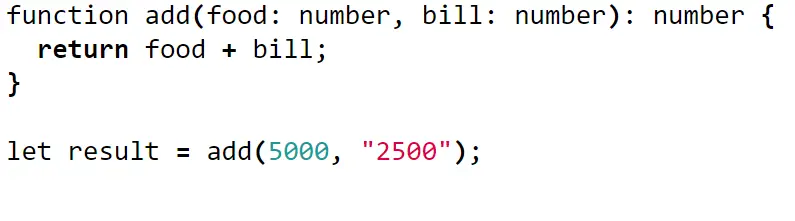
In the provided sample code, the parameters “food” and “bill” are clearly specified as numbers.
If a string is supplied as an argument, TypeScript will trigger a compile-time error:
script.ts(5,24): error TS2345: Argument of type '"2500"' is not assignable to parameter of type 'number'.
This allows for early detection of potential problems.
Components of TypeScript
TypeScript is made up of three main parts:
- Language: This includes syntax, keywords, and type annotations.
- The TypeScript Compiler (TSC): The TSC changes TypeScript code into JavaScript.
- The TypeScript Language Services: This part gives information that helps tools like editors offer better features such as automated refactoring and IntelliSense.
What is in Typescript?
TypeScript is a language that extends JavaScript by adding types. It’s like an upgraded version of JavaScript. It allows you to specify the kind of data each part of your code should handle.
Here are some key features of TypeScript:
Static Typing
It enhances JavaScript by introducing static typing.
This allows developers to define the types of variables, function parameters, and return values.
It helps in catching errors early during the editing process.
For example:
function greet(name: string) {
return `Hello, welcome to${name}!`;
}
console.log(greet("Itsourcecode"));
console.log(greet(123));
Output:
Hello, welcome to Itsourcecode!
Error:
Argument of type 'number' is not assignable to parameter of type 'string'.
Optional Typing
It offers the versatility of opting for static typing or not.
This implies that you have the freedom to define types for variables and function parameters, or you can allow TypeScript to deduce the types on its own, depending on the value given.
For example:
let age: number;
age = 18; // Valid assignment
// age = "18"; // Optional typing allows this, but if uncommented, TypeScript will raise a type error
console.log(age);
Output:
Output: 25
Type Inference
It is designed to understand JavaScript and uses type inference to provide excellent tooling without needing extra code.
For example:
let message = "Hi, Itsourcecode!";
console.log(message.toUpperCase());
TypeScript infers the type of “message” as a string automatically. There’s no need to explicitly specify the type
Output:
HI, ITSOURCECODE!
Compile-Time Type Checking
TypeScript checks if the specified types match before the code is run, not while it’s running. This is known as compile-time type checking.
For example:
function add(x: number, y: number) {
return x + y;
}
console.log(add(50, 30));
// console.log(add("5", 3)); // Error: Argument of type 'string' is not assignable to parameter of type 'number'.
Output:
80
Transpiles to JavaScript
TypeScript code is converted into JavaScript, which can be run anywhere JavaScript is used, like in a browser, on Node.js or Deno, and in your apps.
For example:
function greet(name: string) {
return `Hi, ${name}!`;
}
console.log(greet("Itsourcecode")); // Output: Hi, Itsourcecode!
After compiling using TypeScript compiler (tsc), the above code will be converted to JavaScript:
function greet(name) {
return "Hi, " + name + "!";
}
console.log(greet("Itsourcecode"));
Output:
Hi, Itsourcecode!
Improved Tooling
It offers enhanced tooling at any scale, including features like autocompletion, type checking, and advanced refactoring.
Interfaces and Types
TypeScript lets you define the structure of objects and functions in your code, making it easier to see documentation and issues in your editor.
For example:
interface Person {
name: string;
age: number;
}
function greet(person: Person) {
return `Hi, ${person.name}!`;
}
let user = { name: "Itsourcecode", age: 18};
console.log(greet(user));
Output:
Hi, Itsourcecode!
What is a Typescript File?
A TypeScript file is a special kind of file where you write TypeScript code. It is like JavaScript, but it lets you specify what type of data each piece of your code should be working with.
If you try to use the wrong type of data, it will let you know with an error message.
This helps catch errors right away. These files end with .ts instead of .js which is used for JavaScript files.
After you write your TypeScript code, it gets converted into JavaScript code that can run anywhere JavaScript runs.
This includes web browsers and servers.
What is Typescript used for?
- It is used to add a strong type system to JavaScript, which helps code editors provide features like code refactoring, navigation, and type checking.
- It helps coders avoid bugs that might happen when the code is running.
- TypeScript code is converted to JavaScript, which can run anywhere JavaScript can: in a browser, on Node.js or Deno, and in your apps.
Conclusion
In conclusion, TypeScript is a powerful programming language developed by Microsoft, built as a superset of JavaScript.
It enhances JavaScript with optional static typing and other features, enabling developers to catch errors early during development.
Its key components include language syntax, the TypeScript Compiler (TSC), and TypeScript Language Services.
TypeScript is utilized to add a strong type system to JavaScript, enhancing code editors’ features and aiding in bug prevention.
I am hoping that this article helps you to understand the TypeScript better.