What is an Operator?
An operator in TypeScript is a symbol or keyword that carries out operations on one or more pieces of data.
The data that operators manipulate is known as operands.
As we all know TypeScript is a strongly typed extension of JavaScript, so it encompasses all the conventional operators that JavaScript supports.
Moreover, TypeScript operators can be categorized into three types, depending on the number of operands they work with.
- Unary Operator:
These types of operators only need a single operand to function.
For example:
let a: number = 10
a++
console.log(a)
Please take note that: ++ is an unary operator
Output:
11
- Binary Operator:
These operators require two operands for their operation. Binary operators are the most common operators in TypeScript.
For example:
let x: number = 10,
y: number = 20
let z: number = x + y
console.log(z)
Please note that: + is a binary operator
Output:
30
- Ternary Operator:
These types of operators work with three operands, with the first operand being conditional.
TypeScript includes a ternary operator, represented by the symbol “?”.
let x: number = 10
console.log(x > 10 ? 'greater than 10' : 'less than or equal to 10')
Please note that the above console.log statement will print “greater than 10”.
Ternary Operator is like an if-else block.
For instance if(x>10) console.log(“less than or equal to 10”), else console.log(“greater than 10”).
What are the Different Operators in Typescript?
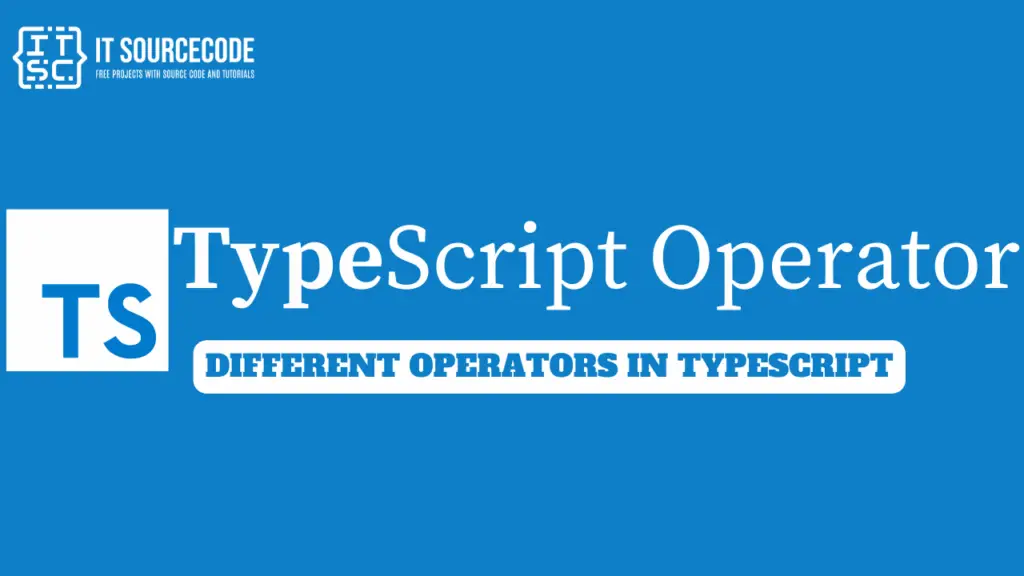
Here are the various types of operators you’ll encounter in TypeScript along with their examples.
1. Arithmetic Operators:
These are used for basic math operations like addition (+), subtraction (-), multiplication (*), division (/), modulus (%), increment (++), and decrement (–).
• Addition (+)
The + (Addition) will return the sum of the operands.
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x + y ✅
console.log(z)
Output:
50
• Subtraction (-)
The subtraction (-) operation will return the difference between the values.
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x + y ✅
console.log(z)
Output:
10
• Multiplication (*)
The Multiplication (*) operation will return the product of the values.
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x * y ✅
console.log(z)
Output:
600
• Division (/)
The Division (/) performs division operation and returns the quotient
Here’s an example:
let x: number = 40,
y: number = 2
let z: number = x / y ✅
console.log(z)
Output:
20
• Modulus (%)
The Modulus (%) operation performs a division operation and returns the remainder.
Here’s an example:
let x: number = 45,
y: number = 2
let z: number = x % y ✅
console.log(z)
Output:
1
• Increment (++)
The increment (++) operation increases the value of the variable by one.
Here’s an example:
let x: number = 10
x++ ✅
console.log(x)
Output:
11
• Decrement (–)
The decrement (++) operation decrements the value of the variable by one.
Here’s an example:
let x: number = 10
x-- ✅
console.log(x)
Output:
9
2. Assignment Operators
Assignment Operators are used to assign values to variables.
There are 6 Assignment operators in TypeScript:
• Simple Assignment (=) operator
The (=) operator designates values from the operand on the right to the operand on the left.
Here’s an example:
let x: number = 10 // = or assign operator
• Add and Assignment (+=) operator
The (+=) operator sums the right operand and the left operand, then assigns the resulting value to the left operand.
Here’s an example:
let x: number = 15,
y: number = 20
y += x // y = y + x ✅
console.log(y)
Output:
35
• Subtract and Assignment (-=) operator
The (-=) operator deducts the right operand from the left operand and then assigns the outcome to the left operand.
Here’s an example:
let x: number = 15,
y: number = 20
y -= x // y = y - x ✅
console.log(y)
Output:
5
• Multiply and Assignment (*=) operator
The (*=) operator multiplies the right operand with the left operand and then assigns the resulting value to the left operand.
Here’s an example:
let x: number = 15,
y: number = 20
y *= x // y = y * x ✅
console.log(y)
Output:
300
• Divide and Assignment (/=) operator
The (/=) operator divides the left operand by the right operand and then assigns the resulting value to the left operand.
Here’s an example:
let x: number = 15,
y: number = 20
y /= x // y = y / x ✅
console.log(y)
Output:
1.3333333333333333
• Modulus and Assignment (%=) operator
The (%=) operator first calculates the modulus of x and y (which is the remainder of the division x / y), and then assigns that result back to x.
If x and y are two variables, the operation x %= y is equivalent to x = x % y.
Here’s an example:
let x: number = 15,
y: number = 20
y %= x // y = y % x ✅
console.log(y)
Output :
5
3. Bitwise Operators
These are operators that are typically used for manipulating bits and performing bitwise operations on one or more operands.
In simple words, these operators work on the binary representations of numbers.
There are seven (7) bitwise operators in TypeScript:
• AND (&) operator
AND (&) operator carries out a Boolean AND operation on each bit of its integer inputs.
Here’s an example:
console.log(4 & 5)
Here’s the explanation:
In binary representation, 4 is 0100 and 5 is 0101. The bitwise AND operation (&) compares each bit of the first operand to the corresponding bit of the second operand.
If both bits are 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0. So, 0100 & 0101 results in 0100, which is 4 in decimal.
Therefore, the output of the console.log(4 & 5) is:
Output:
4
• OR (|) operator
The OR (|) operator carries out a Boolean OR operation on each bit of its integer inputs.
Here’s an example:
console.log(5 | 6)
Output:
5
• XOR (^) operator
The XOR (^) operator carries out a Boolean exclusive OR operation on each bit of its integer arguments.
An exclusive OR implies that either the first operand is true, or the second operand is true, but not both.
Here’s an example:
console.log(4 ^ 5)
Output:
1
Why the output is 1?
The bitwise XOR operation (^) examines each bit of the first value and matches it with the respective bit of the second value.
If the bits are not the same, the corresponding bit in the result is marked as 1.
If not, the matching bit in the result is marked as 0. So, 0100 ^ 0101 results in 0001, which is 1 in decimal.
• NOT (~) operator
The NOT (~) operator is a unary operator that works by flipping or reversing all the bits in the operand.
Here’s an example:
console.log(~4)
As you’ve seen in our previous examples, 4 is 0100 in binary. The bitwise NOT operation (~) flips each bit of its operand.
So, ~0100 results in 1011, which is -5 in decimal (in two’s complement representation).
Therefore, the output is:
-5.
• Right Shift ( >>) operator
The bitwise right shift operator (>>) shifts the bits of the number to the right by a number of bits determined by the right operand.
Here’s an example:
console.log(4 >> 5)
So, 4 >> 5 results in 0000, which is 0 in decimal.
Therefore, the output is:
0
• Left Shift (<<) operator
The bitwise left shift operation (<<) shifts the bits of the number to the left by the specified number of places.
Here’s an example:
console.log(4 << 5)
In other words, moving a value one position to the left is the same as doubling it, while shifting it two positions to the left is like quadrupling it, and so forth.
So, 4 << 5 results in 10000000, which is 128 in decimal.
Therefore, the output is:
128
• Right Shift With Zero ( >>> ) operator
This operator functions similarly to the “>>” operator, with the only difference being that the bits shifted on the left side are consistently zero.
Here’s an example:
console.log(4 >>> 5);
The bitwise right shift with zero operator (>>>) shifts the bits of the number to the right by the specified number of places.
So, 4 >>> 5 results in 0000, which is 0 in decimal.
Therefore, the output is:
0
4. Conditional or Ternary Operator
This operator is utilized to depict a conditional expression. It’s also occasionally known as the ternary operator.
Here’s the syntax:
condition ? value_if_true : value_if_false
Condition – is a boolean expression that is evaluated as true or false.
value_if_true – is the result if the condition is true.
value_if_false – is the result if the condition is false.
This operator initially evaluates the condition. If the condition is true, it returns value_if_true. If the condition is false, it returns value_if_false.
Here’s an example:
let grade = 70;
let type = grade >= 75 ? 'Pass' : 'Fail';
console.log(type);
Output:
Fail
Here’s another example:
let grade = 90;
let type = grade >= 75 ? 'Pass' : 'Fail';
console.log(type);
Output:
Pass
In addition, this operator works with three values and is a shorthand way of writing an if-else statement.
value1 ? value2 : value3
or
if (value1 === True) value2
else value3
Moreover, this operator is useful for shortening if-else statements into one line of code.
It can be used in various scenarios where you need to choose between two values based on a condition.
5. Concatenation Operator
Concatenation Operators are used to join one or more strings and values together in TypeScript.
Plus sign (+) is the most common concatenation operator.
Here’s an example:
let sample: string = 'Hi' + ', welcome to Itsourcecode!' // + or concatenation
console.log(sample)
Output:
Hi, welcome to Itsourcecode!
6. Logical Operators
Logical operators are used to merge multiple conditions into a single expression. Giving a result that is either True or False.
Examples include logical AND (&&), logical OR (||), and logical NOT (!).
• Logical AND (&&) operator
The Logical AND (&&) operator returns true only if both conditions are true.
Here’s an example:
let samplecondition1 = true;
let samplecondition2 = false;
// Using the Logical AND operator
let result = samplecondition1 && samplecondition2; ✅
console.log(result);
In our given example above, the samplecondition2 is false, the result of condition1 && condition2 is false.
Output:
false
On the other hand, if both samplecondition1 and samplecondition2 were true, the result would be true. This is how the Logical AND (&&) operator works.
• Logical OR (||) operator
The Logical OR (||) operator returns true if either one of the conditions is true.
Here’s an example:
let samplecondition1 = true;
let samplecondition2 = false;
// Using the Logical OR operator
let result = samplecondition1 || samplecondition2;
console.log(result);
Output:
true
• Logical NOT (!) operator
The operator gives the opposite outcome of the expression’s result. For instance, if the expression is !(>5)
, it will return false
.
Here’s an example:
let condition = true;
// Using the Logical NOT operator ✅
let result = !condition;
console.log(result);
In one example, the condition is true. The Logical NOT (!) operator returns the inverse of the condition.
Output:
false
So, ! the condition is false. Hence, a false is printed to the console.
If the condition were false,! the condition would be true. This is how the Logical NOT (!) operator works.
7. Relational Operators
Relational operators are used to determine the type of relationship that exists between two entities.
This operator will return a boolean value, meaning they return either true or false.
Examples include: equal to (==), strictly equal to (===), strictly not equal to (!==), not equal to (!=), greater than (>), greater than or equal to (>=), less than (<), and less than or equal to (<=).
• Equal to (==) operator
Here’s an example:
let x: number = 10;
let y: number = 10;
// == is the equal operator
let isEqual: boolean = (x == y);
console.log(isEqual);
Output:
true
• Strictly equal to (===) operator
Here’s an example:
let x: number = 10;
let y: number = 20;
// === is the strict equality operator
let isEqual: boolean = (x === y);
console.log(isEqual);
Output:
false
• Not equal to (!=) operator
Here’s an example:
let x: number = 10;
let y: number = 20;
// != is the not equal operator
let isNotEqual: boolean = (x != y);
console.log(isNotEqual);
Output:
true
• Strictly not equal to (!== ) operator
Here’s an example:
let x: number = 10;
let y: number = 20;
// !== is the strict inequality operator
let isStrictlyNotEqual: boolean = (x !== y);
console.log(isStrictlyNotEqual);
Output:
true
• Greater than (>) operator
Here’s an example:
let x: number = 10;
let y: number = 20;
// > is the greater than operator
let isGreaterThan: boolean = (x > y);
console.log(isGreaterThan);
Outputs:
false
• Greater than or equal to (>=) operator
Here’s an example:
let x: number = 10;
let y: number = 10;
// >= is the greater than or equal to operator
let isGreaterThanOrEqual: boolean = (x >= y);
console.log(isGreaterThanOrEqual);
Output:
true
• Less Than (<) operator
Here’s an example:
let x: number = 20;
let y: number = 10;
// < is the less than operator
let isLessThan: boolean = (x < y);
console.log(isLessThan);
Outputs:
false
• Less than or equal to (<=) operator
Here’s an example:
let x: number = 20;
let y: number = 10;
// <= is the less than or equal to operator
let isLessThanOrEqual: boolean = (x <= y);
console.log(isLessThanOrEqual);
Output:
false
8. Type Operators
Type operators in programming languages are used to determine the data type of a variable or an expression. They return a string that represents the data type.
Type Operators are utilized with objects or aid in handling objects, such as typeof, instanceof, in, or delete, are typically classified under the category of Type operators.
• in operator
Here’s an example:
let car = {
brand: "Toyota",
model: "Camry",
year: 2024
};
// Using the 'in' operator
let hasMakeProperty = "brand" in car;
console.log(hasMakeProperty);
Outputs:
true
• typeof operator
The typeof operator is used to get the data type of a variable or an expression. H
Here’s an example:
let message = "Hi, welcome to Itsourcecode!";
console.log(typeof message);
Outputs:
string
Here’s another example:
let age= 18;
console.log(typeof age);
Outputs:
number
instanceof operator
Here’s an example:
const A: String = new String('Hi, Welcome to Itsourcecode!');
console.log(A instanceof String);
Output:
true
• delete operator
Here’s an example:
// Define an object
let employee = {
name: "ItSourceCode",
age: 18,
Position: "Programmer"
};
console.log(employee);
Output:
{ name: 'ItSourceCode', age: 18, Position: 'Programmer' }
Now, let’s use the delete operator:
// Define an object
let employee = {
name: "ItSourceCode",
age: 18,
Position: "Programmer"
};
// Use the delete operator
delete employee.Position;
console.log(employee);
Output:
{ name: 'ItSourceCode', age: 18 }
9. String Operators
These operators are used with string values.
• Concatenation (+) Operator
As we mentioned earlier this operator is used to combine (concatenate) two or more strings.
Here’s an example:
let greeting = "Hi, welcome to";
let website = "Itsourcecode";
let message = greeting + ", " + website + "!";
console.log(message);
Output:
Hi, welcome to, Itsourcecode!
• Concatenation Assignment (+=) Operator
This operator is used to add a string to the end of an existing string.
Here’s an example:
let message = "Hi, welcome to";
message += ", Itsourcecode!";
console.log(message);
Output:
Hi, welcome to, Itsourcecode!
Conclusion
Finally, we’re done discussing the TypeScript operators.
We’ve learned how they work and seen them in action with examples.
These operators, even though they look simple, are really important. They help us work with data, make choices, and manage our code in TypeScript programs.
I hope this article helps you understand TypeScript operators clearly and gets you excited to try them out in your own coding projects.