Real-Time Drowsiness Detection using OpenCV Python With Source Code
The Real-Time Drowsiness Detection OpenCV Python was developed using Python OpenCV, this Drowsiness Detection is a safety technology that can prevent accidents that are caused by drivers who fall asleep while driving.
In a Drowsiness Detection OpenCV Python project, we will be using OpenCV to gather the images from the webcam and feed them into a Deep Learning model which will classify whether the person’s eyes are ‘Open’ or ‘Closed’.
In this Python OpenCV Project also includes a downloadable Python Project With Source Code for free, just find the downloadable source code below and click to start downloading.
By the way, if you are new to Python programming and don’t know what Python IDE is and its usage. I have here a list of the Best Python IDE for Windows, Linux, and Mac OS that will suit you.
I also have here How to Download and Install the Latest Version of Python on Windows.
To start executing Real-Time Drowsiness Detection OpenCV Python With Source Code, make sure that you have installed Python 3.9 and PyCharm on your computer.
How to run the Real-Time Drowsiness Detection using OpenCV Python? A step-by-step Guide with Source Code
Time needed: 5 minutes
These are the steps on how to run Real-Time Drowsiness Detection OpenCV Python With Source Code
- Step 1: Download the given source code below.
First, download the given source code below and unzip the source code.
- Step 2: Import the project to your PyCharm IDE.
Next, import the source code you’ve downloaded to your PyCharm IDE.
- Step 3: Run the project.
Lastly, run the project with the command “py main.py”
Installed Libraries
from scipy.spatial import distance from imutils import face_utils import imutils import dlib import cv2
Complete Source Code
from scipy.spatial import distance from imutils import face_utils import imutils import dlib import cv2 def eye_aspect_ratio(eye): A = distance.euclidean(eye[1], eye[5]) B = distance.euclidean(eye[2], eye[4]) C = distance.euclidean(eye[0], eye[3]) ear = (A + B) / (2.0 * C) return ear thresh = 0.25 frame_check = 20 detect = dlib.get_frontal_face_detector() predict = dlib.shape_predictor(".\shape_predictor_68_face_landmarks.dat")# Dat file is the crux of the code (lStart, lEnd) = face_utils.FACIAL_LANDMARKS_68_IDXS["left_eye"] (rStart, rEnd) = face_utils.FACIAL_LANDMARKS_68_IDXS["right_eye"] cap=cv2.VideoCapture(0) flag=0 while True: ret, frame=cap.read() frame = imutils.resize(frame, width=450) gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) subjects = detect(gray, 0) for subject in subjects: shape = predict(gray, subject) shape = face_utils.shape_to_np(shape)#converting to NumPy Array leftEye = shape[lStart:lEnd] rightEye = shape[rStart:rEnd] leftEAR = eye_aspect_ratio(leftEye) rightEAR = eye_aspect_ratio(rightEye) ear = (leftEAR + rightEAR) / 2.0 leftEyeHull = cv2.convexHull(leftEye) rightEyeHull = cv2.convexHull(rightEye) cv2.drawContours(frame, [leftEyeHull], -1, (0, 255, 0), 1) cv2.drawContours(frame, [rightEyeHull], -1, (0, 255, 0), 1) if ear < thresh: flag += 1 print (flag) if flag >= frame_check: cv2.putText(frame, "****************ALERT!****************", (10, 30), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 0, 255), 2) cv2.putText(frame, "****************ALERT!****************", (10,325), cv2.FONT_HERSHEY_SIMPLEX, 0.7, (0, 0, 255), 2) #print ("Drowsy") else: flag = 0 cv2.imshow("Frame", frame) key = cv2.waitKey(1) & 0xFF if key == ord("q"): break cv2.destroyAllWindows() cap.stop()
Output:
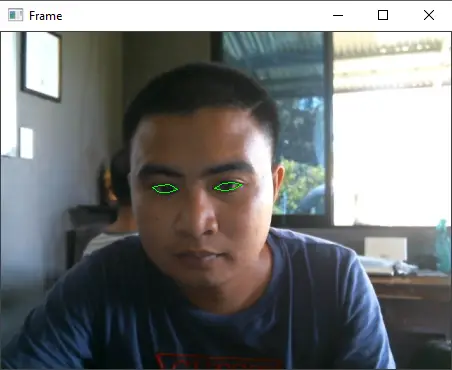
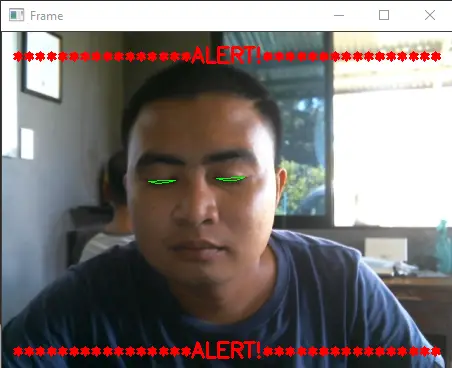
Download the Source Code below
Summary
In this Python project, we have built a drowsy driver alert system that you can implement in numerous ways.
We used OpenCV to detect faces and eyes using a haar cascade classifier and then we used a CNN model to predict the status.
Related Articles
- Code For Game in Python: Python Game Projects With Source Code
- Best Python Projects With Source Code FREE DOWNLOAD
- How to Make a Point of Sale In Python With Source Code
- Python Code For Food Ordering System | FREE DOWNLOAD
- Inventory Management System Project in Python With Source Code
Inquiries
If you have any questions or suggestions about Real-Time Drowsiness Detection OpenCV Python With Source Code, please feel free to leave a comment below.