In this article, we will be creating a file in PHP in the easiest way with a detailed explanation as well as best program examples. This article is a continuation of the previous topic, entitled PHP File to Array.
In PHP, creating a file can simply be done with the use of fopen()
function.
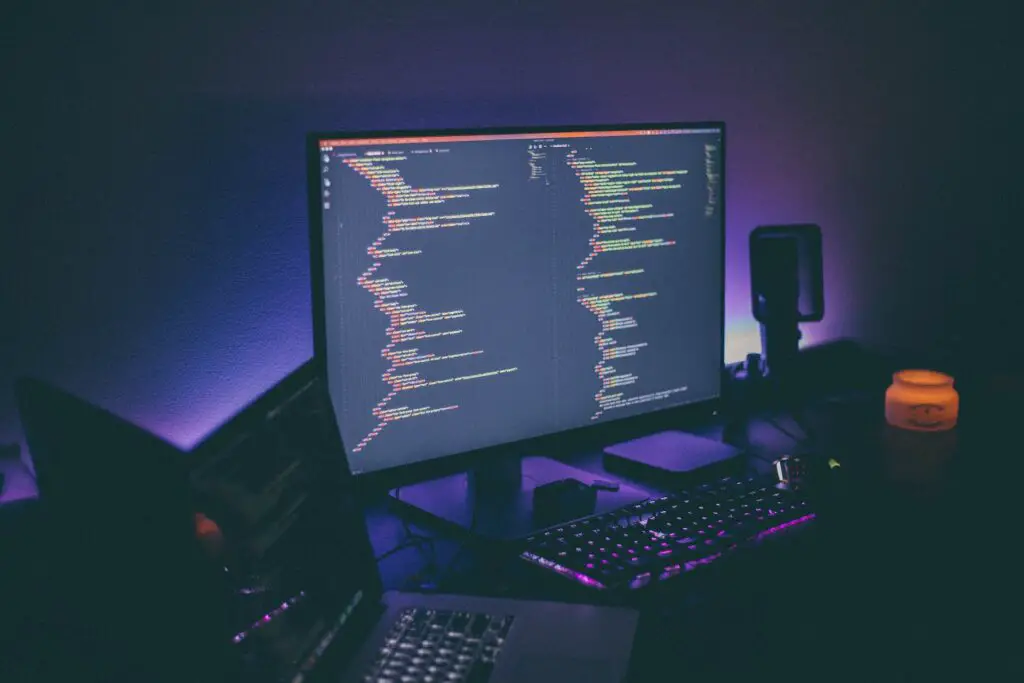
Creating a file using the fopen() function in PHP
The fopen()
is a built-in function which is used for creating a file
. It sounds a little bit confusing, but in PHP (Hypertext Preprocessor). The same function can create a file
and open a files
.
Syntax:
fopen ( string $filename , string $mode , bool $use_include_path = false , resource $context = ? ) : resource
For creating a new file with the use of fopen()
function we need to specify or declared a $filename and one mode.
Modes:
Mode | File Pointer |
---|---|
‘w+’ | At the beginning of the file |
‘a’ | At the end of the file |
‘a+’ | At the end of the file |
‘x’ | At the beginning of the file |
‘x+’ | At the beginning of the file |
‘c’ | At the beginning of the file |
‘c+’ | At the beginning of the file |
TAKE NOTE: If you want to create a (binary file
), you will need to append the character ‘b
‘ to an argument name $mode
. For example, this ‘wb
+’ automatically opens and close the file for writing a binary.
Example:
<?php
// file called
$numbers = [11, 12, 13, 14, 15];
$filename = 'numbers.dat';
$f = fopen($filename, 'wb');
if (!$f) {
die('Error creating the file ' . $filename);
}
foreach ($numbers as $number) {
fputs($f, $number);
}
fclose($f);
?>
The program works in this way.
- First, is to define an array which consists of five numbers from 11 to 15.
- Second, the fopen() and fwrite functions should be used to create a numbers.dat file.
- Third, the fputs() function is used to write each number in the array name $numbers to file.
- Lastly, terminate the file with the use of fclose() function.
Creating a file using the file_put_contents() function in PHP
The file_put_contents() is a function which is used to write data into the existing file.
Syntax:
file_put_contents ( string $filename , mixed $data , int $flags = 0 , resource $context = ? ) : int
TAKE NOTE: Once the file upload is showing results of $filename doesn’t exist, the function successfully creates the file.
In addition, if the function returns file_put_contents()
is identical when calling the fputs()
, fopen()
, and fclose()
functions sequentially to write data in a file.
For example:
<?php
$url = 'https://itsourcecode.com/';
$html = file_get_contents($url);
file_put_contents('home.html', $html);
?>
The program works in this way.
- First, the program will download a webpage
https://itsourcecode.com/
with the use of functionfile_get_contents()
functions. - Lastly, the program will write the HTML (HyperText Markup Language) file using the function
file_put_contents()
.
What does file () do in PHP?
The file() simply works by reading a file into an array. Each element on the array contains a number of lines from the file handling where the newline character is still attached.
How to create a folder and save it in PHP?
In PHP, to create a folder and save, it can simply be done by the function name mkdir()
. This is a built-in function that creates a new folder and directory with a specified pathname.
Furthermore, the path and mode are sent as (parameters) to the function mkdir()
and it will return TRUE if successful otherwise FALSE if fails.
What is file and directory in PHP?
The file and directory
is a set of functions used to retrieve details, update them, and fetch or get information on various system file directories in PHP.
How do I run a PHP file?
The PHP file located inside the (htdocs
) folder, if you want to execute or run the system, you need to open it in any web browser such as Google Chrome, Mozilla Firefox, and Brave and enter the folder name example “localhost/sample. php” and simply click the enter button.
How do I edit a PHP file?
To edit a file you will need to install a text editor such as VS Code
, Sublime Text
and any other PHP code editor online.
What is the file extension of PHP?
The file extension of PHP is (.php
) which need to be put on the end of every file which is similar to a PowerPoint file with a (.ppt) file extension.
Related Articles
- Array Push PHP (With Detailed Explanation)
- Setup PHP Development Environment
- PHP Anonymous Functions with Example Programs
- Session Name PHP With Code Example
- PHP HTML Encode with Example Program
Summary
This article tackle in Creating a file, and also tackles Creating a file using the file_put_contents() function, what does file () does, how to create a folder and save it, what is file and directory, how we run a PHP file, how do I edit a PHP file, and what is the file extension.
I hope this lesson has helped you learn PHP a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.