In this article, we will discuss how PHP remove element from array. With the help of example, it will easily remove an element from an array easily. This article is a continuation of the previous topic, entitled Arrays.
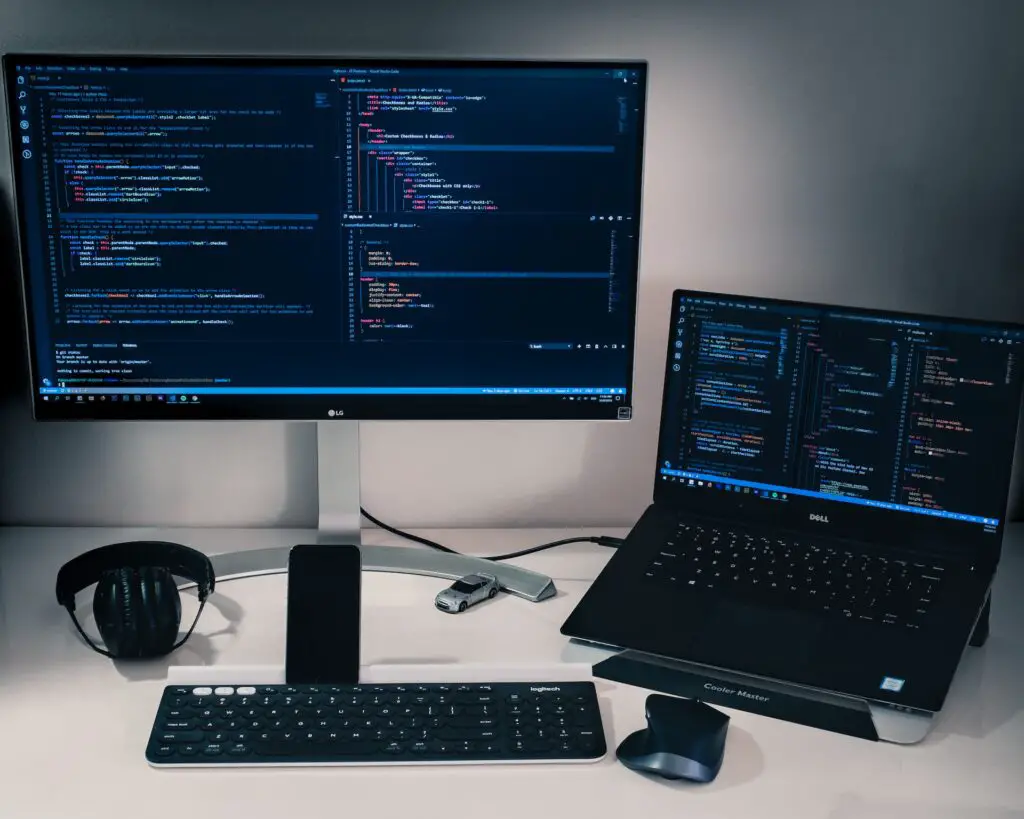
How PHP remove element from array?
In PHP, remove element from array can easily be done by the use of unset()
function which automatically removes an element from array and also with the help of array_values() function which has been indexed automatically in the numerical array.
The following below is the function used to remove an element from array
.
unset() – The unset()
is built-in function in PHP which unsets a variable
that is given. In addition, once the unset()
function is called inside the user-defined function it will automatically unset the local variables.
Syntax:
void unset ( mixed $var [, mixed $... ] )
array_values() – The array_values
is a built-in function in PHP that returns an array that contains all the values of an array.
Syntax:
array array_values ( array $array )
For example:
<?php
// Glenn Magada Azuelo
$arr0ne = array(
'ITSOURCECODE', // [0]
'PIES', // [1]
'DIGITAL' // [2]
);
// remove item at index 1 which is 'for'
unset($arr0ne[1]);
// Print modified array and PHP file
var_dump($arr0ne);
// Re-index the array elements
$arrTwo = array_values($arr0ne);
// Print re-indexed array
var_dump($arrTwo);
?>
Output:
array(2) {
[0]=>
string(12) "ITSOURCECODE"
[2]=>
string(7) "DIGITAL"
}
array(2) {
[0]=>
string(12) "ITSOURCECODE"
[1]=>
string(7) "DIGITAL"
}
What does array_splice() function do give an example?
The array_splice() is a built-in function that removes selected elements from an array and then it will replace them with new elements.
Syntax:
array_splice(array, start, length, array)
Parameter values:
Parameter | Description |
---|---|
array | Required. Specifies an array |
start | Required. Numeric value. Specifies where the function will start removing elements. 0 = the first element. If this value is set to a negative number, the function will start that far from the last element. -2 means start at the second last element of the array search. |
length | Optional. Numeric value. Specifies how many elements will be removed, and also the length of the returned array. If this value is set to a negative number, the function will stop that far from the last element. If this value is not set, the function will remove all elements, starting from the position set by the start parameter. |
array | Optional. Specifies an array with the elements that will be inserted into the original array. If it’s only one element, it can be a string and does not have to be an array. |
For example:
<?php
// Glenn Magada Azuelo
$arrOne = array(
'ITSOURCECODE', // [0]
'PIES', // [1]
'DIGITAL' // [2]
);
// remove item at index 1 which is 'for array search'
array_splice($arrOne, 1, 1);
// Print modified array
var_dump($arrOne);
?>
Output:
array(2) {
[0]=>
string(12) "ITSOURCECODE"
[1]=>
string(7) "DIGITAL"
}
How do you unset multiple values in PHP?
To unset multiple values we will just need to use for loop and foreach loop. But the problem is once we use the foreach loop it will not unset all the variables given and there will be one variable remaining.
for loop example:
for($i=0 ; $i<count($array) ; $i++)
{
unset($array[$i]);
}
foreach loop example:
foreach($array as $arr)
{
unset($array[$arr]);
}
Or use this advanced code:
unset($foo1, $foo2, $foo3);
How to remove last element from an array in PHP?
The array_pop() is a built-in function that deletes the last element of a given result array.
Syntax:
array_pop(array)
Parameter values:
Parameter | Description |
---|---|
array | Required. Specifies an array |
For example:
<?php
$name = array("Adones","Glenn","Jude");
array_pop($name);
print_r($name);
?>
Output:
Array
(
[0] => Adones
[1] => Glenn
)
PHP remove element from array by key
The unset() function is also used to remove elements from array
by key
. This function is used to any variable that needs to destroy and in the same way it is also used to delete any element of the array offset.
In addition, this unset()
command will take the array key as the input and that element will be removed from the multiple arrays.
Syntax:
unset($variable)
Example program to delete an element from one-dimensional array:
<?php
// Glenn Magada Azuelo
// PHP program to delete an array
// element based on index
// Declare arr variable
// and initialize array 0
$name = array('G', 'L', 'E', 'N', 'N');
// Display the array element
print_r($name);
// Use unset() function delete
// elements
unset($name[2]);
// Display the array element
print_r($name);
?>
Output:
Array ( [0] => G [1] => L [2] => E [3] => N [4] => N ) Array ( [0] => G [1] => L [3] => N [4] => N )
Related Articles
- PHP Remove Element From Array With Examples
- Array Push PHP (With Detailed Explanation)
- PHP array_merge With Detailed Explanation
- Session Timer PHP With Detailed Explanation
- PHP Array Iterator with Example Program
Summary
This article discusses on how to Remove Element From Array
, and also tackles what does array_splice()
function do give, how do you unset multiple values, how to remove the last element from an array, and remove an element from an array by key.
I hope this lesson has helped you learn PHP a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.