In this article, we will learn how to convert PHP File to Array with an advanced program example which can quickly solve this issue. This article is a continuation of the previous topic, entitled PHP File Upload.
In PHP, File to Array is a very simple problem with a simple solution we just only need to understand how file and array work.
There are some instances that we need to convert text files to an array in our program which can be helpful to all web developers.
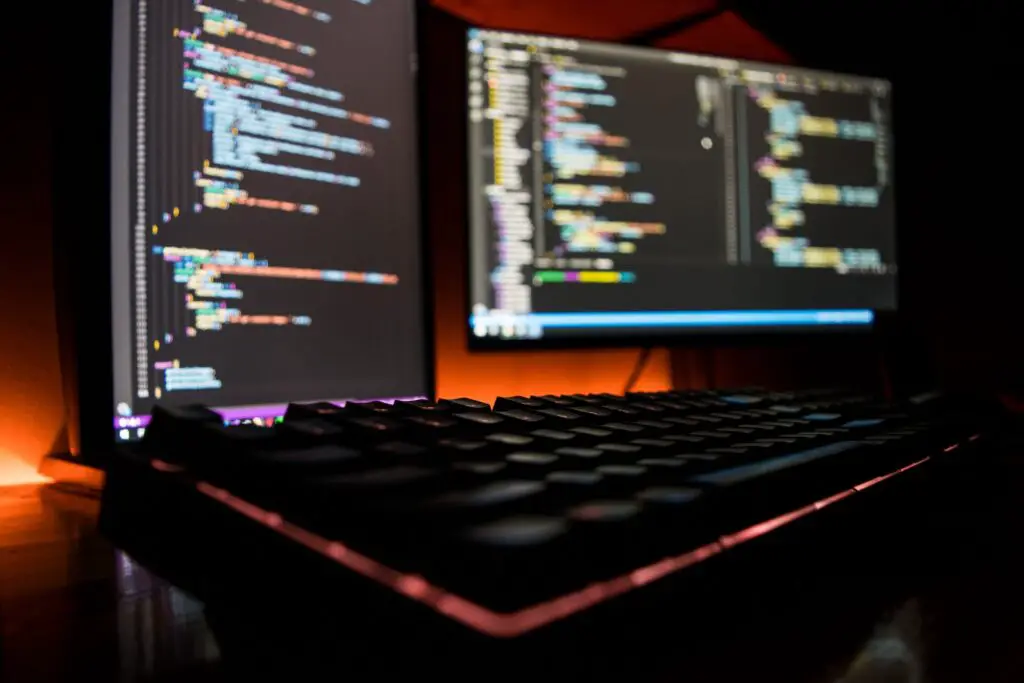
How to Convert File into Array in PHP?
The following program below is a complete and more advanced program that can solve how to convert File to Array using PHP.
index.php
<?php
$file="array.txt";
$fopen = fopen($file, 'r');
$fread = fread($fopen,filesize($file));
fclose($fopen);
$remove = "\n";
$split = explode($remove, $fread);
$array[] = null;
$tab = "\t";
foreach ($split as $string)
{
$row = explode($tab, $string);
array_push($array,$row);
}
echo "<pre>";
print_r($array);
echo "</pre>";
?>
array.txt
The names "John Doe" for males, "Jane Doe" or "Jane Roe" for females, or "Jonnie Doe" and "Janie Doe" for children, or just "Doe" non-gender-specifically, are used as placeholder names for a party whose true identity is unknown or must be withheld in a legal action, case, or discussion.
The names are also used to refer to a corpse or hospital patient whose identity is unknown. This practice is widely used in the United States and Canada but is rarely used in other English-speaking countries, including the United Kingdom itself, from where the use of "John Doe" in a legal context originates. The names Joe Bloggs or John Smith are used in the UK instead, as well as in Australia and New Zealand.
John Doe is sometimes used to refer to a typical male in other contexts as well, in a similar manner to John Q. Public, known in Great Britain as Joe Public, John Smith, or Joe Bloggs. For example, the first name listed on a form is often John Doe, along with a fictional address or other fictional information to provide an example of how to fill in the form.
The name is also frequently used in popular culture, for example in the Frank Capra film Meet John Doe. John Doe was also the name of a 2002 American television series.
Similarly, a child or baby whose identity is unknown may be referred to as Baby Doe. A notorious murder case in Kansas City, Missouri, referred to the baby victim as "Precious Doe. The other unidentified female murder victims are Cali Doe and Princess Doe.
Additional people may be called James Doe, Judy Doe, etc. However, to avoid possible confusion, if two anonymous or unknown parties are cited in a specific case or action, the surnames Doe and Roe may be used simultaneously; for example, "John Doe v. Jane Roe." If several anonymous parties are referenced, they may simply be labelled John Doe #1, John Doe #2, etc. (the U.S.
Operation Delego cited 21 (numbered) "John Doe"s) or labelled with other variants of Doe/Roe/Poe/etc. Other early alternatives, such as John Stiles and Richard Miles, are now rarely used, and Mary Major has been used in some American federal cases.
Output:
Array
(
[0] =>
[1] => Array
(
[0] => The names "John Doe" for males, "Jane Doe" or "Jane Roe" for females, or "Jonnie Doe" and "Janie Doe" for children, or just "Doe" non-gender-specifically, are used as placeholder names for a party whose true identity is unknown or must be withheld in a legal action, case, or discussion.
)
[2] => Array
(
[0] =>
)
[3] => Array
(
[0] => The names are also used to refer to a corpse or hospital patient whose identity is unknown. This practice is widely used in the United States and Canada but is rarely used in other English-speaking countries, including the United Kingdom itself, from where the use of "John Doe" in a legal context originates. The names Joe Bloggs or John Smith are used in the UK instead, as well as in Australia and New Zealand.
)
[4] => Array
(
[0] =>
)
[5] => Array
(
[0] => John Doe is sometimes used to refer to a typical male in other contexts as well, in a similar manner to John Q. Public, known in Great Britain as Joe Public, John Smith, or Joe Bloggs. For example, the first name listed on a form is often John Doe, along with a fictional address or other fictional information to provide an example of how to fill in the form.
)
[6] => Array
(
[0] =>
)
[7] => Array
(
[0] => The name is also frequently used in popular culture, for example in the Frank Capra film Meet John Doe. John Doe was also the name of a 2002 American television series.
)
[8] => Array
(
[0] =>
)
[9] => Array
(
[0] => Similarly, a child or baby whose identity is unknown may be referred to as Baby Doe. A notorious murder case in Kansas City, Missouri, referred to the baby victim as "Precious Doe. The other unidentified female murder victims are Cali Doe and Princess Doe.
)
[10] => Array
(
[0] =>
)
[11] => Array
(
[0] => Additional people may be called James Doe, Judy Doe, etc. However, to avoid possible confusion, if two anonymous or unknown parties are cited in a specific case or action, the surnames Doe and Roe may be used simultaneously; for example, "John Doe v. Jane Roe." If several anonymous parties are referenced, they may simply be labelled John Doe #1, John Doe #2, etc. (the U.S.
)
[12] => Array
(
[0] =>
)
[13] => Array
(
[0] =>
)
[14] => Array
(
[0] => Operation Delego cited 21 (numbered) "John Doe"s) or labelled with other variants of Doe/Roe/Poe/etc. Other early alternatives, such as John Stiles and Richard Miles, are now rarely used, and Mary Major has been used in some American federal cases.
)
)
How to read File into an Array In PHP?
In PHP, to read file into an array is very simple you just need to use the file()
function. In addition, do not try to attempt to read large files with this function.
For example:
<?php
// (A) FILE TO ARRAY
$array = file("array.txt");
print_r($array);
// (B) ADDITIONAL OPTION - SKIP EMPTY LINES
$array = file("array.txt", FILE_SKIP_EMPTY_LINES);
print_r($array);
?>
How to read file line by line in PHP?
To read the file line by line we can use the fgets()
function to easily read it and those not called on the file will ignore each new line.
For example:
<?php
// OPEN FILE
$handle = fopen("array.txt", "r") or die("Error reading file!");
// READ LINE BY LINE
while (($line = fgets($handle)) !== false) {
// To better manage the memory, you can also specify how many bytes to read at once
// while (($line = fgets($handle, 4096)) !== false) {
echo $line;
}
// (C) CLOSE FILE
fclose($handle);
Output:
Hello I'm Glenn Magada Azuelo
How to read file into a string in PHP?
The file_get_contents() is a function that allows you to fetch contents from the URL or a file. Always take note that doesn’t ever try to read massive files or text or else you will run out of memory which can caused an error and possibly causes some technical issues to the server.
For example:
<?php
// (A) READ ENTIRE CONTENTS INTO A STRING
$text = file_get_contents("array.txt");
echo $text;
// (B) ALSO CAN FETCH FROM URL
//$text = file_get_contents("https://itsourcecode.com");
//echo $text;
Output:
Hello I'm Glenn Magada Azuelo
How do I read the contents of a file in PHP?
To read the contents of a file into a string you just need to use the file_get_contents()
function. This is a preferred function that can be easily used to read the contents of (file
) into the string.
More About Array
- PHP array_merge With Detailed Explanation
- PHP Array Iterator with Example Program
- PHP Array Search With Advanced Example
- PHP Arrays (With Advanced Program Examples)
- PHP Remove Element From Array With Examples
Summary
This article discusses how to convert File to Array, and also tackles how to read File into an Array, how to read a file handle line by line, how to read the file into a string, and how do I read the contents of a file.
I hope this lesson has helped you learn PHP a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.