How To Read Data From Text Files in Python With Source Code
Reading Text files in Python is one of the most common tasks that you can do with Python, In this tutorial I will teach you How To Read A Text File In Python.
A simple tutorial Read Text File In Python is mainly for beginner to intermediate Pythonistas, but there are some tips here that more advanced programmers may appreciate as well.
This tutorial also includes the downloadable Read Data From Text File In Python with source code for free.
To start creating this simple tutorial on How To Read Data From Text files in Python, make sure that you have PyCharm IDE installed on your computer.
By the way, if you are new to Python programming and don’t know what Python IDE to use, I have here a list of the Best Python IDE for Windows, Linux, and Mac OS that will suit you.
I also have here How to Download and Install the Latest Version of Python on Windows.
How To Read Data From Text Files In Python: Project Information
Project Name: | How To Read Data From Text File In Python |
Language/s Used: | Python Console Based |
Python version (Recommended): | 2.x or 3.x |
Database: | None |
Type: | Python App |
Developer: | IT SOURCECODE |
Updates: | 0 |
How To Read Data From Text Files in Python With Source Code: A step-by-step Guide
How To Read Data From Text Files in Python With Source Code
- Step 1: Create a project name.
First open Pycharm IDE and then create a “project name” After creating a project name click the “create” button.
- Step 2: Create a python file.
Second, after creating a project name, “right click” your project name and then click “new.” After that click the “python file“.
- Step 3: Name your python file.
Third, after creating a Python file, Name your Python file after that click “enter“.
- Step 4: The actual code.
You are free to copy the code given below and download the full source code below.
The code given below is for importing the module
from tkinter import *
This module imports the tkinter for the Graphical User Interface (GUI).
The code given below is for the design.
root = Tk()
root.title("Read Text File In Python")
width=450
height=350
screen_width=root.winfo_screenwidth()
screen_height=root.winfo_screenheight()
x=(screen_width/2) - (width/2)
y=(screen_height/2) - (height/2)
root.geometry("%dx%d+%d+%d" % (width, height, x, y))
root.resizable(0, 0)
This module is the design of this project.
The code given below is for the declaration of the variable.
FILE = StringVar()
This module which is declaring of the variable FILE to be called in public.
The code given below is for reading the data in the text file
def Read_File():
if FILE.get() == "":
lbl_status.config(fg="Orange", text="Please enter something")
for widget in BottomPane.winfo_children():
widget.destroy()
else:
for widget in BottomPane.winfo_children():
widget.destroy()
file = open(FILE.get() + ".txt", "r")
chars = [line.rstrip('\n') for line in file]
for i in range(len(chars)):
exec ('Label%d=Label(BottomPane,text="%s")\nLabel%d.pack(anchor=W)' % (i, chars[i], i))
file.close()
This module reads the data in a text file and displays it in the Tkinter design.
Complete Source Code
from tkinter import *
#==================================MAIN FRAME=====================================
root = Tk()
root.title("Read Text File In Python")
width=450
height=350
screen_width=root.winfo_screenwidth()
screen_height=root.winfo_screenheight()
x=(screen_width/2) - (width/2)
y=(screen_height/2) - (height/2)
root.geometry("%dx%d+%d+%d" % (width, height, x, y))
root.resizable(0, 0)
#=================================VARIABLES=======================================
FILE = StringVar()
#=================================METHODS=========================================
def Read_File():
if FILE.get() == "":
lbl_status.config(fg="Orange", text="Please enter something")
for widget in BottomPane.winfo_children():
widget.destroy()
else:
for widget in BottomPane.winfo_children():
widget.destroy()
file = open(FILE.get() + ".txt", "r")
chars = [line.rstrip('\n') for line in file]
for i in range(len(chars)):
exec ('Label%d=Label(BottomPane,text="%s")\nLabel%d.pack(anchor=W)' % (i, chars[i], i))
file.close()
#=================================FRAMES==========================================
Top = Frame(root, relief=SOLID, bd=1)
Top.pack(fill=X)
Mid = Frame(root)
Mid.pack(pady=20)
Left = Frame(Mid)
Left.pack(side=LEFT)
Right = Frame(Mid)
Right.pack(side=RIGHT)
BelowMid = Frame(root)
BelowMid.pack()
Bottom = Frame(root)
Bottom.pack()
BottomPane = Frame(root)
BottomPane.pack()
#=================================LABEL WIDGETS===================================
lbl_title = Label(Top, text="Read File Text", font=('Courier new', 16))
lbl_title.pack()
lbl_txt = Label(Left, text="Enter a text file", font=('Courier new', 15))
lbl_txt.pack()
lbl_txt2 = Label(Bottom, text="Result", font=('Courier new', 15))
lbl_txt2.pack()
lbl_status = Label(Bottom,font=('Courier new', 12))
lbl_status.pack()
#=================================ENTRY WIDGETS===================================
txt_file = Entry(Right, font=('Courier new', 15),fg='blue', textvariable=FILE)
txt_file.pack()
#=================================BUTTON WIDGETS==================================
btn_check = Button(BelowMid, text="Read File",bg='green', command=Read_File)
btn_check.pack(pady=10)
#=================================INITIALIZATION==================================
if __name__ == '__main__':
root.mainloop()
Output
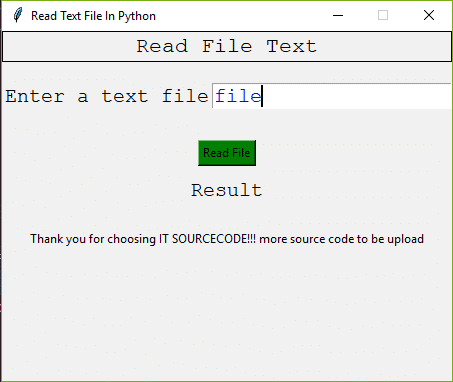
Question
Before we can go into how to work with files in Python, it’s important to understand what exactly a file is and how modern operating systems handle some of their aspects.
At its core, a file is a contiguous set of bytes used to store data. This data is organized in a specific format and can be anything as simple as a text file or as complicated as a program executable. In the end, these byte files are then translated into binary 1
and 0
for easier processing by the computer.
Downloadable Source Code
I have here the list of Best Python Project with Source code free to download for free, I hope this can help you a lot.
Summary
Reading Text File In Python is one of the most common tasks that you can do with Python, This tutorial is written in Python programming language.
Python is very easy to research the syntax emphasizes readability and it is able to reduce time ingesting in development. Also this tutorial is the simplest way for beginners or students to enhance their logical skills in programming.
The project file contains the Python script(index.py) and the text file (file.txt).
Related Articles
- Best Python Project with Source code free to download
- Hotel Management System Project in Python With Source Code
- Student Management System Project in Python with Source Code
- How To Make A Point Of Sale System In Python
- Best Python Projects for Beginners
Inquiries
If you have any questions or suggestions about How To Read Data From Text files in Python, please feel free to leave a comment below.