This article about Color Game Using TKinter In Python is a GUI based which displays random color text and you have to identify its color.
You have 30 seconds to guess as much as word color you can, and you will get score if the guess is right. This project is an interesting and simple project.
Project Information’s
Project Name: | Color Game In Python |
Language/s Used: | Python (GUI) Based |
Python version (Recommended): | 2.x or 3.x |
Database: | None |
Type: | Python App |
Developer: | IT SOURCECODE |
Updates: | 0 |
A Color Game in Python is designed for guessing random word color. Here, you have to type the color of the words, not the word text.
Also, the design of this system is pretty simple so that the user won’t get any difficulties while working on it.
How To Make a Color Game in Python?
Here’s the step by step process on how to create a color game using python.
1. Install Libraries
Code:
from tkinter import *
import random
Explanation:
The code given above are the required libraries to be install in the system.
2. Colors Data
Code:
colours = ['Red','Blue','Green','Pink','Black', 'Yellow','Orange','White','Purple','Brown']
Explanation
The code given above are the list of colors to be guess.
3. Game Starter Function
Code:
#Function that will start the Game
def startGame(event):
if time==30:
#start the countdown timer
countdown()
#run the function to chose the next color
nextcolor()
Explanation:
The code given above is the functions to start the color game.
4. Next Color Function
Code:
def nextcolor():
global score
global time
#if a game is in play
if time > 0:
#make the text entry box active
colour_entry.focus_set()
if colour_entry.get().lower() == colours[1].lower():
score += 1
#clear the entry the box
colour_entry.delete(0, END)
random.shuffle(colours)
# change the colour to type, by changing the
# text _and_ the colour to a random colour value
colour.config(fg= str(colours[1]) , text = str(colours[0]))
# update the score.
scoreLabel.config(text = "Score: " + str(score))
Explanation:
The code given above is the function to give the next guessing color if the first color was guess correctly.
5. Countdown Timer Function
Code:
#Countdown Timer Fuction
def countdown():
global time
#if a game is in play
if time > 0 :
#decrement the value
time -= 1
# update the time left label
timeLabel.config(text = "Time left: "+ str(time))
# run the function again after 1 second.
timeLabel.after(1000, countdown)
Explanation:
The code given above is the function for the countdown timer.
6. Main Function
Code:
#Driver Code
if __name__=='__main__':
root = Tk()
#Setting the title
root.title('Color Game')
#Setting the geometry of the window
root.geometry('375x200')
#set an instruction label
instructions = Label(root, text = 'Type in the colour of the words, and not the word text!', font = ('Helvetica', 12))
instructions.pack()
#Create a Score label
scoreLabel = Label(root, text = 'Score :'+str(score), font=('Helvetica' , 12))
scoreLabel.pack()
#Create a Time Label
timeLabel = Label(root, text = 'Time Left : '+str(time), font=('Helvetica' , 12))
timeLabel.pack()
#create a colour label
colour = Label(root, font=('Helevetica',12))
colour.pack()
#Entry box for input from user
colour_entry = Entry(root)
colour_entry.focus_set()
root.bind('<Return>',startGame)
colour_entry.pack()
root.mainloop()
7. Complete Source Code
from tkinter import *
import random
#list of possible colour.
colours = ['Red','Blue','Green','Pink','Black',
'Yellow','Orange','White','Purple','Brown']
score = 0
#To take in account the time left: initially 30 seconds
time = 30
#Function that will start the Game
def startGame(event):
if time==30:
#start the countdown timer
countdown()
#run the function to chose the next color
nextcolor()
def nextcolor():
global score
global time
#if a game is in play
if time > 0:
#make the text entry box active
colour_entry.focus_set()
if colour_entry.get().lower() == colours[1].lower():
score += 1
#clear the entry the box
colour_entry.delete(0, END)
random.shuffle(colours)
# change the colour to type, by changing the
# text _and_ the colour to a random colour value
colour.config(fg= str(colours[1]) , text = str(colours[0]))
# update the score.
scoreLabel.config(text = "Score: " + str(score))
#Countdown Timer Fuction
def countdown():
global time
#if a game is in play
if time > 0 :
#decrement the value
time -= 1
# update the time left label
timeLabel.config(text = "Time left: "+ str(time))
# run the function again after 1 second.
timeLabel.after(1000, countdown)
#Driver Code
if __name__=='__main__':
root = Tk()
#Setting the title
root.title('Color Game')
#Setting the geometry of the window
root.geometry('375x200')
#set an instruction label
instructions = Label(root, text = 'Type in the colour of the words, and not the word text!', font = ('Helvetica', 12))
instructions.pack()
#Create a Score label
scoreLabel = Label(root, text = 'Score :'+str(score), font=('Helvetica' , 12))
scoreLabel.pack()
#Create a Time Label
timeLabel = Label(root, text = 'Time Left : '+str(time), font=('Helvetica' , 12))
timeLabel.pack()
#create a colour label
colour = Label(root, font=('Helevetica',12))
colour.pack()
#Entry box for input from user
colour_entry = Entry(root)
colour_entry.focus_set()
root.bind('<Return>',startGame)
colour_entry.pack()
root.mainloop()
Output
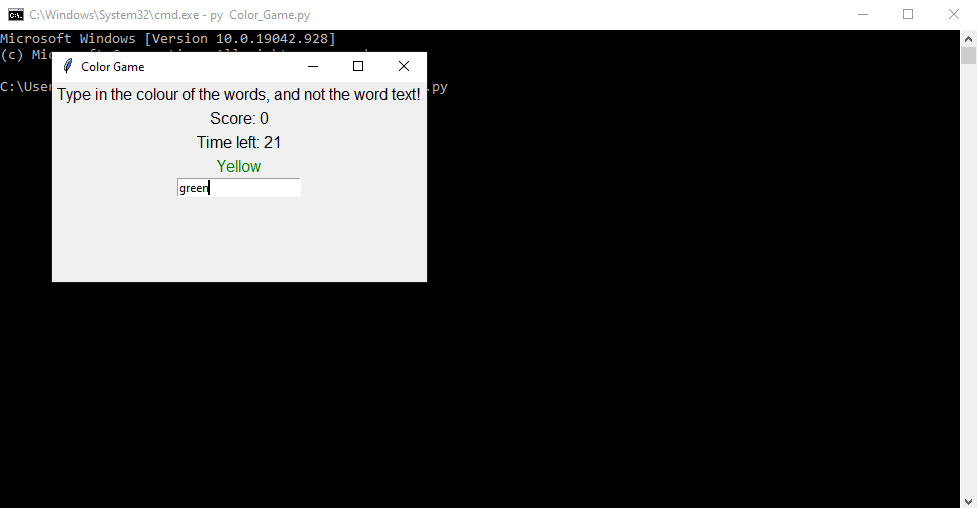
This Color Code Game In Python also includes a downloadable Project With Source Code for free, just find the downloadable source code below and click to start downloading.
By the way if you are new to python programming and you don’t know what would be the the Python IDE to use, I have here a list of Best Python IDE for Windows, Linux, Mac OS that will suit for you. I also have here How to Download and Install Latest Version of Python on Windows.
To start executing Color Game Using Tkinter In Python, make sure that you have installed Python 3.9 and PyCharm in your computer.
Color Game Using Tkinter In Python With Source Code : Steps on how to run the game project
Time needed: 5 minutes
These are the steps on how to run Color Game Using Tkinter In Python With Source Code
- Step 1: Download the given source code below.
First, download the given source code below and unzip the source code.
- Step 2: Import the project to your PyCharm IDE.
Next, import the source code you’ve download to your PyCharm IDE.
- Step 3: Run the project.
last, run the project with the command “py main.py”
Download Source Code below
Summary
The Color Game In Python is a simple project developed using Python. This project is a GUI based which displays random color text and you have to identify its color.
You have 30 seconds to guess as much as word color you can, and you will get score if the guess is right. This project is an interesting and simple project.
Inquiries
If you have any questions or suggestions about Color Game Using Tkinter In Python With Source Code, please feel free to leave a comment below.
why i can beat the color game? it always dodge…why? 1 out of 10 every time i guessed i only got 1 correctly.
guess the color not the name. okay?
every time i played i always loose consecutively…. is it im possible to win on this game?