In this chapter, dynamic memory management in C is explained.
Numerous memory management and allocation functions are available in the C programming language.
This includes stdlib.h header file contains these functions.
SR. No & Function | Description |
---|---|
1. void *calloc(int num, int size); | The size in bytes of each element in the array that this method allocates will be num. |
2. void free(void *address); | The address-specific memory block for which this function is called is released. |
3. void *malloc(size_t size); | With no initialization, this function allocates an array of num bytes. |
4. void *realloc(void *address, int newsize); | With the help of this function, memory is expanded to newsize. |
You must test the C Language code provided in this lesson in your code editor to validate it.
We provide a free C Online Compiler for testing your C Language code.
Introduction of C memory management
Every programming language makes use of the system’s memory.
Every program needs some memory to store its code and data, as well as some temporary memory.
As a result, memory management must be done with extreme caution.
Different sorts of variables and memory needs will be present in a program. The variables that will be used by various functions and blocks throughout the program are known as global variables.
As a result, they are appropriate for allocating memory while the program is being run.
Therefore, these heap memories are used for dynamic memory allocations.
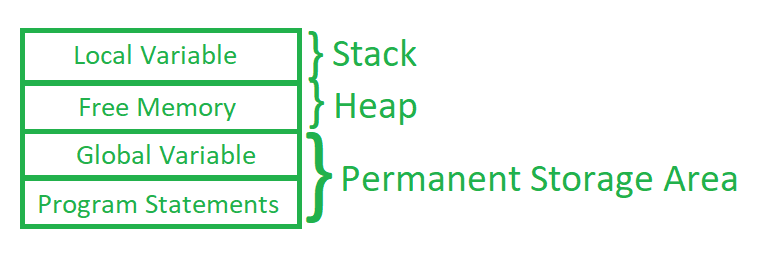
How does memory management work in c?
In C, you can allocate memory with malloc.
The application utilizes malloc’s pointer to access the memory.
When the memory is no longer needed, the pointer is delivered to free to free it up.
C Memory Management Allocation Static
Let’s say we have to add two integers and show the result. Here, we are aware of the number and type of variables used in the calculations.
To store two numbers, we require two integer variables, and the result is stored in an integer variable.
Static memory allocation is the term used to describe this type of variable memory allocation.
Here, memory for the variables doesn’t need to be explicitly allocated.
They don’t have to be straightforward variables; assuming we know their size, they can also be arrays or structures.
Example Code:
int a; int b = 0; const int c = 10;
In each of the examples mentioned above, the compiler is aware of their size and that they are also integers.
As a result, the compiler will allot particular memory regions before running the application.
Allocating Memory in dynamic memory management in c
This is opposed to static memory allocation, where the program knows the amount and type of the variable ahead of time.
Only at the point of execution can it be determined. In this scenario, we are unable to allocate any memory during compilation.
It can only be assigned at run time. When programming, it is simple to define something as an array if you are aware of the size.
char lastname[100];
Let’s say you need to store a lot of text, but you’re not sure how much text there will be.
In this case, you’ll need to use a data structure that can store variable-length text.
Without specifying how much memory is needed at this compile time, we must define a pointer to a character.
Later, based on the need, we can allocate memory as demonstrated in the example below.
#include <stdio.h> #include <stdlib.h> #include <string.h> int main() { char complete_name[100]; char *des; strcpy(complete_name, "Juan Delacruz"); /* allocate memory dynamically */ des = malloc( 200 * sizeof(char) ); if( des == NULL ) { fprintf(stderr, "Error - unable to allocate required memory\n"); } else { strcpy( des, "Juan Delacruz a highschool student in class 4th"); } printf("Name = %s\n", complete_name ); printf("Description: %s\n", des ); }
The result of compiling and running the example code is as follows:
Name = Juan Delacruz
Description: Juan Delacruz a highschool student in class 4th
You can test the above example here! ➡ C Online Compiler
There are various functions to change the size of the memory for the variables and allocate memory to them at runtime.
Pointers, structures, and arrays are the best illustrations of dynamic memory allocation.
Types of Variables in Usage
Memory Allocation | Description |
---|---|
Static Memory Allocation | When variables are declared, they are allocated. The size of the variables is known in advance by the compiler. In a program, allocated memory is fixed and does not change. When the program is finished, allocated memory is released. Statically assigned variables are typically local, static, and global. |
Dynamic Memory | Run-time memory allocation takes place. Before running the program, the compiler is unable to establish the size and type of the variable. Throughout the application, allocated memory may increase, decrease, or stay the same. The software does not necessarily need to use the same address for allocated memory. If the memory is not used, we must explicitly release it in the program. used most frequently with pointers, arrays, and structures. |
malloc() | The beginning of the allotted memory address is pointed to by this function, which also allocates the requested amount of memory in bytes. The variable’s void pointer is what is returned. To determine the variable’s datatype, typecasting is required when memory is being allocated. Since the value is not initialized, it starts out with trash data. The whole amount of memory that must be allocated must be passed. |
calloc() | This function allots a given amount of memory, measured in bytes, and identifies the start of the designated memory address. It gives back the variable’s void pointer. To determine the variable’s datatype, we must typecast while allocating memory. When memory is allocated, the value is initialized to zero. The overall amount of data that must be saved, as well as the size of each individual data, must be passed. |
realloc() | Reassigning the memory to the previously allocated variable will result from this. It has the ability to alter the amount of memory allotted. This will allot memory at a completely different location than the one before. |
free () | The variable’s memory is released as a result. |
malloc()
The most typical run-time memory allocation technique is this one.
This function returns a null pointer to the starting memory address and allows runtime memory to the variables.
This means it doesn’t define the variable’s datatype and only allocates RAM in bytes.
Malloc’s default syntax for allocating memory is:
A pointer variable called ptr in this case allocates 10 bytes of memory.
Here, the variable’s datatype has not been specified, therefore ptr is currently a void pointer.
It will now point to the memory space’s very first byte.
If we store data in an integer variable, each value will take up 4 bytes.
(cast_type *) malloc (size_in_bytes); ptr = malloc(45); // allocates 45 bytes of memory
Additionally, we can specify the quantity of integer-sized data values that must be stored.
ptr = (int*)malloc(10);
The total amount of RAM that will be allocated will be specified in the char malloc argument.
But we can’t always determine the total size.
In order to calculate the overall size of the memory to be allocated, we use the malloc standard library.
Let’s say we want to store 100 elements in a floating-point dynamic array.
Then:
ptr = (int*)malloc(10* sizeof(int));
The total amount of RAM that will be allocated will be specified in the malloc argument.
But we can’t always determine the total size.
In order to calculate the overall size of the memory to be allocated, we use the malloc library.
Let’s say we want to store 100 elements in a floating-point dynamic array.
Then:
arr = (float*)malloc(10 * sizeof(float));
Memory can be allocated for the structures using the same manner. Let’s say there is a student hierarchy.
Then:
struct student *std = (struct student *)malloc(sizeof(struct student));
Here, dynamic memory is allocated for the structure pointer *std and include stdio.h.
It gets the memory allocated for one student and changes the pointer to point to the first student structure member.
Let’s say that the same *std could accommodate 50 pupils. Then, we must set aside RAM to store 50 * sizeof char (student).
struct student *std = (struct student *)malloc(50 * sizeof(struct student));
calloc()
Malloc and this function are comparable.
However memory allocation for arrays and structures is typically done using this function.
When memory is allocated with calloc ().
However, in Calloc(), we pass 50 and student size as two arguments, as is illustrated below.
Other than that, it allocates memory in a manner similar to malloc sizeof int.
(cast_type *) calloc (blocks , size_of_block); struct teacher *std = (struct teacher *)calloc(sizeof(struct student)); struct teacher *std = (struct teacher *)malloc(50, sizeof(struct student));
realloc()
Let’s say we need to change the memory size of a variable that has already been allocated.
In this situation, we can redefine the variable’s memory size using the realloc function.
(cast_type *) realloc (blocks, size_of_block);
It will allot a whole new block of RAM with a new block of memory size.
free()
Once the memory has been allocated, it is always a good idea to release it.
So, let the processor determine integer and pointer memory.
Conclusion
Every programming language deals with memory in the system.
Every variable needs a specified amount of memory, and programs require memory to store themselves.
C provides 2 methods of allocating memory to the variables and programs.
In static memory compile time memory allocation remains constant throughout the program.
Dynamic memory allocation optimizes memory consumption.
About Next Tutorial
In my next post, I’ll create a detailed explanation of Command Line Arguments in C.
This post is about Paging Technique of Memory Management in C Language.
I explain the language’s core grammar with examples. I hope you find it interesting and useful.
PREVIOUS
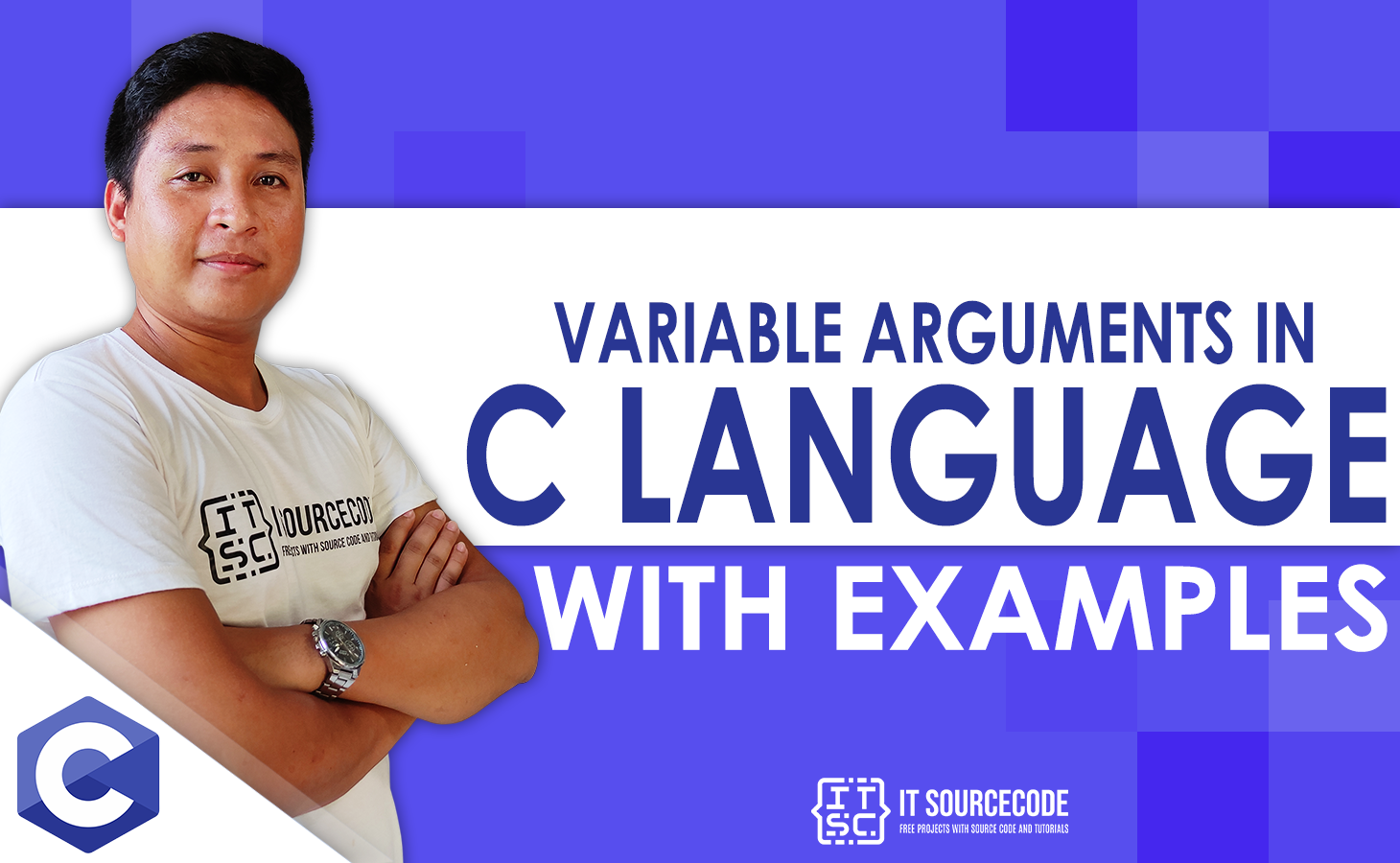
Variable Arguments in C Language