The following declaration and initialization produce a string containing the word “Hello.”
The character array holding the string must be one larger than the number of characters in the word “Hello” to accommodate the null character after the array.
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
Following the array initialization rule, you can write the following statement.
char greeting[] = "Hello";
The memory representation of the above-described string in C/C++ is as follows:
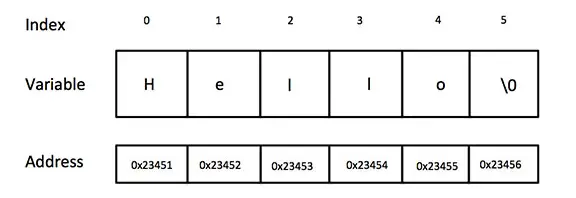
In reality, the null character does not belong at the end of a string constant.
When the C compiler initializes the array, it places the ‘0’ at the end of the string. Let’s see whether we can print the above-mentioned string.
What is String in C Language?
The C Strings are one-dimensional arrays of characters with the null character ‘0’ at the end.
The characters that make up the string are followed by a null in a null-terminated string.
What is the best way to declare a string?
You can declare strings in the following way:
char s[5];
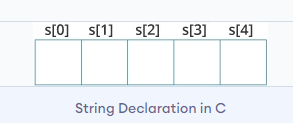
What is the best way to start a string?
Strings can be initialized in a variety of ways.
char c[] = "abcd";
char c[50] = "abcd";
char c[] = {'a', 'b', 'c', 'd', '\0'};
char c[5] = {'a', 'b', 'c', 'd', '\0'};
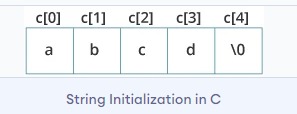
Assigning Values to Strings
In C, arrays and strings are second-class citizens; once declared, they do not support the assignment operator.
As an example:
char c[100];
c = "C programming"; // Error! array type is not assignable.
Read String from the user
To read a string, use the scanf() function.
The scanf() function examines a string of characters until it comes to a whitespace character (space, newline, tab, etc.).
Example of scanf() to read a string
#include <stdio.h>
int main()
{
char name[20];
printf("Enter first name: ");
scanf("%s", name);
printf("Your first name is %s.", name);
return 0;
}
When the example code is compiled and run, the following result is obtained:
Enter first name: Romeo
Your first name is Romeo.
Even though Romeo was typed into the application, the name string simply included “Romeo”.
It’s because following Romeo, there was a blank area.
Also, instead of using &name with scanf, we utilized the code name ().
scanf("%s", name);
Because the name is a char array, we know that in C, array names decay to pointers.
As a result, we don’t need to use & because the name in scanf() already points to the location of the first element in the string.
You can test the above example here! ➡ C Online Compiler
How do you read a single line of text?
To read a line of string, use the fgets() function.
You may also use puts() to show the string.
Example of fgets() and puts()
#include <stdio.h>
int main()
{
char name[30];
printf("Enter first name: ");
fgets(name, sizeof(name), stdin); // read string
printf("First Name: ");
puts(name); // display string
return 0;
}
When the example code is compiled and run, the following output is in the image below
Enter first name: romeo mulita
First Name: romeo mulita
To read a string from the user, we utilized the fgets() function. / read string fgets(name, sizeof(name), stdlin);
The result of sizeof(name) is 30. As a result, we can only accept 30 characters as input, which is the length of the name string.
We used puts(name); to output the string.
Note that the gets() function can also be used to receive user input. However, it is no longer included in the C standard.
It’s because gets() allows you to provide any character length. As a result, a buffer overflow could occur.
You can test the above example here! ➡ C Online Compiler
Passing Strings to Functions
Strings are supplied to functions in the same manner as arrays are.
Find out more about how to pass arrays to a function.
Example of Passing String to a Function
#include <stdio.h>
void displayString(char str[]);
int main()
{
char str[50];
printf("Enter string: ");
fgets(str, sizeof(str), stdin);
displayString(str); // Passing string to a function.
return 0;
}
void displayString(char str[])
{
printf("String Output: ");
puts(str);
}
When the example code is compiled and run, the following output is in the image below:
Enter string: romeo
String Output: romeo
You can test the above example here! ➡ C Online Compiler
Strings and Pointers
String names are “decayed” to pointers in the same way as arrays are. As a result, you can alter string elements with pointers.
Before proceeding with this example, we recommend that you review C Arrays and Pointers.
Example of Strings and Pointers
#include <stdio.h>
int main(void) {
char fullname[] = "Adones Evanz";
printf("%c", *fullname); // Output: A
printf("%c", *(fullname+1)); // Output: d
printf("%c", *(fullname+7)); // Output: e
char *namePtr;
namePtr = fullname;
printf("%c", *namePtr); // Output: A
printf("%c", *(namePtr+1)); // Output: d
printf("%c", *(namePtr+7)); // Output: e
}
Output of the above example:
AdEAdE
You can test the above example here! ➡ C Online Compiler
Frequently used String Functions:
- strlen() is a function that determines the length of a string.
- strcpy() is a function that copies a string to another.
- strcmp() is a function that compares two strings.
- strcat() is a function that joins two strings together.
C Strings Functions
You’ll learn how to manipulate strings in C using library functions like gets(), puts(), strlen(), and more in this article.
You’ll learn how to acquire a string from a user and then manipulate it. You must frequently alter strings in order to solve a problem.
String manipulation can be done manually most of the time, if not all of the time, but this makes programming complex and huge.
To address this, the standard library “string.h” in C includes a significant number of string handling functions.
The following are a few regularly used string handling functions:
No. | Function | Purpose |
---|---|---|
1 | strcpy(s1, s2); | Copies string s2 into string s1. |
2 | strcat(s1, s2); | Concatenates string s2 onto the end of string s1. |
3 | strlen(s1); | Returns the length of string s1. |
4 | strcmp(s1, s2); | Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | strchr(s1, ch); | Returns a pointer to the first occurrence of character ch in string s1. |
6 | strstr(s1, s2); | Returns a pointer to the first occurrence of string s2 in string s1. |
The “string.h” header file defines the string handling functions.
#include <string.h>
To run string handling functions, you must include the code below.
gets() and puts()
As described in the last chapter, the functions gets() and puts() are two string functions that take string input from the user and display it.
Example of gets() and puts():
#include<stdio.h>
int main()
{
char name[30];
printf("Enter First Name: ");
gets(name); //Function to read string from user.
printf("First Name: ");
puts(name); //Function to display string.
return 0;
}
When the example code is compiled and run, the following output is in the below:
Enter First Name:romeo
First Name: romeo
You can test the above example here! ➡ C Online Compiler
C Strings Examples
Finding the Frequency of Characters in a String in C. You will learn how to find the frequency of a character in a string in this example.
To follow along with this example, you should be familiar with the following C programming concepts:
- C Arrays
- C Programming Strings
Calculate a Character’s Frequency
#include <stdio.h>
int main() {
char str[1000], ch;
int count = 0;
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
printf("Enter a character to find its frequency: ");
scanf("%c", &ch);
for (int i = 0; str[i] != '\0'; ++i) {
if (ch == str[i])
++count;
}
printf("Frequency of %c = %d", ch, count);
return 0;
}
When the example code is compiled and run, the following output is in the below:
Enter a string:source code in itsourcecode is free to download
Enter a character to find its frequency: Frequency of o = 7
The string entered by the user is saved in str in this program.
The user is then prompted to enter the character whose frequency is being sought. This is saved in the ch variable.
Note: This application is case-sensitive, which means it distinguishes between uppercase and lowercase versions of the same alphabet.
You can test the above example here! ➡ C Online Compiler
Concatenation of two strings in C
You will learn how to manually concatenate two strings without using the strcat() function in this example.
To follow along with this example, you should be familiar with the following C programming concepts:
- Arrays in C
- C Programming Strings
- C for Loop
The strcat() method is the finest technique to concatenate two strings in C programming, as you may know.
In this example, however, we shall manually concatenate two strings.
Example of Concatenate Two Strings Without Using strcat()
#include <stdio.h>
int main() {
char s1[100] = "In Itsourcecode", s2[] = "programming is no longer a problem";
int length, j;
// store length of s1 in the length variable
length = 0;
while (s1[length] != '\0') {
++length;
}
// concatenate s2 to s1
for (j = 0; s2[j] != '\0'; ++j, ++length) {
s1[length] = s2[j];
}
// terminating the s1 string
s1[length] = '\0';
printf("After concatenation: ");
puts(s1);
return 0;
}
When the preceding code is compiled and run, the following output is in the below:
After concatenation: In Itsourcecodeprogramming is no longer a problem
Two strings, s1 and s2, are concatenated in this case, and the result is saved in s1.
It’s vital to remember that s1 must be long enough to hold the string after concatenation.
If you don’t, you can receive surprising results.
You can test the above example here! ➡ C Online Compiler
Removes all characters except alphabets from a string in C
You will learn how to remove all characters from a string entered by the user except the alphabet in this example.
To follow along with this example, you should be familiar with the following C programming concepts:
- Arrays in C
- Strings in C Programming
- C stands for loop.
- Loop C while and do…while
Remove Characters in String Except Alphabets examples:
#include <stdio.h>
int main() {
char line[150];
printf("Enter a string: ");
fgets(line, sizeof(line), stdin); // take input
for (int i = 0, j; line[i] != '\0'; ++i) {
// enter the loop if the character is not an alphabet
// and not the null character
while (!(line[i] >= 'a' && line[i] <= 'z') && !(line[i] >= 'A' && line[i] <= 'Z') && !(line[i] == '\0')) {
for (j = i; line[j] != '\0'; ++j) {
// if jth element of line is not an alphabet,
// assign the value of (j+1)th element to the jth element
line[j] = line[j + 1];
}
line[j] = '\0';
}
}
printf("Output String: ");
puts(line);
return 0;
}
When the example code is compiled and run, the following output is in the below:
Enter a string:itsourcecode.com
Output String: itsourcecodecom
Summary
Strings are one-dimensional arrays of characters with the null character ‘0’ at the end. Declaration and initialization produce a string that contains the word “Hello”.
The character array holding the string must be one larger than the number of characters in the word.
If you have any questions or suggestions about C – Strings and String Functions with Examples, please feel free to leave a comment below.
About Next Tutorial
In the following post, I’ll create Structures in C and try to explain its many components in full detail. I hope you enjoy this post on C – Strings and String Functions with Examples, in which I attempt to illustrate the language’s core grammar.
PREVIOUS
NEXT