Command Line Arguments in C: We can observe that the main function received no arguments.
The C programming language allows the programmer to add parameters or arguments inside the main function to shorten the code.
This tutorial’s topics include:
- Arguments in a command line
- Command arguments’ components
- Understanding the command line with a C program
What are Command Arguments in C?
The command arguments in C are supplied by the user to the program at the time of execution.
We previously utilized the main() routines without any arguments.
Command Line Arguments C: Components
Typically, 2 parameters are supplied into the main function to implement command arguments:
- The quantity of command-line parameters
- The command line parameters list
The basic syntax for single argument separated is:
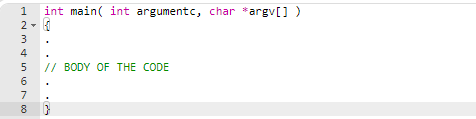
Using command arguments separated in the following various ways:
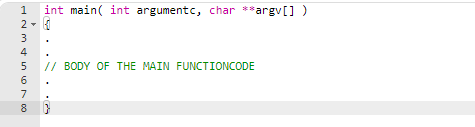
Let’s now go into greater detail about the parts of this code:
- argumentc – It stands for “argc argument count.” The number of command line parameters are stored in the first parameter. It’s critical to remember that argv is a null pointer and to value must be bigger than or equal to 0.
- argv argument vector – It stands for “argument vector.” To list all the command line options, we essentially utilize an array of character pointers.
Command Arguments in C Example Program
Here is some C code that shows how to use the C command line argument.
#include<stdio.h> int main(int argc, char** argv) { printf("Welcome to Command Arguments tutorials!\n\n"); int x; printf("The number of arguments are: %d\n",argc); printf("The arguments are:"); for ( x = 0; x < argc; x++) { printf("%s\n", argv[x]); } return 0; }
Welcome to Command Arguments tutorials!
The number of arguments are: 1
The arguments are:./jdoodle
You can test the above example here! ➡ C Online Compiler
How to use command arguments in C?
- argv 0 if argc outputs the program’s name.
- The first argument that the user provides is printed by argv[1].
- The pointer argv[argc] is null.
- Supplied to the main() method without fail.
- The user passes arguments to the command line from the terminal.
- It is applied to external program control.
C Command Line Argument Usage
- So that we can use shell to chain commands, it permits the usage of standard input and output.
- It is utilized to make it easier to install and run straightforward apps.
Summary
We will learn about command arguments in c and how to use them in this course.
We also spoke about how function pointers operate.
Additionally, we demonstrated how to number of arguments passed.
For any coder, command line arguments in c are incredibly useful.
You can pass data from your operating system’s terminal using double quotes or single quotes command arguments.
PREVIOUS