Arrays in C are a particular sort of data structure called an array that can hold a fixed-size sequential collection of identical-type elements.
It is crucial to think of an array as a collection of variables of the same type, even though it is used to store a collection of data.
You define one array variable, such as numbers, and use numbers[0], numbers[1], and…, numbers[99] to represent individual variables rather than specifying individual variables, such as number 0, number1,…, and number99.
An index is used to reach a specific element in an array. Contiguous memory addresses make up all arrays.
The first element has the lowest address, and the last piece has the highest address.
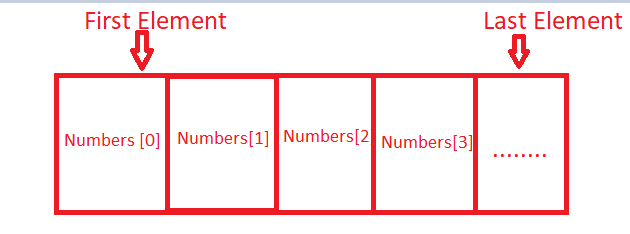
What are arrays in C Programming Language?
The definition of an array on C is a way to group several items of the same type.
These things or things can have data types like int, float, char, double, or user-defined data types like structures.
Single-dimensional and multidimensional arrays are the two types of arrays available in C.
Single-Dimensional Arrays
The simplest type of array in C is the single-dimensional array, sometimes known as a 1-D array.
This kind of array has members with comparable kinds, and its indices can be used to access those elements.
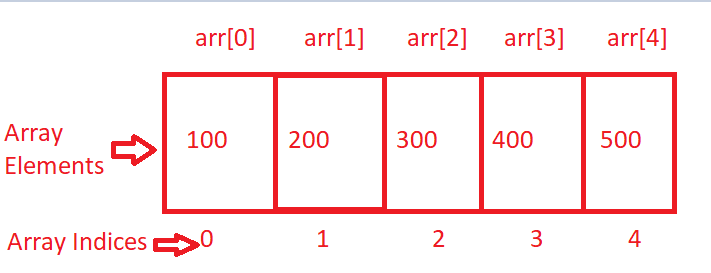
Multi-dimensional Arrays
A 2-D array is the most prevalent sort of multi-dimensional array used in the C programming language.
However, depending on the user’s system’s compiler, there may be more than two dimensions.
These arrays are made up of elements that are arrays.
Why Do We Need Arrays?
If there are only a few components, such as three variables, we can define them individually as var1, var2, and var3.
However, if we need to store a lot of variables, we can utilize arrays.
Array in Details in C
Array in Details in C is important because they are crucial to C, arrays demand a lot more attention.
A C programmer should understand the following crucial array principles.
Concept | Description |
---|---|
Multi-dimensional arrays | Multidimensional arrays are supported in C. The two-dimensional array is the most basic type of multidimensional array. |
Passing arrays to functions | By giving the function the array’s name without an index, you can send it a pointer to the array. |
Return array from a function | A function may return an array C. |
Pointer to an array | With just the array name and no index, you can create a pointer to the first element of an array. |
Declaration and Initialization of Arrays C
The declaration and initialization of an array on C can be done in a variety of ways.
Any data type (such as an int, float, double, or char) may be declared as an array C.
An array C can be declared and initialized in the ways shown below.
Array Declaration by Specifying the Size in C
Declaring an array involves stating its size or its element count. The maximum number of elements that an array can contain is specified by its size.
For Example:
// declare an array by specifying size in []. int my_array1[100]; char my_array2[45]; // declare an array by specifying user defined size. int size = 100; int my_array3[size];
When an array is created but no values are assigned to it, it maintains a trash value.
Like any uninitialized variable, any uninitialized array value will return a trash value if you access it.
Array Declaration by Initializing Elements
When an array is declared, it can be initialized.
The compiler will allocate an array with the same size as the number of array elements when using this way of array declaration.
An array can be simultaneously declared and initialized using the following syntax.
// initialize an array at the time of declaration. int my_array[] = {100, 200, 300, 400, 500}
Although the array size has not been given in the above syntax, the compiler will allocate a size of 5 integer elements to the formed array of 5.
Array Declaration by Specifying the Size and Initializing Elements in C
Another way to create an array is to declare it, set its size, and then assign its components.
The prior approach of creating arrays is not the same as this one.
// declare an array by specifying size and // initializing at the time of declaration int my_array1[5] = {100, 200, 300, 400, 500}; // my_array1 = {100, 200, 300, 400, 500} // int my_array2[5] = {100, 200, 300}; // my_array2 = {100, 200, 300, 0, 0}
My array1 is a 5-element array with all five items populated in the array syntax above.
My array2 has a 5-element array, however, only three of its entries have been initialized.
The compiler will initialize the final two entries of the second array to 0.
Array Initialization Using a Loop in C
Array Initialization Using a Loop in C can also be used to initialize an array.
Beginning with 0, the loop accesses all array indices by iterating from 0 to (size – 1).
The array elements are initialized using a “for loop” with the following syntax.
// declare an array. int my_array[5]; // initialize array using a "for" loop. int i; for(i = 0; i < 5; i++) { my_array[i] = 2 * i; } // my_array = {0, 2, 4, 6, 8}
An array of size 5 is specified first in the syntax above.
A for loop is then used to initialize the array, iterating through the elements of the array from index 0 to (size – 1).
Access Array Elements in C
Access Array Elements in C by indexing the array name, an element can be obtained.
After the array’s name, do this by adding square brackets around the element’s index.
For example:
double salary = balance[20];
The preceding sentence will choose the array’s tenth element and assign its value to the salary variable.
The example that follows demonstrates the usage of all three of the aforementioned ideas, namely declaration, assignment, and accessing arrays.
Advantages of C Array
Arrays are extremely important. While programming, they offer the programmers a number of benefits.
Among them are:
- Because we can keep numerous elements in an array at once and avoid having to write or initialize them repeatedly, arrays help to optimize and clean up the code.
- A single loop can be used to iterate through every element in an array.
- Sorting is significantly simpler using arrays. You can sort elements by writing a few lines of code.
- Any array element can be retrieved in O(1) time in any direction, from the front or the back.
- In an array, elements can be added or removed with linear complexity.
Disadvantages of Array on C
There are drawbacks to everything that is advantageous. The same is valid for arrays.
The array C has a few drawbacks, some of which are listed below:
- Accessing an array improperly: Their primary drawback is that arrays are statically allocated. This implies that their size cannot be changed once it has been initialized. Take into account the following example to better grasp this point:
Summary
In conclusion, you have learned about the C Program in array notion in this tutorial.
You began with a brief overview of the array data structure before gradually moving on to talk about the use, benefits, and drawbacks of arrays.
The various techniques to declare and initialize arrays in C were then covered.
About Next Tutorial
In the next post, I’ll create a Pointers in C and try to explain its many components in full detail.
I hope you enjoy this post on Array in C, in which I attempt to illustrate the language’s core grammar.
PREVIOUS
NEXT