Volume Control With Hand Detection OpenCV Python With Source Code
The Volume Control With Hand Detection OpenCV Python was developed using Python OpenCV, In this Python OpenCV Project With Source Code we are going Building a Volume Controller with OpenCV , To change the volume of a computer.
We first look into hand tracking and then we will use the hand landmarks to find gesture of our hand to change the volume.
This project is module based which means we will be using a previously created hand module which makes the hand tracking very easy.
Building a Volume Controller with OpenCV can be accomplished in just 3 simple steps:
- Step 1. Detect Hand landmarks
- Step 2. Calculate the distance between thumb tip and index finger tip.
- Step 3. Map the distance of thumb tip and index finger tip with volume range. For my case, distance between thumb tip and index finger tip was within the range of 15 – 220 and the volume range was from -63.5 – 0.0.
What is OpenCV?
OpenCV is short for Open Source Computer Vision. Intuitively by the name, it is an open-source Computer Vision and Machine Learning library.
This library is capable of processing real-time image and video while also boasting analytical capabilities. It supports the Deep Learning frameworks.
In this Python OpenCV Project also includes a downloadable Python Project With Source Code for free, just find the downloadable source code below and click to start downloading.
By the way if you are new to python programming and you don’t know what would be the the Python IDE to use, I have here a list of Best Python IDE for Windows, Linux, Mac OS that will suit for you. I also have here How to Download and Install Latest Version of Python on Windows.
To start executing Volume Control With Hand Detection OpenCV Python With Source Code, make sure that you have installed Python 3.9 and PyCharm in your computer.
Volume Control With Hand Detection OpenCV Python With Source Code : Steps on how to run the project
Time needed: 5 minutes
These are the steps on how to run Volume Control With Hand Detection OpenCV Python With Source Code
- Step 1: Download the given source code below.
First, download the given source code below and unzip the source code.
- Step 2: Import the project to your PyCharm IDE.
Next, import the source code you’ve download to your PyCharm IDE.
- Step 3: Run the project.
last, run the project with the command “py main.py”
Installed Libraries
import cv2 import mediapipe as mp from math import hypot from ctypes import cast, POINTER from comtypes import CLSCTX_ALL from pycaw.pycaw import AudioUtilities, IAudioEndpointVolume import numpy as np
Complete Source Code
import cv2 import mediapipe as mp from math import hypot from ctypes import cast, POINTER from comtypes import CLSCTX_ALL from pycaw.pycaw import AudioUtilities, IAudioEndpointVolume import numpy as np cap = cv2.VideoCapture(0) mpHands = mp.solutions.hands hands = mpHands.Hands() mpDraw = mp.solutions.drawing_utils devices = AudioUtilities.GetSpeakers() interface = devices.Activate(IAudioEndpointVolume._iid_, CLSCTX_ALL, None) volume = cast(interface, POINTER(IAudioEndpointVolume)) volMin,volMax = volume.GetVolumeRange()[:2] while True: success,img = cap.read() imgRGB = cv2.cvtColor(img,cv2.COLOR_BGR2RGB) results = hands.process(imgRGB) lmList = [] if results.multi_hand_landmarks: for handlandmark in results.multi_hand_landmarks: for id,lm in enumerate(handlandmark.landmark): h,w,_ = img.shape cx,cy = int(lm.x*w),int(lm.y*h) lmList.append([id,cx,cy]) mpDraw.draw_landmarks(img,handlandmark,mpHands.HAND_CONNECTIONS) if lmList != []: x1,y1 = lmList[4][1],lmList[4][2] x2,y2 = lmList[8][1],lmList[8][2] cv2.circle(img,(x1,y1),4,(255,0,0),cv2.FILLED) cv2.circle(img,(x2,y2),4,(255,0,0),cv2.FILLED) cv2.line(img,(x1,y1),(x2,y2),(255,0,0),3) length = hypot(x2-x1,y2-y1) vol = np.interp(length,[15,220],[volMin,volMax]) print(vol,length) volume.SetMasterVolumeLevel(vol, None) # Hand range 15 - 220 # Volume range -63.5 - 0.0 cv2.imshow('Image',img) if cv2.waitKey(1) & 0xff==ord('q'): break
Output
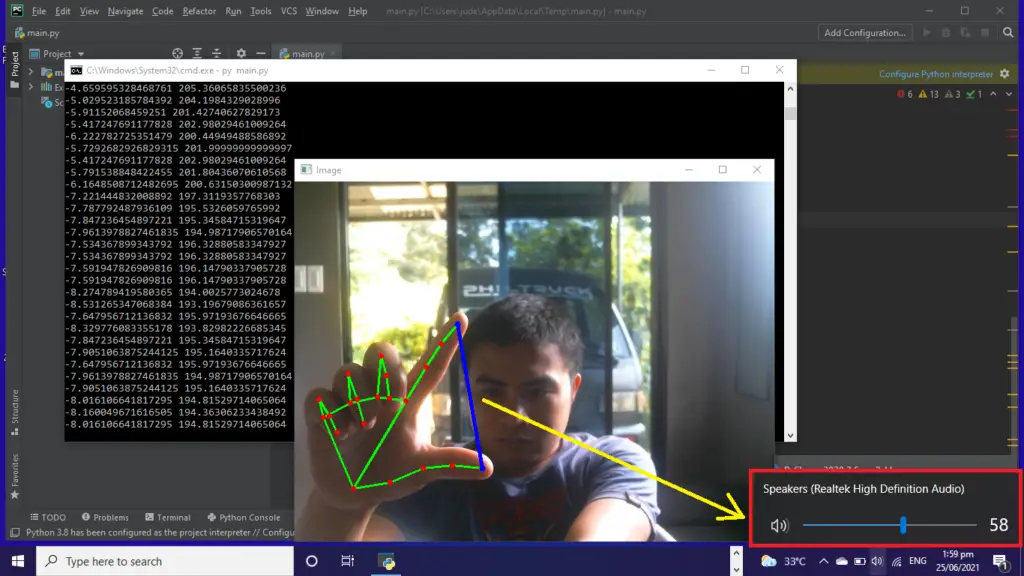
Volume Control With Hand Detection OpenCV Python: Project Information
Project Name: | Volume Control With Hand Detection OpenCV Python |
Language/s Used: | Python OpenCV |
Python version (Recommended): | 3.8 |
Database: | None |
Type: | Deep Learning |
Developer: | IT SOURCECODE |
Updates: | 0 |
Run Quick Virus Scan for secure Download
<a id="scan" class="classname" onclick="scan()">Run Quick Scan for secure Download</a>
Download Source Code below
Anyway, if you want to level up your programming knowledge, especially Python OpenCV, try this new article I’ve made for you Best OpenCV Projects With Source Code For Beginners 2021.
Summary
Gesture recognition helps computers to understand human body language. This helps to build a more potent link between humans and machines, rather than just the basic text user interfaces or graphical user interfaces (GUIs).
In this project for gesture recognition, the human body’s motions are read by computer camera. The computer then makes use of this data as input to handle applications.
The objective of this project is to develop an interface which will capture human hand gesture dynamically and will control the volume level.
For this, Deep Learning techniques such as Yolo model, Inception Net model+LSTM, 3-D CNN+LSTM and Time Distributed CNN+LSTM have been studied to compare the results of hand detection.
The results of Yolo model outperform the other three models. The models were trained using Kaggle and 20% of the videos available in 20 billion jester dataset.
After the hand detection in captured frames, the next step is to control the system volume depending on direction of hand movement.
The hand movement direction is determined by generating and locating the bounding box on the detected hand.
Related Articles
- Code For Game in Python: Python Game Projects With Source Code
- Best Python Projects With Source Code 2020 FREE DOWNLOAD
- How to Make a Point of Sale In Python With Source Code 2021
- Python Code For Food Ordering System | FREE DOWNLOAD | 2020
- Inventory Management System Project in Python With Source Code
Inquiries
If you have any questions or suggestions about Volume Control With Hand Detection OpenCV Python With Source Code, please feel free to leave a comment below.
which algorithm is used in this volume control using hand detection project?
can u have literally survive