In this article, we will learn about the PHP array_merge function with a detailed explanation as well as sample programs that can be used and applied to your projects.
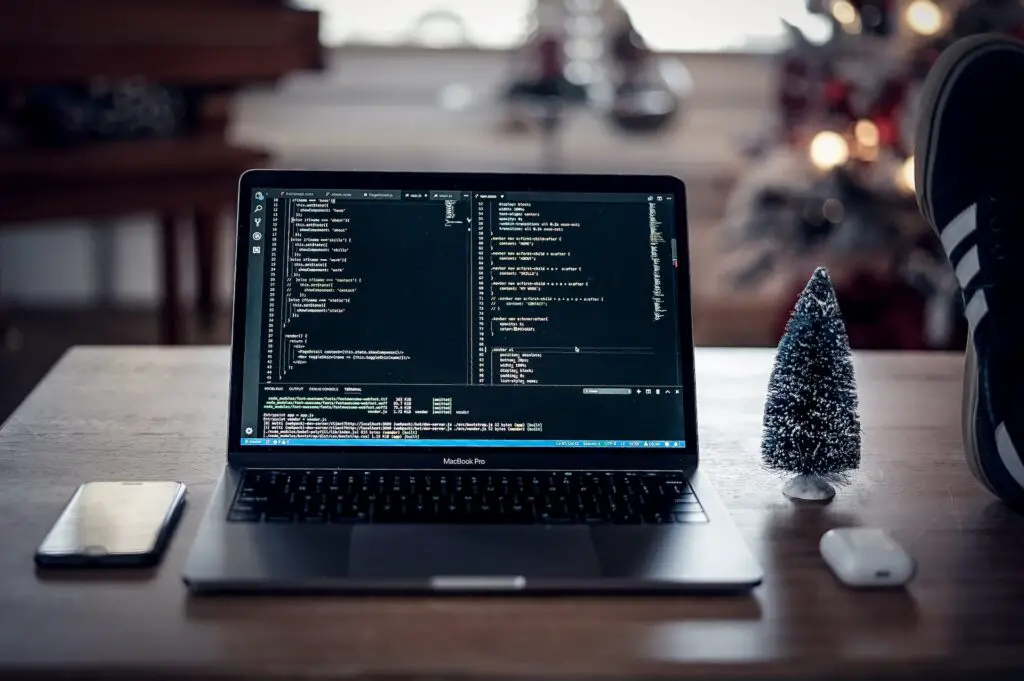
What is array_merge function in PHP?
In PHP, array_merge() is a built-in function which used to (merge
) two or more arrays into a single array.
In addition, the merging
occurs in a way in which the values of the single array were appended at the end of the previous array.
This function also takes a list of arrays which is separated by commas as a parameter that needs to be merged and returns a new set of arrays with a merged value of arrays passed as a parameter.
Syntax:
array_merge(array1, array2, array3, ...)
Parameter values:
Parameter | Description |
---|---|
array1 | Required. Specifies an array |
array2 | Optional. Specifies an array |
array3… | Optional. Specifies an array |
For example:
<?php
// php 7.4
$x = array("Glenn","Jude");
$y = array("Adones","Paul");
print_r(array_merge($x,$y));
?>
Output:
Array
(
[0] => Glenn
[1] => Jude
[2] => Adones
[3] => Paul
)
Merging two simple arrays in PHP
The array_merge() function can also pass two or more arrays, then the values of one array are appended at the end of the previous array. Once the two elements have the same key strings and then the latter value will override and match string keys.
Then integer keys will be rearranged which started to be from zero
.
For example:
<?php
// array 0
$my_array1 = array("name" => "big", 2,26 );
$my_array2 = array("x", "y", "name" => "Glenn Magada Azuelo",
"Position" => "Writer", 4);
$res = array_merge($my_array1, $my_array2);
print_r($res);
?>
Output:
Array
(
[name] => Glenn Magada Azuelo
[0] => 2
[1] => 26
[2] => x
[3] => y
[Position] => Writer
[4] => 4
)
Passing parameter with integer keys in PHP
The array_merge() function can also be passed a parameter with integer keys. Once the keys of the parameter are integer, then the output array will be rearranged starting from 0 and will increment for the next elements by 1.
For example:
<?php
$my_array = array(1 => "IT SOURCE CODE", 3=>"FOR", 2=>"INFORMATION TECHNOLOGY STUDENTS");
$pies = array_merge($my_array);
print_r($pies);
?>
Output:
Array
(
[0] => IT SOURCE CODE
[1] => FOR
[2] => INFORMATION TECHNOLOGY STUDENTS
)
PHP array_merge_recursive unique
The array_merge_recursive() is a function used to merge elements from one or more arrays together so that the (values
) of the one can be appended at the end of the previous one and will return a resulting array.
In addition, once the input arrays are the same string keys, then the values of all the keys are merged together into an (array), and the entire process is done recursively so that if one of the values contains an array itself, the function will automatically merge with its corresponding entry into another array too.
However, if the arrays contain the same numeric key the key will be renumbered, Then original value will not overwrite the previous value, but will be appended successfully and returns a new array.
Syntax:
array_merge_recursive(array ...$arrays): array
For example:
<?php
$ar1 = array("name" => array("Glenn Magada Azuelo" => "red"), 5);
$ar2 = array(10, "name" => array("Glenn Magada Azuelo" => "green", "blue"));
$result = array_merge_recursive($ar1, $ar2);
print_r($result);
?>
Output:
Array
(
[name] => Array
(
[Glenn Magada Azuelo] => Array
(
[0] => red
[1] => green
)
[0] => blue
)
[0] => 5
[1] => 10
)
Array merge multidimensional PHP
In PHP, merging a multidimensional array is very similar to a simple array
. It will only take two arrays as input and merge them.
Furthermore, the multidimensional array index is automatically assigned in an increment order and automatically appended after the parent array elements.
Now let’s see three example programs below.
First example:
<?php
$arr = [
[
'name' => 'Glenn Magada Azuelo',
'address' => 'Philippines'
]
];
$arr2 = [
[
'name' => 'Angel Jude Suarez',
'address' => 'Philippines'
]
];
print_r(array_merge($arr, $arr2));
Output:
Array
(
[0] => Array
(
[name] => Glenn Magada Azuelo
[address] => Philippines
)
[1] => Array
(
[name] => Angel Jude Suarez
[address] => Philippines
)
)
Second example:
<?php
$permanent_location = [
'employee name' => [
'address' => 'Glenn Magada Azuelo'
]
];
$employment_location = [
'employee name' => [
'address' => 'PH'
]
];
print_r(array_merge($permanent_location, $employment_location));
Output:
Array
(
[employee name] => Array
(
[address] => PH
)
)
Third example:
<?php
$permanent_location = [
'employeehome' => [
'address' => 'PH'
]
];
$employment_location = [
'employeecurrent' => [
'address' => 'PH'
]
];
print_r(array_merge($permanent_location, $employment_location));
Output:
Array
(
[employeehome] => Array
(
[address] => PH
)
[employeecurrent] => Array
(
[address] => PH
)
)
How can I connect two array in PHP?
The array_merge() function is the best way to connect two arrays and can also be used to merge two or more arrays into one array with numeric keys
. In addition, this function is also used to merge elements or values of two or more arrays together in one key starting array.
PHP array_merge vs spread
In PHP, the spread operator provides a better performance rather than array_merge() function. For the reason of the spread, union operator is a language structure while the array_merge
is purely a function.
More About Array
- Not in Array PHP (With Advanced Program Examples)
- Sort An Array PHP (With Example Programs)
- PHP Echo Array with Example
- PHP File To Array With Program Examples
- PHP Remove Element From Array With Examples
Summary
This article discussed the array_merge and also tackles what is array_merge function, merging two simple arrays, passing parameters with integer keys, PHP array_merge_recursive unique, array merge multidimensional PHP, and how can I connect two arrays, and array_merge vs spread.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.