When you start your web development journey, deciding between TypeScript and JavaScript can make a big difference.
In this article, we’ll talk about the differences between JavaScript vs TypeScript, which are two popular programming languages with different purposes and characteristics.
We’ll explore their features, uses, and how they compare in various ways.
What is a Typescript?
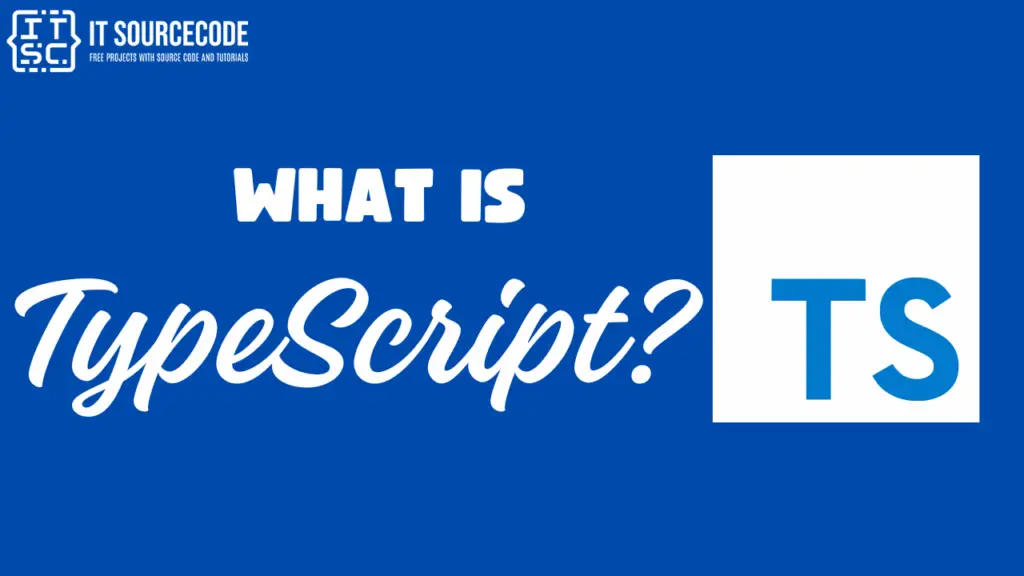
- TypeScript is a high-level programming language that is both free and open-source and was created by Microsoft.
- It is a superset of JavaScript, which means any valid JavaScript code is also valid TypeScript code.
- However, TypeScript introduces optional static typing and other features that make it a powerful tool for building large-scale applications.
What is in Typescript?
TypeScript is a language that extends JavaScript by adding types. It’s like an upgraded version of JavaScript.
It allows you to specify the kind of data each part of your code should handle.
Here are some key :
Static Typing
It enhances JavaScript by introducing static typing.
This allows developers to define the types of variables, function parameters, and return values.
It helps in catching errors early during the editing process.
For example:
function greet(name: string) {
return `Hello, welcome to${name}!`;
}
console.log(greet("Itsourcecode"));
console.log(greet(123));
Output:
Hello, welcome to Itsourcecode!
Error:
Argument of type 'number' is not assignable to parameter of type 'string'.
Optional Typing
It offers the versatility of opting for static typing or not.
This implies that you have the freedom to define types for variables and function parameters, or you can allow TypeScript to deduce the types on its own, depending on the value given.
For example:
let age: number;
age = 18; // Valid assignment
// age = "18"; // Optional typing allows this, but if uncommented, TypeScript will raise a type error
console.log(age);
Output:
Output: 25
Type Inference
It is designed to understand JavaScript and uses type inference to provide excellent tooling without needing extra code.
For example:
let message = "Hi, Itsourcecode!";
console.log(message.toUpperCase());
TypeScript infers the type of “message” as a string automatically. There’s no need to explicitly specify the type
Output:
HI, ITSOURCECODE!
Compile-Time Type Checking
TypeScript checks if the specified types match before the code is run, not while it’s running. This is known as compile-time type checking.
For example:
function add(x: number, y: number) {
return x + y;
}
console.log(add(50, 30));
// console.log(add("5", 3)); // Error: Argument of type 'string' is not assignable to parameter of type 'number'.
Output:
80
Transpiles to JavaScript
TypeScript code is converted into JavaScript, which can be run anywhere JavaScript is used, like in a browser, on Node.js or Deno, and in your apps.
For example:
function greet(name: string) {
return `Hi, ${name}!`;
}
console.log(greet("Itsourcecode")); // Output: Hi, Itsourcecode!
After compiling using TypeScript compiler (tsc), the above code will be converted to JavaScript:
function greet(name) {
return "Hi, " + name + "!";
}
console.log(greet("Itsourcecode"));
Output:
Hi, Itsourcecode!
Improved Tooling
It offers enhanced tooling at any scale, including features like autocompletion, type checking, and advanced refactoring.
Interfaces and Types
TypeScript lets you define the structure of objects and functions in your code, making it easier to see documentation and issues in your editor.
For example:
interface Person {
name: string;
age: number;
}
function greet(person: Person) {
return `Hi, ${person.name}!`;
}
let user = { name: "Itsourcecode", age: 18};
console.log(greet(user));
Output:
Hi, Itsourcecode!
TypeScript Advantages
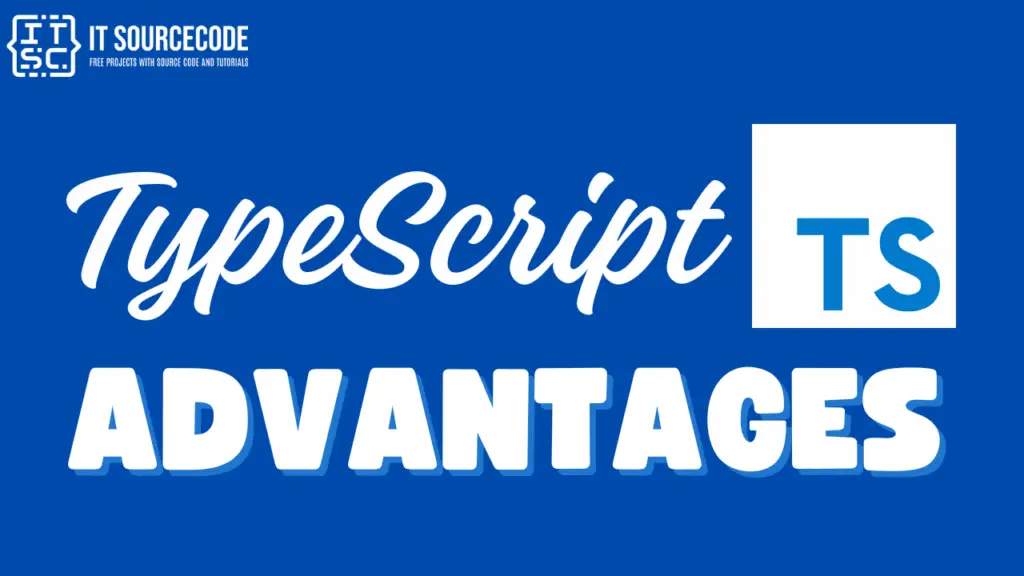
Here are the following advantages of TypeScript:
✅ Type Safety
- TypeScript enforces static typing, which means you explicitly define the data types of variables.
- During compilation, TypeScript catches type-related errors, preventing issues at runtime.
- This leads to more reliable code and better maintenance.
✅ Improved Tooling
- TypeScript provides enhanced tooling support in development environments (IDEs).
- Features like code completion, refactoring, and navigation are more accurate.
- Developers can work more efficiently and catch errors early.
✅ Scalability
- For large projects, TypeScript’s type system helps manage complexity.
- As codebases grow, maintaining consistency becomes crucial.
- TypeScript allows multiple developers to collaborate effectively.
✅ Interfaces and Generics
- TypeScript introduces interfaces and generics.
- Interfaces define clear contracts for objects, ensuring consistency.
- Generics enable reusable components by allowing flexible type parameters.
✅ Compatibility
- TypeScript seamlessly integrates with existing JavaScript libraries and frameworks.
- Whether you’re using React, Vue, or Angular, TypeScript plays well with others.
TypeScript Disadvantages
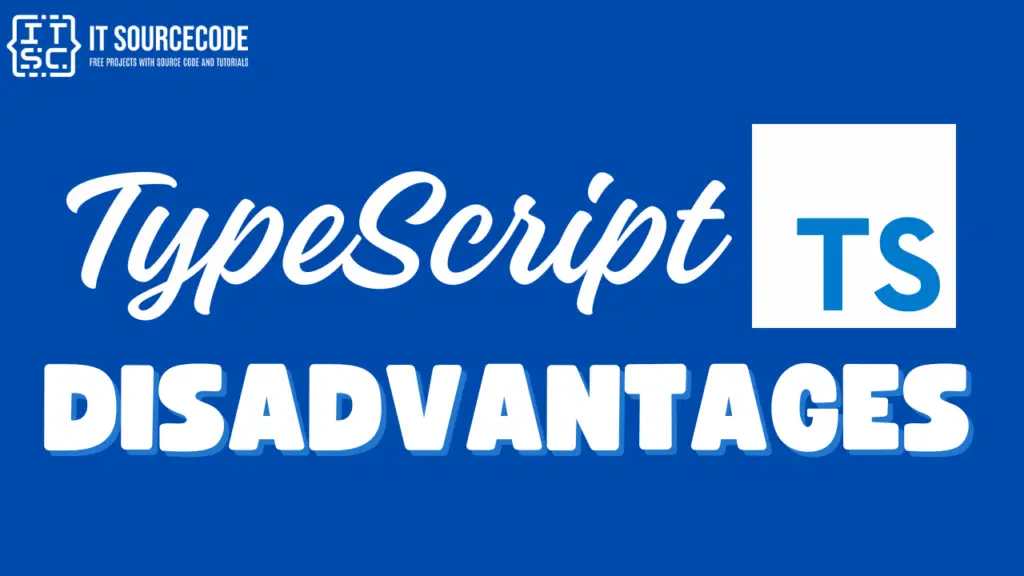
Here are the following disadvantages of TypeScript:
❌ Learning Curve
- Developers familiar with JavaScript may find TypeScript’s type system challenging initially.
- Understanding type annotations, interfaces, and other features takes time.
❌ Increased Compile Time
- TypeScript requires compilation to JavaScript.
- This extra step can slow down development cycles compared to pure JavaScript.
❌ Smaller Ecosystem
- While TypeScript’s ecosystem is growing, it’s still smaller than JavaScript’s.
- Some libraries and tools may lack official TypeScript support.
❌ Integration Challenges
- Integrating TypeScript into an existing project can be complex.
- Especially if the project heavily relies on dynamic typing, transitioning can be tricky.
TypeScript offers benefits like type safety and improved tooling, but it’s essential to consider the learning curve and integration challenges.
What is JavaScript?
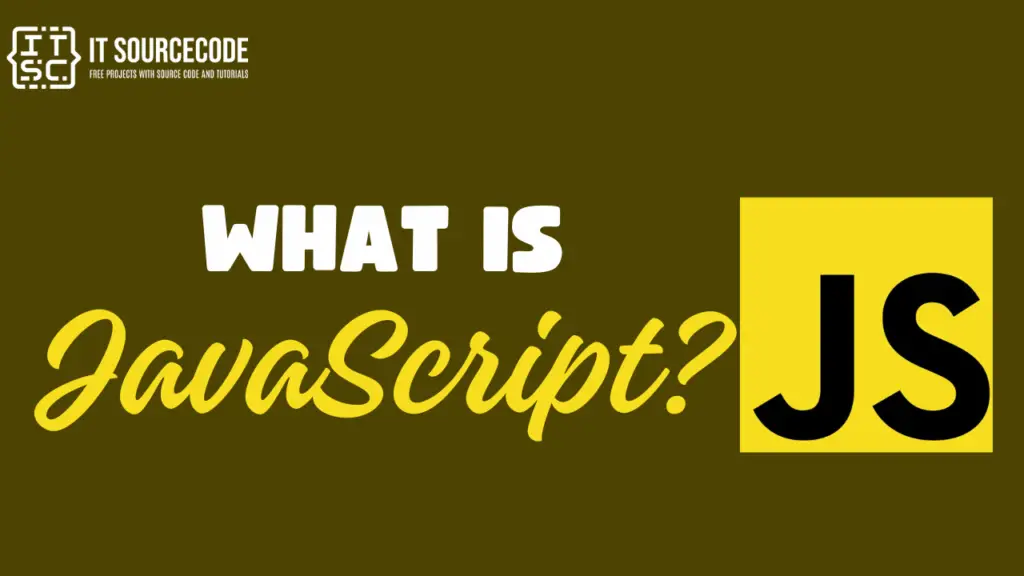
- JavaScript is a computer programming language that gives developers and programmers the power to make web pages dynamic and interactive.
- JavaScript is a widely used language that works alongside HTML and CSS for web development.
- It has been the go-to choice for many years, and major web browsers have dedicated engines to run JavaScript code.
- JavaScript is a versatile programming language that is often described as lightweight. It can be interpreted or just-in-time compiled, and it boasts first-class functions.
- It is commonly recognized as the scripting language for web pages, it has expanded its reach to non-browser environments like Node.js, Apache CouchDB, and Adobe Acrobat.
- It is known for making websites interactive and responsive to users’ actions. It has built-in libraries and frameworks on top of JavaScript, enhancing its functionality.
- JavaScript is a widely used scripting language often called the “language of the web.” Brendan Eich created it in year 1995.
JavaScript Syntax
JavaScript offers a syntax that is more adaptable and forgiving.
It is a dynamically typed language, which means you don’t have to explicitly declare variable types.
Here’s an illustration:
function greet(data) {
console.log("Hi, " + data + "!");
}
var name = "It Sourcecode";
greet(name);
Output:
Hi, It Sourcecode!
JavaScript Advantages
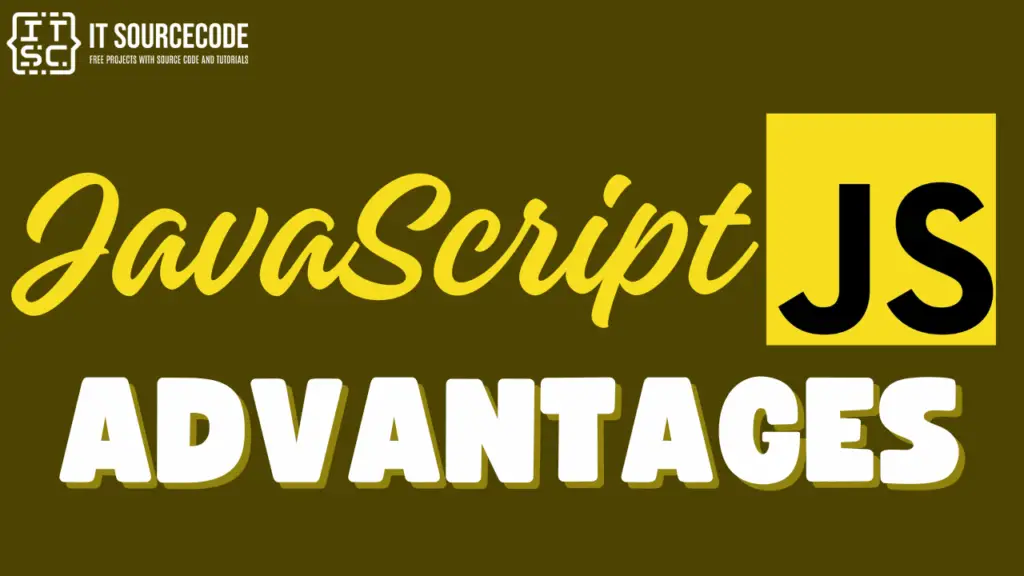
Here are the advantages of JavaScript:
✅ JavaScript is known for its high speed since it is commonly executed directly within the user’s web browser.
✅ It is used by many developers and programmers all around the world.
✅ The availability of libraries and frameworks makes it easy for developers to choose the functions they need the most while creating web apps, mobile apps, and other digital products.
✅ It is compatible with all major web browsers and almost all other web browsers.
✅ JavaScript executes on the client-side instead of the backend, helping to save bandwidth.
✅ JavaScript enables interactive and dynamic web pages, enhancing user experience.
✅ It has a vast ecosystem of libraries and frameworks, enabling faster development.
✅ It supports asynchronous programming, improving performance.
✅ It can be used for server-side development with Node.js, promoting code reuse.
✅ JavaScript’s flexibility allows for quick prototyping and experimentation.
JavaScript Disadvantages
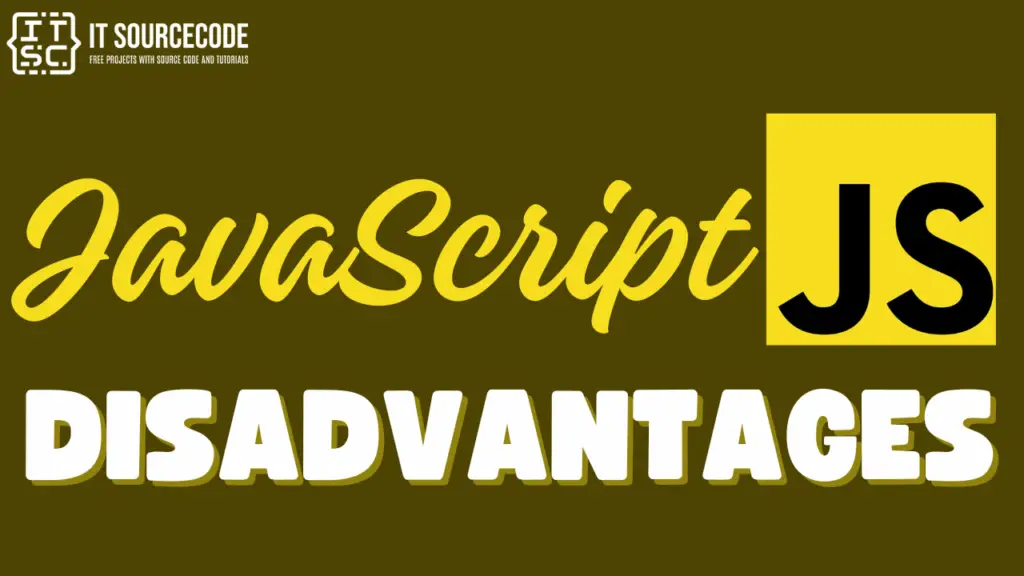
Here are the disadvantages of JavaScript:
❌ Large projects demand significant effort for configuration.
❌ The Document Object Model (DOM) interprets JavaScript at a slower pace compared to HTML and WebAssembly (Wasm).
❌ User security may be compromised due to potential vulnerabilities on the client-side. Consequently, some individuals opt to disable JavaScript altogether.
❌ JavaScript behavior may vary across different browsers, requiring compatibility testing.
❌ On the client side can be susceptible to security vulnerabilities.
❌ JavaScript’s interpreted nature may result in slower performance for certain tasks.
❌ Debugging JavaScript can be challenging due to runtime errors.
❌ JavaScript’s flexibility and quirks can make it challenging for beginners to learn.
It’s important to think about the advantages and disadvantages of using JavaScript for web development to ensure that your applications are efficient and secure.
JavaScript vs. TypeScript Difference
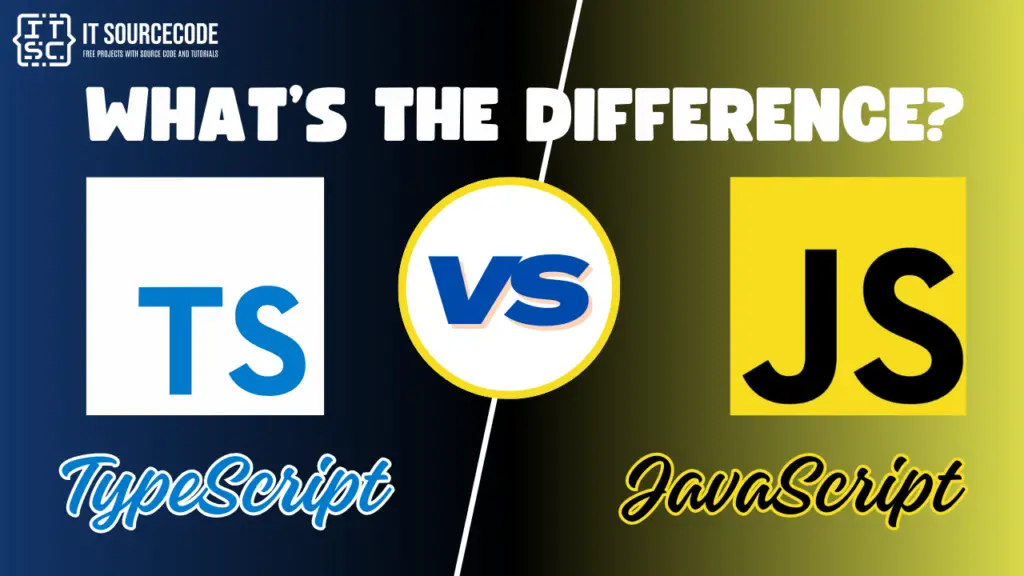
TypeScript, developed by Microsoft, is a superset of JavaScript. It offers static type checking and other features that enhance code quality and maintainability.
On the other hand, JavaScript is more flexible but lacks some of TypeScript’s advantages.
We know that TypeScript offers more features than JavaScript. TypeScript is a modern-age language – a syntactical superset of JavaScript, whereas JavaScript is a scripting language – a subset of TypeScript.
Web Development:
TypeScript | TypeScript is increasingly favored for large-scale web applications. Its type-checking capabilities provide enhanced developer confidence and maintainability. |
JavaScript | JavaScript remains foundational for client-side scripting in web browsers. It powers interactive elements and enhances user interfaces across websites. |
Backend Services:
TypeScript | Similarly, TypeScript can be used with Node.js, offering the added benefit of type safety and improved scalability in complex backend systems. |
JavaScript | Node.js enables JavaScript to run server-side, facilitating backend services and APIs with its asynchronous event-driven architecture. |
Mobile Applications
TypeScript | React Native also supports TypeScript, providing the same advantages of type safety and code structure for mobile development projects. |
JavaScript | Frameworks like React Native allow developers to build cross-platform mobile applications using JavaScript, leveraging its extensive library ecosystem. |
Conclusion
Choosing between TypeScript and JavaScript depends on the specific needs and goals of your development project.
While JavaScript remains versatile and widely adopted, TypeScript offers additional benefits such as type safety and improved code structure, particularly for larger and more complex applications.
I hope this article has given you some insights and helped you understand the difference between JavaScript vs. TypeScript.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.