Are you curious what’s the difference between library vs. module vs. package in Python?
So, let’s dive in and demystify the difference between Python modules, packages, and libraries along with examples.
What is a Python Module?
A module is like a container that holds different useful things such as:
- functions
- classes
- variables
- constants
It’s a way to package these elements together so they can be easily used by many different programs.
Rather than creating a separate program just to store these elements, a module can be included within another program.
This makes it convenient to reuse code across different projects.
Basically, a module in Python is just a file with a .py extension that contains Python code.
For example, let’s define a Python executable function to greet our visitors to a certain event:
def greeting_message(event_name):
print("Welcome guest to our " + event_name+ ". Before proceeding, let's take a pause and watch the beginning of the show.")
This code is saved in a file called “welcome.py” because we want this function to be part of the “welcome” module.
We need to import the module using the import statement before we can use the code in our application.
We can call the function defined in the module by using the module.function() syntax.
import welcome
welcome.greeting_message (“Python Module Tutorial”)
Output:
Welcome guest to our Python Module Tutorial.
It’s possible to import only a specific function from a module, rather than the entire module.
To do this, you can use the following syntax:
from welcome import greeting_message
If you’ve used Python before, it’s likely that you’ve used modules.
For instance, you might have used:
- random module – a module that can be used to make pseudo-random number generators for different distributions.
- datetime module – You can change the date and time with the datetime module.
- html module – enables you to view HTML pages.
- re module – Python allows you to recognize and work with regular expressions.
In order for you to test your Python code provided in this lesson, you must test the code on your code editor like PyCharm.
But if you wish to run this code online, we also have an Online Compiler in Python or interpreter for you to test your Python code for free.
What is a Python Package?
The package is a directory containing collections of modules, including an __init__.py file that tells the interpreter to treat it as a package.
Additionally, a package is simply a namespace that can also contain sub-packages and modules.
For example, we can put modules related to our data science project in the following package:
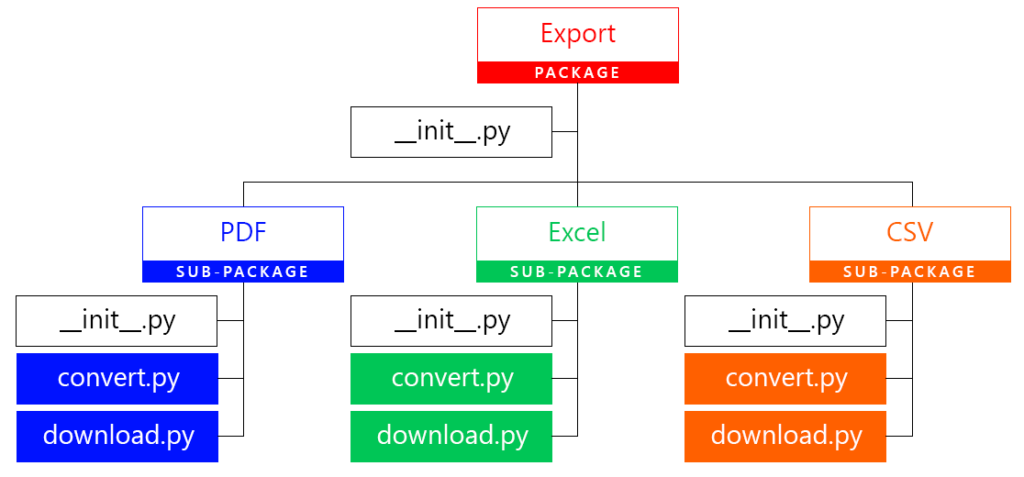
The dot notation lets us import only certain modules from this package.
For example, we can use one of the following code snippets to import the download module from the Excel sub-package above:
import export.excel.download
or
from export.excel import download
Next, we could choose to import only the file_download() function from our download.py module. Either of these options will work:
import export.excel.download.load_dataset()
or
from export.excel.download import file_download()
You probably already use a lot of the built-in and open-source Python packages.
For instance:
As your application gets bigger and uses more modules, Python packages become an important part of optimizing the structure of your code.
Creating a Package
We have included an __init__.py file inside a directory to tell Python that this directory is a package.
You need to include this file whenever you want to create a package. Depending on your preferences, you can either write code inside of it or leave it blank.
To make a package in Python, follow the steps below.
- Make a directory and put an __init__.py file in it to tell Python that the directory is a package.
- Include any additional files or sub-packages that you desire.
- Next, use the valid import statements to get to them.
What is a Python Library?
A library is a collection of code, usually related modules and packages. This term is often used conversely with the “Python package”.
However many people think that a package is a collection of modules and that a library is a collection of packages.
Developers create Python libraries to share code with others. This way, they don’t have to write code from scratch every time they need a specific function.
Today, there are a lot of useful libraries to choose from. I’ll give just a few examples:
Python Standard Library
The Python Standard Library is a collection of Python’s core syntax, tokens, and semantics. It comes bundled with the Python distribution.
Python is a language that is written in C and handles core functionality like I/O and other modules.
The core of the standard library is made up of more than 200 core modules. This library is already part of Python.
Difference between Python Modules, Packages and Libraries
A library in Python is a collection of modules or packages that provide specific functionality for various tasks.
Modules are single files containing Python code that define functions, classes, and variables, which can be imported and used in other programs.
Packages are a way of organizing related modules into a directory hierarchy, allowing for better organization and avoiding naming conflicts.
Packages typically consist of multiple modules and sub-packages and are represented by directories with an init.py file.
Overall, libraries encompass multiple modules or packages, modules are individual code files, and packages are a way to organize and structure related modules in a directory hierarchy.
Summary
This tutorial has discussed library vs module vs package Python differences. I have high hopes that you will acquire new knowledge from reading this article.
The next post, “File Handling Functions in Python“, consists of the basic functions regarding inputs and outputs used in Python Programming.