In this deque Python tutorial, we will learn to understand how deque works in Python with some examples. We will also answer some questions regarding deque.
What is deque in Python?
Deque (double-ended queue) is where elements can be inserted and removed from either the left or right end. The collections module in Python provides an implementation of a deque.
When we need faster append and pop operations from both ends of the container, deque is preferred over a list because deque has an O(1) time complexity for append and pop operations, whereas a list has an O(n) time complexity.
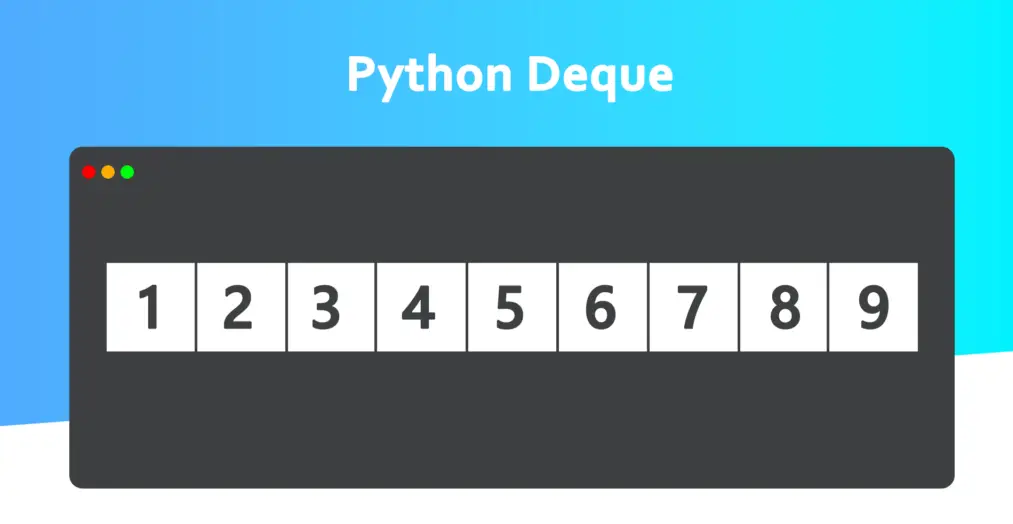
Various Operations on Python deque
Below are the different operations on the deque:
Appending Items Efficiently
To append items in Python deque we will use the following functions:
- append() – The value in its argument is inserted to the right end of the deque using this function.
- appendleft() – This function inserts the value in its argument to the deque’s left end.
import collections as col
sample_de = col.deque([1, 2, 3])
print("queue: ", sample_de)
# This function will append to the queue's end.
sample_de.append(4)
print("\nappend on the right side:", sample_de)
# This function will append to the beginning of the queue.
sample_de.appendleft(5)
print("append on the left side: ", sample_de)
Output:
queue: deque([1, 2, 3])
append on the right side: deque([1, 2, 3, 4]) append on the left side: deque([5, 1, 2, 3, 4])
Popping Items Efficiently
To pop an element or items in Python deque we will use the following functions:
- pop() – This function is used to remove an argument from the right end of the deque.
- popleft() – Use this function to remove an argument from the left end of the deque.
import collections as col
sample_de = col.deque([1, 2, 3])
print("list of items:", sample_de)
# This function will pop the last item..
sample_de.pop()
print("\npop the item on the right side:", sample_de)
# This function will pop first item.
sample_de.popleft()
print("pop the item on the left side: ", sample_de)
Output:
list of items: deque([1, 2, 3])
pop the item on the right side: deque([1, 2])
pop the item on the left side: deque([2])
Accessing Items in a deque
The following functions are used to access items in a deque:
- index(value, beg, end) – This function searches from the beg index to the end index to find the index position of the value given in the arguments.
- insert(index, argument) – This function inserts the value at the index given in arguments.
- remove() – This function removes the first appearance of the given value in the arguments.
- count() – This function counts the number of mentioned values in the arguments.
import collections as col
sample_de = col.deque([1, 2, 3, 4, 5, 6, 3])
print(sample_de)
# insert the value 3 at 5th index position
sample_de.insert(4, 3)
print("Inserting 3 at the 5th position:", sample_de)
# count the occurrences of 3
print("The number of occurrence of 3:", sample_de.count(3), "times")
# remove the first occurrence of 3
sample_de.remove(3)
print("delete the first occurrence of 3 is:", sample_de)
Output:
deque([1, 2, 3, 4, 5, 6, 3])
Inserting 3 at the 5th position: deque([1, 2, 3, 4, 3, 5, 6, 3])
The number of occurrence of 3: 3 times
delete the first occurrence of 3 is: deque([1, 2, 4, 3, 5, 6, 3])
Different operations on deque
- extend
(
iterable)
– This function is used to add more than one value to the end of the deque on the right. The argument passed is iterable. - extendleft
(
iterable)
– In this function, you can add more than one value to the beginning of the deque. The argument passed is iterable. Because of the appends on the left, the order is backward. - reverse() – This function is used to reverse the order of elements in the deque.
- rotate() – This function rotates the deque by the number specified in arguments. If the number specified is negative, rotation occurs to the left. Else rotation is to right.
import collections as col
sample_de = col.deque([1, 2, 3, 4, 5, 6, 7])
print(sample_de)
sample_de.extend([8, 9, 10])
print("extending deque at end is:", sample_de)
# add the value 4 5 6 at the beginning of the deque in reverse
sample_de.extendleft([0, -1, -2, -3])
print("extending deque at beginning is:", sample_de)
# using reverse() to reverse the deque
sample_de.reverse()
print("reversing deque is:", sample_de)
# rotates by 4 to right
sample_de.rotate(4)
print("rotating deque by 4 to the right is:", sample_de)
Output :
deque([1, 2, 3, 4, 5, 6, 7])
extending deque at end is: deque([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
extending deque at beginning is: deque([-3, -2, -1, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
reversing deque is: deque([10, 9, 8, 7, 6, 5, 4, 3, 2, 1, 0, -1, -2, -3])
rotating deque by 4 to the right is: deque([0, -1, -2, -3, 10, 9, 8, 7, 6, 5, 4, 3, 2, 1])
Removing any item in a deque in Python
We know that the operation remove() is used to remove items in the deque. But there is also another method to remove items in a deque.
Example:
In this example, we’re going to use the del function:
import collections as col
sample_de = col.deque([1, 2, 3, 4, 5, 6, 7])
print(sample_de)
del sample_de[4]
print("Deleted the 4th index in deque:",sample_de)
Output:
deque([1, 2, 3, 4, 5, 6, 7])
Deleted the 4th index in deque: deque([1, 2, 3, 4, 6, 7])
The del function removes an element at a specified index. In the case of the example above, del removes the 4th index in a deque, which is “5”. After removing the 4th index, the program prints out the deque.
Retrieving an item in Python deque
In our previous examples, we learned how to append, pop, remove, insert, extend, rotate, and delete an item/s in a deque. But how about retrieving an item in a deque?
To retrieve an item in a deque check the following code below:
import collections as col
sample_de = col.deque([1, 2, 3, 4, 5, 6, 7])
print(sample_de)
retrieve_item = sample_de[4]
print("Retrieving the 4th index in deque:",retrieve_item)
Output:
deque([1, 2, 3, 4, 5, 6, 7])
Retrieving the 4th index in deque: 5
In the above example, we declare a variable called retrieve_item and inside that variable, we retrieve the 4th index in the deque.
Sort a Deque in Python
Sorting the order of the values in Deque is possible using the sorted() function. It can sort both string and numeric data. The sorted() function also helps sort any collection or sequence.
This takes the collection object or sequence as a parameter inside the deque and gives back a sorted output.
Below is the example code of how to sort a Deque using the built-in sorted() function.
import collections as col
sample_de = col.deque([1, 7, 5, 6, 4, 3, 2])
print("Original Deque:",sample_de)
print("Sorted Deque:",sorted(sample_de))
print("Sorted Deque in Descending:",sorted(sample_de, reverse=True))
Output:
Original Deque: deque([1, 7, 5, 6, 4, 3, 2])
Sorted Deque: [1, 2, 3, 4, 5, 6, 7]
Sorted Deque in Descending: [7, 6, 5, 4, 3, 2, 1]
Some of the things about the sorted() function is shown here:
- The sorted() function is a built-in function, it is available under the standard Python installation which is efficient.
- The default of the sorted function is ascending order, which means it will sort from smallest to largest.
The differences between deques and lists in terms of performance are: Deques perform appendleft() and popleft() in O(1) time, whereas lists perform insert (0, value) and pop(0) in O(n) time.
So, in terms of performance, the deque Python is better.
Note that one problem with deque is that it takes a long time to get to things in the middle.
The Python queue is a built-in library that lets you make a list that follows the “first in, first out” or FIFA rule. Python deque is also a built-in library that uses the opposite rule, LIFO queue, or “last in, first out”.
The popleft() in Python is used to remove or delete the first element from the queue.
Additionally, according to the official Python Documentation, popleft() remove and return an element from the left side of the deque. If no elements are present, raises an IndexError.
To use or implement a deque module, you need to access it in the “collections” library and declare the deque by assigning a name. And we do not need any classes to implement this built-in method because it can be implemented directly.
Summary
In summary, deque in Python can be inserted and removed from either the left or right end of the queue. And comparing the list and deque in terms of performance, then deque will be the best option.
Lastly, if you want to learn more about how to use deque in Python, please leave a comment below. We’ll be happy to hear it!